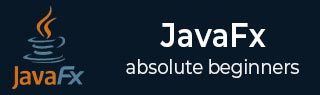
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- 使用 Netbeans 安装 JavaFX
- 使用 Eclipse 安装 JavaFX
- 使用 Visual Studio Code 安装 JavaFX
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 2D 对象属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线帽属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 特效
- JavaFX - 特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图片输入特效
- JavaFX - 混合特效
- JavaFX - 辉光特效
- JavaFX - 泛光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 棕褐色调特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内阴影特效
- JavaFX - 光照特效
- JavaFX - 远光源特效
- JavaFX - 聚光灯特效
- JavaFX - 点光源特效
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转转换
- JavaFX - 缩放转换
- JavaFX - 平移转换
- JavaFX - 淡入淡出转换
- JavaFX - 填充转换
- JavaFX - 描边转换
- JavaFX - 顺序转换
- JavaFX - 并行转换
- JavaFX - 暂停转换
- JavaFX - 路径转换
- JavaFX 图片
- JavaFX - 图片
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 超链接
超链接是允许用户在点击时导航到网页或执行操作的 UI 组件。它们类似于按钮,但外观和行为不同。例如,我们可以在显示窗口顶部的地址栏中找到任何网页的超链接,如下面的图所示:
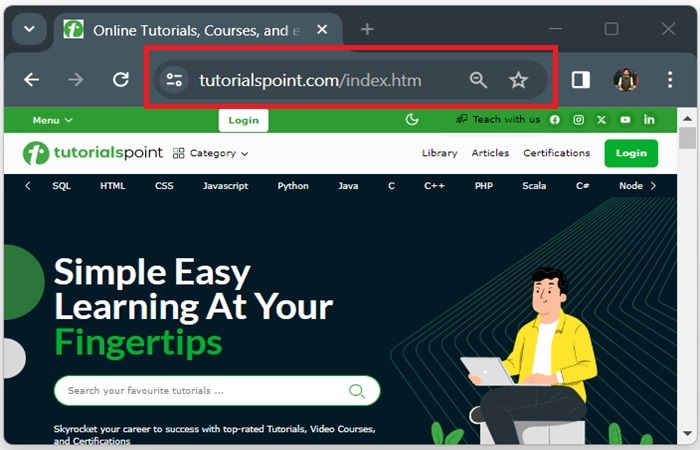
在 JavaFX 中创建超链接
在 JavaFX 中,超链接由名为Hyperlink的类表示。此类属于包javafx.scene.control。通过实例化此类,我们可以在 JavaFX 中创建超链接。Hyperlink 类的构造函数如下所示:
Hyperlink() - 这是默认构造函数,它创建一个没有标签文本的超链接。
Hyperlink(String str) - 它创建一个具有指定标签文本的新超链接。
Hyperlink(String str, Node icons) - 它创建一个具有指定文本和图形标签的新超链接。
在 JavaFX 中,超链接以文本和图像两种形式创建。创建超链接时,我们的第一步是使用上述任何构造函数实例化 Hyperlink 类。然后,指定用户点击链接时应执行的操作。为此,我们需要向其setOnAction()方法添加一个EventHandler。此外,此 EventHandler 将调用指定的方法,这有助于导航。
通过更改setOnAction()方法的实现,我们可以为本地资源以及远程服务器上可用的资源创建超链接。
示例
在下面的 JavaFX 程序中,我们将创建一个文本超链接。将此代码保存在名为HyperlinkExample.java的文件中。
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Hyperlink; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; public class HyperlinkExample extends Application { @Override public void start(Stage stage) { // Creating a Label Label labelText = new Label("On clicking the below text, it will redirect us to tutorialspoint"); // Create a hyperlink with text Hyperlink textLink = new Hyperlink("Visit TutorialsPoint"); // Set the action of the hyperlink textLink.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { // Open the web page in the default browser getHostServices().showDocument("https://tutorialspoint.com/index.htm"); } }); // Create a scene with the hyperlink VBox root = new VBox(labelText, textLink); root.setAlignment(Pos.CENTER); Scene scene = new Scene(root, 400, 300); // Set the title and scene of the stage stage.setTitle("Hyperlink in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
要从命令提示符编译和执行保存的 Java 文件,请使用以下命令:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkExample
输出
执行上述程序将生成以下输出。
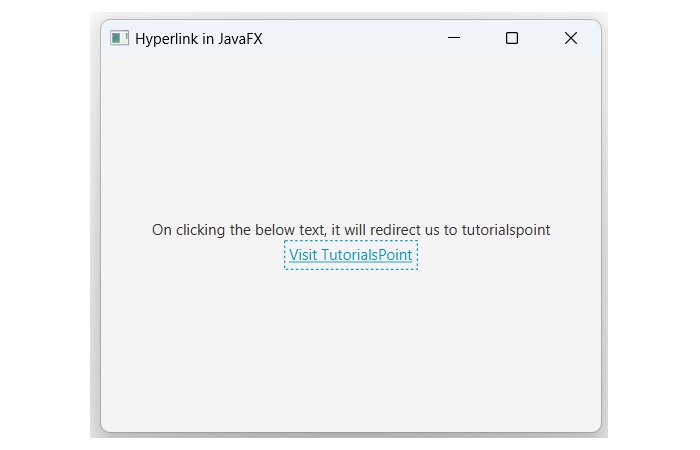
创建图像超链接
要创建带有图像的超链接,请实例化ImageView类并将它的对象作为参数值传递给Hyperlink类的构造函数,如下一个示例所示。将此代码保存在名为HyperlinkImage.java的文件中。
import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.scene.Scene; import javafx.scene.control.Hyperlink; import javafx.scene.control.Label; import javafx.scene.control.ContentDisplay; import javafx.scene.layout.VBox; import javafx.stage.Stage; import javafx.geometry.Pos; import javafx.scene.image.Image; import javafx.scene.image.ImageView; public class HyperlinkImage extends Application { @Override public void start(Stage stage) { // Creating a Label Label labelText = new Label("On clicking the below image, it will redirect us to tutorialspoint"); // Create a hyperlink with image Image image = new Image("tutorials_point.jpg"); ImageView imageV = new ImageView(image); imageV.setFitWidth(150); imageV.setFitHeight(150); Hyperlink imageLink = new Hyperlink("visit: ", imageV); // Set the content display position of the image imageLink.setContentDisplay(ContentDisplay.RIGHT); // Set the action of the hyperlink imageLink.setOnAction(new EventHandler() { @Override public void handle(ActionEvent event) { // Open the web page in the default browser getHostServices().showDocument("https://tutorialspoint.com/index.htm"); } }); // Create a scene with the hyperlink VBox root = new VBox(labelText, imageLink); root.setAlignment(Pos.CENTER); Scene scene = new Scene(root, 400, 300); // Set the title and scene of the stage stage.setTitle("Hyperlink in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件:
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkImage.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HyperlinkImage
输出
执行上述代码将生成以下输出。
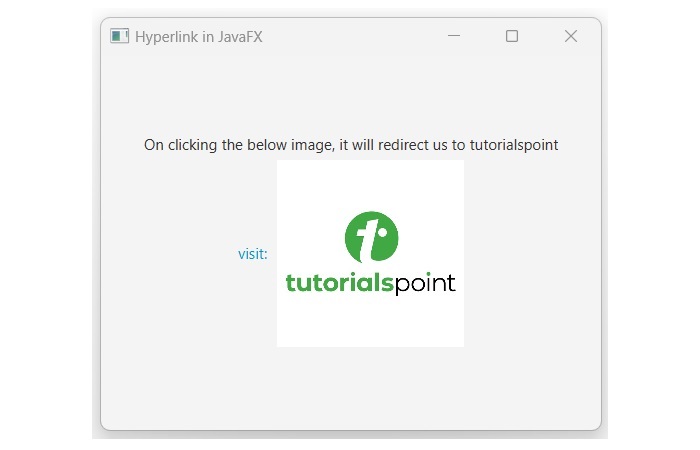