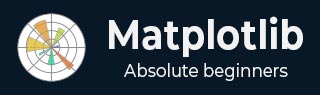
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境搭建
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色映射
- Matplotlib - 颜色映射归一化
- Matplotlib - 选择颜色映射
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性刻度和对数刻度
- Matplotlib - 对称对数刻度和logit刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是LaTeX?
- Matplotlib - LaTeX用于数学表达式
- Matplotlib - 在注释中使用LaTeX文本格式
- Matplotlib - PostScript
- 启用注释中的LaTeX渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用Cycler进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴刻度
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定绘图元素
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 缎带框
- Matplotlib - 填充螺旋线
- Matplotlib - findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建Logo
- Matplotlib - 多页PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 游标小部件
- Matplotlib - 带注释的游标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 区间选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 路径
路径是指引导从一个地方到另一个地方移动的路线(轨迹)。它可以是物理的,例如小径或道路,也可以是抽象的,例如实现目标的一系列步骤。路径提供方向并帮助我们浏览空间。它们标志着到达目的地的途径。
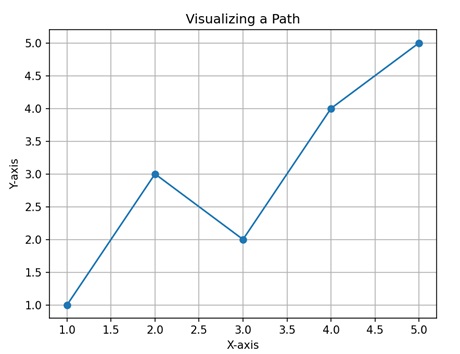
Matplotlib中的路径
在 Matplotlib 中,路径是用于在绘图上绘制形状和线条的基本对象。路径由一系列连接的点组成,称为顶点或节点,以及关于如何连接这些点以形成形状(如线、曲线或多边形)的指令。
可以使用“Path”类在 Matplotlib 中创建路径。这些路径可以用于创建各种类型的绘图,例如线、曲线、多边形,甚至自定义形状。路径提供了一种定义绘图中对象外观的方法,允许您控制它们的大小、位置和样式。
让我们从绘制一条基本的直线路径开始。
直线路径
在 Matplotlib 中,直线路径指的是由两点用直线连接的基本几何元素。此路径是通过指定线的起点(通常称为“原点”)和终点的坐标来创建的。然后,Matplotlib 在这两点之间绘制一条直线,形成路径。
示例
在下面的示例中,我们使用 Matplotlib 中的 Path 类创建一个直线路径。我们定义了两个顶点,分别表示线的起点和终点。此外,我们指定路径代码以指示路径移动到第一个顶点,然后创建一条线到第二个顶点。通过使用这些顶点和代码构造路径,我们实现了直线的表示 -
import matplotlib.pyplot as plt from matplotlib.path import Path import matplotlib.patches as patches # Defining the vertices for the straight line path verts = [ # Starting point (0, 0), # Ending point (1, 1) ] # Defining the codes for the straight line path codes = [Path.MOVETO, Path.LINETO] # Creating the straight line path path = Path(verts, codes) # Plotting the path fig, ax = plt.subplots() patch = patches.PathPatch(path, facecolor='none', lw=2) ax.add_patch(patch) ax.set_title('Straight Line Path') ax.set_xlim(0, 1) ax.set_ylim(0, 1) ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') ax.grid(True) plt.show()
输出
以下是上述代码的输出 -
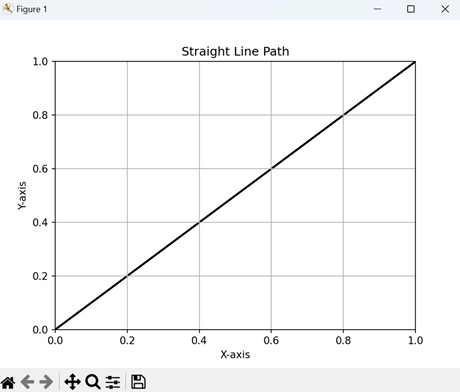
圆形路径
在 Matplotlib 中,圆形路径表示绘图上绘制的圆形。它是通过指定圆的中心点及其半径来创建的。然后,Matplotlib 在圆的圆周周围生成一系列点,并将它们连接起来形成一个封闭的路径。
示例
在这里,我们使用 Matplotlib 中的 Path 类创建一个圆形路径。我们通过定义在单位圆周围均匀分布的角度来生成圆周上的一系列顶点。使用三角函数,我们计算这些顶点的坐标。随后,我们定义路径代码以用线连接这些顶点 -
import matplotlib.pyplot as plt from matplotlib.path import Path import matplotlib.patches as patches import numpy as np # Defining the angles for the circle path theta = np.linspace(0, 2*np.pi, 100) # Defining the vertices for the circle path verts = np.column_stack([np.cos(theta), np.sin(theta)]) # Definng the codes for the circle path codes = [Path.MOVETO] + [Path.LINETO] * (len(verts) - 1) # Creating the circle path path = Path(verts, codes) # Plotting the path fig, ax = plt.subplots() patch = patches.PathPatch(path, facecolor='none', lw=2) ax.add_patch(patch) ax.set_title('Circle Path') ax.set_xlim(-1.1, 1.1) ax.set_ylim(-1.1, 1.1) ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') ax.grid(True) plt.show()
输出
执行上述代码后,我们将得到以下输出 -
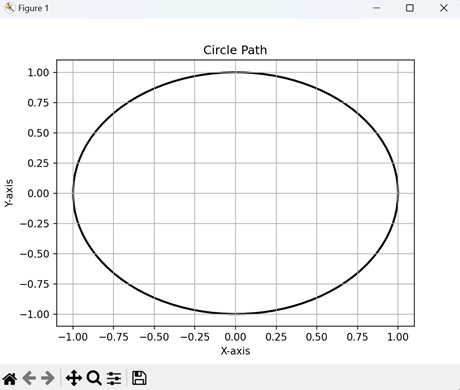
贝塞尔曲线路径
在 Matplotlib 中,贝塞尔曲线路径是用控制点创建的平滑曲线。
要构造贝塞尔曲线,您需要指定一系列控制点来引导曲线的路径。这些控制点决定曲线的走向和形状,使您可以创建平滑流畅的形状。然后,Matplotlib 通过连接这些控制点来生成曲线。
示例
现在,我们使用 Path 类在 Matplotlib 中创建一个贝塞尔曲线路径。我们指定定义贝塞尔曲线形状的控制点。此外,我们定义路径代码以指示连续控制点之间曲线段的类型。通过使用这些控制点和代码构造路径,我们创建了一个平滑的贝塞尔曲线 -
import matplotlib.pyplot as plt from matplotlib.path import Path import matplotlib.patches as patches # Defining the control points for the Bezier curve verts = [ (0.1, 0.1), (0.2, 0.8), (0.8, 0.8), (0.9, 0.1), ] # Defining the codes for the Bezier curve path codes = [Path.MOVETO, Path.CURVE4, Path.CURVE4, Path.CURVE4] # Creating the Bezier curve path path = Path(verts, codes) # Plotting the path fig, ax = plt.subplots() patch = patches.PathPatch(path, facecolor='none', lw=2) ax.add_patch(patch) ax.set_title('Bezier Curve Path') ax.set_xlim(0, 1) ax.set_ylim(0, 1) ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') ax.grid(True) plt.show()
输出
执行上述代码后,我们将得到以下输出 -
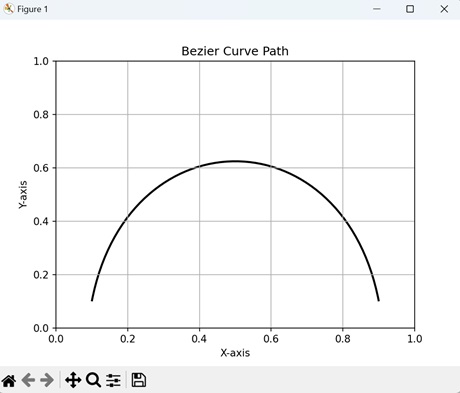
多边形路径
在 Matplotlib 中,多边形路径表示具有多条直边的封闭形状,类似于几何多边形。此形状是通过按特定顺序指定其顶点或角点的坐标来创建的。然后,Matplotlib 顺序连接这些点以形成多边形的边,并通过连接最后一个点到第一个点来闭合路径。
多边形路径是用于表示各种形状的多功能元素,例如三角形、矩形、五边形或任何其他多边形。它们可以用颜色填充以表示区域,或用作轮廓以突出显示绘图中的特定区域。
示例
在此示例中,我们使用 Matplotlib 中的 Path 类创建一个多边形路径。我们定义了多边形的顶点,表示多边形形状的角点。此外,我们指定路径代码以连接这些顶点并闭合多边形。通过使用顶点和代码构造路径,我们形成了一个封闭的多边形形状 -
import matplotlib.pyplot as plt from matplotlib.path import Path import matplotlib.patches as patches # Defining the vertices for the polygon verts = [ (0.1, 0.1), (0.5, 0.9), (0.9, 0.1), # Closing the polygon (0.1, 0.1) ] # Defining the codes for the polygon path codes = [Path.MOVETO, Path.LINETO, Path.LINETO, Path.CLOSEPOLY] # Creating the polygon path path = Path(verts, codes) # Plotting the path fig, ax = plt.subplots() patch = patches.PathPatch(path, facecolor='none', lw=2) ax.add_patch(patch) ax.set_title('Polygon Path') ax.set_xlim(0, 1) ax.set_ylim(0, 1) ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') ax.grid(True) plt.show()
输出
执行上述代码后,我们将得到以下输出 -
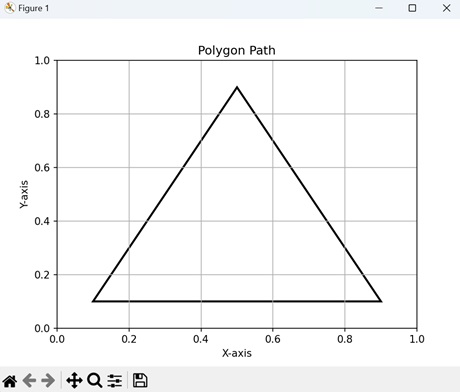