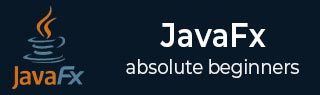
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 褐色调效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建线形图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 线形图
线形图或线图以一系列数据点(标记)的形式显示信息,这些数据点通过直线段连接。线形图显示数据在相等的时间频率下如何变化。
以下是描绘不同年份学校数量的线形图。
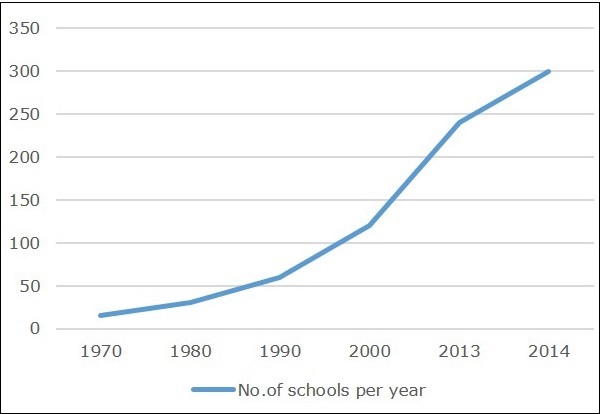
JavaFX 中的线形图
在 JavaFX 中,线形图由名为 LineChart 的类表示。此类属于 javafx.scene.chart 包。通过实例化此类,您可以在 JavaFX 中创建 LineChart 节点。
要在 JavaFX 中生成线形图,您应该按照以下步骤操作。
步骤 1:定义轴
在 Application 类的 start() 方法中定义线形图的 X 轴和 Y 轴,并为其设置标签。在我们的示例中,X 轴表示从 1960 年到 2020 年的年份,每十年有一个主要刻度标记。
public class ClassName extends Application { @Override public void start(Stage primaryStage) throws Exception { //Defining X axis NumberAxis xAxis = new NumberAxis(1960, 2020, 10); xAxis.setLabel("Years"); //Defining y axis NumberAxis yAxis = new NumberAxis(0, 350, 50); yAxis.setLabel("No.of schools"); } }
步骤 2:创建线形图
通过实例化 javafx.scene.chart 包中名为 LineChart 的类来创建线形图。在该类的构造函数中,传递表示在上一步骤中创建的 X 轴和 Y 轴的对象。
LineChart linechart = new LineChart(xAxis, yAxis);
步骤 3:准备数据
实例化 XYChart.Series 类。然后将数据(一系列 x 和 y 坐标)添加到此类的 Observable 列表中,如下所示:
XYChart.Series series = new XYChart.Series(); series.setName("No of schools in an year"); series.getData().add(new XYChart.Data(1970, 15)); series.getData().add(new XYChart.Data(1980, 30)); series.getData().add(new XYChart.Data(1990, 60)); series.getData().add(new XYChart.Data(2000, 120)); series.getData().add(new XYChart.Data(2013, 240)); series.getData().add(new XYChart.Data(2014, 300));
步骤 4:将数据添加到线形图
将上一步骤中准备的数据系列添加到线形图中,如下所示:
//Setting the data to Line chart linechart.getData().add(series);
步骤 5:创建 Group 对象
在 start() 方法中,通过实例化名为 Group 的类来创建一个组对象。这属于 javafx.scene 包。
将上一步骤中创建的 LineChart(节点)对象作为参数传递给 Group 类的构造函数。应执行此操作以将其添加到组中,如下所示:
Group root = new Group(linechart);
步骤 6:启动应用程序
最后,请按照以下步骤正确启动应用程序:
首先,通过将 Group 对象作为参数值传递给其构造函数来实例化名为 Scene 的类。在此构造函数中,您还可以将应用程序屏幕的尺寸作为可选参数传递。
然后,使用 Stage 类的 setTitle() 方法为舞台设置标题。
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到舞台。
使用名为 show() 的方法显示场景的内容。
最后,借助 launch() 方法启动应用程序。
示例
下表显示了从 1970 年到 2014 年某个地区学校的数量。
年份 | 学校数量 |
---|---|
1970 | 15 |
1980 | 30 |
1990 | 60 |
2000 | 120 |
2013 | 240 |
2014 | 300 |
以下是一个 Java 程序,它使用 JavaFX 生成一个描绘上述数据的线形图。
将此代码保存在名为 LineChartExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.chart.LineChart; import javafx.scene.chart.NumberAxis; import javafx.scene.chart.XYChart; public class LineChartExample extends Application { @Override public void start(Stage stage) { //Defining the x axis NumberAxis xAxis = new NumberAxis(1960, 2020, 10); xAxis.setLabel("Years"); //Defining the y axis NumberAxis yAxis = new NumberAxis (0, 350, 50); yAxis.setLabel("No.of schools"); //Creating the line chart LineChart linechart = new LineChart(xAxis, yAxis); //Prepare XYChart.Series objects by setting data XYChart.Series series = new XYChart.Series(); series.setName("No of schools in an year"); series.getData().add(new XYChart.Data(1970, 15)); series.getData().add(new XYChart.Data(1980, 30)); series.getData().add(new XYChart.Data(1990, 60)); series.getData().add(new XYChart.Data(2000, 120)); series.getData().add(new XYChart.Data(2013, 240)); series.getData().add(new XYChart.Data(2014, 300)); //Setting the data to Line chart linechart.getData().add(series); //Creating a Group object Group root = new Group(linechart); //Creating a scene object Scene scene = new Scene(root, 600, 400); //Setting title to the Stage stage.setTitle("Line Chart"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls LineChartExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls LineChartExample
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的线形图。
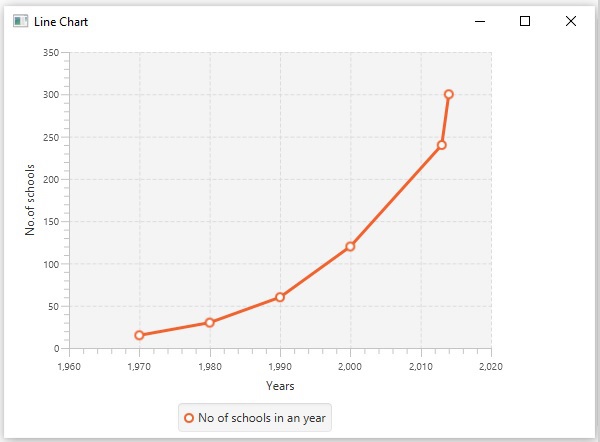
示例
下表说明了每月销售的电子产品数量。
产品 | 销量(每月) |
---|---|
笔记本电脑 | 176 |
电视 | 30 |
手机 | 540 |
智能手表 | 250 |
MacBook | 60 |
在另一个示例中,使用 JavaFX 生成一个描绘上述数据的线形图。将此代码保存在名为 LineChartItems.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.chart.LineChart; import javafx.scene.chart.NumberAxis; import javafx.scene.chart.CategoryAxis; import javafx.scene.chart.XYChart; public class LineChartItems extends Application { @Override public void start(Stage stage) { //Defining the x axis CategoryAxis xAxis = new CategoryAxis(); xAxis.setLabel("Items"); //Defining the y axis NumberAxis yAxis = new NumberAxis(); yAxis.setLabel("Sales (per month)"); //Creating the line chart LineChartlinechart = new LineChart (xAxis, yAxis); //Prepare XYChart.Series objects by setting data XYChart.Series series = new XYChart.Series(); series.setName("Items sold per month"); series.getData().add(new XYChart.Data("Laptop", 176)); series.getData().add(new XYChart.Data("TV", 30)); series.getData().add(new XYChart.Data("Mobile", 540)); series.getData().add(new XYChart.Data("Smart Watch", 250)); series.getData().add(new XYChart.Data("MacBook", 60)); //Creating a scene object Scene scene = new Scene(linechart, 600, 400); //Setting the data to Line chart linechart.getData().add(series); //Setting title to the Stage stage.setTitle("Line Chart"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls LineChartItems.java java --module-path %PATH_TO_FX% --add-modules javafx.controls LineChartItems
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的线形图。
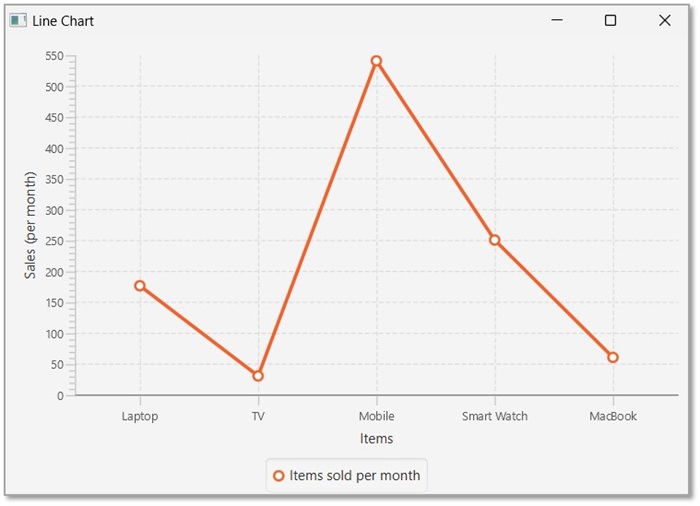