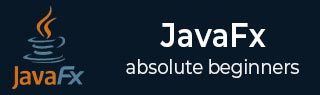
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维形状
- JavaFX - 二维形状
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图片输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 怀旧色调效果
- JavaFX - 阴影效果
- JavaFX - 投影阴影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转转换
- JavaFX - 缩放转换
- JavaFX - 平移转换
- JavaFX - 淡入淡出转换
- JavaFX - 填充转换
- JavaFX - 描边转换
- JavaFX - 顺序转换
- JavaFX - 并行转换
- JavaFX - 暂停转换
- JavaFX - 路径转换
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维形状
- JavaFX - 三维形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 线性渐变图案
除了纯色,您还可以在 JavaFX 中显示颜色渐变。在色彩科学中,颜色渐变定义为颜色根据其位置而变化的过渡。因此,颜色渐变也称为颜色渐变或颜色过渡。
传统上,颜色渐变包含按顺序或线性排列的颜色。但是,在线性渐变图案中,颜色沿单一方向流动。即使要着色的形状不是线性的,例如圆形或椭圆形,颜色仍然会沿一个方向排列。
让我们在本节中学习如何在二维形状上应用线性渐变图案。
应用线性渐变图案
要将线性渐变图案应用于节点,请实例化LinearGradient类并将它的对象传递给setFill(),setStroke()方法。
此类的构造函数接受五个参数,即:
startX, startY - 这些双精度属性表示渐变起点的 x 和 y 坐标。
endX, endY - 这些双精度属性表示渐变终点的 x 和 y 坐标。
cycleMethod - 此参数定义如何填充渐变边界(由起点和终点定义)之外的区域。
proportional - 这是一个布尔变量;将此属性设置为true时,起点和终点位置将设置为比例。
Stops - 此参数定义沿渐变线的颜色停止点。
//Setting the linear gradient Stop[] stops = new Stop[] { new Stop(0, Color.DARKSLATEBLUE), new Stop(1, Color.DARKRED) }; LinearGradient linearGradient = new LinearGradient(0, 0, 1, 0, true, CycleMethod.NO_CYCLE, stops);
示例 1
以下示例演示如何在 JavaFX 中将渐变图案应用于节点。在这里,我们正在创建一个圆形和文本节点,并向它们应用线性渐变图案。
将此代码保存在名为LinearGradientExample.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.paint.CycleMethod; import javafx.scene.paint.LinearGradient; import javafx.scene.paint.Stop; import javafx.stage.Stage; import javafx.scene.shape.Circle; import javafx.scene.text.Font; import javafx.scene.text.Text; public class LinearGradientExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the properties of the circle circle.setCenterX(300.0f); circle.setCenterY(180.0f); circle.setRadius(90.0f); //Drawing a text Text text = new Text("This is a colored circle"); //Setting the font of the text text.setFont(Font.font("Edwardian Script ITC", 55)); //Setting the position of the text text.setX(140); text.setY(50); //Setting the linear gradient Stop[] stops = new Stop[] { new Stop(0, Color.DARKSLATEBLUE), new Stop(1, Color.DARKRED) }; LinearGradient linearGradient = new LinearGradient(0, 0, 1, 0, true, CycleMethod.NO_CYCLE, stops); //Setting the linear gradient to the circle and text circle.setFill(linearGradient); text.setFill(linearGradient); //Creating a Group object Group root = new Group(circle, text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Linear Gradient Example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls LinearGradientExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls LinearGradientExample
输出
执行上述程序后,将生成如下 JavaFX 窗口:
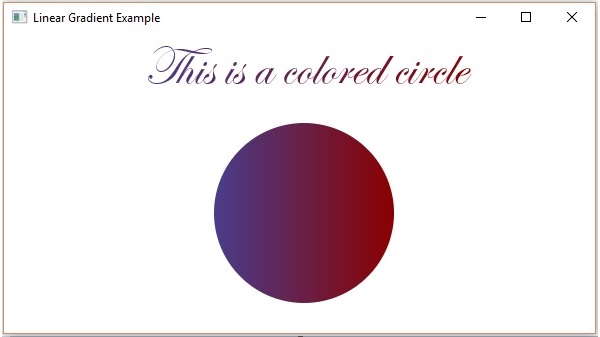
示例 2
除了圆形之外,您还可以将这种类型的渐变应用于其他封闭形状,例如多边形。在这里,我们正在创建一个三角形并使用某种线性渐变图案为其着色。
将此代码保存在名为TriangleLinearGradient.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.paint.CycleMethod; import javafx.scene.paint.LinearGradient; import javafx.scene.paint.Stop; import javafx.stage.Stage; import javafx.scene.shape.Polygon; public class TriangleLinearGradient extends Application { @Override public void start(Stage stage) { Polygon triangle = new Polygon(); triangle.getPoints().addAll(new Double[]{ 100.0, 50.0, 170.0, 150.0, 100.0, 250.0, }); Stop[] stops = new Stop[] { new Stop(0, Color.ORANGE), new Stop(1, Color.YELLOW) }; LinearGradient linearGradient = new LinearGradient(0, 0, 1, 0, true, CycleMethod.NO_CYCLE, stops); triangle.setFill(linearGradient); Group root = new Group(triangle); Scene scene = new Scene(root, 300, 300); stage.setTitle("Linear Gradient Example"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TriangleLinearGradient.java java --module-path %PATH_TO_FX% --add-modules javafx.controls TriangleLinearGradient
输出
执行上述程序后,将生成如下 JavaFX 窗口:
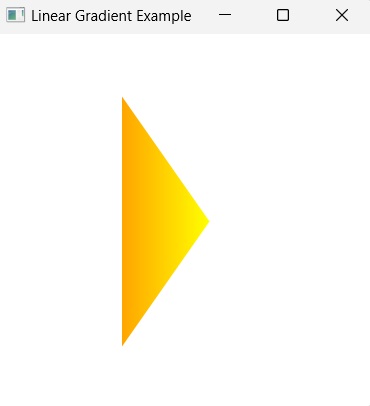