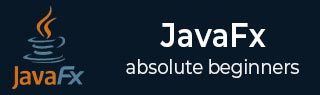
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 2D 形状
- JavaFX - 2D 形状
- JavaFX - 绘制线条
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制圆弧
- JavaFX - 绘制 SVGPath
- JavaFX 2D 对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图像输入效果
- JavaFX - 混合效果
- JavaFX - 辉光效果
- JavaFX - 泛光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 褐色调效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 照明效果
- JavaFX - Light.Distant 效果
- JavaFX - Light.Spot 效果
- JavaFX - Point.Spot 效果
- JavaFX - 位移映射
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转过渡
- JavaFX - 缩放过渡
- JavaFX - 平移过渡
- JavaFX - 淡入淡出过渡
- JavaFX - 填充过渡
- JavaFX - 描边过渡
- JavaFX - 顺序过渡
- JavaFX - 并行过渡
- JavaFX - 暂停过渡
- JavaFX - 路径过渡
- JavaFX 图像
- JavaFX - 图像
- JavaFX 3D 形状
- JavaFX - 3D 形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- 3D 对象的属性
- JavaFX - 剔除面属性
- JavaFX - 绘制模式属性
- JavaFX - 材质属性
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建柱状图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠柱状图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 中的媒体
- JavaFX - 处理媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - HBox 布局
JavaFX 中的 HBox 布局
HBox,也称为水平盒,是一个布局面板,它将 JavaFX 应用程序的所有节点排列在单个水平行中。HBox 布局面板由名为HBox的类表示,该类属于javafx.scene.layout包。实例化此类以创建 HBox 布局。HBox 类的构造函数如下:
HBox() - 这是默认构造函数,它使用 0 间距构造一个 HBox 布局。
HBox(double spacingVal) - 它使用节点之间指定的间距构造一个新的 HBox 布局。
HBox(double spacingVal, Node nodes) - HBox 类的此参数化构造函数接受子节点以及它们之间的间距,并使用指定的组件创建一个新的 HBox 布局。
HBox(Node nodes) - 它使用指定的子节点和 0 间距创建 HBox 布局。
在下面的快照中,我们可以看到红色框内的 UI 组件放置在水平布局中:
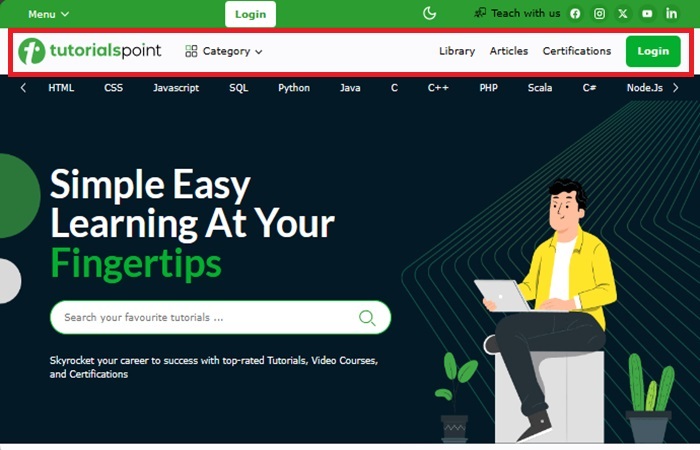
HBox 类具有以下属性:
alignment - 此属性表示节点在 HBox 边界内的对齐方式。我们可以使用 setter 方法setAlignment()为该属性设置值。
fillHeight - 此属性为布尔类型,将其设置为 true 时,HBox 中的可调整大小的节点将调整为 HBox 的高度。我们可以使用 setter 方法setFillHeight()为该属性设置值。
spacing - 此属性为双精度类型,它表示 HBox 子节点之间的空间。我们可以使用 setter 方法setSpacing()为该属性设置值。
padding - 它表示 HBox 的边框与其子节点之间的空间。我们可以使用 setter 方法setPadding()为该属性设置值,该方法接受Insets构造函数作为参数值。
除了这些之外,HBox类还提供了一些方法,如下所示:
序号 | 方法和描述 |
---|---|
1 | setHgrow()
设置子节点在 HBox 中包含时的水平增长优先级。此方法接受一个节点和一个优先级值。可能的优先级值可以是Policy.ALWAYS、Policy.SOMETIMES和Policy.NEVER。 |
2 | setMargin()
使用此方法,您可以为 HBox 设置边距。此方法接受一个节点和一个 Insets 类对象(矩形区域 4 边的内部偏移量集)。 |
示例
以下程序是 HBox 布局的示例。在这里,我们插入一个文本字段和两个名为播放和停止的按钮。将此 JavaFX 代码保存在名为HBoxExample.java的文件中。
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.TextField; import javafx.stage.Stage; import javafx.scene.layout.HBox; public class HBoxExample extends Application { @Override public void start(Stage stage) { //creating a text field TextField textField = new TextField(); //Creating the play button Button playButton = new Button("Play"); //Creating the stop button Button stopButton = new Button("stop"); //Instantiating the HBox class HBox hbox = new HBox(); //Setting the space between the nodes of a HBox pane hbox.setSpacing(10); //Adding all the nodes to the HBox hbox.getChildren().addAll(textField, playButton, stopButton); //Setting the margin to the nodes hbox.setMargin(textField, new Insets(20, 20, 20, 20)); hbox.setMargin(playButton, new Insets(20, 20, 20, 20)); hbox.setMargin(stopButton, new Insets(20, 20, 20, 20)); //Creating a scene object Scene scene = new Scene(hbox, 400, 300); //Setting title to the Stage stage.setTitle("Hbox Example in JavaFX"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HBoxExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HBoxExample
输出
执行上述程序后,将生成一个 JavaFX 窗口,如下所示。
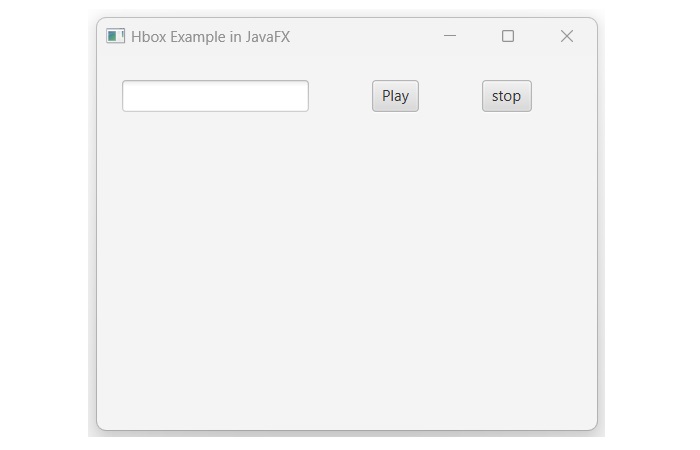
示例
在以下示例中,我们将使用 HBox 类的参数化构造函数来创建水平布局。将此 JavaFX 代码保存在名为HBoxExample.java的文件中。
import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.TextField; import javafx.stage.Stage; import javafx.scene.layout.HBox; public class HBoxExample extends Application { @Override public void start(Stage stage) { //creating a text field TextField textField = new TextField(); //Creating the play button Button playButton = new Button("Play"); //Creating the stop button Button stopButton = new Button("stop"); //Instantiating the HBox class HBox box = new HBox(10, textField, playButton, stopButton); box.setAlignment( Pos.CENTER); //Creating a scene object Scene scene = new Scene(box, 400, 300); //Setting title to the Stage stage.setTitle("Hbox Example in JavaFX"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls HBoxExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls HBoxExample
输出
执行上述程序后,将生成以下输出。
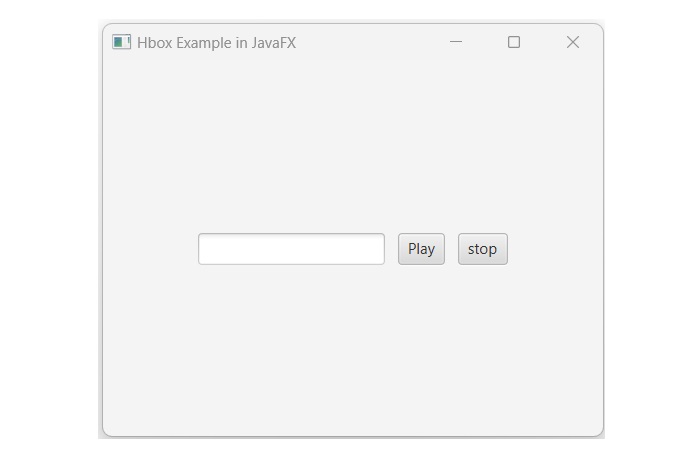