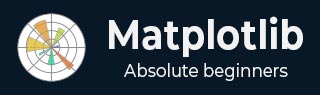
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 色标
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性与对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 艺术家
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化程序
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 固定艺术家
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 飘带框
- Matplotlib - 填充螺旋
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建徽标
- Matplotlib - 多页 PDF
- Matplotlib - 多处理
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - 带 EEG 的 MRI
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 观景镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 计时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 带注释的光标
什么是带注释的光标?
带注释的光标是数据可视化中的一项功能,它将光标跟踪与其他信息、注释或工具提示结合起来,这些信息在光标在绘图上移动时会显示在光标位置旁边。它通过提供上下文信息来帮助用户深入了解特定数据点或感兴趣区域,从而获得见解。
在 Matplotlib 或其他绘图库的视图中,带注释的光标通常涉及在绘图上光标的位置显示注释、工具提示或补充信息。此信息可能包括数据值、标签或与绘制数据点相关的任何相关详细信息。
带注释的光标通过提供有关特定数据坐标或区域的即时和上下文信息来增强绘图的交互式探索,从而帮助进行数据分析和解释。
实现带注释的光标涉及使用光标跟踪事件(例如 Matplotlib 中的'motion_notify_event')来根据光标的位置动态更新注释或工具提示。这使用户能够交互式地探索数据点,并通过访问与绘制数据相关的其他信息获得更深入的见解。
带注释的光标功能
以下是 Matplotlib 小部件的带注释光标的功能。
交互式光标
带注释的光标类似于标准光标,但它使用户能够通过在光标移过数据点时显示坐标信息(x、y 值)来浏览绘图。
注释功能
带注释的光标除了用于显示坐标外,还允许使用文本或标记标记或注释特定数据点,以突出显示或提供有关这些点的其他信息。
自定义
光标和注释的可自定义属性,例如标记样式、文本内容、位置、颜色和其他视觉属性。
Matplotlib 中的带注释的光标
在 Matplotlib 中使用光标小部件涉及捕获光标移动或点击并执行函数以使用注释或有关特定数据点的信息来增强绘图。让我们更深入地了解如何使用光标小部件在 Matplotlib 中创建带注释的小部件。
带注释的小部件中的光标小部件
要在带注释的小部件中使用光标小部件,有两种方法。让我们一一看看它们。
事件处理
带注释的光标中的事件处理涉及捕获特定事件(例如光标移动或鼠标点击)并执行函数以在 Matplotlib 中的绘图中添加注释或删除注释。以下是带注释的光标中事件处理的详细分解。
定义事件处理函数
以下是必须为事件处理定义的函数。
annotate_point(event) - 此函数在光标悬停在绘图上时触发。它检索光标坐标,找到最近的数据点并使用信息(例如,x 和 y 值)对其进行注释。
在鼠标点击时删除注释
remove_annotation(event) - 此函数在绘图区域内发生鼠标点击时删除注释。
处理事件
plt.connect('motion_notify_event', annotate_point) - 将光标移动事件连接到 annotate_point 函数。当光标移过绘图时,将触发此函数以注释最近的数据点。
plt.connect('button_press_event', remove_annotation) - 将鼠标点击事件连接到 remove_annotation 函数。当绘图区域内发生鼠标点击时,此函数会删除注释。
带注释的光标中事件处理的示例
在此示例中,我们演示了带注释的光标中的事件处理。当光标悬停在绘图上时,会动态添加注释以显示有关特定数据点的信息。在绘图区域内点击后,注释将被删除,从而可以清楚地探索绘图而不会出现混乱。
示例
import matplotlib.pyplot as plt import numpy as np # Generating sample data x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a plot fig, ax = plt.subplots() line, = ax.plot(x, y, label='Sine Wave') # Annotate points on cursor hover def annotate_point(event): if event.inaxes: x_cursor, y_cursor = event.xdata, event.ydata index = np.argmin(np.abs(x - x_cursor)) # Find nearest index to the cursor position ax.annotate( f'({x[index]:.2f}, {y[index]:.2f})', xy=(x[index], y[index]), xytext=(x[index] + 1, y[index] + 0.5), arrowprops=dict(facecolor='black', arrowstyle='->'), fontsize=8, color='black') fig.canvas.draw_idle() # Remove annotations on mouse click def remove_annotation(event): if event.inaxes: for annotation in ax.texts: annotation.remove() fig.canvas.draw_idle() # Connect events to functions plt.connect('motion_notify_event', annotate_point) plt.connect('button_press_event', remove_annotation) plt.show()
输出
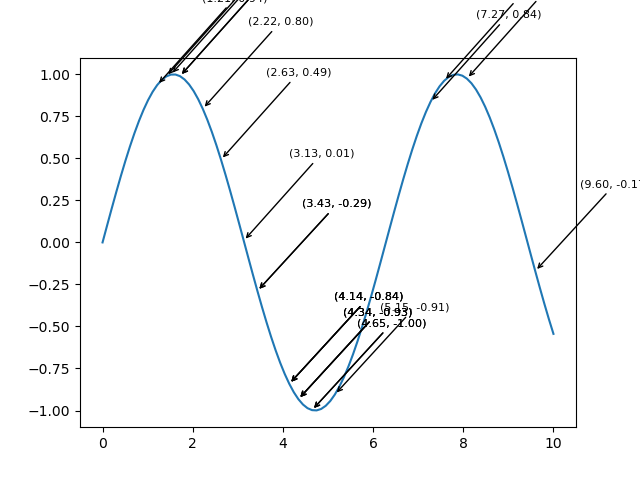
添加注释
注释或文本标签添加到绘图中,以显示有关数据点的信息,当光标悬停在其上或单击它们时。注释可以包含数据值、坐标或与绘制数据相关的任何相关信息。
带注释的小部件中的光标小部件
此示例说明了如何使用光标小部件在光标移过绘图时动态注释数据点,从而提供有关特定坐标的有价值信息。通过允许清楚地探索绘图,在单击时删除注释。
示例
import matplotlib.pyplot as plt import numpy as np # Generating sample data x = np.linspace(0, 10, 100) y = np.cos(x) # Creating a plot fig, ax = plt.subplots() line, = ax.plot(x, y, label='Cosine Wave') # Annotate points on mouse hover def annotate_point(event): if event.inaxes: x_cursor, y_cursor = event.xdata, event.ydata index = np.argmin(np.abs(x - x_cursor)) # Find nearest index to the cursor position ax.annotate( f'({x[index]:.2f}, {y[index]:.2f})', xy=(x[index], y[index]), xytext=(x[index] + 1, y[index] + 0.5), arrowprops=dict(facecolor='black', arrowstyle='->'), fontsize=8, color='black') fig.canvas.draw_idle() # Remove annotations on mouse click def remove_annotation(event): if event.inaxes: for annotation in ax.texts: annotation.remove() fig.canvas.draw_idle() # Connect events to functions plt.connect('motion_notify_event', annotate_point) plt.connect('button_press_event', remove_annotation) plt.show()
输出
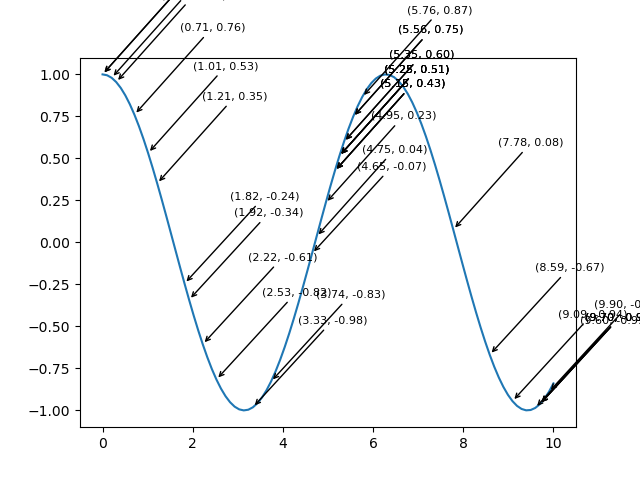
用例
数据突出显示:使用注释强调特定数据点或区域。
信息标记 - 为关键数据点提供其他详细信息或标签。
交互式探索 - 将光标交互与注释结合起来以进行动态数据探索。