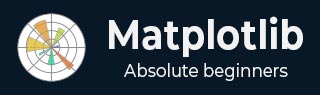
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图片
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色映射
- Matplotlib - 颜色映射归一化
- Matplotlib - 选择颜色映射
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性和对数比例尺
- Matplotlib - 对称对数和 Logit 比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 启用注释中的 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定绘图元素
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 飘带框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 游标小部件
- Matplotlib - 带注释的游标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标游标
- Matplotlib - 多游标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 矢羽图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 选择颜色映射
颜色映射 也称为颜色表或调色板,是一系列表示连续值范围的颜色。允许您通过颜色变化有效地表示信息。
请参见下图,其中引用了 matplotlib 中的一些内置颜色映射 -
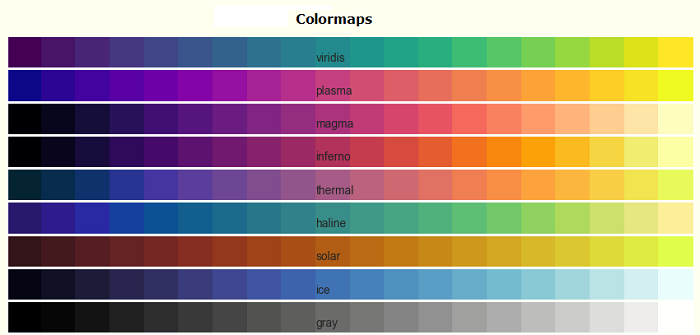
Matplotlib 提供了各种内置(在 matplotlib.colormaps 模块中可用)和第三方颜色映射,适用于各种应用。
在 Matplotlib 中选择颜色映射
选择合适的颜色映射涉及为您的数据集找到在 3D 颜色空间中的合适表示。
为任何给定的数据集选择合适的颜色映射的因素包括 -
数据的性质 - 是否表示形式数据或度量数据
对数据集的了解 - 理解数据集的特性。
直观的颜色方案 - 考虑被绘制参数是否存在直观的颜色方案。
领域标准 - 考虑观众是否可能期望该领域的标准。
对于大多数应用,建议使用感知上统一的颜色映射,以确保数据中的相等步长在颜色空间中被感知为相等步长。这提高了人脑的解释能力,尤其是在亮度变化比色相变化更容易感知的情况下。
颜色映射的类别
颜色映射根据其功能进行分类 -
顺序型 - 亮度和饱和度的增量变化,通常使用单一色调。适用于表示有序信息。
发散型 - 两种颜色的亮度和饱和度变化,在不饱和颜色处相遇。非常适合具有关键中间值的数据,例如地形或围绕零偏差的数据。
循环型 - 两种颜色的亮度变化,在中间相遇,并在端点处开始/结束于不饱和颜色。适用于在端点处环绕的值,例如相位角或一天中的时间。
定性型 - 各种颜色,没有特定的顺序。用于表示没有排序或关系的信息。
顺序型颜色映射
顺序型颜色映射显示随着其在颜色映射中增加,亮度值单调递增。此特性确保颜色变化感知的平滑过渡,使其适合表示有序信息。但是,必须注意,根据其跨越的值范围,颜色映射之间的感知范围可能会有所不同。
让我们通过可视化它们的渐变并了解它们的亮度值如何演变来探索顺序型颜色映射。
示例
以下示例提供了 Matplotlib 中可用的不同顺序型颜色映射的渐变的可视化表示。
import matplotlib.pyplot as plt import numpy as np import matplotlib as mpl # List of Sequential colormaps to visualize cmap_list = ['Greys', 'Purples', 'Blues', 'Greens', 'Oranges', 'Reds', 'YlOrBr', 'YlOrRd', 'OrRd', 'PuRd', 'RdPu', 'BuPu', 'GnBu', 'PuBu', 'YlGnBu', 'PuBuGn', 'BuGn', 'YlGn'] # Plot the color gradients gradient = np.linspace(0, 1, 256) gradient = np.vstack((gradient, gradient)) # Create figure and adjust figure height to the number of colormaps nrows = len(cmap_list) figh = 0.35 + 0.15 + (nrows + (nrows - 1) * 0.1) * 0.22 fig, axs = plt.subplots(nrows=nrows + 1, figsize=(7, figh)) fig.subplots_adjust(top=1 - 0.35 / figh, bottom=0.15 / figh, left=0.2, right=0.99) axs[0].set_title('Sequential colormaps', fontsize=14) for ax, name in zip(axs, cmap_list): ax.imshow(gradient, aspect='auto', cmap=mpl.colormaps[name]) ax.text(-0.1, 0.5, name, va='center', ha='right', fontsize=10, transform=ax.transAxes) # Turn off all ticks & spines, not just the ones with colormaps. for ax in axs: ax.set_axis_off() # Show the plot plt.show()
输出
执行上述代码后,我们将获得以下输出 -
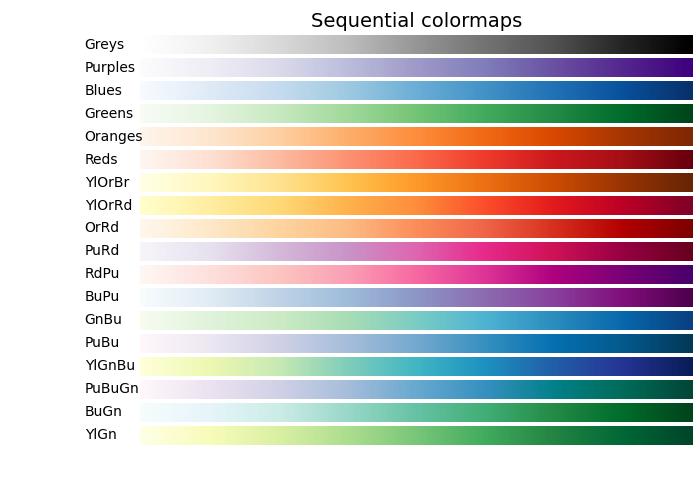
发散型颜色映射
发散型颜色映射的特点是亮度值单调递增然后递减,最大亮度接近中性中点。
示例
以下示例提供了 Matplotlib 中可用的不同发散型颜色映射的渐变的可视化表示。
import matplotlib.pyplot as plt import numpy as np import matplotlib as mpl # List of Diverging colormaps to visualize cmap_list = ['PiYG', 'PRGn', 'BrBG', 'PuOr', 'RdGy', 'RdBu', 'RdYlBu', 'RdYlGn', 'Spectral', 'coolwarm', 'bwr', 'seismic'] # Plot the color gradients gradient = np.linspace(0, 1, 256) gradient = np.vstack((gradient, gradient)) # Create figure and adjust figure height to the number of colormaps nrows = len(cmap_list) figh = 0.35 + 0.15 + (nrows + (nrows - 1) * 0.1) * 0.22 fig, axs = plt.subplots(nrows=nrows + 1, figsize=(7, figh)) fig.subplots_adjust(top=1 - 0.35 / figh, bottom=0.15 / figh, left=0.2, right=0.99) axs[0].set_title('Diverging colormaps', fontsize=14) for ax, name in zip(axs, cmap_list): ax.imshow(gradient, aspect='auto', cmap=mpl.colormaps[name]) ax.text(-0.1, 0.5, name, va='center', ha='left', fontsize=10, transform=ax.transAxes) # Turn off all ticks & spines, not just the ones with colormaps. for ax in axs: ax.set_axis_off() # Show the plot plt.show()
输出
执行上述代码后,我们将获得以下输出 -
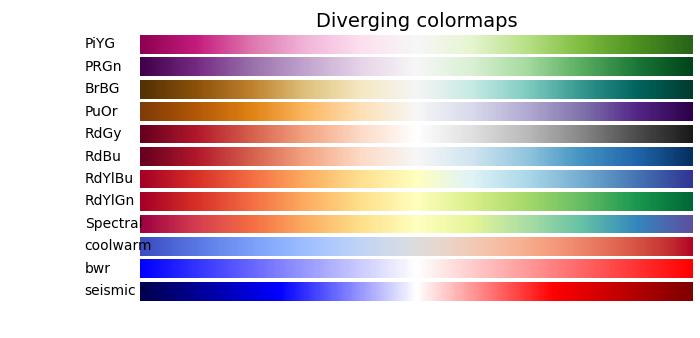
循环型颜色映射
循环型颜色映射呈现出独特的设计,其中颜色映射在同一种颜色上开始和结束,在对称中心点相遇。亮度值的进展应该从开始到中间单调变化,从中间到结束则反向变化。
示例
在以下示例中,您可以浏览和可视化 Matplotlib 中可用的各种循环型颜色映射。
import matplotlib.pyplot as plt import numpy as np import matplotlib as mpl # List of Cyclic colormaps to visualize cmap_list = ['twilight', 'twilight_shifted', 'hsv'] # Plot the color gradients gradient = np.linspace(0, 1, 256) gradient = np.vstack((gradient, gradient)) # Create figure and adjust figure height to the number of colormaps nrows = len(cmap_list) figh = 0.35 + 0.15 + (nrows + (nrows - 1) * 0.1) * 0.22 fig, axs = plt.subplots(nrows=nrows + 1, figsize=(7, figh)) fig.subplots_adjust(top=1 - 0.35 / figh, bottom=0.15 / figh, left=0.2, right=0.99) axs[0].set_title('Cyclic colormaps', fontsize=14) for ax, name in zip(axs, cmap_list): ax.imshow(gradient, aspect='auto', cmap=mpl.colormaps[name]) ax.text(-0.1, 0.5, name, va='center', ha='left', fontsize=10, transform=ax.transAxes) # Turn off all ticks & spines, not just the ones with colormaps. for ax in axs: ax.set_axis_off() # Show the plot plt.show()
输出
执行上述代码后,我们将获得以下输出 -

定性型颜色映射
定性型颜色映射不同于感知映射,因为它们并非旨在提供感知一致性,而是用于表示不具有排序或关系的信息。亮度值在整个颜色映射中差异很大,并且不是单调递增的。因此,不建议将定性型颜色映射用作感知颜色映射。
示例
以下示例提供了 Matplotlib 中可用的不同定性型颜色映射的可视化表示。
import matplotlib.pyplot as plt import numpy as np import matplotlib as mpl # List of Qualitative colormaps to visualize cmap_list = ['Pastel1', 'Pastel2', 'Paired', 'Accent', 'Dark2', 'Set1', 'Set2', 'Set3', 'tab10', 'tab20', 'tab20b', 'tab20c'] # Plot the color gradients gradient = np.linspace(0, 1, 256) gradient = np.vstack((gradient, gradient)) # Create figure and adjust figure height to the number of colormaps nrows = len(cmap_list) figh = 0.35 + 0.15 + (nrows + (nrows - 1) * 0.1) * 0.22 fig, axs = plt.subplots(nrows=nrows + 1, figsize=(7, figh)) fig.subplots_adjust(top=1 - 0.35 / figh, bottom=0.15 / figh, left=0.2, right=0.99) axs[0].set_title('Qualitative colormaps', fontsize=14) for ax, name in zip(axs, cmap_list): ax.imshow(gradient, aspect='auto', cmap=mpl.colormaps[name]) ax.text(-0.1, 0.5, name, va='center', ha='left', fontsize=10, transform=ax.transAxes) # Turn off all ticks & spines, not just the ones with colormaps. for ax in axs: ax.set_axis_off() # Show the plot plt.show()
输出
执行上述代码后,我们将获得以下输出 -
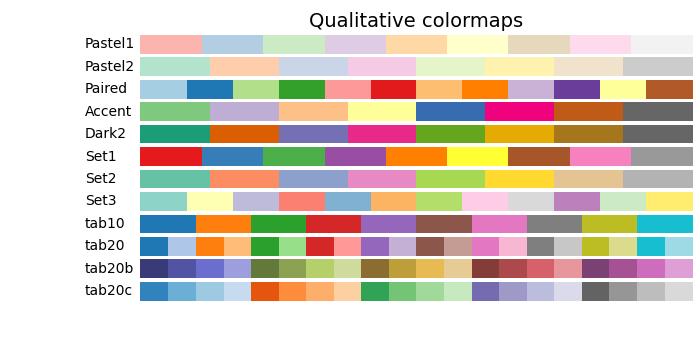