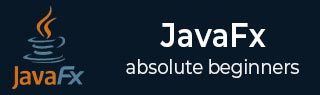
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 特效
- JavaFX - 特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图像输入特效
- JavaFX - 混合特效
- JavaFX - 光晕特效
- JavaFX - 辉光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 褐色调特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内部阴影特效
- JavaFX - 照明特效
- JavaFX - Light.Distant 特效
- JavaFX - Light.Spot 特效
- JavaFX - Point.Spot 特效
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建柱状图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠柱状图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 并集运算
从数学角度来看,并集运算在集合论的概念中得到了根本性的应用。此运算定义为将两个不同的集合组合成一个集合。在此,一个集合的所有元素都合并到另一个集合中,而不管是否存在重复。然后,这个概念被计算机编程中的各种技术所采用。
例如,在 SQL 中执行并集运算时,会将两个或多个结果集组合成一个公共结果集。它也用作编程语言(如 C、C++、Java、Python 等)中的运算符或方法。
类似地,JavaFX 也提供对 2D 形状的并集运算。
JavaFX 中的并集运算
JavaFX 提供了可以对 2D 形状执行的并集运算。在此,两个或多个形状的区域组合在一起以形成更大更复杂的形状。因此,并集运算将两个或多个形状作为输入,并返回它们占据的组合区域,如下所示。
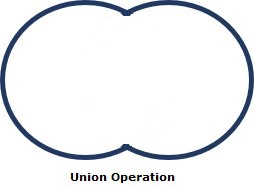
您可以使用名为 union() 的方法对形状执行并集运算。由于这是一个静态方法,因此您应使用类名(Shape 或其子类)调用它,如下所示。
Shape shape = Shape.subtract(circle1, circle2);
示例
以下是并集运算的示例。在此,我们绘制了两个圆形并对其执行并集运算。将此代码保存在名为 unionExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.shape.Circle; import javafx.scene.shape.Shape; public class UnionExample extends Application { @Override public void start(Stage stage) { //Drawing Circle1 Circle circle1 = new Circle(); //Setting the position of the circle circle1.setCenterX(250.0f); circle1.setCenterY(135.0f); //Setting the radius of the circle circle1.setRadius(100.0f); //Setting the color of the circle circle1.setFill(Color.DARKSLATEBLUE); //Drawing Circle2 Circle circle2 = new Circle(); //Setting the position of the circle circle2.setCenterX(350.0f); circle2.setCenterY(135.0f); //Setting the radius of the circle circle2.setRadius(100.0f); //Setting the color of the circle circle2.setFill(Color.BLUE); //Performing union operation on the circle Shape shape = Shape.union(circle1, circle2); //Setting the fill color to the result shape.setFill(Color.DARKSLATEBLUE); //Creating a Group object Group root = new Group(shape); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Union Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls UnionExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls UnionExample
输出
执行上述程序后,将生成一个 JavaFX 窗口,显示以下输出:
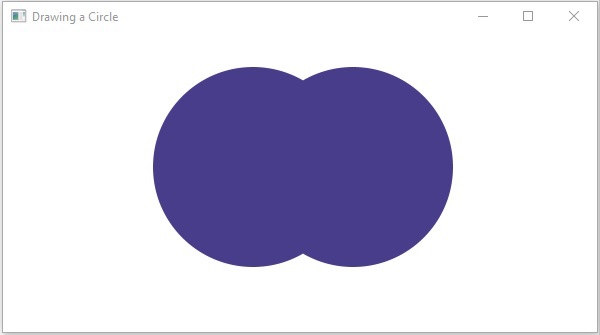
示例
让我们尝试对除圆形之外的其他形状(如椭圆形)执行并集运算。将此文件保存在名为 EllipseUnionOperation.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.shape.Ellipse; import javafx.scene.shape.Shape; public class EllipseUnionOperation extends Application { @Override public void start(Stage stage) { Ellipse ellipse1 = new Ellipse(); ellipse1.setCenterX(250.0f); ellipse1.setCenterY(100.0f); ellipse1.setRadiusX(150.0f); ellipse1.setRadiusY(75.0f); ellipse1.setFill(Color.BLUE); Ellipse ellipse2 = new Ellipse(); ellipse2.setCenterX(350.0f); ellipse2.setCenterY(100.0f); ellipse2.setRadiusX(150.0f); ellipse2.setRadiusY(75.0f); ellipse2.setFill(Color.RED); Shape shape = Shape.union(ellipse1, ellipse2); //Setting the fill color to the result shape.setFill(Color.DARKSLATEBLUE); //Creating a Group object Group root = new Group(shape); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Union Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseUnionOperation.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseUnionOperation
输出
执行上述程序后,将生成一个 JavaFX 窗口,显示以下输出:
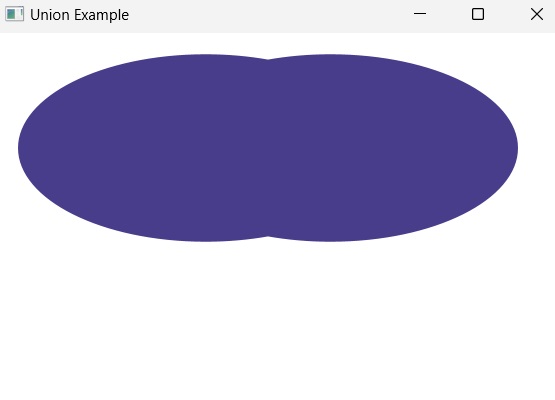
广告