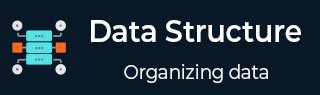
- 数据结构与算法
- DSA - 首页
- DSA - 概述
- DSA - 环境搭建
- DSA - 算法基础
- DSA - 渐近分析
- 数据结构
- DSA - 数据结构基础
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表数据结构
- DSA - 双向链表数据结构
- DSA - 循环链表数据结构
- 栈与队列
- DSA - 栈数据结构
- DSA - 表达式解析
- DSA - 队列数据结构
- 搜索算法
- DSA - 搜索算法
- DSA - 线性搜索算法
- DSA - 二分搜索算法
- DSA - 插值搜索
- DSA - 跳跃搜索算法
- DSA - 指数搜索
- DSA - 斐波那契搜索
- DSA - 子列表搜索
- DSA - 哈希表
- 排序算法
- DSA - 排序算法
- DSA - 冒泡排序算法
- DSA - 插入排序算法
- DSA - 选择排序算法
- DSA - 归并排序算法
- DSA - 希尔排序算法
- DSA - 堆排序
- DSA - 桶排序算法
- DSA - 计数排序算法
- DSA - 基数排序算法
- DSA - 快速排序算法
- 图数据结构
- DSA - 图数据结构
- DSA - 深度优先遍历
- DSA - 广度优先遍历
- DSA - 生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树的遍历
- DSA - 二叉搜索树
- DSA - AVL树
- DSA - 红黑树
- DSA - B树
- DSA - B+树
- DSA - 伸展树
- DSA - Trie树
- DSA - 堆数据结构
- 递归
- DSA - 递归算法
- DSA - 使用递归的汉诺塔
- DSA - 使用递归的斐波那契数列
- 分治法
- DSA - 分治法
- DSA - 最大最小问题
- DSA - Strassen矩阵乘法
- DSA - Karatsuba算法
- 贪心算法
- DSA - 贪心算法
- DSA - 旅行商问题(贪心法)
- DSA - Prim最小生成树
- DSA - Kruskal最小生成树
- DSA - Dijkstra最短路径算法
- DSA - 地图着色算法
- DSA - 分数背包问题
- DSA - 带截止日期的作业排序
- DSA - 最优合并模式算法
- 动态规划
- DSA - 动态规划
- DSA - 矩阵链乘法
- DSA - Floyd-Warshall算法
- DSA - 0-1背包问题
- DSA - 最长公共子序列算法
- DSA - 旅行商问题(动态规划法)
- 近似算法
- DSA - 近似算法
- DSA - 顶点覆盖算法
- DSA - 集合覆盖问题
- DSA - 旅行商问题(近似算法)
- 随机化算法
- DSA - 随机化算法
- DSA - 随机化快速排序算法
- DSA - Karger最小割算法
- DSA - Fisher-Yates洗牌算法
- DSA有用资源
- DSA - 问答
- DSA - 快速指南
- DSA - 有用资源
- DSA - 讨论
哈密顿回路
什么是哈密顿回路?
哈密顿回路(或环路)是在图中的一条路径,它访问每个顶点恰好一次并返回到起始顶点,形成一个闭环。只有当图包含哈密顿回路时,该图才被称为哈密顿图,否则被称为非哈密顿图。
图是一种抽象数据类型 (ADT),由通过链接连接的一组对象组成。
哈密顿回路问题的实际应用可见于网络设计、交付系统等许多领域。但是,该问题的解决方案仅适用于小型图,而不适用于大型图。
输入输出场景
假设给定的无向图 G(V, E) 及其邻接矩阵如下所示:
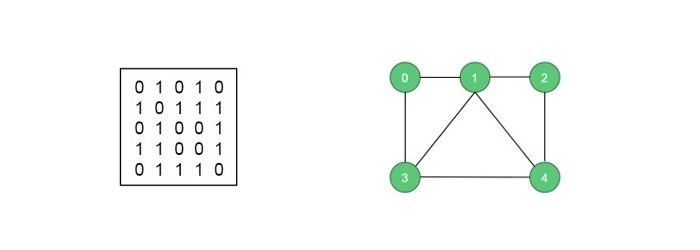
可以使用回溯算法在上述图中查找哈密顿路径。如果找到,算法将返回路径;如果没有找到,则返回 false。对于这种情况,输出应为 (0, 1, 2, 4, 3, 0)。
使用回溯法查找哈密顿回路
解决哈密顿回路问题的朴素方法是生成所有可能的顶点配置,并检查是否有任何配置满足给定的约束条件。但是,这种方法不适用于大型图,因为其时间复杂度为 (O(N!))。
以下步骤解释了回溯方法的工作原理:
首先,创建一个空的路径数组,并将起始顶点 0 添加到其中。
接下来,从顶点 1 开始,然后逐个添加其他顶点。
添加顶点时,检查给定顶点是否与先前添加的顶点相邻,并且尚未添加。
如果找到任何这样的顶点,则将其作为解决方案的一部分添加到路径中,否则返回 false。
示例
以下示例演示如何在给定的无向图中查找哈密顿回路。
#include <stdio.h> #define NODE 5 int graph[NODE][NODE] = { {0, 1, 0, 1, 0}, {1, 0, 1, 1, 1}, {0, 1, 0, 0, 1}, {1, 1, 0, 0, 1}, {0, 1, 1, 1, 0}, }; int path[NODE]; // Function to display the Hamiltonian cycle void displayCycle() { printf("Cycle Found: "); for (int i = 0; i < NODE; i++) printf("%d ", path[i]); // Print the first vertex again printf("%d\n", path[0]); } // Function to check if adding vertex v to the path is valid int isValid(int v, int k) { // If there is no edge between path[k-1] and v if (graph[path[k - 1]][v] == 0) return 0; // Check if vertex v is already taken in the path for (int i = 0; i < k; i++) if (path[i] == v) return 0; return 1; } // Function to find the Hamiltonian cycle int cycleFound(int k) { // When all vertices are in the path if (k == NODE) { // Check if there is an edge between the last and first vertex if (graph[path[k - 1]][path[0]] == 1) return 1; else return 0; } // Try adding each vertex (except the starting point) to the path for (int v = 1; v < NODE; v++) { if (isValid(v, k)) { path[k] = v; if (cycleFound(k + 1) == 1) return 1; // Backtrack: Remove v from the path path[k] = -1; } } return 0; } // Function to find and display the Hamiltonian cycle int hamiltonianCycle() { for (int i = 0; i < NODE; i++) path[i] = -1; // Set the first vertex as 0 path[0] = 0; if (cycleFound(1) == 0) { printf("Solution does not exist\n"); return 0; } displayCycle(); return 1; } int main() { hamiltonianCycle(); return 0; }
#include <iostream> #define NODE 5 using namespace std; int graph[NODE][NODE] = { {0, 1, 0, 1, 0}, {1, 0, 1, 1, 1}, {0, 1, 0, 0, 1}, {1, 1, 0, 0, 1}, {0, 1, 1, 1, 0}, }; int path[NODE]; // Function to display the Hamiltonian cycle void displayCycle() { cout << "Cycle Found: "; for (int i = 0; i < NODE; i++) cout << path[i] << " "; // Print the first vertex again cout << path[0] << endl; } // Function to check if adding vertex v to the path is valid bool isValid(int v, int k) { // If there is no edge between path[k-1] and v if (graph[path[k - 1]][v] == 0) return false; // Check if vertex v is already taken in the path for (int i = 0; i < k; i++) if (path[i] == v) return false; return true; } // function to find the Hamiltonian cycle bool cycleFound(int k) { // When all vertices are in the path if (k == NODE) { // Check if there is an edge between the last and first vertex if (graph[path[k - 1]][path[0]] == 1) return true; else return false; } // adding each vertex to the path for (int v = 1; v < NODE; v++) { if (isValid(v, k)) { path[k] = v; if (cycleFound(k + 1) == true) return true; // Remove v from the path path[k] = -1; } } return false; } // Function to find and display the Hamiltonian cycle bool hamiltonianCycle() { for (int i = 0; i < NODE; i++) path[i] = -1; // Set the first vertex as 0 path[0] = 0; if (cycleFound(1) == false) { cout << "Solution does not exist" << endl; return false; } displayCycle(); return true; } int main() { hamiltonianCycle(); }
public class HamiltonianCycle { static final int NODE = 5; static int[][] graph = { {0, 1, 0, 1, 0}, {1, 0, 1, 1, 1}, {0, 1, 0, 0, 1}, {1, 1, 0, 0, 1}, {0, 1, 1, 1, 0} }; static int[] path = new int[NODE]; // method to display the Hamiltonian cycle static void displayCycle() { System.out.print("Cycle Found: "); for (int i = 0; i < NODE; i++) System.out.print(path[i] + " "); // Print the first vertex again System.out.println(path[0]); } // method to check if adding vertex v to the path is valid static boolean isValid(int v, int k) { // If there is no edge between path[k-1] and v if (graph[path[k - 1]][v] == 0) return false; // Check if vertex v is already taken in the path for (int i = 0; i < k; i++) if (path[i] == v) return false; return true; } // method to find the Hamiltonian cycle static boolean cycleFound(int k) { // When all vertices are in the path if (k == NODE) { // Check if there is an edge between the last and first vertex if (graph[path[k - 1]][path[0]] == 1) return true; else return false; } // adding each vertex (except the starting point) to the path for (int v = 1; v < NODE; v++) { if (isValid(v, k)) { path[k] = v; if (cycleFound(k + 1)) return true; // Remove v from the path path[k] = -1; } } return false; } // method to find and display the Hamiltonian cycle static boolean hamiltonianCycle() { for (int i = 0; i < NODE; i++) path[i] = -1; // Set the first vertex as 0 path[0] = 0; if (!cycleFound(1)) { System.out.println("Solution does not exist"); return false; } displayCycle(); return true; } public static void main(String[] args) { hamiltonianCycle(); } }
NODE = 5 graph = [ [0, 1, 0, 1, 0], [1, 0, 1, 1, 1], [0, 1, 0, 0, 1], [1, 1, 0, 0, 1], [0, 1, 1, 1, 0] ] path = [None] * NODE # Function to display the Hamiltonian cycle def displayCycle(): print("Cycle Found:", end=" ") for i in range(NODE): print(path[i], end=" ") # Print the first vertex again print(path[0]) # Function to check if adding vertex v to the path is valid def isValid(v, k): # If there is no edge between path[k-1] and v if graph[path[k - 1]][v] == 0: return False # Check if vertex v is already taken in the path for i in range(k): if path[i] == v: return False return True # Function to find the Hamiltonian cycle def cycleFound(k): # When all vertices are in the path if k == NODE: # Check if there is an edge between the last and first vertex if graph[path[k - 1]][path[0]] == 1: return True else: return False # adding each vertex (except the starting point) to the path for v in range(1, NODE): if isValid(v, k): path[k] = v if cycleFound(k + 1): return True # Remove v from the path path[k] = None return False # Function to find and display the Hamiltonian cycle def hamiltonianCycle(): for i in range(NODE): path[i] = None # Set the first vertex as 0 path[0] = 0 if not cycleFound(1): print("Solution does not exist") return False displayCycle() return True if __name__ == "__main__": hamiltonianCycle()
输出
Cycle Found: 0 1 2 4 3 0
广告