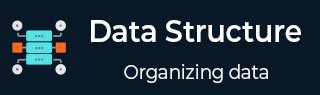
- 数据结构与算法
- DSA - 首页
- DSA - 概述
- DSA - 环境设置
- DSA - 算法基础
- DSA - 渐进分析
- 数据结构
- DSA - 数据结构基础
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表数据结构
- DSA - 双向链表数据结构
- DSA - 循环链表数据结构
- 栈与队列
- DSA - 栈数据结构
- DSA - 表达式解析
- DSA - 队列数据结构
- 搜索算法
- DSA - 搜索算法
- DSA - 线性搜索算法
- DSA - 二分搜索算法
- DSA - 插值搜索
- DSA - 跳跃搜索算法
- DSA - 指数搜索
- DSA - 斐波那契搜索
- DSA - 子列表搜索
- DSA - 哈希表
- 排序算法
- DSA - 排序算法
- DSA - 冒泡排序算法
- DSA - 插入排序算法
- DSA - 选择排序算法
- DSA - 归并排序算法
- DSA - 希尔排序算法
- DSA - 堆排序
- DSA - 桶排序算法
- DSA - 计数排序算法
- DSA - 基数排序算法
- DSA - 快速排序算法
- 图数据结构
- DSA - 图数据结构
- DSA - 深度优先遍历
- DSA - 广度优先遍历
- DSA - 生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树的遍历
- DSA - 二叉搜索树
- DSA - AVL树
- DSA - 红黑树
- DSA - B树
- DSA - B+树
- DSA - 伸展树
- DSA - 字典树
- DSA - 堆数据结构
- 递归
- DSA - 递归算法
- DSA - 使用递归实现汉诺塔
- DSA - 使用递归实现斐波那契数列
- 分治法
- DSA - 分治法
- DSA - 最大最小问题
- DSA - Strassen矩阵乘法
- DSA - Karatsuba算法
- 贪心算法
- DSA - 贪心算法
- DSA - 旅行商问题(贪心算法)
- DSA - Prim最小生成树
- DSA - Kruskal最小生成树
- DSA - Dijkstra最短路径算法
- DSA - 地图着色算法
- DSA - 分数背包问题
- DSA - 带截止日期的作业排序
- DSA - 最优合并模式算法
- 动态规划
- DSA - 动态规划
- DSA - 矩阵链乘法
- DSA - Floyd Warshall算法
- DSA - 0-1背包问题
- DSA - 最长公共子序列算法
- DSA - 旅行商问题(动态规划)
- 近似算法
- DSA - 近似算法
- DSA - 顶点覆盖算法
- DSA - 集合覆盖问题
- DSA - 旅行商问题(近似算法)
- 随机化算法
- DSA - 随机化算法
- DSA - 随机化快速排序算法
- DSA - Karger最小割算法
- DSA - Fisher-Yates洗牌算法
- DSA有用资源
- DSA - 问答
- DSA - 快速指南
- DSA - 有用资源
- DSA - 讨论
单词拆分问题
什么是单词拆分问题?
单词拆分问题是计算机科学中的一道逻辑谜题,要求我们检查给定的字符串是否可以分割成字典中的一系列单词。例如,如果给定的字符串是“ramhaspenandapple”,字典是["ram", "and", "has", "apple", "pen"],则答案为真,因为该字符串可以分割成“ram has pen and apple”。
使用回溯法解决单词拆分问题
在本问题的输入中,给出一个没有空格的句子,另一个字典也提供了一些有效的英文单词。我们必须找到将句子分解成单个字典单词的所有可能方法。给定的字符串和字典如下:
Dictionary: {ram, samuel, winter, man, mango, icecream, and, i, love, ice, cream} Given String: "ilovewinterandicecream"
我们将尝试从字符串的左侧开始搜索以找到一个有效的单词,当找到一个有效的单词时,我们将搜索该字符串下一部分中的单词。将字符串分解成给定单词的所有可能方法如下:
i love winter and ice cream i love winter and icecream
解决此问题的一种方法是使用回溯法。这是一种尝试不同组合并在部分解决方案无效时回溯的技术。基本思想是从字符串的开头开始,检查前缀是否为指定字典中的单词。如果是,则我们递归地检查剩余的后缀是否可以分割成单词。如果前缀和后缀都有效,则我们返回true并将其标记为解决方案的一部分。否则,我们回溯并尝试不同的前缀。
伪代码
以下是使用回溯法解决单词拆分问题的伪代码:
Begin for i := 0 to n, do subStr := substring of given string from (0..i) if subStr is in dictionary, then if i = n, then result := result + subStr display the result return wordBreak(substring from (i..n-i), n-i, result, subStr, ‘space’) done End
示例
在下面的示例中,我们将实际演示如何解决单词拆分问题。
#include <stdio.h> #include <string.h> #define N 13 char *dictionary[N] = {"mobile","samsung","sam","sung","man","mango", "icecream","and", "go","i","love","ice","cream"}; //check whether the word is in dictionary or not int isInDict(char *word){ for (int i = 0; i < N; i++) if (strcmp(dictionary[i], word) == 0) return 1; return 0; } void wordBreak(char *str, int n, char *result) { for (int i=1; i<=n; i++) { //get string from 0 to ith location of the string char subStr[100]; strncpy(subStr, str, i); subStr[i] = '\0'; //if subStr is found in the dictionary if (isInDict(subStr)) { if (i == n) { //add substring in the result strcat(result, subStr); printf("%s\n", result); return; } //otherwise break rest part char newResult[100]; strcpy(newResult, result); strcat(newResult, subStr); strcat(newResult, " "); wordBreak(str + i, n-i, newResult); } } } int main() { char str[] = "iloveicecreamandmango"; char result[100] = ""; wordBreak(str, strlen(str), result); return 0; }
#include <iostream> #define N 13 using namespace std; string dictionary[N] = {"mobile","samsung","sam","sung","man","mango", "icecream","and", "go","i","love","ice","cream"}; //check whether the word is in dictionary or not int isInDict(string word){ for (int i = 0; i < N; i++) if (dictionary[i].compare(word) == 0) return true; return false; } void wordBreak(string str, int n, string result) { for (int i=1; i<=n; i++) { //get string from 0 to ith location of the string string subStr = str.substr(0, i); //if subStr is found in the dictionary if (isInDict(subStr)) { if (i == n) { //add substring in the result result += subStr; cout << result << endl; return; } //otherwise break rest part wordBreak(str.substr(i, n-i), n-i, result + subStr + " "); } } } int main() { string str="iloveicecreamandmango"; wordBreak(str, str.size(),""); }
import java.util.Arrays; import java.util.List; public class Main { static final List<String> DICTIONARY = Arrays.asList("mobile","samsung","sam","sung","man","mango", "icecream","and", "go","i","love","ice","cream"); //check whether the word is in dictionary or not public static boolean isInDict(String word){ return DICTIONARY.contains(word); } public static void wordBreak(String str, int n, String result) { for (int i=1; i<=n; i++) { //get string from 0 to ith location of the string String subStr = str.substring(0, i); //if subStr is found in the dictionary if (isInDict(subStr)) { if (i == n) { //add substring in the result result += subStr; System.out.println(result); return; } //otherwise break rest part wordBreak(str.substring(i, n), n-i, result + subStr + " "); } } } public static void main(String[] args) { String str = "iloveicecreamandmango"; wordBreak(str, str.length(), ""); } }
# Define the dictionary dictionary = ["mobile","samsung","sam","sung","man","mango", "icecream","and", "go","i","love","ice","cream"] # Check whether the word is in dictionary or not def isInDict(word): return word in dictionary # Function to break the word def wordBreak(str, n, result): for i in range(1, n+1): # Get string from 0 to ith location of the string subStr = str[:i] # If subStr is found in the dictionary if isInDict(subStr): if i == n: # Add substring in the result result += subStr print(result) return # Otherwise break rest part wordBreak(str[i:], n-i, result + subStr + " ") # Main function def main(): str = "iloveicecreamandmango" wordBreak(str, len(str), "") # Call the main function if __name__ == "__main__": main()
输出
i love ice cream and man go i love ice cream and mango i love icecream and man go i love icecream and mango
广告