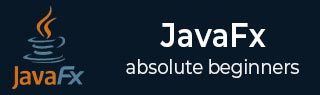
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 辉光效果
- JavaFX - 发光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转转换
- JavaFX - 缩放转换
- JavaFX - 平移转换
- JavaFX - 淡入淡出转换
- JavaFX - 填充转换
- JavaFX - 描边转换
- JavaFX - 顺序转换
- JavaFX - 并行转换
- JavaFX - 暂停转换
- JavaFX - 路径转换
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局窗格
- JavaFX - 布局窗格
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 绘制椭圆
椭圆由两个点定义,每个点称为焦点。如果取椭圆上的任意一点,则到焦点点的距离之和为常数。椭圆的大小由这两个距离之和决定。这两个距离之和等于长轴的长度(椭圆的最长直径)。实际上,圆是椭圆的特例。
椭圆具有三个属性,它们是:
中心 - 椭圆内部的一个点,它是连接两个焦点的线段的中点。长轴和短轴的交点。
长轴 - 椭圆的最长直径。
短轴 - 椭圆的最短直径。
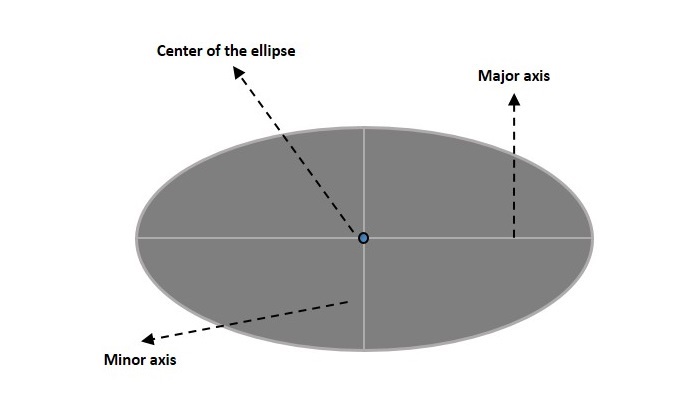
JavaFX 中的椭圆
在 JavaFX 中,椭圆由名为 Ellipse 的类表示。此类属于 javafx.scene.shape 包。
通过实例化此类,您可以在 JavaFX 中创建椭圆节点。
此类具有 4 个 double 数据类型的属性,即:
centerX - 椭圆中心点的 x 坐标(以像素为单位)。
centerY - 椭圆中心点的 y 坐标(以像素为单位)。
radiusX - 椭圆的宽度(以像素为单位)。
radiusY - 椭圆的高度(以像素为单位)。
要绘制椭圆,您需要将值传递给这些属性,可以通过按相同顺序将它们传递给此类的构造函数,或者使用 setter 方法。
绘制椭圆的步骤
请按照以下步骤在 JavaFX 中绘制椭圆。
步骤 1:创建椭圆
您可以在 JavaFX 中通过实例化名为 Ellipse 的类来创建椭圆,该类属于 javafx.scene.shape 包,位于 start() 方法内部。您可以按如下方式实例化此类。
public class ClassName extends Application { public void start(Stage primaryStage) throws Exception { //Creating an Ellipse object Ellipse ellipse = new Ellipse(); } }
步骤 2:设置椭圆的属性
指定椭圆中心的 x、y 坐标→椭圆沿 x 轴和 y 轴的宽度(长轴和短轴),通过设置 X、Y、RadiusX 和 RadiusY 属性来设置圆。
这可以通过使用它们各自的 setter 方法来完成,如下面的代码块所示。
ellipse.setCenterX(300.0f); ellipse.setCenterY(150.0f); ellipse.setRadiusX(150.0f); ellipse.setRadiusY(75.0f);
步骤 3:创建 Group 对象
在 start() 方法中,通过实例化名为 Group 的类来创建一个组对象,该类属于 javafx.scene 包。通过将前面步骤中创建的 Ellipse(节点)对象作为参数传递给 Group 类的构造函数来实例化此类。这应该为了将其添加到组中,如下面的代码块所示:
Group root = new Group(ellipse);
步骤 4:启动应用程序
创建 2D 对象后,请按照以下步骤正确启动应用程序:
首先,通过将 Group 对象作为参数值传递给其构造函数来实例化名为 Scene 的类。对于此构造函数,您还可以将应用程序屏幕的尺寸作为可选参数传递。
然后,使用 Stage 类的 setTitle() 方法设置舞台的标题。
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到舞台。
使用名为 show() 的方法显示场景的内容。
最后,使用 launch() 方法启动应用程序。
示例
以下是一个使用 JavaFX 生成椭圆的程序。将此代码保存在名为 EllipseExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Ellipse; public class EllipseExample extends Application { @Override public void start(Stage stage) { //Drawing an ellipse Ellipse ellipse = new Ellipse(); //Setting the properties of the ellipse ellipse.setCenterX(300.0f); ellipse.setCenterY(150.0f); ellipse.setRadiusX(150.0f); ellipse.setRadiusY(75.0f); //Creating a Group object Group root = new Group(ellipse); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing an Ellipse"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseExample
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示如下所示的椭圆。
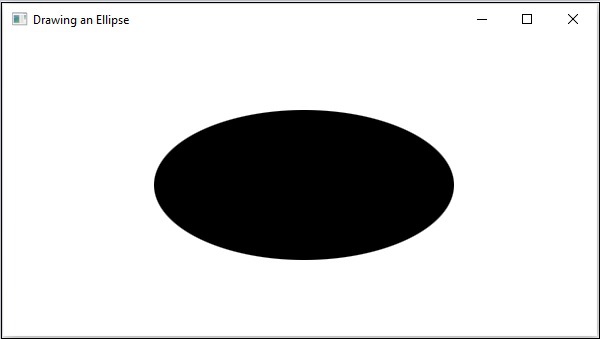
示例
在下面给出的另一个示例中,让我们尝试绘制一个圆形行星的椭圆轨道。将此文件命名为 PlanetOrbit.java。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Ellipse; import javafx.scene.shape.Circle; import javafx.scene.paint.Color; import javafx.animation.PathTransition; import javafx.application.Application; import javafx.stage.Stage; import javafx.util.Duration; public class PlanetOrbit extends Application { @Override public void start(Stage stage) { //Drawing an orbit Ellipse orbit = new Ellipse(); orbit.setFill(Color.WHITE); orbit.setStroke(Color.BLACK); //Setting the properties of the ellipse orbit.setCenterX(300.0f); orbit.setCenterY(150.0f); orbit.setRadiusX(150.0f); orbit.setRadiusY(100.0f); // Drawing a circular planet Circle planet = new Circle(300.0f, 50.0f, 40.0f); //Creating the animation PathTransition pathTransition = new PathTransition(); pathTransition.setDuration(Duration.millis(1000)); pathTransition.setNode(planet); pathTransition.setPath(orbit); pathTransition.setOrientation(PathTransition.OrientationType.ORTHOGONAL_TO_TANGENT); pathTransition.setCycleCount(50); pathTransition.setAutoReverse(false); pathTransition.play(); //Creating a Group object Group root = new Group(); root.getChildren().addAll(orbit, planet); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Planet Orbit"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls PlanetOrbit.java java --module-path %PATH_TO_FX% --add-modules javafx.controls PlanetOrbit
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示如下所示的轨道。
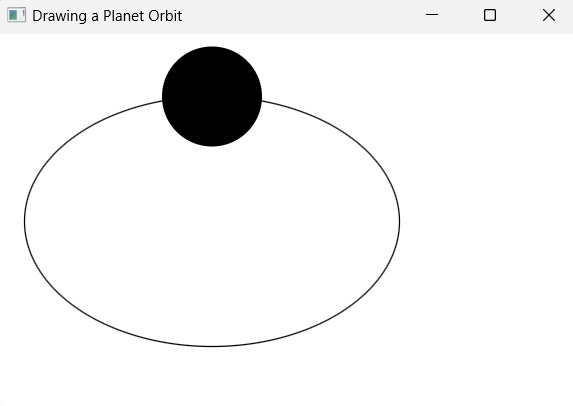