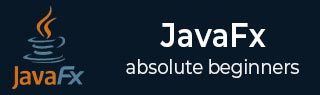
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用
- JavaFX 二维图形
- JavaFX - 二维图形
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图片输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 褐色调效果
- JavaFX - 阴影效果
- JavaFX - 投影阴影效果
- JavaFX - 内阴影效果
- JavaFX - 光照效果
- JavaFX - 远光源效果
- JavaFX - 聚光灯效果
- JavaFX - 点光源效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维图形
- JavaFX - 三维图形
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 气泡图
气泡图用于绘制三维数据;第三维由气泡的大小(半径)表示。
下面是一个描绘已完成工作的示例气泡图。
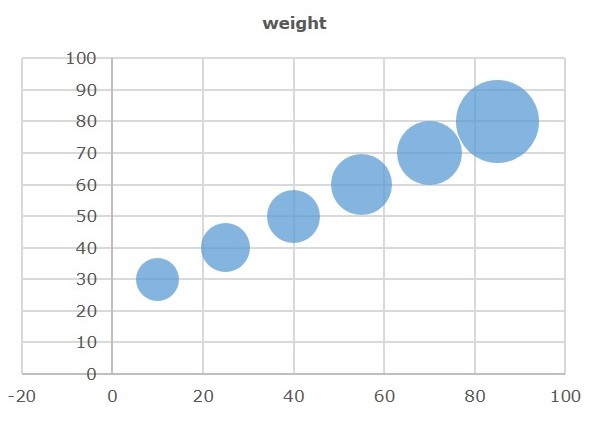
JavaFX 中的气泡图
在 JavaFX 中,气泡图由名为 BubbleChart 的类表示。此类属于 javafx.scene.chart 包。通过实例化此类,您可以在 JavaFX 中创建气泡图节点。
要在 JavaFX 中生成气泡图,请按照以下步骤操作。
步骤 1:定义轴
定义气泡图的 X 轴和 Y 轴,并为其设置标签。在我们的示例中,X 轴代表年龄,Y 轴代表体重。气泡的半径代表已完成的工作量。
public class ClassName extends Application { @Override public void start(Stage primaryStage) throws Exception { //Defining the X axis NumberAxis xAxis = new NumberAxis(0, 100, 10); xAxis.setLabel("Age"); //Defining Y axis NumberAxis yAxis = new NumberAxis(20, 100, 10); yAxis.setLabel("Weight"); } }
步骤 2:创建气泡图
通过实例化 javafx.scene.chart 包中名为 BubbleChart 的类来创建一个气泡图。将表示在先前步骤中创建的 X 轴和 Y 轴的对象传递给此类的构造函数。
//Creating the Bubble chart BubbleChart bubbleChart = new BubbleChart(xAxis, yAxis);
步骤 3:准备数据
实例化 XYChart.Series 类并将数据(一系列 x 和 y 坐标)添加到此类的 Observable 列表中,如下所示:
//Prepare XYChart.Series objects by setting data XYChart.Series series = new XYChart.Series(); series.setName("work"); series.getData().add(new XYChart.Data(10,30,4)); series.getData().add(new XYChart.Data(25,40,5)); series.getData().add(new XYChart.Data(40,50,9)); series.getData().add(new XYChart.Data(55,60,7)); series.getData().add(new XYChart.Data(70,70,9)); series.getData().add(new XYChart.Data(85,80,6));
步骤 4:将数据添加到气泡图
将上一步中准备的数据系列添加到气泡图中,如下所示:
//Setting the data to bar chart bubbleChart.getData().add(series);
步骤 5:创建 Group 对象
在 start() 方法中,通过实例化名为 Group 的类来创建一个 Group 对象。这属于 javafx.scene 包。
将上一步中创建的气泡图(节点)对象作为参数传递给 Group 类的构造函数。这应该按如下方式完成,以便将其添加到组中:
Group root = new Group(bubbleChart);
步骤 6:启动应用程序
最后,按照以下步骤正确启动应用程序:
首先,通过将 Group 对象作为参数值传递给其构造函数来实例化名为 Scene 的类。您可以将应用程序屏幕的尺寸作为可选参数传递给此构造函数。
然后,使用 Stage 类的 setTitle() 方法设置舞台的标题。
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到舞台。
使用名为 show() 的方法显示场景的内容。
最后,使用 launch() 方法启动应用程序。
示例
让我们考虑不同的个体及其年龄、体重和工作能力。工作能力可以视为工作小时数,在图表中绘制为气泡。
体重 | ||||||||
---|---|---|---|---|---|---|---|---|
年龄 | ||||||||
30 | 40 | 50 | 60 | 70 | 80 | |||
10 | 4 | 工作量 | ||||||
25 | 5 | |||||||
40 | 6 | |||||||
55 | 8 | |||||||
70 | 9 | |||||||
85 | 15 |
以下是一个 Java 程序,它使用 JavaFX 生成一个气泡图,描绘了上述数据。
将此代码保存在名为 BubbleChartExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.chart.BubbleChart; import javafx.stage.Stage; import javafx.scene.chart.NumberAxis; import javafx.scene.chart.XYChart; public class BubbleChartExample extends Application { @Override public void start(Stage stage) { //Defining the axes NumberAxis xAxis = new NumberAxis(0, 100, 10); xAxis.setLabel("Age"); NumberAxis yAxis = new NumberAxis(20, 100, 10); yAxis.setLabel("Weight"); //Creating the Bubble chart BubbleChart bubbleChart = new BubbleChart(xAxis, yAxis); //Prepare XYChart.Series objects by setting data XYChart.Series series = new XYChart.Series(); series.setName("work"); series.getData().add(new XYChart.Data(10,30,4)); series.getData().add(new XYChart.Data(25,40,5)); series.getData().add(new XYChart.Data(40,50,9)); series.getData().add(new XYChart.Data(55,60,7)); series.getData().add(new XYChart.Data(70,70,9)); series.getData().add(new XYChart.Data(85,80,6)); //Setting the data to bar chart bubbleChart.getData().add(series); //Creating a Group object Group root = new Group(bubbleChart); //Creating a scene object Scene scene = new Scene(root, 600, 400); //Setting title to the Stage stage.setTitle("Bubble Chart"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BubbleChartExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BubbleChartExample
输出
执行上述程序后,将生成一个 JavaFX 窗口,显示如下所示的气泡图。
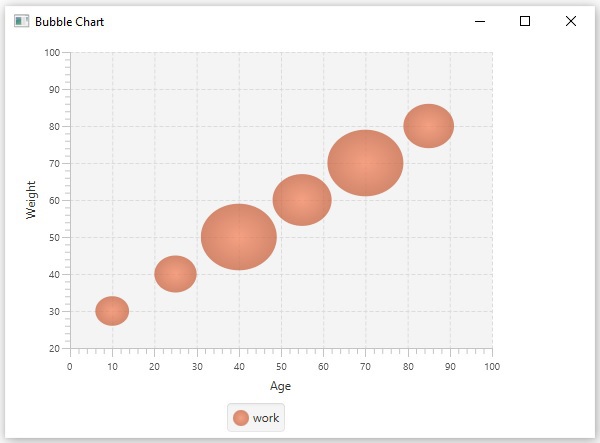
示例
下表显示了 1970 年至 2014 年某地区学校的数量。
年份 | 学校数量 |
---|---|
1970 | 15 |
1980 | 30 |
1990 | 60 |
2000 | 120 |
2013 | 240 |
2014 | 300 |
以下是一个 Java 程序,它使用 JavaFX 生成一个气泡图,描绘了上述数据。
将此代码保存在名为 BubbleChartSchools.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.chart.BubbleChart; import javafx.scene.chart.NumberAxis; import javafx.scene.chart.XYChart; public class BubbleChartSchools extends Application { @Override public void start(Stage stage) { //Defining the x axis NumberAxis xAxis = new NumberAxis(1960, 2020, 10); xAxis.setLabel("Years"); //Defining the y axis NumberAxis yAxis = new NumberAxis(20, 320, 20); yAxis.setLabel("No.of schools"); //Creating the Bubble chart BubbleChart bubblechart = new BubbleChart(xAxis, yAxis); //Prepare XYChart.Series objects by setting data XYChart.Series series = new XYChart.Series(); series.setName("No of schools in an year"); // Add a third coordinate representing the radius of bubble series.getData().add(new XYChart.Data(1970, 25, 1)); series.getData().add(new XYChart.Data(1980, 30, 2)); series.getData().add(new XYChart.Data(1990, 60, 3)); series.getData().add(new XYChart.Data(2000, 120, 4)); series.getData().add(new XYChart.Data(2013, 240, 5)); series.getData().add(new XYChart.Data(2014, 300, 6)); //Setting the data to bubble chart bubblechart.getData().add(series); //Creating a Group object Group root = new Group(bubblechart); //Creating a scene object Scene scene = new Scene(root, 600, 400); //Setting title to the Stage stage.setTitle("Bubble Chart"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BubbleChartSchools.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BubbleChartSchools
输出
执行上述程序后,将生成一个 JavaFX 窗口,显示如下所示的气泡图。
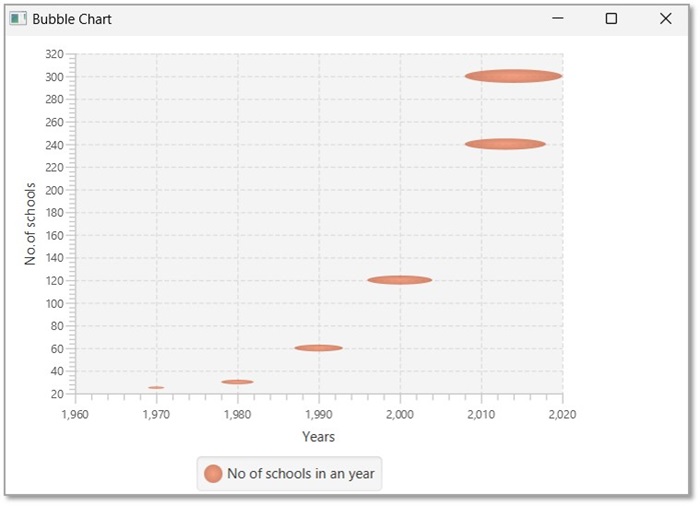