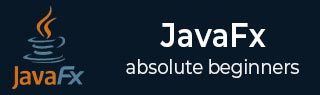
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境配置
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维图形
- JavaFX - 二维图形
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制圆弧
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 视觉特效
- JavaFX - 视觉特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图片输入特效
- JavaFX - 混合特效
- JavaFX - 光晕特效
- JavaFX - 辉光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 棕褐色特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内阴影特效
- JavaFX - 照明特效
- JavaFX - 远光灯特效
- JavaFX - 聚光灯特效
- JavaFX - 点光源特效
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维图形
- JavaFX - 三维图形
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 警报框
警报框指的是出现在屏幕上用于告知用户错误或任何事件的弹出窗口或对话框。警报框的信息不仅仅限于错误消息,它可以是任何消息,包括简单的“你好”。例如,下图显示了关于文件夹删除的通知 -
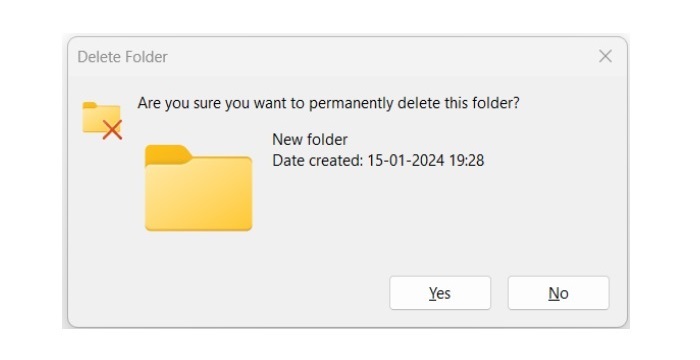
JavaFX 中的警报框
在 JavaFX 中,警报框由名为 Alert 的类表示。此类属于包 javafx.scene.control。通过实例化此类,我们可以在 JavaFX 中创建警报框。此外,我们需要将 Alert.AlertType 枚举值传递给构造函数。此值决定对话框的默认属性,例如标题、标题栏、图形和按钮。Alert 类的构造函数如下所示:
Alert(Alert.AlertType typeOfalert) − 用于使用指定的警报类型构造警报框。
Alert(Alert.AlertType typeOfalert, String str, ButtonType buttons) − 使用指定的警报类型、预定义文本和按钮类型构造警报框。
如何在 JavaFX 中创建警报框?
请按照以下步骤在 JavaFX 中创建警报框。
步骤 1:实例化 Alert 类
要创建警报框,请实例化 Alert 类并将 Alert.AlertType 枚举值作为参数值传递给其构造函数,如下面的代码所示:
// Creating an Alert Alert alert = new Alert(AlertType.INFORMATION);
步骤 2:设置警报框标题
使用 setTitle() 方法为警报框设置合适的标题,如下面的代码块所示:
// setting the title of alert box alert.setTitle("Alert Box");
步骤 3:设置警报框标题栏文本
setHeaderText() 方法用于设置警报框的标题栏文本。我们使用下面的代码块将标题栏文本设置为“null”:
// setting header text alert.setHeaderText(null);
步骤 4:设置警报框内容文本
要设置警报框的内容文本,请使用 setContentText() 方法。它接受 String 类型的文本,如下面的代码所示:
// setting content text alert.setContentText("Showing an Alert in JavaFX!");
步骤 5:启动应用程序
创建警报框并设置其属性后,创建一个按钮,单击该按钮将显示警报框。接下来,通过将 Button 对象传递给其构造函数来定义布局面板,例如 VBox 和 HBox。然后,设置 Scene 和 Stage。最后,使用 launch() 方法启动应用程序。
示例
下面的 JavaFX 程序演示了如何在 JavaFX 应用程序中生成警报框。将此代码保存在名为 ShowAlert.java 的文件中。
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Alert; import javafx.scene.control.Alert.AlertType; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; public class ShowAlert extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("On clicking the below button, it will display an alert...."); // Creating a Button Button button = new Button("Show Alert"); // Creating an Alert Alert alert = new Alert(AlertType.INFORMATION); alert.setTitle("Alert Box"); alert.setHeaderText(null); alert.setContentText("Showing an Alert in JavaFX!"); // Setting the Button's action button.setOnAction(e -> alert.showAndWait()); // Create a VBox to hold the Label and Button VBox vbox = new VBox(label, button); vbox.setAlignment(Pos.CENTER); // Create a Scene with the VBox as its root node Scene scene = new Scene(vbox, 400, 300); // Set the Title of the Stage stage.setTitle("Alert in JavaFX"); // Set the Scene of the Stage stage.setScene(scene); // Display the Stage stage.show(); } public static void main(String[] args) { launch(args); } }
要从命令提示符编译和执行保存的 Java 文件,请使用以下命令:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ShowAlert.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ShowAlert
输出
执行上述代码时,将生成以下输出。
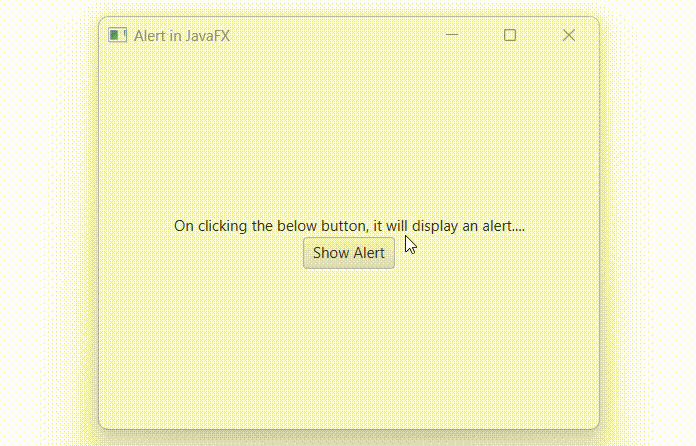
JavaFX 中的警报框类型
Alert 类是 Dialog 类的子类,它支持许多预构建的对话框(或警报框)类型,即确认、警告、信息、错误和无。通过更改 Alert.AlertType 枚举的值,我们可以使用这些不同的警报框类型。
示例
在下面的示例中,我们将演示 JavaFX 中的警报框类型。将此代码保存在名为 JavafxAlert.java 的文件中。
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Alert; import javafx.scene.control.Alert.AlertType; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.scene.layout.HBox; import javafx.stage.Stage; import javafx.geometry.Pos; import javafx.geometry.Insets; public class JavafxAlert extends Application { @Override public void start(Stage stage) { // Creating a Label Label label = new Label("Types of alert in JavaFX..."); // Creating Buttons Button cnfrmButtn = new Button("Confirm"); Button infrmButtn = new Button("Inform"); Button warngButtn = new Button("Warning"); Button errorButtn = new Button("Error"); // Creating information Alert and setting Button's action Alert infrmAlert = new Alert(AlertType.INFORMATION); infrmAlert.setContentText("It is an Information Alert!"); infrmButtn.setOnAction(e -> infrmAlert.showAndWait()); // Creating confirmation Alert and setting Button's action Alert cnfrmAlert = new Alert(AlertType.CONFIRMATION); cnfrmAlert.setContentText("It is a Confirmation Alert!"); cnfrmButtn.setOnAction(e -> cnfrmAlert.showAndWait()); // Creating warning Alert and setting Button's action Alert warngAlert = new Alert(AlertType.WARNING); warngAlert.setContentText("It is a Warning Alert!"); warngButtn.setOnAction(e -> warngAlert.showAndWait()); // Creating error Alert and setting Button's action Alert errorAlert = new Alert(AlertType.ERROR); errorAlert.setContentText("It is an Error Alert!"); errorButtn.setOnAction(e -> errorAlert.showAndWait()); // Create a HBox to hold the Buttons HBox box = new HBox(cnfrmButtn, infrmButtn, warngButtn, errorButtn); box.setAlignment(Pos.CENTER); box.setPadding(new Insets(15)); box.setSpacing(10); // Create a VBox to hold the Label and Button VBox vbox = new VBox(label, box); vbox.setAlignment(Pos.CENTER); // Create a Scene with the VBox as its root node Scene scene = new Scene(vbox, 400, 300); // Set the Title of the Stage stage.setTitle("Alert in JavaFX"); // Set the Scene of the Stage stage.setScene(scene); // Display the Stage stage.show(); } public static void main(String[] args) { launch(args); } }
使用以下命令从命令提示符编译和执行保存的 Java 文件:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxAlert.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxAlert
输出
执行上述代码后,它将生成一个显示四个按钮的窗口。每个按钮都与不同的警报框相关联。
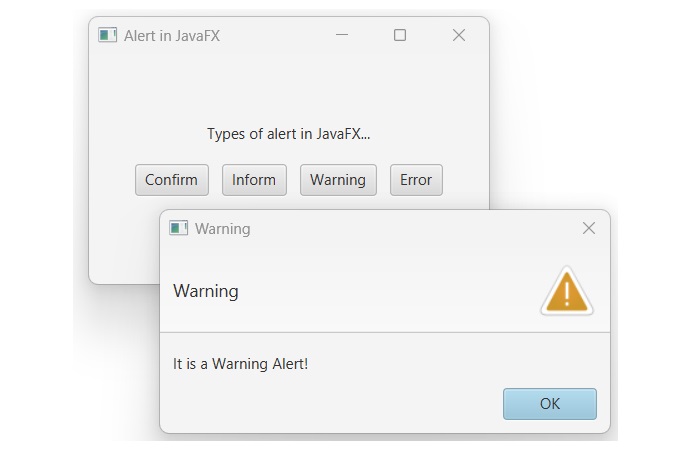