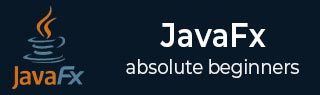
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 笔触类型属性
- JavaFX - 笔触宽度属性
- JavaFX - 笔触填充属性
- JavaFX - 笔触属性
- JavaFX - 笔触连接属性
- JavaFX - 笔触斜接限制属性
- JavaFX - 笔触端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转转换
- JavaFX - 缩放转换
- JavaFX - 平移转换
- JavaFX - 淡入淡出转换
- JavaFX - 填充转换
- JavaFX - 笔触转换
- JavaFX - 顺序转换
- JavaFX - 并行转换
- JavaFX - 暂停转换
- JavaFX - 路径转换
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建柱状图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠柱状图
- JavaFX 布局窗格
- JavaFX - 布局窗格
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 剔除面属性
JavaFX 还为 3D 对象提供了各种属性。这些属性的范围可以从决定形状的材质:内部和外部,渲染 3D 对象几何体以及剔除 3D 形状的面。
提供所有这些属性是为了改进 3D 对象的外观和感觉;并检查什么适合应用程序并应用它们。
在本章中,让我们进一步了解剔除面属性。
剔除面属性
通常,剔除是指移除形状方向错误的部分(在视图区域中不可见的部分)。
剔除面属性的类型为CullFace,它表示 3D 形状的剔除面。您可以使用setCullFace()方法设置形状的剔除面,如下所示:
box.setCullFace(CullFace.NONE);
形状的笔触类型可以是:
None - 不执行剔除 (CullFace.NONE)。
Front - 剔除所有正面多边形 (CullFace.FRONT)。
Back - 剔除所有背面多边形 (StrokeType.BACK)。
默认情况下,三维形状的剔除面为 Back。
示例
以下程序是一个示例,演示了球体的各种剔除面。将此代码保存在名为SphereCullFace.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.stage.Stage; import javafx.scene.shape.Sphere; public class SphereCullFace extends Application { @Override public void start(Stage stage) { //Drawing Sphere1 Sphere sphere1 = new Sphere(); //Setting the radius of the Sphere sphere1.setRadius(50.0); //Setting the position of the sphere sphere1.setTranslateX(100); sphere1.setTranslateY(150); //setting the cull face of the sphere to front sphere1.setCullFace(CullFace.FRONT); //Drawing Sphere2 Sphere sphere2 = new Sphere(); //Setting the radius of the Sphere sphere2.setRadius(50.0); //Setting the position of the sphere sphere2.setTranslateX(300); sphere2.setTranslateY(150); //Setting the cull face of the sphere to back sphere2.setCullFace(CullFace.BACK); //Creating a Group object Group root = new Group(sphere1, sphere2); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Sphere"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls SphereCullFace.java java --module-path %PATH_TO_FX% --add-modules javafx.controls SphereCullFace
输出
执行后,上述程序将生成一个 JavaFX 窗口,显示两个球体,分别具有FRONT和BACK的剔除面值,如下所示:
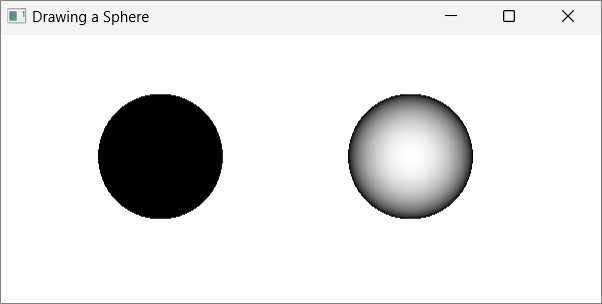
示例
以下程序是一个示例,演示了在长方体上使用 NONE 属性时不应用剔除面属性的情况。将此代码保存在名为BoxCullFace.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.stage.Stage; import javafx.scene.shape.Box; public class BoxCullFace extends Application { @Override public void start(Stage stage) { //Drawing Box1 Box box1 = new Box(); //Setting the dimensions of the Box box1.setWidth(100.0); box1.setHeight(100.0); box1.setDepth(100.0); //Setting the position of the box box1.setTranslateX(100); box1.setTranslateY(150); box1.setTranslateZ(0); //setting the cull face of the box to NONE box1.setCullFace(CullFace.NONE); //Creating a Group object Group root = new Group(box1); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Box"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BoxCullFace.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BoxCullFace
输出
执行后,上述程序将生成一个 JavaFX 窗口,显示一个长方体,其剔除面值为NONE,如下所示:
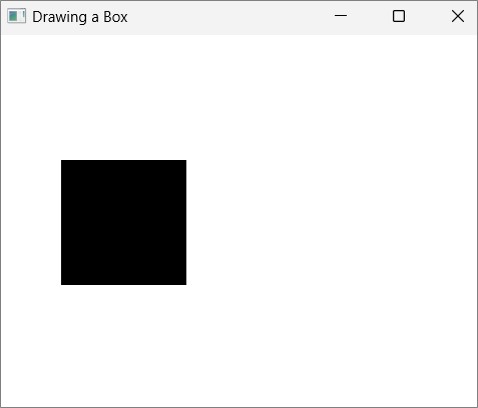
广告