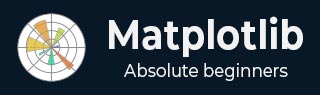
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性与对数比例尺
- Matplotlib - 对称对数与 Logit 比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 启用注释中的 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图形元素
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - subplots() 函数
- Matplotlib - subplot2grid() 函数
- Matplotlib - 锚定图形元素
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 计时器
- Matplotlib - viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - Span Selector
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 基本单位
什么是基本单位?
在 Matplotlib 中,基本单位指的是用于定义和测量绘图各个方面(例如长度、宽度、位置和坐标)的基本元素。了解这些单位对于准确地在绘图中放置元素和控制其整体布局至关重要。
Matplotlib 中常见的基本单位
以下是 Matplotlib 库中常见的可用基本单位。
磅 (pt)
在 Matplotlib 中,**磅**通常指的是用于指定绘图中各种视觉属性(例如线宽、标记大小和文本大小)的度量单位。磅 (pt) 是排版和图形学中的标准单位,经常因其与设备无关的大小表示而被使用。
磅在 Matplotlib 中的用法
**线宽** - Matplotlib 函数(例如 **plt.plot()**)中的 **linewidth** 参数允许我们以磅为单位指定线的宽度。
**文本大小** - Matplotlib 函数 **plt.xlabel()、plt.ylabel()、plt.title()** 中的 **fontsize** 参数允许设置文本大小(以磅为单位)。
**标记大小** - plot 函数(例如 plt.plot())中的 **markersize** 参数以磅为单位定义标记的大小。
**图形大小** - 使用 **plt.figure(figsize=(width, height))** 指定图形大小时,尺寸以英寸为单位。但是,默认大小在不同系统上可能有所不同,例如 **1** 英寸并不总是等于 **72** 磅,但这是一种典型的约定。
磅与其他单位的转换
Matplotlib 在内部使用磅,但在设置图形大小或尺寸时,通常使用英寸。作为参考,**1** 英寸大约等于 **72** 磅。
- 1 英寸 = 72 磅
- 1 磅 ≈ 0.01389 英寸
理解和利用磅作为 Matplotlib 中的度量单位,可以精确控制绘图中元素的视觉属性,确保所需的显示效果和可读性。
示例
在这个例子中,我们为线图设置了以磅为单位的线宽。
import matplotlib.pyplot as plt plt.figure(figsize=(6, 4)) # 6 inches wide, 4 inches tall plt.plot([1, 2, 3], [4, 5, 6], linewidth=3.5) # Line width in points plt.xlabel('X-axis', fontsize=12) # Text size in points plt.ylabel('Y-axis', fontsize=12) plt.show()
输出
以下是上述代码的输出 -
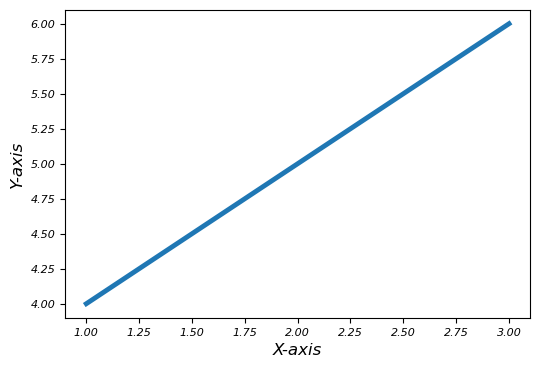
英寸 (in)
英寸用于在 Matplotlib 中指定图形或子图的整体大小。例如,**figsize = (width, height)**(以英寸为单位)定义图形的宽度和高度。
示例
在这个例子中,我们以英寸为单位指定了图形的大小。
import matplotlib.pyplot as plt plt.figure(figsize=(4, 4)) # 6 inches wide, 4 inches tall plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Example Plot with 4x4 Inches') plt.show()
输出
以下是上述代码的输出 -
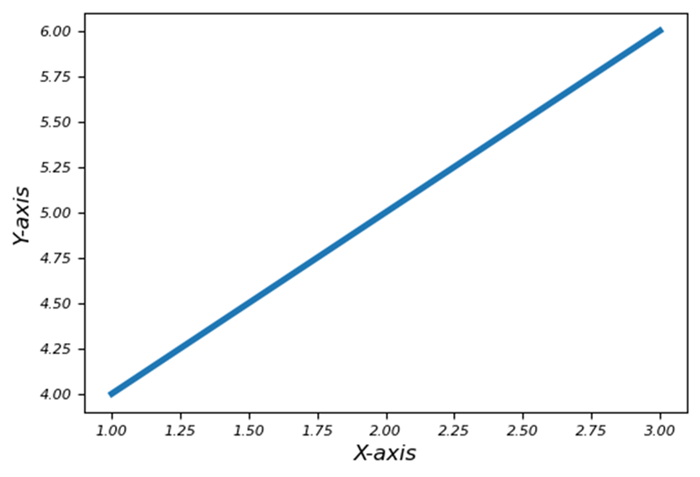
转换
英寸与 Matplotlib 中常用的其他单位之间的转换系数
1 英寸 ≈ 2.54 厘米
数据单位
数据单位表示沿 x 轴和 y 轴绘制的实际值。Matplotlib 使用这些单位将数据点绘制到图表上。从数据单位到绘图上物理单位的转换由坐标轴比例尺(例如线性、对数等)决定。
关于 Matplotlib 中数据单位的关键点
**数值数据** - 数据单位表示我们沿 x 轴和 y 轴绘制的数值。
**映射到绘图空间** - Matplotlib 根据定义的坐标轴限制和缩放比例,将这些数值数据值映射到绘图空间中的特定位置。
**绘制数据** - 当我们使用 Matplotlib 绘制数据时,我们必须使用数值数据指定 x 和 y 坐标。Matplotlib 会处理这些数据点在绘图中的缩放和定位。
**坐标轴限制** - 使用 **xlim** 设置 x 轴限制,使用 **ylim** 设置 y 轴限制,定义绘图中显示的数据单位范围。
**坐标轴刻度** - 坐标轴上的刻度表示特定数据单位,为绘图中存在的数据范围提供视觉参考。
示例
在这个例子中,我们设置了绘图的数据单位。
import matplotlib.pyplot as plt # Sample data x = [1, 2, 3, 4, 5] y = [2, 4, 6, 8, 10] # Plotting using data units plt.plot(x, y, marker='o') # Setting axis limits in data units plt.xlim(0, 6) # X-axis limits from 0 to 6 plt.ylim(0, 12) # Y-axis limits from 0 to 12 plt.xlabel('X-axis (Data Units)') plt.ylabel('Y-axis (Data Units)') plt.title('Plot with Data Units') plt.show()
输出
以下是上述代码的输出 -
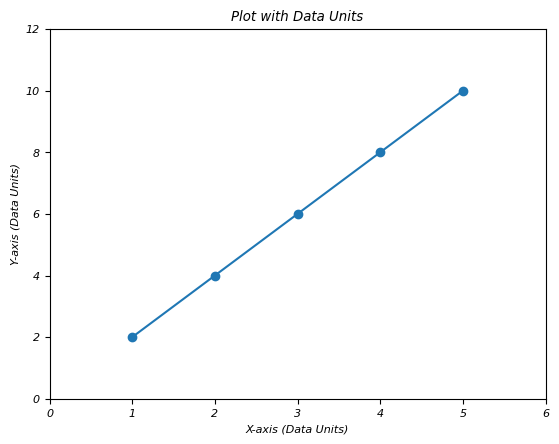
理解 Matplotlib 中的数据单位对于准确地可视化数据并确保绘制的表示与底层数值正确对应至关重要。
坐标轴坐标
坐标轴坐标的范围是从 0.0 到 1.0,它指定单个子图或坐标轴内的相对位置。**(0, 0)** 表示子图的左下角,**(1, 1)** 表示子图的右上角。
坐标轴坐标提供了一种灵活的方法,可以在子图内相对于其大小和边界来定位注释、文本或其他元素,确保在不同绘图大小或尺寸下的一致性和响应性定位。
示例
在这个例子中,我们设置了绘图的坐标轴坐标 x 和 y。
import matplotlib.pyplot as plt # Creating a subplot fig, ax = plt.subplots() # Plotting data ax.plot([1, 2, 3], [2, 4, 3]) # Annotating at axes coordinates ax.annotate('Important Point', xy=(2, 4), # Data point to annotate xytext=(0.5, 0.5), # Position in axes coordinates textcoords='axes fraction', # Specifying axes coordinates arrowprops=dict(facecolor='black', arrowstyle='->')) plt.show()
输出
以下是上述代码的输出 -
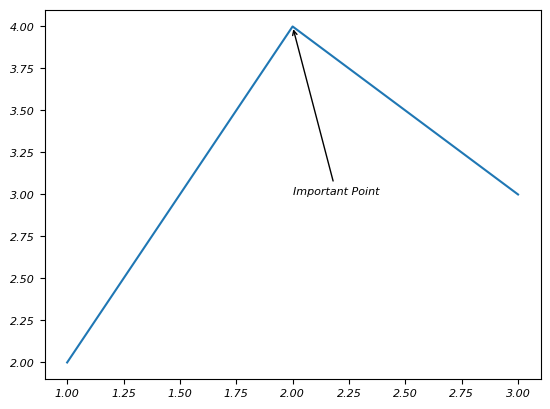
图形坐标
图形坐标的范围也是从 **0.0** 到 **1.0**,但是它相对于整个图形画布指定位置。**(0, 0)** 是整个图形的左下角,**(1, 1)** 是整个图形的右上角。
示例
这是一个设置绘图图形坐标的示例。
import matplotlib.pyplot as plt # Creating subplots fig, (ax1, ax2) = plt.subplots(1, 2) # Plotting data in subplot 1 ax1.plot([1, 2, 3], [2, 4, 3]) # Plotting data in subplot 2 ax2.plot([1, 2, 3], [3, 1, 4]) # Annotating at figure coordinates fig.text(0.5, 0.9, 'Figure Annotation', ha='center', fontsize=12, color='red') plt.show()
输出
以下是上述代码的输出 -
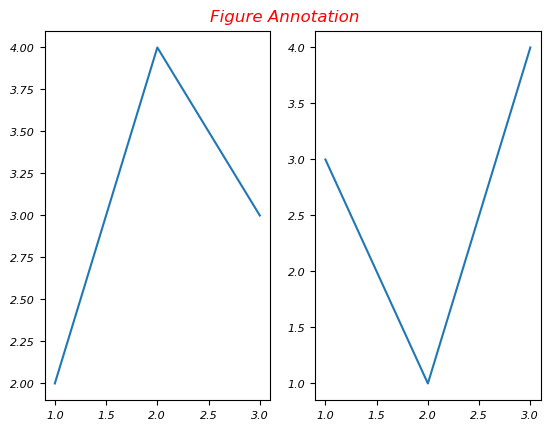
使用 Matplotlib 绘制所有基本单位的图形
此示例展示了在 Matplotlib 中使用各种单位,例如英寸表示图形大小,磅表示文本大小,数据单位表示绘制数据点,以及坐标轴坐标表示在子图中定位注释。
示例
import matplotlib.pyplot as plt # Define a figure with specified size in inches fig = plt.figure(figsize=(6, 4)) # Width: 6 inches, Height: 4 inches # Create an axis within the figure ax = fig.add_subplot(111) # Plotting data points using data units x = [1, 2, 3, 4] y = [2, 4, 6, 8] ax.plot(x, y, marker='o') # Setting labels and titles using text in points ax.set_xlabel('X-axis Label', fontsize=12) ax.set_ylabel('Y-axis Label', fontsize=12) ax.set_title('Plot Title', fontsize=14) # Setting the position of a text annotation using axes coordinates ax.text(0.5, 0.5, 'Annotation', transform=ax.transAxes, fontsize=10) plt.show()
输出
以下是上述代码的输出 -
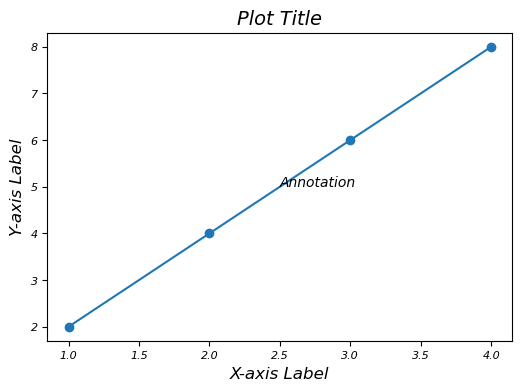