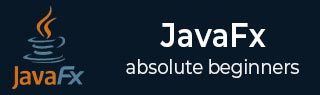
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 光晕效果
- JavaFX - 辉光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 褐色调效果
- JavaFX - 阴影效果
- JavaFX - 投影阴影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建柱状图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠柱状图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 创建圆柱体
圆柱体是一个封闭的立体,它有两个平行的(通常是圆形的)底面,由一个曲面连接而成。为了便于理解,您可以将 3D 圆柱体想象成一堆 2D 圆形,这些圆形堆叠到一定高度;因此,即使它由两个参数描述,它也成为一个三维形状。
圆柱体的参数有 – 其圆形底面的**半径**和圆柱体的**高度**,如下面的图所示:
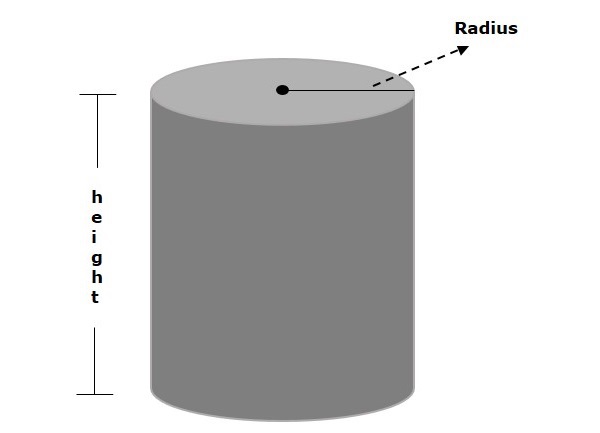
JavaFX 中的圆柱体
在 JavaFX 中,圆柱体由名为**Cylinder**的类表示。此类属于包**javafx.scene.shape**。通过实例化此类,您可以在 JavaFX 中创建一个圆柱体节点。
此类具有 2 个双精度数据类型的属性,即:
**height** - 圆柱体的高度。
**radius** - 圆柱体的半径。
要绘制圆柱体,您需要通过将其传递给此类的构造函数来为这些属性传递值。这可以在实例化 Cylinder 类时按相同的顺序完成;或者,使用它们各自的 setter 方法。
绘制 3D 圆柱体的步骤
要在 JavaFX 中绘制圆柱体(3D),请按照以下步骤操作。
步骤 1:创建类
通过实例化名为 Cylinder 的类(属于包**javafx.scene.shape**)在 JavaFX 中创建 Cylinder 对象。您可以在 start() 方法中实例化此类,如下所示:
public class ClassName extends Application { @Override public void start(Stage primaryStage) throws Exception { //Creating an object of the Cylinder class Cylinder cylinder = new Cylinder(); } }
步骤 2:设置圆柱体的属性
使用其各自的 setter 设置圆柱体的**高度**和**半径**,如下所示。
//Setting the properties of the Cylinder cylinder.setHeight(300.0f); cylinder.setRadius(100.0f);
步骤 3:创建 Group 对象
现在,通过实例化名为**Group**的类(属于包**javafx.scene**)来创建一个 Group 对象。然后,将上一步骤中创建的 Cylinder(节点)对象作为参数传递给 Group 类的构造函数。这应该按顺序完成,以便将其添加到组中,如下所示:
Group root = new Group(cylinder);
步骤 4:启动应用程序
创建 3D 对象后,请按照以下步骤启动 JavaFX 应用程序:
通过将 Group 对象作为参数值传递给其构造函数来实例化名为**Scene**的类。您还可以将应用程序屏幕的尺寸作为可选参数传递给构造函数。
使用**Stage**类的**setTitle()**方法设置舞台的标题。
使用名为**Stage**的类的**setScene()**方法将场景对象添加到舞台。
使用名为**show()**的方法显示场景的内容。
最后,应用程序在 Application 类中的**launch()**方法的帮助下启动。
示例
以下程序显示了如何使用 JavaFX 生成圆柱体。将此代码保存在名为**CylinderExample.java**的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.scene.shape.Cylinder; import javafx.stage.Stage; public class CylinderExample extends Application { @Override public void start(Stage stage) { //Drawing a Cylinder Cylinder cylinder = new Cylinder(); //Setting the properties of the Cylinder cylinder.setHeight(300.0f); cylinder.setRadius(100.0f); //Creating a Group object Group root = new Group(cylinder); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a cylinder"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls CylinderExample
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的圆柱体。
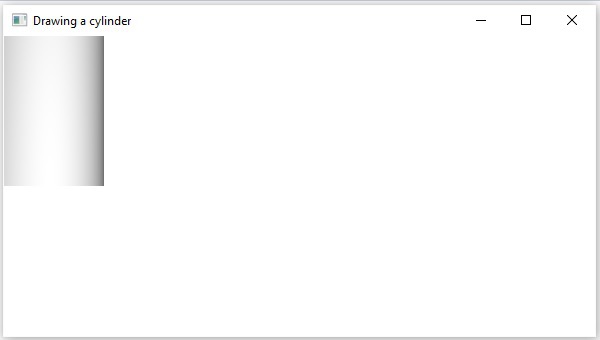
示例
您还可以对 3D 形状应用变换。在此示例中,我们尝试对 3D 圆柱体应用平移变换,并将其重新定位到应用程序上。将此代码保存在名为**TranslateCylinderExample.java**的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.scene.shape.Cylinder; import javafx.scene.paint.Color; import javafx.scene.transform.Translate; import javafx.stage.Stage; public class TranslateCylinderExample extends Application { @Override public void start(Stage stage) { //Drawing a Cylinder Cylinder cylinder = new Cylinder(); //Setting the properties of the Cylinder cylinder.setHeight(150.0f); cylinder.setRadius(100.0f); Translate translate = new Translate(); translate.setX(200); translate.setY(150); translate.setZ(25); cylinder.getTransforms().addAll(translate); //Creating a Group object Group root = new Group(cylinder); //Creating a scene object Scene scene = new Scene(root, 400, 300); scene.setFill(Color.web("#81c483")); //Setting title to the Stage stage.setTitle("Drawing a cylinder"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TranslateCylinderExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls TranslateCylinderExample
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的圆柱体。
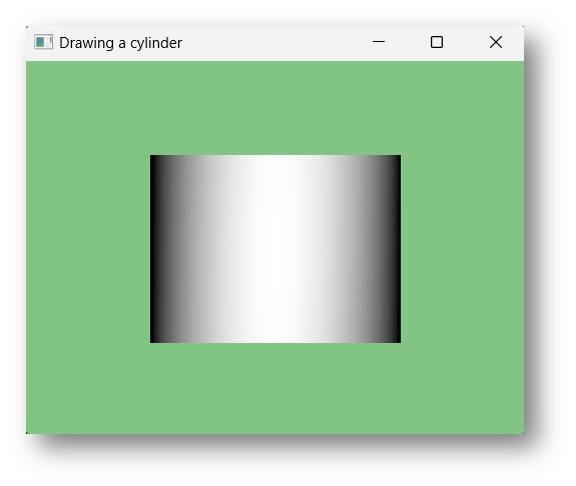