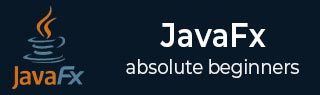
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境配置
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用
- JavaFX 二维图形
- JavaFX - 二维图形
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 特效
- JavaFX - 特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图片输入特效
- JavaFX - 混合特效
- JavaFX - 光晕特效
- JavaFX - 辉光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 棕褐色调特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内阴影特效
- JavaFX - 照明特效
- JavaFX - 远光源特效
- JavaFX - 聚光灯特效
- JavaFX - 点光源特效
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维图形
- JavaFX - 三维图形
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图 (ListView)
- JavaFX - 手风琴 (Accordion)
- JavaFX - 按钮栏 (ButtonBar)
- JavaFX - 选择框 (ChoiceBox)
- JavaFX - HTML 编辑器 (HTMLEditor)
- JavaFX - 菜单栏 (MenuBar)
- JavaFX - 分页 (Pagination)
- JavaFX - 进度指示器 (ProgressIndicator)
- JavaFX - 滚动面板 (ScrollPane)
- JavaFX - 分隔符 (Separator)
- JavaFX - 滑块 (Slider)
- JavaFX - 微调器 (Spinner)
- JavaFX - 分割面板 (SplitPane)
- JavaFX - 表格视图 (TableView)
- JavaFX - 标签页面板 (TabPane)
- JavaFX - 工具栏 (ToolBar)
- JavaFX - 树视图 (TreeView)
- JavaFX - 标签 (Label)
- JavaFX - 复选框 (CheckBox)
- JavaFX - 单选按钮 (RadioButton)
- JavaFX - 文本字段 (TextField)
- JavaFX - 密码字段 (PasswordField)
- JavaFX - 文件选择器 (FileChooser)
- JavaFX - 超链接 (Hyperlink)
- JavaFX - 工具提示 (Tooltip)
- JavaFX - 警报框 (Alert)
- JavaFX - 日期选择器 (DatePicker)
- JavaFX - 文本区域 (TextArea)
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建柱状图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠柱状图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 表格视图 (TableView)
TableView 是一种图形用户界面组件,用于以表格形式显示数据。类似于典型的表格,TableView 包含列、行和单元格,每个单元格可以容纳任何类型的数据。一般来说,表格表示如下所示:
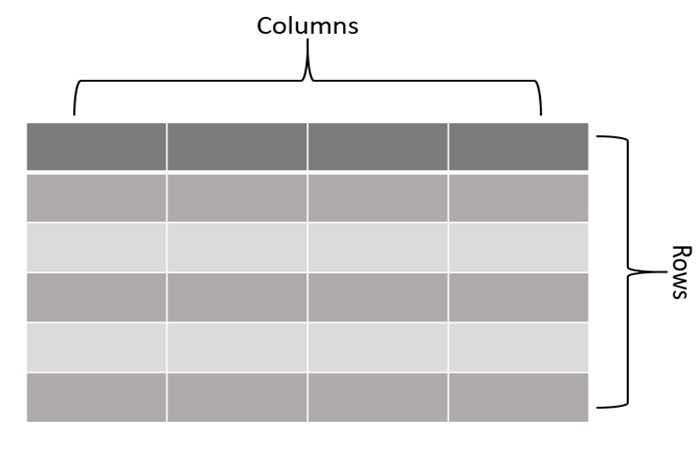
JavaFX 中的 TableView
在 JavaFX 中,表格视图由TableView 类表示。此类是名为javafx.scene.control 包的一部分。通过实例化此类,我们可以在 JavaFX 中创建一个 TableView 节点。它有两个构造函数:
TableView() - 这是默认构造函数,用于创建一个没有任何预定义数据的表格视图。
TableView(ObservableList items) - 这是 TableView 类的参数化构造函数,它接受项目列表并创建一个包含指定项目的新表格视图。
在 JavaFX 中创建 TableView 的步骤
按照以下步骤在 JavaFX 中创建表格视图。
步骤 1:创建一个类来表示表格中的数据
首先,我们需要创建一个类来表示要在 TableView 中显示的数据。此类应具有与表格列对应的属性。在这里,我们将使用下面的代码块创建一个名为 movie 的类,其属性为标题、演员、价格和评分:
// The data model class public static class Movie { private final SimpleStringProperty title; private final SimpleStringProperty actor; private final SimpleDoubleProperty price; private final SimpleIntegerProperty rating;
步骤 2:为 TableView 创建列
创建 TableColumn 对象,并使用setCellValueFactory() 方法设置其单元格值。单元格值工厂是一个函数,它告诉列如何从上面声明的数据模型类中提取数据。以下代码块显示了如何创建列:
// Creating columns for the TableView TableColumn<Movie, String> titleColumn = new TableColumn<>("Movie Name"); titleColumn.setCellValueFactory(cellData -> cellData.getValue().titleProperty()); TableColumn<Movie, String> actorColumn = new TableColumn<>("Actor"); actorColumn.setCellValueFactory(cellData -> cellData.getValue().authorProperty()); TableColumn<Movie, Number> priceColumn = new TableColumn<>("Price"); priceColumn.setCellValueFactory(cellData -> cellData.getValue().priceProperty()); TableColumn<Movie, Number> ratingColumn = new TableColumn<>("IMDB Rating"); ratingColumn.setCellValueFactory(cellData -> cellData.getValue().ratingProperty());
步骤 3:实例化 TableView 类
实例化javafx.scene.control 包的TableView 类,无需向其构造函数传递任何参数值,并使用以下代码块添加所有列:
// Creating a TableView TableView<Movie> tableView = new TableView<>(); // Adding the columns to the TableView tableView.getColumns().addAll(titleColumn, actorColumn, priceColumn, ratingColumn);
步骤 4:启动应用程序
创建表格视图并添加所有数据后,请按照以下步骤正确启动应用程序:
首先,通过将 TableView 对象作为参数值传递给其构造函数来实例化名为BorderPane 的类。
然后,通过将 BorderPane 对象作为参数值传递给其构造函数来实例化名为Scene 的类。我们还可以将应用程序屏幕的尺寸作为可选参数传递给此构造函数。
然后,使用Stage 类的setTitle() 方法设置阶段的标题。
现在,使用名为Stage 的类的setScene() 方法将 Scene 对象添加到阶段。
使用名为show() 的方法显示场景的内容。
最后,在launch() 方法的帮助下启动应用程序。
示例
以下是将使用 JavaFX 创建 TableView 的程序。将此代码保存在名为JavafxTableview.java 的文件中。
import javafx.application.Application; import javafx.beans.property.SimpleDoubleProperty; import javafx.beans.property.SimpleIntegerProperty; import javafx.beans.property.SimpleStringProperty; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.scene.Scene; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.layout.BorderPane; import javafx.stage.Stage; public class JavafxTableview extends Application { // The data model class public static class Movie { private final SimpleStringProperty title; private final SimpleStringProperty actor; private final SimpleDoubleProperty price; private final SimpleIntegerProperty rating; // constructor public Movie(String title, String actor, double price, int rating) { this.title = new SimpleStringProperty(title); this.actor = new SimpleStringProperty(actor); this.price = new SimpleDoubleProperty(price); this.rating = new SimpleIntegerProperty(rating); } // getters and setters to access the data public SimpleStringProperty titleProperty() { return title; } public SimpleStringProperty authorProperty() { return actor; } public SimpleDoubleProperty priceProperty() { return price; } public SimpleIntegerProperty ratingProperty() { return rating; } } // main method starts here @Override public void start(Stage stage) throws Exception { // adding some sample data ObservableList<Movie> movies = FXCollections.observableArrayList( new Movie("The Batman", "Robert Pattinson", 299, 7), new Movie("John Wick: Chapter 4", "Keanu Reeves", 199, 7), new Movie("12th Fail", "Vikrant Massey", 199, 9), new Movie("Money Heist", "Alvaro Morte", 499, 8), new Movie("The Family Man", "Manoj Bajpayee", 399, 8) ); // Creating a TableView TableView<Movie> tableView = new TableView<>(); // Creating columns for the TableView TableColumn<Movie, String> titleColumn = new TableColumn<>("Movie Name"); titleColumn.setCellValueFactory(cellData -> cellData.getValue().titleProperty()); TableColumn<Movie, String> actorColumn = new TableColumn<>("Actor"); actorColumn.setCellValueFactory(cellData -> cellData.getValue().authorProperty()); TableColumn<Movie, Number> priceColumn = new TableColumn<>("Price"); priceColumn.setCellValueFactory(cellData -> cellData.getValue().priceProperty()); TableColumn<Movie, Number> ratingColumn = new TableColumn<>("IMDB Rating"); ratingColumn.setCellValueFactory(cellData -> cellData.getValue().ratingProperty()); // Adding the columns to the TableView tableView.getColumns().addAll(titleColumn, actorColumn, priceColumn, ratingColumn); // Set the items of the TableView tableView.setItems(movies); // Create a BorderPane and set the TableView as its center BorderPane root = new BorderPane(); root.setCenter(tableView); // Create a Scene and set it on the Stage Scene scene = new Scene(root, 400, 300); stage.setTitle("Table View in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTableview.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTableview
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示一个 Tableview,其中包含如下所示的电影列表。
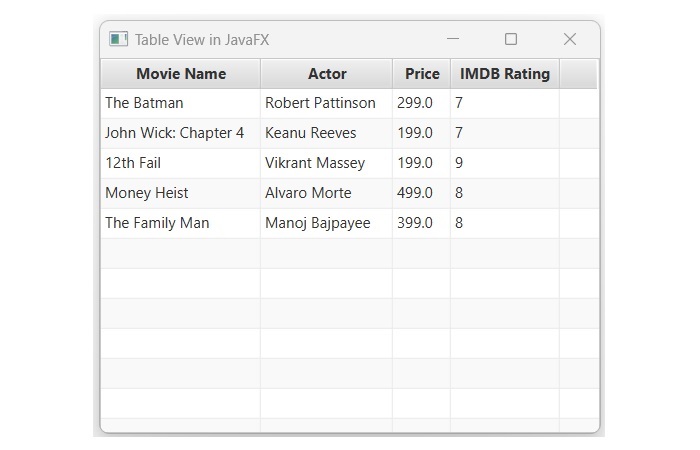
创建 TableView 的嵌套列
有时,我们需要在多个子列中显示单个列的数据。当单个实体具有多个属性时,这很常见,例如,一个人可以有多个联系电话或电子邮件帐户。在这种情况下,我们创建所需的列,并使用getColumns() 方法将它们添加到父列中,如下面的 JavaFX 代码所示。将此代码保存在名为NestedTableview.java 的文件中。
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.layout.VBox; import javafx.scene.text.Font; import javafx.stage.Stage; public class NestedTableview extends Application { @Override public void start(Stage stage) { // Defining the columns TableColumn firstCol = new TableColumn("Column One"); TableColumn secondCol = new TableColumn("Column Two"); // creating sub-columns of secondCol TableColumn firstSubCol = new TableColumn("Sub Col1"); TableColumn secondSubCol = new TableColumn("Sub Col2"); // adding the sub-columns to secondCol secondCol.getColumns().addAll(firstSubCol, secondSubCol); TableColumn lastCol = new TableColumn("Column Three"); // instantiating the TableView class TableView newTableview = new TableView(); newTableview.getColumns().addAll(firstCol, secondCol, lastCol); VBox root = new VBox(); root.setSpacing(5); root.getChildren().addAll(newTableview); // Create a Scene and set it on the Stage Scene scene = new Scene(root, 400, 300); stage.setTitle("Nested TableView in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
要从命令提示符编译并执行保存的 Java 文件,请使用以下命令:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls NestedTableview.java java --module-path %PATH_TO_FX% --add-modules javafx.controls NestedTableview
输出
执行上述代码后,将生成以下输出。
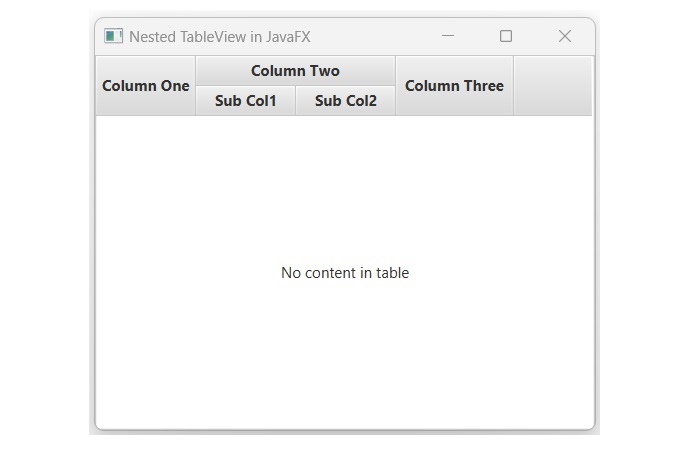