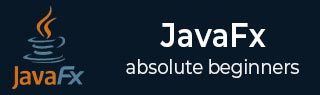
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 辉光效果
- JavaFX - 闪光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - 光源.远光效果
- JavaFX - 光源.聚光效果
- JavaFX - 光源.点光效果
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 选项卡窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局窗格
- JavaFX - 布局窗格
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 径向渐变图案
类似线性渐变图案,还有各种类型的渐变图案,它们描述了渐变的流动方式。其他类型包括径向、角形、反射和菱形渐变图案。在本章中,我们将学习径向渐变图案。
径向渐变图案是另一种类型的渐变图案,它从中心点开始,以圆形方式流动到半径处。简单来说,径向渐变包含以同心圆形式表示的两个或多个颜色停止点。
JavaFX 还提供这种类型的颜色图案来填充圆形类型的 2D 形状,例如正圆形或椭圆形等。
应用径向渐变图案
要将径向渐变图案应用于节点,请实例化 GradientPattern 类并将它的对象传递给 setFill()、setStroke() 方法。
此类的构造函数接受一些参数,其中一些参数是 -
startX, startY - 这些双精度属性表示渐变起点的 x 和 y 坐标。
endX, endY - 这些双精度属性表示渐变终点的 x 和 y 坐标。
cycleMethod - 此参数定义如何通过起点和终点定义颜色渐变边界外的区域,以及如何填充这些区域。
proportional - 这是一个布尔变量;将此属性设置为 true 时,将开始和结束位置设置为比例。
Stops - 此参数定义沿渐变线的颜色停止点。
//Setting the radial gradient Stop[] stops = new Stop[] { new Stop(0.0, Color.WHITE), new Stop(0.3, Color.RED), new Stop(1.0, Color.DARKRED) }; RadialGradient radialGradient = new RadialGradient(0, 0, 300, 178, 60, false, CycleMethod.NO_CYCLE, stops);
示例
以下示例演示了如何在 JavaFX 中将径向渐变图案应用于节点。在这里,我们创建了一个圆形和一个文本节点,并向它们应用渐变图案。
将此代码保存在名为 RadialGradientExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.paint.CycleMethod; import javafx.scene.paint.RadialGradient; import javafx.scene.paint.Stop; import javafx.stage.Stage; import javafx.scene.shape.Circle; import javafx.scene.text.Font; import javafx.scene.text.Text; public class RadialGradientExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the properties of the circle circle.setCenterX(300.0f); circle.setCenterY(180.0f); circle.setRadius(90.0f); //Drawing a text Text text = new Text("This is a colored circle"); //Setting the font of the text text.setFont(Font.font("Edwardian Script ITC", 50)); //Setting the position of the text text.setX(155); text.setY(50); //Setting the radial gradient Stop[] stops = new Stop[] { new Stop(0.0, Color.WHITE), new Stop(0.3, Color.RED), new Stop(1.0, Color.DARKRED) }; RadialGradient radialGradient = new RadialGradient(0, 0, 300, 178, 60, false, CycleMethod.NO_CYCLE, stops); //Setting the radial gradient to the circle and text circle.setFill(radialGradient); text.setFill(radialGradient); //Creating a Group object Group root = new Group(circle, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Radial Gradient Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]) { launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RadialGradientExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls RadialGradientExample
输出
执行后,上述程序生成一个 JavaFX 窗口,如下所示 -
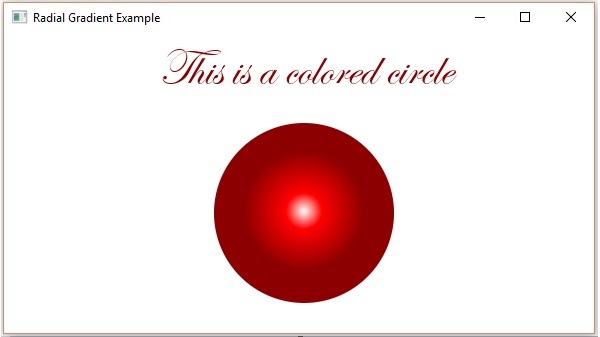
示例
径向渐变图案不适用于非圆形形状;也就是说,您只能在圆形和椭圆形形状上应用径向渐变。
在下面的示例中,让我们尝试将径向渐变图案应用于矩形形状。将此代码保存在名为 RectangleRadialGradient.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.paint.CycleMethod; import javafx.scene.paint.RadialGradient; import javafx.scene.paint.Stop; import javafx.stage.Stage; import javafx.scene.shape.Rectangle; public class RectangleRadialGradient extends Application { @Override public void start(Stage stage) { Rectangle rct = new Rectangle(50.0f, 50.0f, 200.0f, 100.0f); Stop[] stops = new Stop[] { new Stop(0.0, Color.WHITE), new Stop(0.3, Color.RED), new Stop(1.0, Color.DARKRED) }; RadialGradient radialGradient = new RadialGradient(0, 0, 300, 178, 60, false, CycleMethod.NO_CYCLE, stops); rct.setFill(radialGradient); Group root = new Group(rct); Scene scene = new Scene(root, 300, 300); stage.setTitle("Radial Gradient Example"); stage.setScene(scene); stage.show(); } public static void main(String args[]) { launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleRadialGradient.java java --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleRadialGradient
输出
执行后,您将只看到形状中最外层的渐变颜色,如下所示 -
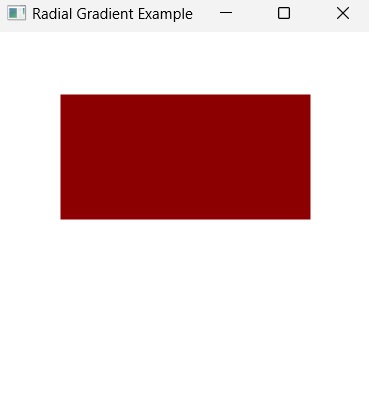