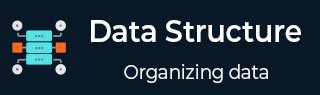
- 数据结构与算法
- DSA - 首页
- DSA - 概述
- DSA - 环境搭建
- DSA - 算法基础
- DSA - 渐近分析
- 数据结构
- DSA - 数据结构基础
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表数据结构
- DSA - 双向链表数据结构
- DSA - 循环链表数据结构
- 栈与队列
- DSA - 栈数据结构
- DSA - 表达式解析
- DSA - 队列数据结构
- 搜索算法
- DSA - 搜索算法
- DSA - 线性搜索算法
- DSA - 二分搜索算法
- DSA - 插值搜索
- DSA - 跳跃搜索算法
- DSA - 指数搜索
- DSA - 斐波那契搜索
- DSA - 子列表搜索
- DSA - 哈希表
- 排序算法
- DSA - 排序算法
- DSA - 冒泡排序算法
- DSA - 插入排序算法
- DSA - 选择排序算法
- DSA - 归并排序算法
- DSA - 希尔排序算法
- DSA - 堆排序
- DSA - 桶排序算法
- DSA - 计数排序算法
- DSA - 基数排序算法
- DSA - 快速排序算法
- 图数据结构
- DSA - 图数据结构
- DSA - 深度优先遍历
- DSA - 广度优先遍历
- DSA - 生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树的遍历
- DSA - 二叉搜索树
- DSA - AVL树
- DSA - 红黑树
- DSA - B树
- DSA - B+树
- DSA - 伸展树
- DSA - 字典树
- DSA - 堆数据结构
- 递归
- DSA - 递归算法
- DSA - 使用递归实现汉诺塔
- DSA - 使用递归实现斐波那契数列
- 分治法
- DSA - 分治法
- DSA - 最大最小问题
- DSA - Strassen矩阵乘法
- DSA - Karatsuba算法
- 贪心算法
- DSA - 贪心算法
- DSA - 旅行商问题(贪心法)
- DSA - Prim最小生成树
- DSA - Kruskal最小生成树
- DSA - Dijkstra最短路径算法
- DSA - 地图着色算法
- DSA - 分数背包问题
- DSA - 带截止时间的作业排序
- DSA - 最优合并模式算法
- 动态规划
- DSA - 动态规划
- DSA - 矩阵链乘法
- DSA - Floyd-Warshall算法
- DSA - 0-1背包问题
- DSA - 最长公共子序列算法
- DSA - 旅行商问题(动态规划法)
- 近似算法
- DSA - 近似算法
- DSA - 顶点覆盖算法
- DSA - 集合覆盖问题
- DSA - 旅行商问题(近似算法)
- 随机化算法
- DSA - 随机化算法
- DSA - 随机化快速排序算法
- DSA - Karger最小割算法
- DSA - Fisher-Yates洗牌算法
- DSA有用资源
- DSA - 问答
- DSA - 快速指南
- DSA -有用资源
- DSA - 讨论
数据结构中的字符串
什么是字符串?
字符串是一种用于存储字符序列的原始数据结构类型。它通常用于存储、操作和处理文本,例如用户输入、消息、标签等。每种编程语言都有自己独特的字符串表示规则。例如,在Java中,字符串被视为对象,而在C中,它表示为char数据类型的数组。在下图中,我们可以看到一个字符串:
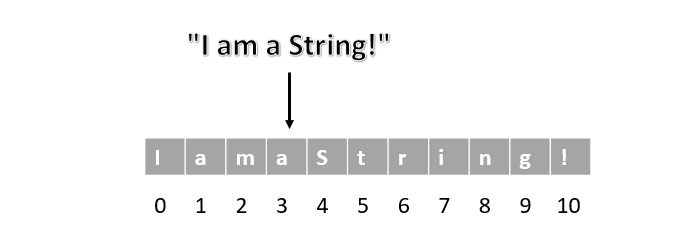
在本教程中,我们将探讨字符串的一些属性和操作,以及它们如何在不同的编程语言中实现。
语法
在C编程语言中创建字符串的语法:
char string_name[string_size] = {chars within single quotes and separated by commas}; or, char string_name[string_size] = "string in double quotes";
除了上述语法外,C++还提供了一种创建字符串的替代方法:
string string_name = "string in double quotes";
在Java编程语言中创建字符串:
String string_name = "string in double quotes"; or, String string_name = new String("values");
在Python编程语言中创建字符串:
string_name = "string in double quotes"
字符串的必要性
字符串是应用程序与用户交互最简单且最常用的方式。它们易于理解和使用,因为它们基于人类可读的字符。此外,它们还用于存储各种数据,例如文本、数字、符号、二进制、十六进制等。
字符串表示
与数组类似,字符串也表示为存储桶的集合,每个存储桶存储一个字符。这些存储桶的索引从“0”到“n-1”,其中n是该特定字符串的长度。例如,大小为10的字符串将具有从0到9的索引存储桶。下图说明了字符串是如何表示的:
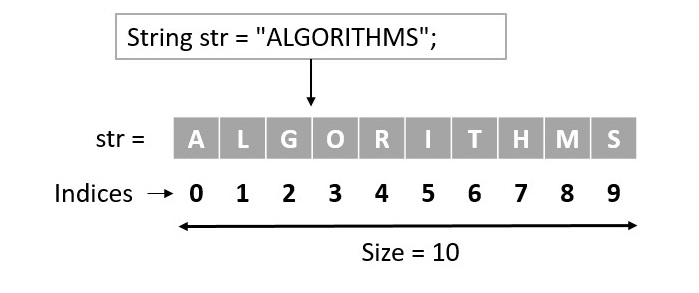
以下是上述表示的一些要点。
索引总是从0开始。
如果索引从0到9,则表示字符串有10个元素。
可以通过其索引访问每个字符。
字符串的基本操作
可以对字符串执行许多操作,例如搜索、分割、修剪、索引等等。每种编程语言都有自己的一套内置函数或方法,可以轻松地执行这些操作。
以下是可对给定字符串执行的基本操作:
- 连接 - 将两个或多个字符串连接在一起。
- 长度 - 打印字符串中的字符数。
- 子字符串 - 查找更大字符串的一部分。
- 反转 - 反向打印字符串字符。
- 索引 - 使用其索引访问特定字符。
连接操作
连接操作是将两个或多个字符串连接在一起以形成新字符串的过程。例如,将两个字符串“Tutorials”和“Point”连接在一起将得到“TutorialsPoint”。这可以使用不同的运算符或方法来完成,具体取决于编程语言。
算法
以下是连接两个字符串的算法。
1. Start 2. Declare and Initialize two strings. 3. Perform concatenation. 4. Print the result. 5. Stop
示例
在这里,我们看到连接操作的实际实现,我们使用两个不同的字符串来形成一个新的字符串:
#include <stdio.h> #include <string.h> int main(){ // defining two strings char sOne[15] = "Tutorials"; char sTwo[15] = "Point"; // concatenating the strings strcat(sOne, sTwo); // printing the result printf("New String: %s\n", sOne); return 0; }
#include <iostream> #include <string> using namespace std; int main() { // defining two strings string sOne = "Tutorials"; string sTwo = "Point"; // concatenating the strings string newStr = sOne + sTwo; // printing the result cout <<"New String: " << newStr << endl; }
public class ConctStr { public static void main(String []args){ // defining two strings String sOne = "Tutorials"; String sTwo = "Point"; // concatenating the strings String newStr = sOne + sTwo; // printing the result System.out.println("New String: " + newStr); } }
# defining two strings sOne = "Tutorials" sTwo = "Point" # concatenating the strings newStr = sOne + sTwo # printing the result print("New String: " + newStr)
输出
New String: TutorialsPoint
查找字符串的长度
查找字符串的长度意味着打印该字符串中的字符数。例如,字符串“Tutorix”的长度为7。字符串的长度可用于迭代其字符、访问给定索引处的特定字符或从原始字符串中切片子字符串。
算法
查找字符串长度的算法如下:
1. Start 2. Declare and Initialize a string. 3. Find the number of characters. 4. Print the result. 5. Stop
示例
以下是此操作在各种编程语言中的实现:
#include <stdio.h> #include <string.h> int main() { // the string given as char name[] = "tutorialspoint"; // loop to find the length of given string int strLength = 0; while (name[strLength] != '\0') { strLength++; } // to print the result printf("The length of given string is: %d\n", strLength); return 0; }
#include <iostream> #include <string> using namespace std; int main() { // the string given as string name = "tutorialspoint"; // to ind the length of given string int strLength = name.length(); // to print the result cout << "The length of given string is: " << strLength << endl; return 0; }
public class Main { public static void main(String[] args) { // the string given as String name = "tutorialspoint"; // to ind the length of given string int strLength = name.length(); // to print the result System.out.println("The length of given string is: " + strLength); } }
# the string given as name = "tutorialspoint" # to find the length of given string length = len(name) # Print the result print("The length of given string is:", length)
输出
The length of given string is: 14
在字符串中查找子字符串
子字符串指的是字符串的一部分或子集。在此操作中,我们需要找到给定子字符串的索引。
算法
假设我们有一个名为newSubStr的子字符串,我们需要找到它的索引号。以下是查找子字符串的算法。
1. Start 2. Declare and Initialize a string. 3. Define a sub-string. 4. Check index of sub-string. 5. If found, print index of first character of sub-string otherwise print "-1". 6. Stop
示例
在下面的示例中,我们演示了此操作在各种编程语言中的实现:
#include <stdio.h> #include <string.h> int main() { // Original string char orgnlStr[] = "tutorialspoint"; // Substring to find char newSubStr[] = "point"; // to find the pointer to the substring char *indX = strstr(orgnlStr, newSubStr); // to check if the substring is present if (indX == NULL) { printf("-1\n"); } else { printf("Substring found at index: %ld\n", indX - orgnlStr); } return 0; }
#include <iostream> #include <string> using namespace std; int main() { // Original string string orgnlStr = "tutorialspoint"; // Substring to find string newSubStr = "point"; // to find index of the substring int indX = orgnlStr.find(newSubStr); // Check if the substring is present if (indX == -1) { cout << "-1" << endl; } else { cout << "Substring found at index: " << indX << endl; } return 0; }
public class Substr { public static void main(String[] args) { // Original string String orgnlStr = "tutorialspoint"; // Substring to find String newSubStr = "point"; // to find index of the substring int indX = orgnlStr.indexOf(newSubStr); // to check if the substring is found if (indX == -1) { System.out.println("-1"); } else { System.out.println("Substring found at index: " + indX); } } }
# Original string orgnlStr = "tutorialspoint" # Substring to find newSubStr = "point" # to find index of the substring indX = orgnlStr.find(newSubStr) # to check if the substring is found if indX == -1: print("-1") else: print("Substring found at index:", indX)
输出
Substring found at index: 9
反转字符串的内容
在反转操作中,我们反转字符串中字符的顺序。这将产生一个新字符串,其中包含与原始字符串相同的字符,但顺序相反。
算法
假设我们有一个字符串,我们需要反转它。以下是反转字符串的算法。
1. Start 2. Declare an empty string. 3. Define a for loop to iterate over the characters of original string. 4. Append each character to the reversed string. 5. Print the result. 6. Stop
示例
以下是此操作在各种编程语言中的实现:
#include <stdio.h> #include <string.h> #include <stdlib.h> // function to reverse the string char* rev_str(char* orgnlStr) { int len = strlen(orgnlStr); // to store the reversed string char* revStr = (char*)malloc(len + 1); // loop to reverse the string for (int i = 0; i < len; i++) { revStr[i] = orgnlStr[len - i - 1]; } // Return the reversed string revStr[len] = '\0'; return revStr; } int main() { printf("Printing the string in reverse order: \n"); // calling the function to print the result printf("%s\n", rev_str("tutorials")); printf("%s\n", rev_str("point")); return 0; }
#include <iostream> #include <string> using namespace std; // function to reverse the string string rev_str(string orgnlStr) { // Initializing an empty string string revStr = ""; // loop to reverse the string for (int i = orgnlStr.length() - 1; i >= 0; i--) { // Append each character to the reversed string revStr += orgnlStr[i]; } // Return the reversed string return revStr; } int main() { cout << "Printing the string in reverse order: " << endl; // calling the function to print the result cout << rev_str("tutorials") << endl; cout << rev_str("point") << endl; return 0; }
public class Main { // method to reverse the string public static String rev_str(String orgnlStr) { // Initializing an empty string String revStr = ""; // loop to reverse the string for (int i = orgnlStr.length() - 1; i >= 0; i--) { // Append each character to the reversed string revStr += orgnlStr.charAt(i); } // Return the reversed string return revStr; } public static void main(String[] args) { System.out.println("Printing the string in reverse order:"); // calling the method to print the result System.out.println(rev_str("tutorials")); System.out.println(rev_str("point")); } }
# function to reverse the string def rev_str(orgnlStr): # Initializing an empty string revStr = "" # loop to reverse the string for i in range(len(orgnlStr) - 1, -1, -1): # Append each character to the reversed string revStr += orgnlStr[i] # Return the reversed string return revStr # calling the function to print the result print("Printing the string in reverse order:") print(rev_str("tutorials")) print(rev_str("point"))
输出
Printing the string in reverse order: slairotut tniop
字符串中的索引
在此操作中,我们尝试在索引的帮助下访问或定位特定字符。
算法
从给定字符串中访问指定字符的算法如下:
1. Start 2. Declare and Initialize a string. 3. Find the index number of specified character. 4. Print the result. 5. Stop
示例
在这里,我们看到了索引操作的实际实现:
#include <stdio.h> #include <string.h> int main(){ char str[] = "Tutorials Point"; char *ptr = strchr(str, 'o'); int indX = ptr - str; // print the result printf("The index of given character is: %d\n", indX); return 0; }
#include <iostream> #include <string> using namespace std; int main() { string str = "Tutorials Point"; int indX = str.find('o'); // print the result cout << "The index of given character is: " << indX << endl; return 0; }
public class Main { public static void main(String[] args) { String str = "Tutorials Point"; int indX = str.indexOf('o'); System.out.println("The index of given character is: " + indX); } }
# defining a string str = "Tutorials Point" indX = str.find('o') # print the result print("The index of given character is:", indX)
输出
The index of given character is: 3