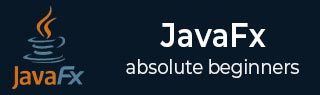
- JavaFX 教程
- JavaFX - 首页
- JavaFX - 概述
- JavaFX 安装和架构
- JavaFX - 环境
- JavaFX - 使用 Netbeans 安装
- JavaFX - 使用 Eclipse 安装
- JavaFX - 使用 Visual Studio Code 安装
- JavaFX - 架构
- JavaFX - 应用程序
- JavaFX 二维形状
- JavaFX - 二维形状
- JavaFX - 绘制直线
- JavaFX - 绘制矩形
- JavaFX - 绘制圆角矩形
- JavaFX - 绘制圆形
- JavaFX - 绘制椭圆
- JavaFX - 绘制多边形
- JavaFX - 绘制折线
- JavaFX - 绘制三次贝塞尔曲线
- JavaFX - 绘制二次贝塞尔曲线
- JavaFX - 绘制弧线
- JavaFX - 绘制 SVG 路径
- JavaFX 二维对象的属性
- JavaFX - 描边类型属性
- JavaFX - 描边宽度属性
- JavaFX - 描边填充属性
- JavaFX - 描边属性
- JavaFX - 描边线连接属性
- JavaFX - 描边斜接限制属性
- JavaFX - 描边线端点属性
- JavaFX - 平滑属性
- JavaFX 路径对象
- JavaFX - 路径对象
- JavaFX - LineTo 路径对象
- JavaFX - HLineTo 路径对象
- JavaFX - VLineTo 路径对象
- JavaFX - QuadCurveTo 路径对象
- JavaFX - CubicCurveTo 路径对象
- JavaFX - ArcTo 路径对象
- JavaFX 颜色和纹理
- JavaFX - 颜色
- JavaFX - 线性渐变图案
- JavaFX - 径向渐变图案
- JavaFX 文本
- JavaFX - 文本
- JavaFX 效果
- JavaFX - 效果
- JavaFX - 颜色调整效果
- JavaFX - 颜色输入效果
- JavaFX - 图片输入效果
- JavaFX - 混合效果
- JavaFX - 辉光效果
- JavaFX - 泛光效果
- JavaFX - 方框模糊效果
- JavaFX - 高斯模糊效果
- JavaFX - 运动模糊效果
- JavaFX - 反射效果
- JavaFX - 棕褐色调效果
- JavaFX - 阴影效果
- JavaFX - 投影效果
- JavaFX - 内阴影效果
- JavaFX - 光照效果
- JavaFX - 远光源效果
- JavaFX - 聚光灯效果
- JavaFX - 点光源效果
- JavaFX - 位移贴图
- JavaFX - 透视变换
- JavaFX 动画
- JavaFX - 动画
- JavaFX - 旋转动画
- JavaFX - 缩放动画
- JavaFX - 平移动画
- JavaFX - 淡入淡出动画
- JavaFX - 填充动画
- JavaFX - 描边动画
- JavaFX - 顺序动画
- JavaFX - 并行动画
- JavaFX - 暂停动画
- JavaFX - 路径动画
- JavaFX 图片
- JavaFX - 图片
- JavaFX 三维形状
- JavaFX - 三维形状
- JavaFX - 创建长方体
- JavaFX - 创建圆柱体
- JavaFX - 创建球体
- JavaFX 事件处理
- JavaFX - 事件处理
- JavaFX - 使用便捷方法
- JavaFX - 事件过滤器
- JavaFX - 事件处理器
- JavaFX UI 控件
- JavaFX - UI 控件
- JavaFX - 列表视图
- JavaFX - 手风琴
- JavaFX - 按钮栏
- JavaFX - 选择框
- JavaFX - HTML 编辑器
- JavaFX - 菜单栏
- JavaFX - 分页
- JavaFX - 进度指示器
- JavaFX - 滚动窗格
- JavaFX - 分隔符
- JavaFX - 滑块
- JavaFX - 微调器
- JavaFX - 分割窗格
- JavaFX - 表格视图
- JavaFX - 标签页窗格
- JavaFX - 工具栏
- JavaFX - 树视图
- JavaFX - 标签
- JavaFX - 复选框
- JavaFX - 单选按钮
- JavaFX - 文本字段
- JavaFX - 密码字段
- JavaFX - 文件选择器
- JavaFX - 超链接
- JavaFX - 工具提示
- JavaFX - 警报
- JavaFX - 日期选择器
- JavaFX - 文本区域
- JavaFX 图表
- JavaFX - 图表
- JavaFX - 创建饼图
- JavaFX - 创建折线图
- JavaFX - 创建面积图
- JavaFX - 创建条形图
- JavaFX - 创建气泡图
- JavaFX - 创建散点图
- JavaFX - 创建堆叠面积图
- JavaFX - 创建堆叠条形图
- JavaFX 布局面板
- JavaFX - 布局面板
- JavaFX - HBox 布局
- JavaFX - VBox 布局
- JavaFX - BorderPane 布局
- JavaFX - StackPane 布局
- JavaFX - TextFlow 布局
- JavaFX - AnchorPane 布局
- JavaFX - TilePane 布局
- JavaFX - GridPane 布局
- JavaFX - FlowPane 布局
- JavaFX CSS
- JavaFX - CSS
- JavaFX 多媒体
- JavaFX - 处理多媒体
- JavaFX - 播放视频
- JavaFX 有用资源
- JavaFX - 快速指南
- JavaFX - 有用资源
- JavaFX - 讨论
JavaFX - 绘制弧线
在简单的几何学中,弧线定义为椭圆或圆周的一部分。或者,简单地说,它是一条连接两个端点的曲线。弧线在圆心处形成小于或等于 360 度的角。它由以下属性描述:
长度 - 沿弧线的距离。
角度 - 曲线在圆心处形成的角度。
radiusX - 当前弧线所属的完整椭圆的宽度。
radiusY - 当前弧线所属的完整椭圆的高度。
如果 radiusX 和 radiusY 相同,则该弧线是圆周的一部分。
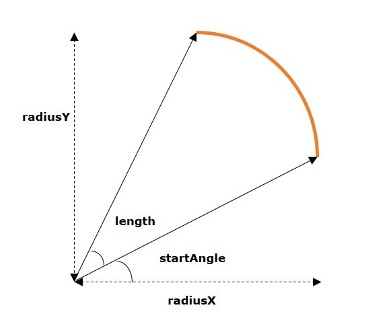
JavaFX 中的弧线
在 JavaFX 中,弧线由名为 Arc 的类表示。此类属于包 javafx.scene.shape。
通过实例化此类,您可以在 JavaFX 中创建弧线节点。
此类具有几个双精度数据类型的属性,即:
centerX - 弧线中心的 x 坐标。
centerY - 弧线中心的 y 坐标。
radiusX - 当前弧线所属的完整椭圆的宽度。
radiusY - 当前弧线所属的完整椭圆的高度。
startAngle - 弧线的起始角度(以度为单位)。
length - 弧线的角度范围(以度为单位)。
要绘制弧线,您需要向这些属性传递值,可以通过在实例化时按相同的顺序将它们传递给此类的构造函数,或者使用它们各自的 setter 方法来实现。
弧线的类型
在 JavaFX 中,您可以绘制三种类型的弧线:
打开 - 完全未闭合的弧线称为开放弧线。
弦 - 弦是一种用直线闭合的弧线。
圆形 - 圆形弧线是通过连接起始点和结束点到椭圆中心来闭合的弧线。
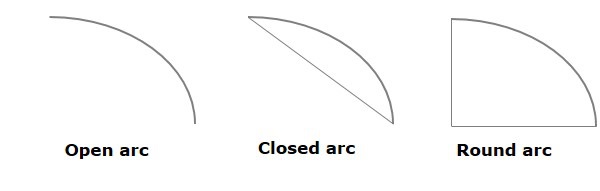
您可以使用 setType() 方法通过传递以下属性之一来设置弧线的类型:ArcType.OPEN, ArcType.CHORD, ArcType.ROUND。
绘制弧线的步骤
要在 JavaFX 中绘制弧线,请按照以下步骤操作。
步骤 1:创建弧线
您可以通过实例化名为 Arc 的类(属于包 javafx.scene.shape)来在 JavaFX 中创建弧线。您可以像下面这样在 Application 类的 start() 方法内实例化此类。
public class ClassName extends Application { public void start(Stage primaryStage) throws Exception { //Creating an object of the class Arc Arc arc = new Arc(); } }
步骤 2:设置弧线的属性
指定椭圆(此弧线所属的椭圆)中心的 x、y 坐标。这些坐标包括 – radiusX、radiusY、起始角度和弧线的长度,使用它们各自的 setter 方法,如下面的代码块所示。
//Setting the properties of the arc arc.setCenterX(300.0f); arc.setCenterY(150.0f); arc.setRadiusX(90.0f); arc.setRadiusY(90.0f); arc.setStartAngle(40.0f); arc.setLength(239.0f);
步骤 3:设置弧线的类型
您还可以使用 setType() 方法设置弧线的类型(圆形、弦、打开),如下面的代码块所示。
//Setting the type of the arc arc.setType(ArcType.ROUND);
步骤 4:将弧线对象添加到 Group
在 start() 方法中,通过将上一步中创建的弧线对象作为参数值传递给其构造函数来实例化 Group 类:
Group root = new Group(arc);
步骤 5:启动应用程序
创建二维对象后,请按照以下步骤正确启动应用程序:
首先,通过将 Group 对象作为参数值传递给其构造函数来实例化名为 Scene 的类。对于此构造函数,您还可以将应用程序屏幕的尺寸作为可选参数传递。
然后,使用 Stage 类的 setTitle() 方法设置舞台的标题。
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到舞台。
使用名为 show() 的方法显示场景的内容。
最后,在 launch() 方法的帮助下启动应用程序。
示例
以下程序生成一个弧线。将此代码保存在名为 ArcExample.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Arc; import javafx.scene.shape.ArcType; public class ArcExample extends Application { public void start(Stage stage) { //Drawing an arc Arc arc = new Arc(); //Setting the properties of the arc arc.setCenterX(300.0f); arc.setCenterY(150.0f); arc.setRadiusX(90.0f); arc.setRadiusY(90.0f); arc.setStartAngle(40.0f); arc.setLength(239.0f); //Setting the type of the arc arc.setType(ArcType.ROUND); //Creating a Group object Group root = new Group(arc); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing an Arc"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ArcExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ArcExample
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示如下屏幕截图所示的弧线。
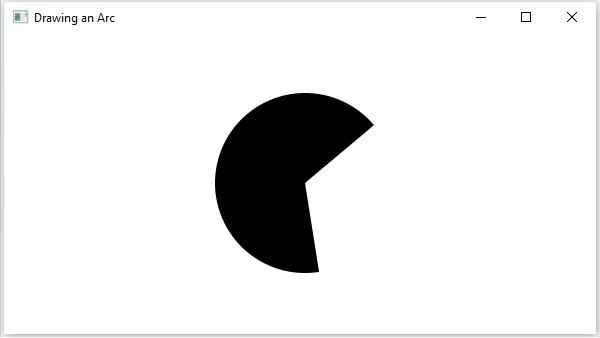
示例
让我们尝试在下面的示例中绘制另一个“打开”类型的弧线。将此代码保存在名为 ArcOpen.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Arc; import javafx.scene.shape.ArcType; public class ArcOpen extends Application { public void start(Stage stage) { //Drawing an arc Arc arc = new Arc(); //Setting the properties of the arc arc.setCenterX(300.0f); arc.setCenterY(150.0f); arc.setRadiusX(90.0f); arc.setRadiusY(90.0f); arc.setStartAngle(40.0f); arc.setLength(239.0f); //Setting the type of the arc arc.setType(ArcType.OPEN); //Creating a Group object Group root = new Group(arc); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing an Open Arc"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ArcOpen.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ArcOpen
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示如下屏幕截图所示的弧线。
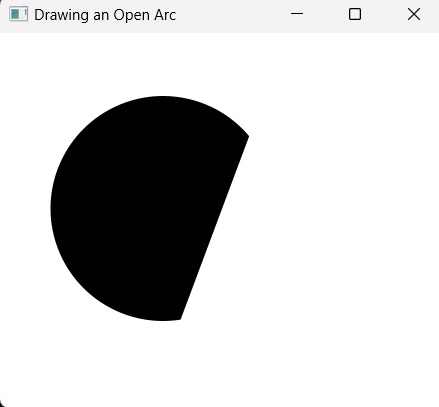
示例
现在,我们尝试在下面的示例中绘制另一个“弦”类型的弧线。将此代码保存在名为 ArcChord.java 的文件中。
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Arc; import javafx.scene.shape.ArcType; public class ArcChord extends Application { public void start(Stage stage) { //Drawing an arc Arc arc = new Arc(); //Setting the properties of the arc arc.setCenterX(300.0f); arc.setCenterY(150.0f); arc.setRadiusX(90.0f); arc.setRadiusY(90.0f); arc.setStartAngle(40.0f); arc.setLength(239.0f); //Setting the type of the arc arc.setType(ArcType.CHORD); //Creating a Group object Group root = new Group(arc); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Chord Arc"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls ArcChord.java java --module-path %PATH_TO_FX% --add-modules javafx.controls ArcChord
输出
执行上述程序后,将生成一个 JavaFX 窗口,其中显示如下屏幕截图所示的弧线。
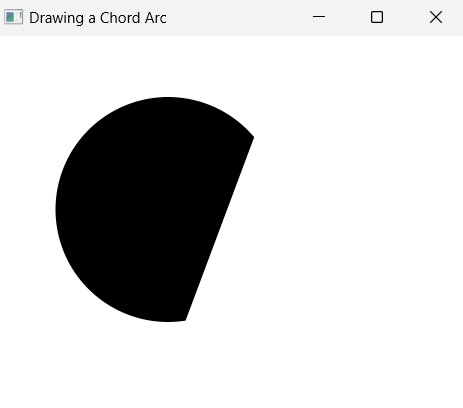