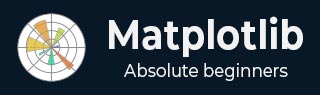
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性与对数比例尺
- Matplotlib - 对称对数与Logit比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - 在注释中使用LaTeX文本格式
- Matplotlib - PostScript
- 在注释中启用LaTex渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用Cycler进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - subplots() 函数
- Matplotlib - subplot2grid() 函数
- Matplotlib - 固定位置绘图元素
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 区间选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 矢羽图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 刻度格式化器
理解刻度和刻度标签
一般来说,在图表和绘图中,刻度是显示x轴和y轴比例尺的小短线,清晰地表示每个刻度关联的值。刻度标签是与轴上每个刻度关联的文本或数字注释,清晰地表示每个刻度关联的值。
下图显示了图表上的刻度和刻度标签:
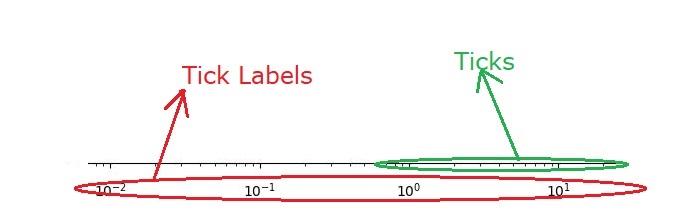
在此背景下,**刻度格式化器**控制刻度标签的外观,指定刻度标记的显示方式。此自定义可以包括格式选项,例如指定小数位数、添加单位、使用科学计数法或应用日期和时间格式。
Matplotlib中的刻度格式化器
Matplotlib允许用户通过**matplotlib.ticker**模块自定义刻度属性,包括位置和标签。此模块包含用于配置刻度定位和格式化的类。它提供了一系列通用的刻度定位器和格式化器,以及特定领域的自定义刻度定位器和格式化器。
要设置刻度格式,Matplotlib允许您使用:
- 一个**格式字符串**,
- 一个**函数**,
- 或一个**Formatter子类**的实例。
应用刻度格式化器
Matplotlib提供了一种直接的方法来使用set_major_formatter和set_minor_formatter函数配置刻度格式化器。这些函数使您可以为特定轴设置主刻度和次刻度标签格式。
语法如下:
语法
ax.xaxis.set_major_formatter(xmajor_formatter) ax.xaxis.set_minor_formatter(xminor_formatter) ax.yaxis.set_major_formatter(ymajor_formatter) ax.yaxis.set_minor_formatter(yminor_formatter)
字符串格式化
字符串格式化是一种技术,它隐式地创建StrMethodFormatter方法,允许您使用新式格式字符串(str.format)。
示例
在这个示例中,x轴刻度标签将使用字符串进行格式化。
import matplotlib.pyplot as plt from matplotlib import ticker # Create a sample plot fig, ax = plt.subplots(figsize=(7,4)) ax.plot([1, 2, 3, 4], [10, 20, 15, 20]) # Set up the major formatter for the x-axis ax.xaxis.set_major_formatter('{x} km') ax.set_title('String Formatting') plt.show()
输出
执行上述代码后,我们将得到以下输出:
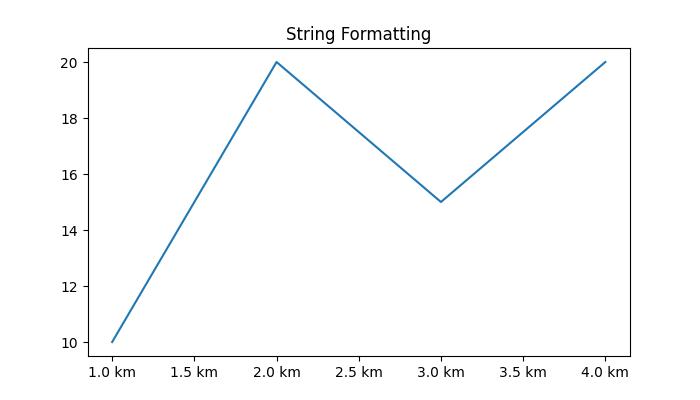
基于函数的格式化
这种方法提供了一种灵活的方式,可以使用**用户定义**函数自定义刻度标签。该函数应接受两个输入:x(刻度值)和pos(轴上刻度的位置)。然后它返回一个字符串,表示与给定输入对应的所需刻度标签。
示例
此示例演示了如何使用函数格式化x轴刻度标签。
from matplotlib.ticker import FuncFormatter from matplotlib import pyplot as plt def format_tick_labels(x, pos): return '{0:.2f}%'.format(x) # sample data values = range(20) # Create a plot f, ax = plt.subplots(figsize=(7,4)) ax.plot(values) # Set up the major formatter for the x-axis using a function ax.xaxis.set_major_formatter(FuncFormatter(format_tick_labels)) ax.set_title('Function Based Formatting') plt.show()
输出
执行上述代码后,我们将得到以下输出:
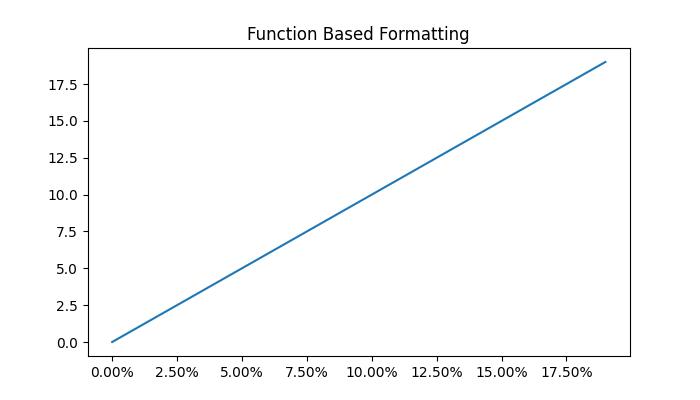
格式化器对象格式化
格式化器对象格式化允许使用特定的格式化器子类高级自定义刻度标签。一些常见的Formatter子类包括:
- **NullFormatter** - 此对象确保刻度上不显示任何标签。
- **StrMethodFormatter** - 此对象使用字符串str.format方法格式化刻度标签。
- **FormatStrFormatter** - 此对象使用%-style格式化刻度标签。
- **FuncFormatter** - 它通过自定义函数定义标签。
- **FixedFormatter** - 它允许用户显式设置标签字符串。
- **ScalarFormatter** - 它是标量的默认格式化器。
- **PercentFormatter** - 它将标签格式化为百分比。
示例1
以下示例演示了如何将不同的Formatter对象应用于x轴,以在刻度标签上实现不同的格式化效果。
from matplotlib import ticker from matplotlib import pyplot as plt # Create a plot fig, axs = plt.subplots(5, 1, figsize=(7, 5)) fig.suptitle('Formatter Object Formatting', fontsize=16) # Set up the formatter axs[0].xaxis.set_major_formatter(ticker.NullFormatter()) axs[0].set_title('NullFormatter()') # Add other formatters axs[1].xaxis.set_major_formatter(ticker.StrMethodFormatter("{x:.3f}")) axs[1].set_title('StrMethodFormatter("{x:.3f}")') axs[2].xaxis.set_major_formatter(ticker.FormatStrFormatter("#%d")) axs[2].set_title('FormatStrFormatter("#%d")') axs[3].xaxis.set_major_formatter(ticker.ScalarFormatter(useMathText=True)) axs[3].set_title('ScalarFormatter(useMathText=True)') axs[4].xaxis.set_major_formatter(ticker.PercentFormatter(xmax=5)) axs[4].set_title('PercentFormatter(xmax=5)') plt.tight_layout() plt.show()
输出
执行上述代码后,我们将得到以下输出:
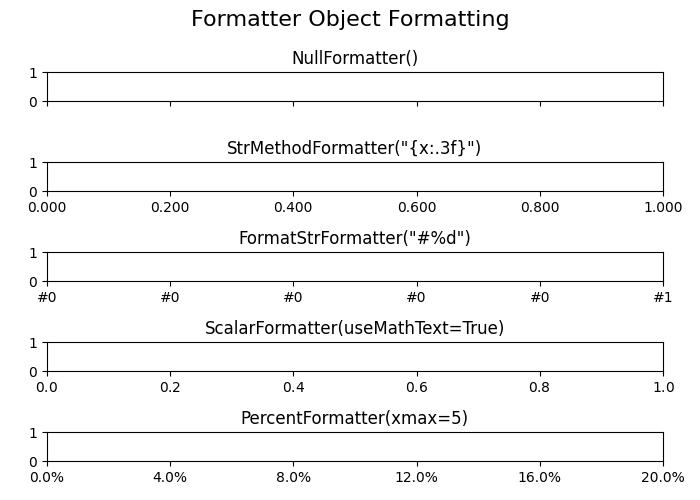
示例2
此示例演示了如何使用字符串格式化方法(StrMethodFormatter)格式化x轴和y轴上的刻度标签,以显示带逗号分隔符的数字。
import matplotlib.pyplot as plt from matplotlib.ticker import StrMethodFormatter # Data x = [10110, 20110, 40110, 6700] y = [20110, 10110, 30110, 9700] # Create plot fig, ax = plt.subplots(figsize=(7, 4)) ax.plot(x, y) # Format tick labels for both x-axis and y-axis to include comma separators ax.yaxis.set_major_formatter(StrMethodFormatter('{x:,}')) ax.xaxis.set_major_formatter(StrMethodFormatter('{x:,}')) # Show plot plt.show()
输出
执行上述代码后,您将得到以下输出:
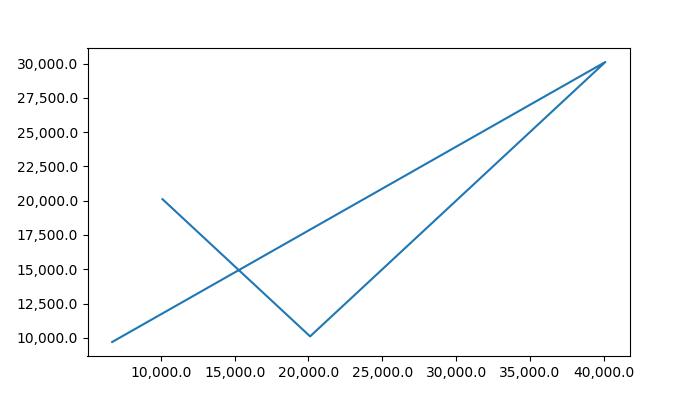