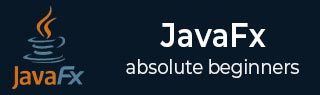
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 泛光效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 选项卡窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局窗格
- JavaFX - 布局窗格
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 透视变换
通常,2D 对象是指只能在二维平面上绘制,并且仅用两个维度进行测量的对象。但是,使用 JavaFX 应用程序,您可以提供 2D 对象的 3D 错觉。此效果称为透视变换效果。
透视变换效果将在 Z 轴方向创建透视,使对象看起来可以在 XYZ 平面上测量,而实际上它仅在 XY 平面上测量。此效果提供非仿射变换,其中源对象中直线的直线度在输出对象中得以保留。但是,平行性会丢失;与仿射变换不同。
仿射变换是一种线性变换,其中点、直线和平行性从源图像到输出图像都得以保留。
您可以使用 javafx.scene.effect 包中的 PerspectiveTransform 类将此效果应用于 JavaFX 节点。此类具有以下属性:
input - 此效果的输入。
llx - 输出位置的 x 坐标,源的左下角映射到该位置。
lly - 输出位置的 y 坐标,源的左下角映射到该位置。
lrx - 输出位置的 x 坐标,源的右下角映射到该位置。
lry - 输出位置的 y 坐标,源的右下角映射到该位置。
ulx - 输出位置的 x 坐标,源的左上角映射到该位置。
uly - 输出位置的 y 坐标,源的左上角映射到该位置。
urx - 输出位置的 x 坐标,源的右上角映射到该位置。
ury - 输出位置的 y 坐标,源的右上角映射到该位置。
示例
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Rectangle; import javafx.scene.effect.Effect; import javafx.scene.effect.PerspectiveTransform; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; public class PerspectiveTransformExample extends Application { @Override public void start(Stage stage) { PerspectiveTransform prst = new PerspectiveTransform(); prst.setUlx(10.0); prst.setUly(10.0); prst.setUrx(310.0); prst.setUry(40.0); prst.setLrx(310.0); prst.setLry(60.0); prst.setLlx(10.0); prst.setLly(90.0); Group g = new Group(); g.setEffect(prst); g.setCache(true); Rectangle rect = new Rectangle(); rect.setX(10.0); rect.setY(10.0); rect.setWidth(280.0); rect.setHeight(80.0); rect.setFill(Color.BLUE); Text text = new Text(); text.setX(20.0); text.setY(65.0); text.setText("JavaFX App"); text.setFill(Color.WHITE); text.setFont(Font.font(null, FontWeight.BOLD, 36)); g.getChildren().addAll(rect, text); //Creating a Group object Group root = new Group(g); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Perspective Transform Effect"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls PerspectiveTransformExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls PerspectiveTransformExample
输出
执行后,上述程序将生成一个 JavaFX 窗口,如下所示。
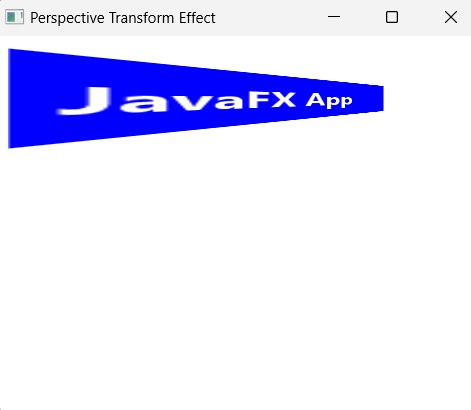
广告