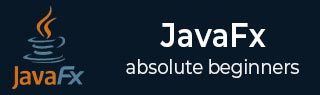
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维图形
- JavaFX - 二维图形
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 特效
- JavaFX - 特效
- JavaFX - 颜色调整特效
- JavaFX - 颜色输入特效
- JavaFX - 图片输入特效
- JavaFX - 混合特效
- JavaFX - 辉光特效
- JavaFX - 泛光特效
- JavaFX - 方框模糊特效
- JavaFX - 高斯模糊特效
- JavaFX - 运动模糊特效
- JavaFX - 反射特效
- JavaFX - 棕褐色调特效
- JavaFX - 阴影特效
- JavaFX - 投影阴影特效
- JavaFX - 内阴影特效
- JavaFX - 光照特效
- JavaFX - Light.Distant 特效
- JavaFX - Light.Spot 特效
- JavaFX - Point.Spot 特效
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维图形
- JavaFX - 三维图形
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理程序
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTMLEditor
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报框
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 创建球体
球体是在三维空间中一个完美的几何圆形物体,是完全圆形球的表面。
球体定义为三维空间中所有与给定点距离均为 r 的点的集合。此距离r是球体的半径,给定点是球体的中心。
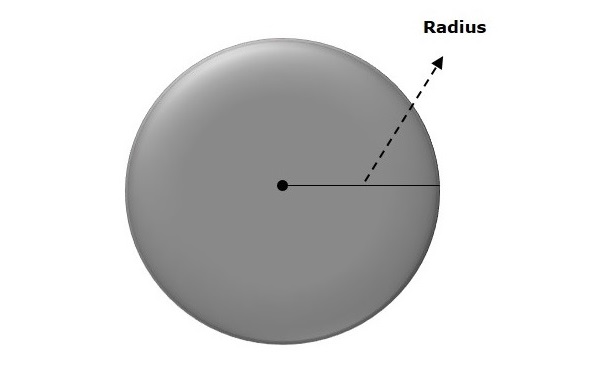
JavaFX 中的球体
在 JavaFX 中,球体由名为Sphere的类表示。此类属于包javafx.scene.shape。通过实例化此类,可以在 JavaFX 中创建球体节点。
此类具有名为radius的双精度数据类型属性。它表示球体的半径。要绘制球体,需要通过在实例化时将其传递给此类的构造函数来为此属性设置值;或者,使用名为setRadius()的 setter 方法。
绘制 3D 球体的步骤
按照以下步骤在 JavaFX 中绘制球体 (3D)。
步骤 1:创建球体
通过实例化名为Sphere的类(属于包javafx.scene.shape)在 JavaFX 中创建球体。可以在 start() 方法中实例化此类,如下所示。
public class ClassName extends Application { @Override public void start(Stage primaryStage) throws Exception { //Creating an object of the class Sphere Sphere sphere = new Sphere(); } }
步骤 2:设置球体的属性
使用名为setRadius()的方法设置球体的半径,如下所示。
//Setting the radius of the Sphere sphere.setRadius(300.0);
步骤 3:创建 Group 对象
通过将球体对象作为参数传递给其构造函数来实例化 Group 类,如下所示:
Group root = new Group(sphere);
步骤 4:启动应用程序
创建 3D 对象后,请按照以下步骤启动 JavaFX 应用程序:
通过将 Group 对象作为参数值传递给其构造函数来实例化名为Scene的类。也可以将应用程序屏幕的尺寸作为可选参数传递给构造函数。
使用Stage类的setTitle()方法设置舞台的标题。
使用名为Stage的类的setScene()方法将场景对象添加到舞台。
使用名为show()的方法显示场景的内容。
最后,应用程序在 Application 类中使用launch()方法启动。
示例
以下程序演示如何使用 JavaFX 生成球体。将此代码保存在名为SphereExample.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Sphere; public class SphereExample extends Application { @Override public void start(Stage stage) { //Drawing a Sphere Sphere sphere = new Sphere(); //Setting the properties of the Sphere sphere.setRadius(50.0); sphere.setTranslateX(200); sphere.setTranslateY(150); //Creating a Group object Group root = new Group(sphere); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Sphere - draw fill"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls SphereExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls SphereExample
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的球体。
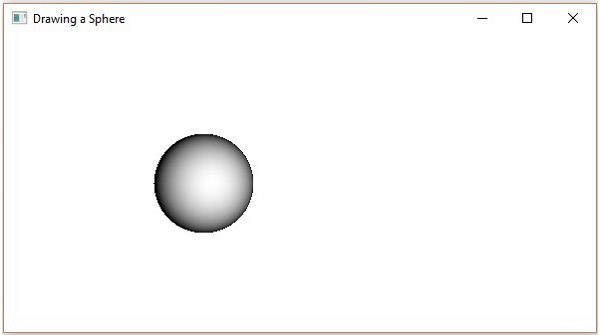
示例
在下面的程序中,我们通过为 JavaFX 应用程序的场景着色来应用一些 JavaFX CSS。将此代码保存在名为CSSSphereExample.java的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.paint.Color; import javafx.scene.shape.Sphere; public class CSSSphereExample extends Application { @Override public void start(Stage stage) { //Drawing a Sphere Sphere sphere = new Sphere(); //Setting the properties of the Sphere sphere.setRadius(50.0); sphere.setTranslateX(100); sphere.setTranslateY(150); //Creating a Group object Group root = new Group(sphere); //Creating a scene object Scene scene = new Scene(root, 300, 300); scene.setFill(Color.ORANGE); //Setting title to the Stage stage.setTitle("Drawing a Sphere"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CSSSphereExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls CSSSphereExample
输出
执行上述程序后,会生成一个 JavaFX 窗口,其中显示如下所示的球体。
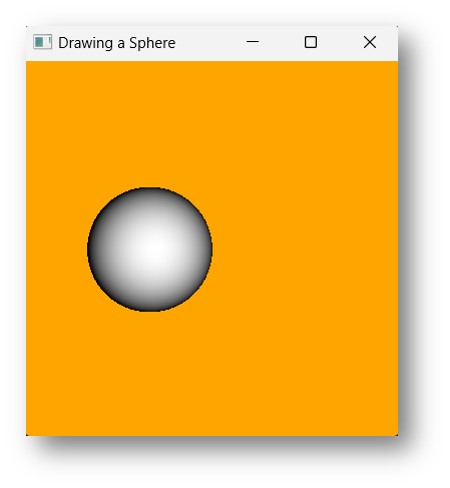