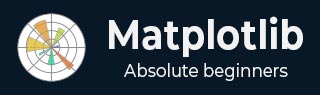
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图片
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性与对数比例尺
- Matplotlib - 对称对数和 Logit 比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 启用注释中的 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图元
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化程序
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定图元
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 区间选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 按键事件
在计算机编程中,检测和处理按键事件对于捕获用户输入并在应用程序中启用各种功能至关重要。无论是浏览菜单、输入文本、触发操作还是控制交互式元素,按键事件都在用户交互中扮演着关键角色。
Matplotlib 提供了处理按键事件的工具,允许您在交互式绘图会话中捕获和响应键盘输入。当按下键时,Matplotlib 会触发key_press_event,允许用户根据按下的键定义自定义操作。
该库默认附加了一些按键回调以增强交互性。这些默认回调在 Matplotlib 文档的“导航键盘快捷键”部分中有说明。
示例
让我们来看这个基本的示例,它演示了 Matplotlib 中默认的按键事件。
import numpy as np import matplotlib.pyplot as plt # Define a function to handle keypress events def press(event): print('You pressed:', event.key) # Create a Matplotlib figure and axis fig, ax = plt.subplots() # Connect the keypress event handler to the figure canvas cid = fig.canvas.mpl_connect('key_press_event', press) # Plot sin wave x = np.linspace(0, 10, 100) ax.plot(x, np.sin(x)) # Display the plot plt.show()
输出
执行上述程序后,您将获得以下图形,然后按任意键观察此示例的工作方式:
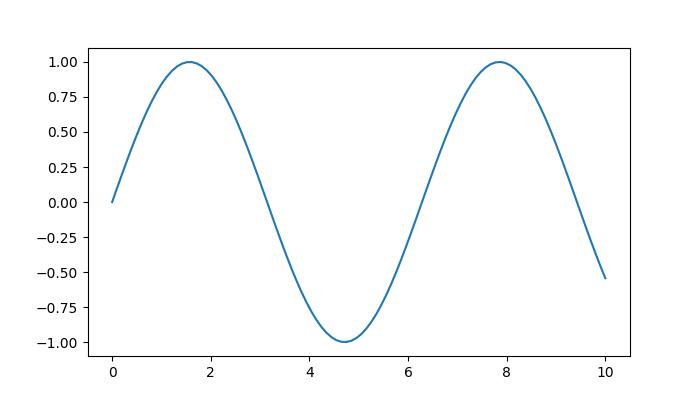
You pressed: tab You pressed: caps_lock You pressed: shift You pressed: control You pressed: alt You pressed: meta You pressed: a You pressed: b You pressed: c You pressed: d You pressed: s You pressed: g You pressed: g You pressed: g You pressed: g You pressed: k You pressed: k You pressed: k You pressed: k You pressed: k You pressed: k You pressed: l You pressed: l You pressed: p You pressed: p You pressed: q
观看下面的视频,了解此按键事件功能在此处如何工作。

从以上输出中,您可以观察到一些默认键的工作方式,例如“g”(切换网格),“s”(保存图形),“q”(关闭图形)等等。
创建自定义按键事件函数
要根据特定需求处理按键事件,您可以创建一个自定义函数,并使用mpl_connect方法将其连接到key_press_event。
示例
以下示例演示了一个简单的按键事件函数,当按下“x”键时,该函数会切换 x 轴标签的可见性。
import sys import matplotlib.pyplot as plt import numpy as np def on_press(event): print('Pressed key:', event.key) sys.stdout.flush() # Toggle visibility of xlabel when 'x' key is pressed if event.key == 'x': visible = xl.get_visible() xl.set_visible(not visible) fig.canvas.draw() fig, ax = plt.subplots() fig.canvas.mpl_connect('key_press_event', on_press) # Plot random data points ax.plot(np.random.rand(12), np.random.rand(12), 'go') xl = ax.set_xlabel('Toggle this label when the "x" key is pressed', fontsize=16, color='red') ax.set_title('Press x key') plt.show()
输出
执行上述程序后,您将获得以下图形,然后按任意键观察此示例的工作方式:
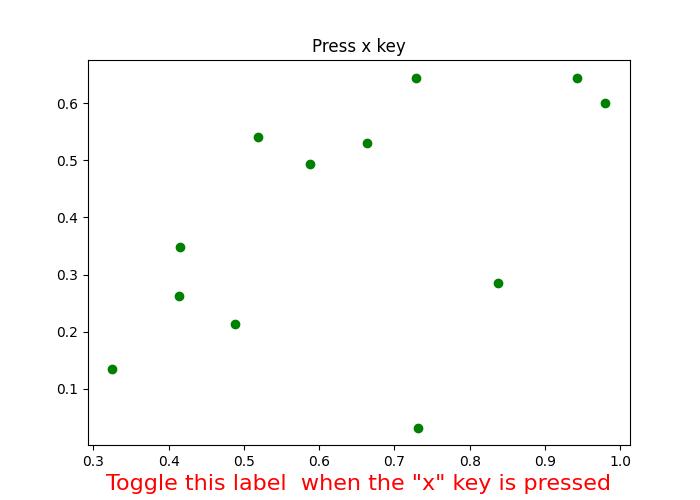
Pressed key: x Pressed key: x Pressed key: x Pressed key: x Pressed key: a Pressed key: enter
观看下面的视频,了解此按键事件功能在此处如何工作。

示例
这是另一个示例,它演示了如何添加Esc键以退出图形(除了“q”键之外),并计算Enter键被按下的次数。
import numpy as np import matplotlib.pyplot as plt # Define a function to handle keypress events def press(event): print('Pressed key:', event.key) # If the 'enter' key is pressed, append 1 to the count list if event.key == 'enter': cnt.append(1) # If the 'escape' key is pressed, close the plot if event.key == "escape": plt.close() cnt = [] # Create a figure fig, ax = plt.subplots() # Connect the keypress event handler to the figure canvas fig.canvas.mpl_connect('key_press_event', press) ax.plot(np.random.rand(12), np.random.rand(12), 'go') plt.show() # Calculate and print the sum of counts result = sum(cnt) print(result, cnt)
输出
执行上述程序后,您将获得以下图形,然后按任意键观察此示例的工作方式:
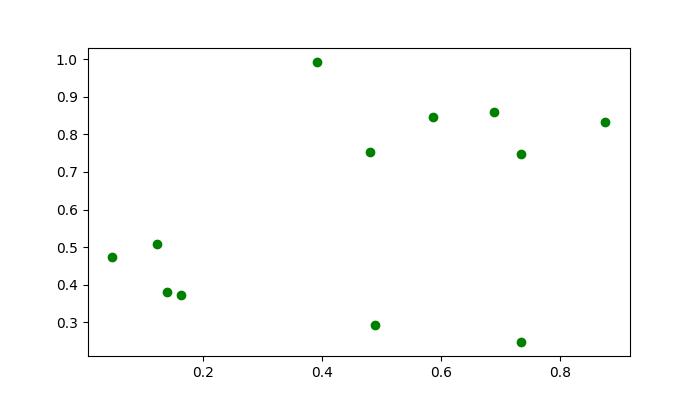
Pressed key: b Pressed key: enter Pressed key: enter Pressed key: caps_lock Pressed key: caps_lock Pressed key: enter Pressed key: escape 3 [1, 1, 1]

广告