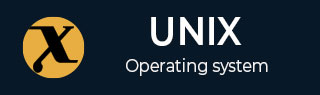
- Unix Commands Reference
- Unix Commands - Home
continue Command in Linux
continue is a Linux command that you can use within loops (like for, while or until) to skip the current iteration.
When you use the continue command in a program, it immediately terminates the current loop iteration and forwards towards the next one. Thus, it helps you in controlling the flow of execution by skipping specific parts of the loop based on conditions.
You can also specify an optional parameter [N] to continue from the N-th enclosing loop, although by default, it continues from the immediate loop.
Syntax of continue Command
The basic syntax to use the continue command in Linux is given below −
continue
Or
continue [N]
Examples of continue Command in Linux
Let’s explore a few examples of continue command on Linux system −
1. Using continue in a for Loop
You can use the continue command in a for loop for skipping the current iteration and moving onto the next one. Once you do, it bypasses the remaining code within the loop for the current iteration and forwards towards the next value.
Here is an example −
#!/bin/bash for i in $(seq 1 10); do if ((i == 5)); then continue fi echo $i done
You have to copy the code and paste it into a script file of your choice. The above code will skip printing the value of i when it equals 5, resulting in output from 1 to 10 except for 5.
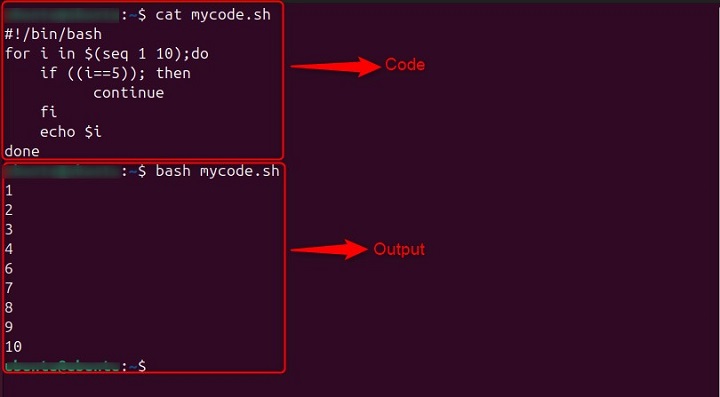
2. Using continue in a while Loop
In a while loop, the continue command behaves similarly, as it skips the iteration and proceeds to the next one. The example to use the continue command in a while loop is given below −
#!/bin/bash i=1 while ((i <= 10)); do ((++i)) if ((i == 5)); then continue fi echo $i done
The above code will ensure that the continue command will skip the number 5 during the loop iteration, resulting in output from 2 to 10 except for 5.
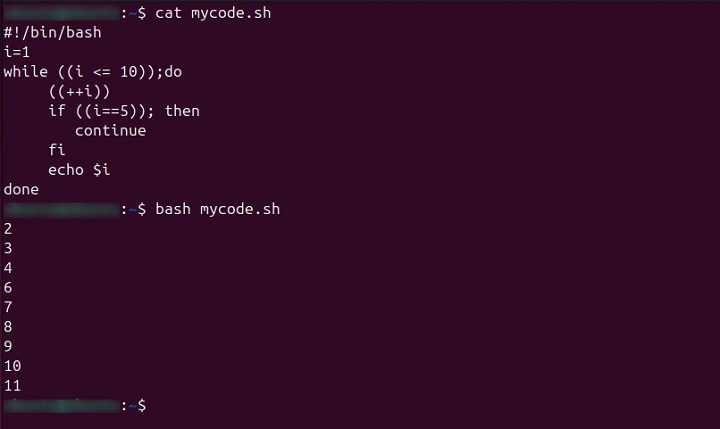
3. Using continue in an until Loop
The until loop also supports the continue command and allows you to skip specific interaction in a bash program. For example, consider the following code −
#!/bin/bash i=0 until ((i == 10)); do ((++i)) if ((i == 5)); then continue fi echo $i done
The above code will skip number 5 and output other numbers from 1 to 10.
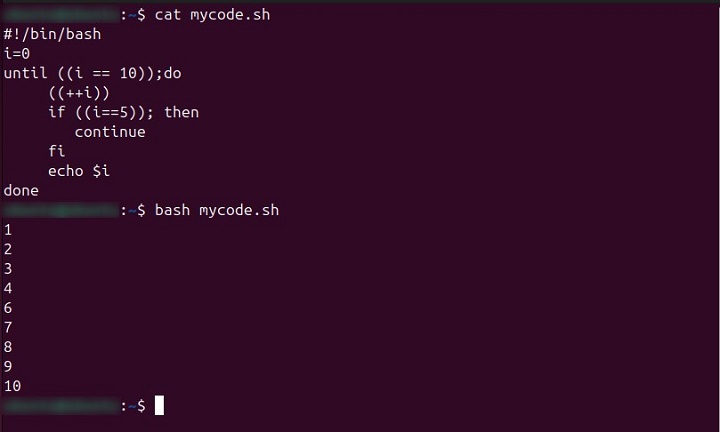
In this way, you can use the continue command in a program to selectively skip specific iterations within loops and control the flow of execution based on conditions.
Conclusion
The continue command is a versatile tool that you can use within the loops to skip specific iterations based on conditions. Whether in a for, while or until loop, the continue command will help you fine-tune your program’s behavior.
In this tutorial, we explained the syntax of continue command, along with a few examples to showcase the advantages of continue command. You must use the command wisely on your Linux system to enhance your code efficiency and readability.