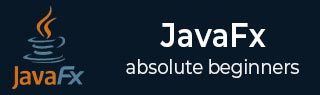
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维形状
- JavaFX - 二维形状
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧形
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 特效
- JavaFX - 特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图片输入特效
- JavaFX - 混合特效
- JavaFX - 光晕特效
- JavaFX - 辉光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 棕褐色调特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内阴影特效
- JavaFX - 照明特效
- JavaFX - 远光源特效
- JavaFX - 聚光灯特效
- JavaFX - 点光源特效
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维形状
- JavaFX - 三维形状
- JavaFX - 创建立方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 描边类型属性
在几何学中,二维 (2D) 形状通常是指只有两个度量维度的结构,通常是长度和宽度,并位于 XY 平面上。这些结构可以是开放或封闭图形。
开放图形包括三次曲线、四边曲线等曲线,而封闭图形包括所有类型的多边形、圆形等。
在 JavaFX 中,可以通过导入 **javafx.scene.shape** 包在应用程序上绘制这些 2D 形状。但是,为了提高形状的质量,可以对其执行各种属性和操作。
在本章中,我们将详细了解描边类型属性。
描边类型属性
二维形状的描边类型属性用于指定二维形状必须具有的边界线的类型。在 JavaFX 中,此属性使用 StrokeType 类设置。您可以使用以下方法设置描边的类型:**setStrokeType()** −
Path.setStrokeType(StrokeType.stroke_type);
形状的描边类型可以是:
**内部 (Inside)** - 边界线将绘制在形状边缘(轮廓)内部 (StrokeType.INSIDE)。
**外部 (Outside)** - 边界线将绘制在形状边缘(轮廓)外部 (StrokeType.OUTSIDE)。
**居中 (Centered)** - 边界线将以这样的方式绘制:形状的边缘(轮廓)正好穿过线的中心 (StrokeType.CENTERED)。
默认情况下,形状的描边类型为居中。以下是具有不同描边类型的三角形的图:
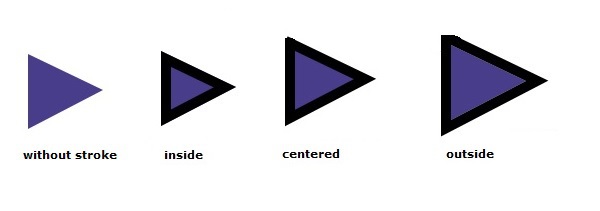
示例
让我们来看一个演示在二维形状上使用 StrokeType 属性的示例。将此文件保存为 **StrokeTypeExample.java**。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Polygon; import javafx.scene.shape.StrokeType; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeTypeExample extends Application { @Override public void start(Stage stage) { //Creating a Triangle Polygon triangle = new Polygon(); //Adding coordinates to the polygon triangle.getPoints().addAll(new Double[]{ 100.0, 50.0, 170.0, 150.0, 100.0, 250.0, }); triangle.setFill(Color.BLUE); triangle.setStroke(Color.BLACK); triangle.setStrokeWidth(7.0); triangle.setStrokeType(StrokeType.CENTERED); //Creating a Group object Group root = new Group(triangle); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Triangle"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeExample
输出
执行后,上述程序将生成一个 JavaFX 窗口,其中显示一个具有居中描边类型的三角形,如下所示。
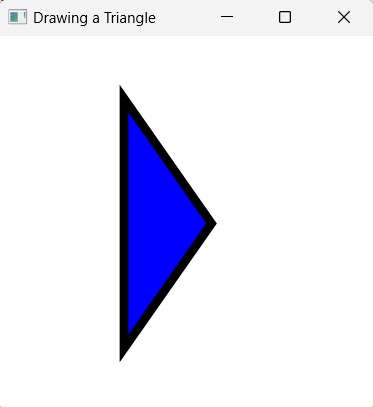
示例
让我们来看一个演示在二维形状上使用 INSIDE StrokeType 属性的示例。为了正确显示 INSIDE 和 CENTERED 描边类型之间的区别,我们在此处使用了更大的宽度。将此文件保存为 **StrokeTypeEllipse.java**。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Ellipse; import javafx.scene.shape.StrokeType; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeTypeEllipse extends Application { @Override public void start(Stage stage) { //Drawing an ellipse Ellipse ellipse = new Ellipse(); //Setting the properties of the ellipse ellipse.setCenterX(150.0f); ellipse.setCenterY(100.0f); ellipse.setRadiusX(100.0f); ellipse.setRadiusY(50.0f); ellipse.setFill(Color.ORANGE); ellipse.setStroke(Color.BLACK); ellipse.setStrokeWidth(20.0); ellipse.setStrokeType(StrokeType.INSIDE); //Creating a Group object Group root = new Group(ellipse); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Ellipse"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeEllipse.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeTypeEllipse
输出
执行后,上述程序将生成一个 JavaFX 窗口,其中显示一个具有居中描边类型的三角形,如下所示。
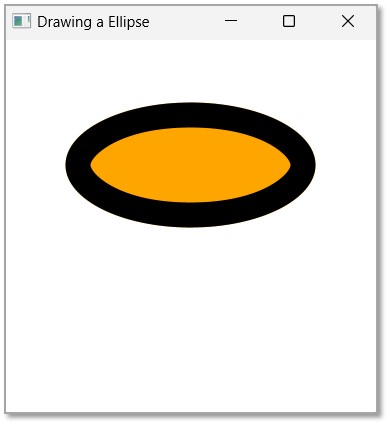