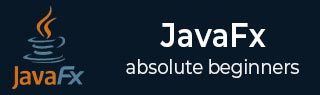
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维形状
- JavaFX - 二维形状
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线帽属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图片输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影阴影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维形状
- JavaFX - 三维形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 事件过滤器
事件过滤器允许您在事件处理的事件捕获阶段处理事件。事件处理中的事件捕获阶段是指事件在调度链中遍历所有节点的阶段。此调度链中的节点可以拥有一个或多个过滤器来处理事件,也可以根本没有过滤器。
事件过滤器在此事件捕获阶段处理事件,例如鼠标事件、滚动事件、键盘事件等。但是,这些事件过滤器需要在节点上注册,以便为在节点上生成的事件提供事件处理逻辑。
如果节点不包含事件过滤器,则事件将直接传递给目标节点。否则,单个过滤器可用于多个节点和事件类型。简单总结一下,事件过滤器用于使父节点能够为其子节点提供通用处理;以及拦截事件并阻止子节点对事件进行操作。
添加和删除事件过滤器
要向节点添加事件过滤器,您需要使用**Node**类的**addEventFilter()**方法注册此过滤器。
//Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Adding event Filter Circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);
同样,您可以使用如下所示的removeEventFilter()方法删除过滤器:
circle.removeEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler);
示例
以下是一个示例,演示了使用事件过滤器在 JavaFX 中进行事件处理。将此代码保存在名为**EventFiltersExample.java**的文件中。
import javafx.application.Application; import static javafx.application.Application.launch; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.input.MouseEvent; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.stage.Stage; public class EventFiltersExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(300.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(25.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Setting the text Text text = new Text("Click on the circle to change its color"); //Setting the font of the text text.setFont(Font.font(null, FontWeight.BOLD, 15)); //Setting the color of the text text.setFill(Color.CRIMSON); //setting the position of the text text.setX(150); text.setY(50); //Creating the mouse event handler EventHandler<MouseEvent> eventHandler = new EventHandler<MouseEvent>() { @Override public void handle(MouseEvent e) { System.out.println("Hello World"); circle.setFill(Color.DARKSLATEBLUE); } }; //Registering the event filter circle.addEventFilter(MouseEvent.MOUSE_CLICKED, eventHandler); //Creating a Group object Group root = new Group(circle, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting the fill color to the scene scene.setFill(Color.LAVENDER); //Setting title to the Stage stage.setTitle("Event Filters Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EventFiltersExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EventFiltersExample
输出
执行后,上述程序将生成一个如下图所示的 JavaFX 窗口。
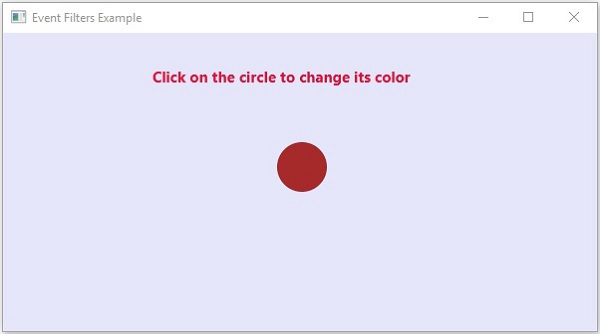
示例
我们已经看到了事件过滤器如何处理鼠标事件。现在,让我们尝试在其他事件(如键盘事件)上注册事件过滤器。将此代码保存在名为**EventFilterKeyboard.java**的文件中。
import javafx.application.Application; import javafx.event.EventHandler; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.input.KeyEvent; import javafx.scene.paint.Color; import javafx.stage.Stage; public class EventFilterKeyboard extends Application{ @Override public void start(Stage primaryStage) throws Exception { //Adding Labels and TextFileds to the scene Label label1 = new Label("Insert Key"); Label label2 = new Label("Event"); label1.setTranslateX(100); label1.setTranslateY(100); label2.setTranslateX(100); label2.setTranslateY(150); TextField text1 = new TextField(); TextField text2 = new TextField(); text1.setTranslateX(250); text1.setTranslateY(100); text2.setTranslateX(250); text2.setTranslateY(150); //Creating EventHandler Object EventHandler<KeyEvent> filter = new EventHandler<KeyEvent>() { @Override public void handle(KeyEvent event) { text2.setText("Event : "+event.getEventType()); text1.setText(event.getText()); event.consume(); } }; //Registering Event Filter for the event generated on text field text1.addEventFilter(KeyEvent.ANY, filter); //Setting Group and Scene Group root = new Group(); root.getChildren().addAll(label1,label2,text1,text2); Scene scene = new Scene(root, 500, 300); primaryStage.setScene(scene); primaryStage.setTitle("Adding Event Filter"); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EventFilterKeyboard.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EventFilterKeyboard
输出
执行后,上述程序将生成一个如下图所示的 JavaFX 窗口。
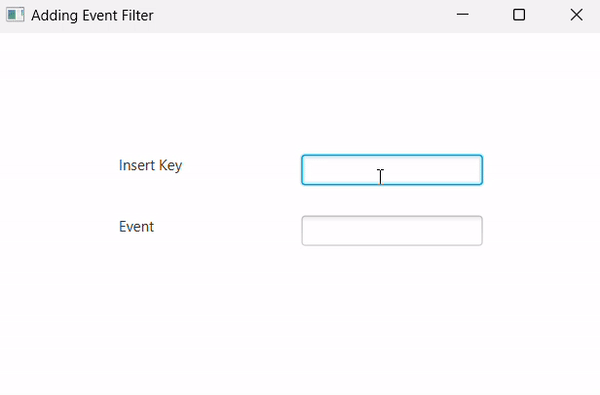
广告