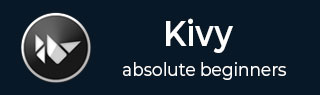
- Kivy 教程
- Kivy - 首页
- Kivy 基础
- Kivy - 入门
- Kivy - 安装
- Kivy - 架构
- Kivy - 文件语法
- Kivy - 应用程序
- Kivy - Hello World
- Kivy - 应用生命周期
- Kivy - 事件
- Kivy - 属性
- Kivy - 输入
- Kivy - 行为
- Kivy 按钮
- Kivy - 按钮
- Kivy - 按钮事件
- Kivy - 按钮颜色
- Kivy - 按钮大小
- Kivy - 按钮位置
- Kivy - 圆形按钮
- Kivy - 禁用按钮
- Kivy - 图像按钮
- Kivy 组件
- Kivy - 组件
- Kivy - 标签
- Kivy - 文本输入
- Kivy - 画布
- Kivy - 线
- Kivy - 复选框
- Kivy - 下拉列表
- Kivy - 窗口
- Kivy - 滚动视图
- Kivy - 走马灯
- Kivy - 滑块
- Kivy - 图像
- Kivy - 弹出窗口
- Kivy - 开关
- Kivy - 微调器
- Kivy - 分隔器
- Kivy - 进度条
- Kivy - 气泡
- Kivy - 标签页面板
- Kivy - 散点图
- Kivy - 手风琴
- Kivy - 文件选择器
- Kivy - 颜色拾取器
- Kivy - 代码输入
- Kivy - 模态视图
- Kivy - 切换按钮
- Kivy - 相机
- Kivy - 树视图
- Kivy - reStructuredText
- Kivy - 操作栏
- Kivy - 媒体播放器
- Kivy - 模板视图
- Kivy - 虚拟键盘
- Kivy - 触摸水波纹
- Kivy - 音频
- Kivy - 视频
- Kivy - 拼写检查
- Kivy - 效果
- Kivy - 输入记录器
- Kivy - OpenGL
- Kivy - 文本
- Kivy - 文本标记
- Kivy - 设置
- Kivy 布局
- Kivy - 布局
- Kivy - 浮动布局
- Kivy - 网格布局
- Kivy - 箱式布局
- Kivy - 堆叠布局
- Kivy - 锚点布局
- Kivy - 相对布局
- Kivy - 页面布局
- Kivy - 回收布局
- Kivy - 布局嵌套
- Kivy 高级概念
- Kivy - 配置对象
- Kivy - 图集
- Kivy - 数据加载器
- Kivy - 缓存管理器
- Kivy - 控制台
- Kivy - 动画
- Kivy - 多笔画
- Kivy - 时钟
- Kivy - SVG
- Kivy - UrlRequest
- Kivy - 剪贴板
- Kivy - 工厂
- Kivy - 手势
- Kivy - 语言
- Kivy - 图形
- Kivy - 绘制
- Kivy - 打包
- Kivy - 花园
- Kivy - 存储
- Kivy - 矢量
- Kivy - 工具函数
- Kivy - 检查器
- Kivy - 工具
- Kivy - 日志记录器
- Kivy - 帧缓冲区
- Kivy 应用程序和项目
- Kivy - 绘图应用程序
- Kivy - 计算器应用程序
- Kivy - 计时器应用程序
- Kivy - 相机处理
- Kivy - 图像查看器
- Kivy - 贝塞尔曲线
- Kivy - 画布压力测试
- Kivy - 圆形绘制
- Kivy - 组件动画
- Kivy - 其他
- Kivy 有用资源
- Kivy - 快速指南
- Kivy - 有用资源
- Kivy - 讨论
Kivy - 图像查看器
在本章中,我们将使用 Kivy 构建一个简单的图像查看器应用程序。以下代码使用 Kivy 的 Image 组件和按钮来浏览选定文件夹中图像列表。还有一个按钮可以打开文件选择器,让用户选择不同的文件夹以查看其中的图像。
首先,我们需要构建当前选定文件夹中所有图像文件的列表。为此,我们使用 os.listdir() 方法。
import os self.idx=0 self.fillist=[] dir_path = '.' for file in os.listdir(dir_path): if file.endswith('.png'): self.fillist.append(file)
应用程序界面的构建涉及一个垂直的箱式布局,其中 Image 对象放置在顶部,另一个水平的箱式布局包含三个按钮。
lo=BoxLayout(orientation='vertical') self.img= Image(source=self.fillist[self.idx]) lo.add_widget(self.img) hlo=BoxLayout(orientation='horizontal', size_hint=(1, .1)) self.b1 = Button(text = 'Dir', on_press=self.onButtonPress) self.b2 = Button(text = 'Prev', on_press=self.previmg) self.b3 = Button(text = 'Next', on_press=self.nextimg) hlo.add_widget(self.b1) hlo.add_widget(self.b2) hlo.add_widget(self.b3) lo.add_widget(hlo)
Image 组件显示第一个可用的图像以开始。当点击标题为“下一页”的按钮时,指向文件列表的索引会递增;当点击“上一页”按钮时,索引会递减,并在 Image 对象中加载索引的图像文件。
def previmg(self, instance): self.idx-=1 if self.idx<0: self.idx=0 self.img.source=self.fillist[self.idx] def nextimg(self, instance): self.idx+=1 if self.idx>=len(self.fillist): self.idx=len(self.fillist)-1 self.img.source=self.fillist[self.idx]
第三个按钮(标题为 Dir)会弹出一个文件选择器对话框。您可以选择所需的文件夹进行查看。
def onButtonPress(self, button): layout=GridLayout(cols=1) fw=FileChooserListView(dirselect=True, filters=["!*.sys"]) fw.bind(on_selection=self.onselect) closeButton = Button( text = "OK", size_hint=(None, None), size=(100,75) ) layout.add_widget(fw) layout.add_widget(closeButton) self.popup = Popup( title='Choose Folder', content=layout, auto_dismiss=False, size_hint=(None, None), size=(400, 400) ) self.popup.open()
示例
当前文件夹中的图像会填充文件列表以开始。以下是完整代码:
from kivy.app import App from kivy.uix.boxlayout import BoxLayout from kivy.uix.gridlayout import GridLayout from kivy.uix.label import Label from kivy.uix.popup import Popup from kivy.uix.image import Image from kivy.uix.button import Button from kivy.uix.filechooser import FileChooserListView from kivy.core.window import Window Window.size = (720, 400) class ImageViewerApp(App): def previmg(self, instance): self.idx -= 1 if self.idx < 0: self.idx = 0 self.img.source = self.fillist[self.idx] def nextimg(self, instance): self.idx += 1 if self.idx >= len(self.fillist): self.idx = len(self.fillist) - 1 self.img.source = self.fillist[self.idx] def onButtonPress(self, button): layout = GridLayout(cols=1) fw = FileChooserListView(dirselect=True, filters=["!*.sys"]) fw.bind(on_selection=self.onselect) closeButton = Button( text="OK", size_hint=(None, None), size=(100, 75) ) layout.add_widget(fw) layout.add_widget(closeButton) self.popup = Popup( title='Choose Folder', content=layout, auto_dismiss=False, size_hint=(None, None), size=(400, 400) ) self.popup.open() closeButton.bind(on_press=self.on_close) def onselect(self, *args): print(args[1][0]) def on_close(self, event): self.popup.dismiss() def build(self): import os self.idx = 0 self.fillist = [] dir_path = '.' for file in os.listdir(dir_path): if file.endswith('.png'): self.fillist.append(file) lo = BoxLayout(orientation='vertical') self.img = Image(source=self.fillist[self.idx]) lo.add_widget(self.img) hlo = BoxLayout(orientation='horizontal', size_hint=(1, .1)) self.b1 = Button(text='Dir', on_press=self.onButtonPress) self.b2 = Button(text='Prev', on_press=self.previmg) self.b3 = Button(text='Next', on_press=self.nextimg) hlo.add_widget(self.b1) hlo.add_widget(self.b2) hlo.add_widget(self.b3) lo.add_widget(hlo) return lo ImageViewerApp().run()
输出
运行此代码时,它将显示索引“0”处的图像:
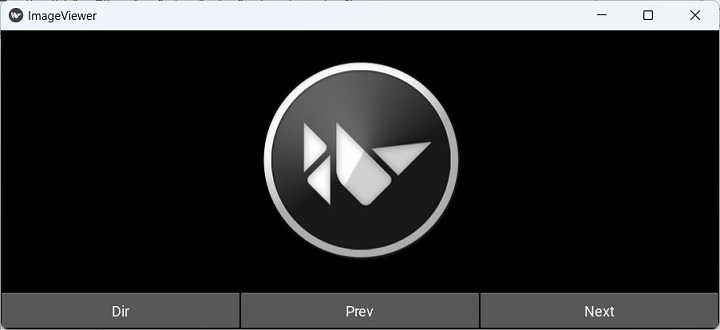
点击导航按钮向前和向后。点击“Dir”按钮选择新文件夹。
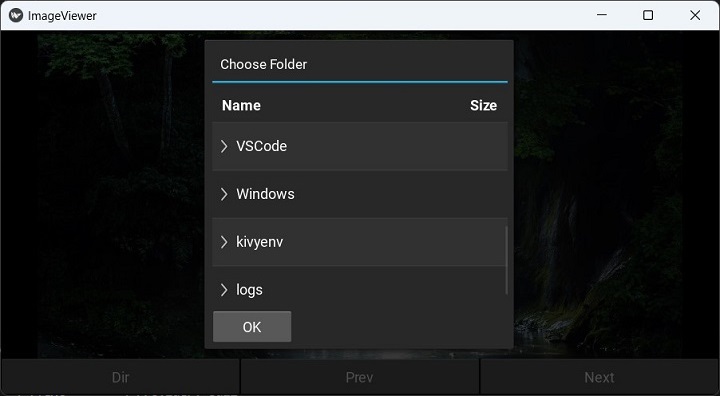
广告