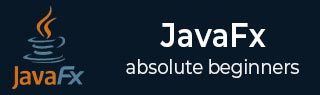
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 辉光效果
- JavaFX - 发光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局窗格
- JavaFX - 布局窗格
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - FlowPane 布局
JavaFX 中的 FlowPane 布局
FlowPane 布局将其所有节点排列成流。在水平 FlowPane 中,元素根据其高度进行换行,而在垂直 FlowPane 中,元素根据其宽度进行换行。
在 JavaFX 中,名为 FlowPane 的类(位于 javafx.scene.layout 包中)表示 FlowPane。要在我们的 JavaFX 应用程序中创建 FlowPane 布局,请使用以下任何构造函数实例化此类:
FlowPane() - 它构造一个新的水平 FlowPane 布局。
FlowPane(double hGap, double vGap) - 它创建一个新的水平 FlowPane 布局,并具有指定的 hGap 和 vGap。
FlowPane(double hGap, double vGap, Node childNodes) - 构造一个水平 FlowPane 布局,并具有指定的 hGap、vGap 和节点。
FlowPane(Orientation orientation) - 它创建一个新的 FlowPane 布局,并具有指定的 orientation。它可以是 HORIZONTAL 或 VERTICAL。
此类包含以下属性:
序号 | 属性和描述 |
---|---|
1 | alignment
此属性表示 FlowPane 内容的对齐方式。我们可以使用 setter 方法 setAllignment() 设置此属性。 |
2 | columnHalignment
此属性表示垂直 FlowPane 中节点的水平对齐方式。 |
3 | rowValignment
此属性表示水平 FlowPane 中节点的垂直对齐方式。 |
4 | Hgap
此属性为 double 类型,它表示 FlowPane 的行/列之间的水平间距。 |
5 | Orientation
此属性表示 FlowPane 的方向。 |
6 | Vgap
此属性为 double 类型,它表示 FlowPane 的行/列之间的垂直间距。 |
示例
以下程序是 FlowPane 布局的一个示例。在此,我们将四个按钮插入水平 FlowPane 中。将此代码保存在名为 FlowPaneExample.java 的文件中。
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.FlowPane; import javafx.scene.shape.Sphere; import javafx.stage.Stage; public class FlowPaneExample extends Application { @Override public void start(Stage stage) { //Creating button1 Button button1 = new Button("Button1"); //Creating button2 Button button2 = new Button("Button2"); //Creating button3 Button button3 = new Button("Button3"); //Creating button4 Button button4 = new Button("Button4"); //Creating a Flow Pane FlowPane flowPane = new FlowPane(); //Setting the horizontal gap between the nodes flowPane.setHgap(25); //Setting the margin of the pane flowPane.setMargin(button1, new Insets(20, 0, 20, 20)); //Adding all the nodes to the flow pane flowPane.getChildren().addAll(button1, button2, button3, button4); //Creating a scene object Scene scene = new Scene(flowPane, 400, 300); //Setting title to the Stage stage.setTitle("Flow Pane Example in JavaFX"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls FlowPaneExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls FlowPaneExample
输出
执行后,上述程序将生成一个 JavaFX 窗口,如下所示。
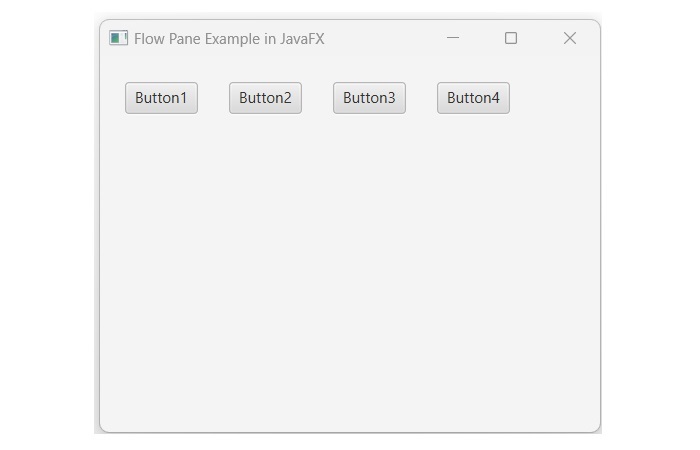
示例
在以下示例中,我们将创建一个方向为垂直的 FlowPane。要设置方向,我们将使用 FlowPane 类的带参数构造函数,并将 Orientation.VERTICAL 枚举值作为参数传递。将此代码保存在名为 JavafxFlowpane.java 的文件中。
import javafx.application.Application; import javafx.geometry.Orientation; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.FlowPane; import javafx.scene.shape.Sphere; import javafx.stage.Stage; public class JavafxFlowpane extends Application { @Override public void start(Stage stage) { //Creating button1 Button button1 = new Button("Button1"); //Creating button2 Button button2 = new Button("Button2"); //Creating button3 Button button3 = new Button("Button3"); //Creating button4 Button button4 = new Button("Button4"); //Creating a Flow Pane FlowPane flowPane = new FlowPane(Orientation.VERTICAL); //Setting the horizontal gap between the nodes flowPane.setVgap(15); //Setting the margin of the pane flowPane.setAlignment(Pos.CENTER); //Adding all the nodes to the flow pane flowPane.getChildren().addAll(button1, button2, button3, button4); //Creating a scene object Scene scene = new Scene(flowPane, 400, 300); //Setting title to the Stage stage.setTitle("Flow Pane Example in JavaFX"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
要从命令提示符编译并执行保存的 java 文件,请使用以下命令:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxFlowpane.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxFlowpane
输出
当我们执行上述 Java 程序时,它将生成一个 JavaFX 窗口,显示以下输出:
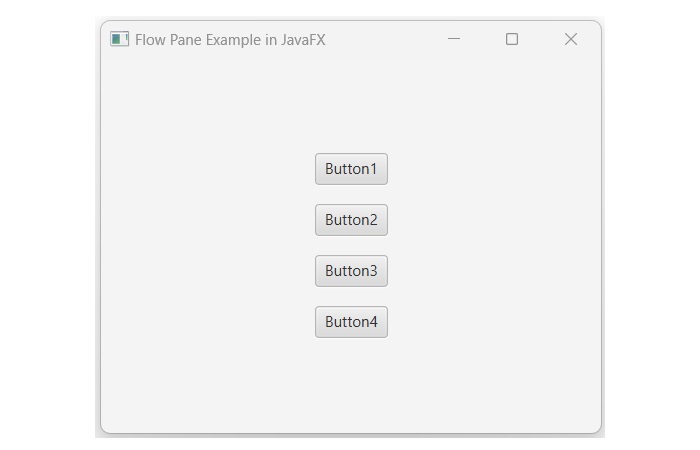