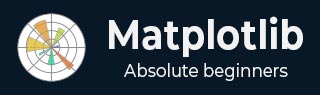
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性与对数比例尺
- Matplotlib - 对称对数与Logit比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是LaTeX?
- Matplotlib - 用于数学表达式的LaTeX
- Matplotlib - 注释中的LaTeX文本格式
- Matplotlib - PostScript
- 启用注释中的LaTex渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用Cycler进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 坐标轴格式化
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 固定位置的绘图元素
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 飘带框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建Logo
- Matplotlib - 多页PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 控件
- Matplotlib - 光标控件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮控件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单控件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块控件
- Matplotlib - 区间选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 矢羽图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 椭圆选择器
简介
Matplotlib 没有直接内置的椭圆选择器控件,但我们可以通过使用 Matplotlib 的事件处理功能实现自定义椭圆选择器来实现类似的功能。
椭圆选择器的概念
椭圆选择器是一个交互式工具,允许用户绘制并选择椭圆区域内的 数据点。此工具在探索数据集或图像并希望关注由椭圆定义的特定感兴趣区域时特别有用。
椭圆选择器的关键特征
以下是椭圆选择器的关键特征。
交互式选择 - 椭圆选择器允许用户在绘图中交互式地定义和修改椭圆区域。这对于选择数据可视化中特定感兴趣的区域非常有价值。
动态更新 - 当用户拖动或调整椭圆大小,所选区域会动态更新。实时反馈增强了用户体验并允许精确选择。
与绘图集成 - 椭圆选择器通常集成到 Matplotlib 绘图中,使用户能够在选定的椭圆内目视检查和交互式地处理数据点。
实现方法
创建椭圆选择器涉及捕获鼠标事件以定义和更新椭圆区域。以下是两种在 Matplotlib 中实现椭圆选择器的潜在方法。
使用 matplotlib.patches.Ellipse
我们可以利用 Matplotlib 中的Ellipse补丁来直观地表示椭圆选择器。其思想是处理鼠标事件以定义椭圆的中心、宽度、高度和旋转。
示例
import matplotlib.pyplot as plt from matplotlib.patches import Ellipse class EllipseSelector: def __init__(self, ax): self.ax = ax self.ellipse = None self.cid_press = ax.figure.canvas.mpl_connect('button_press_event', self.on_press) self.cid_release = ax.figure.canvas.mpl_connect('button_release_event', self.on_release) self.cid_motion = ax.figure.canvas.mpl_connect('motion_notify_event', self.on_motion) def on_press(self, event): if event.inaxes == self.ax: center = (event.xdata, event.ydata) self.ellipse = Ellipse(center, 1, 1, edgecolor='red', alpha=0.5) self.ax.add_patch(self.ellipse) def on_release(self, event): self.ellipse = None def on_motion(self, event): if self.ellipse: width = event.xdata - self.ellipse.center[0] height = event.ydata - self.ellipse.center[1] self.ellipse.width = width self.ellipse.height = height self.ax.figure.canvas.draw() # Create a scatter plot with random data np.random.seed(42) x_data = np.random.rand(50) y_data = np.random.rand(50) fig, ax = plt.subplots() ax.scatter(x_data, y_data) # Initialize the EllipseSelector ellipse_selector = EllipseSelector(ax) plt.show()
输出
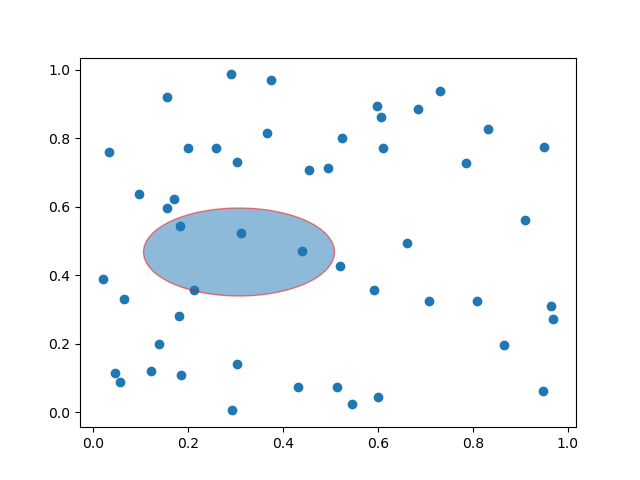
使用 matplotlib.widgets(自定义控件)
另一种方法是使用matplotlib.widgets创建一个自定义控件来封装椭圆选择器的逻辑。这种方法可能提供更模块化的解决方案。
示例
import matplotlib.pyplot as plt from matplotlib.widgets import EllipseSelector import numpy as np # Function to be triggered when the ellipse is selected def on_select(eclick, erelease): print(f"Selected Ellipse: Center={eclick.xdata, eclick.ydata}, Width={abs(erelease.xdata - eclick.xdata)}, Height={abs(erelease.ydata - eclick.ydata)}") # Create a scatter plot with random data np.random.seed(42) x_data = np.random.rand(50) y_data = np.random.rand(50) fig, ax = plt.subplots() ax.scatter(x_data, y_data) # Create Ellipse Selector ellipse_selector = EllipseSelector(ax, on_select, props=dict(facecolor='red', edgecolor='red', alpha=0.5)) plt.show()
输出
Selected Ellipse: Center=(0.17951154118643367, 0.2584893480158592), Width=0.3678226677251435, Height=0.5797088949246063
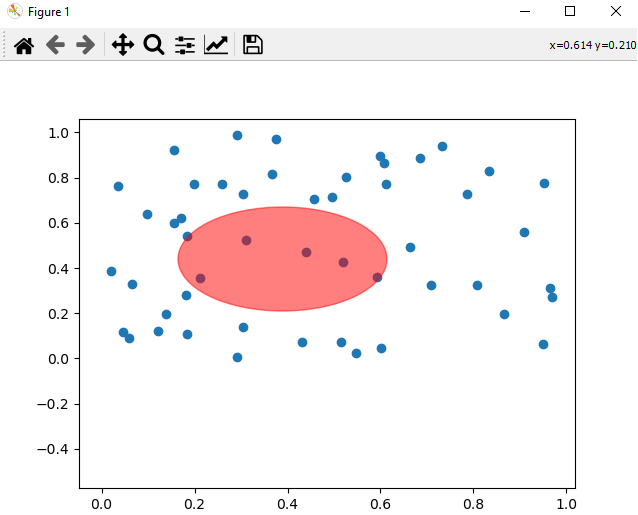
用例和注意事项
以下是椭圆选择器控件的用例和注意事项。
数据区域选择 - 椭圆选择器可用于选择散点图或其他类型的数据可视化中的特定感兴趣区域。
数据分析 - 用户可以使用椭圆选择器在选定的椭圆区域内直观地分析和解释数据点的模式或集群。
与回调函数集成 - 为了增强功能,椭圆选择器可以与回调函数相关联,这些回调函数会响应选择。例如,我们可以根据椭圆内的 数据点执行操作。
视觉探索 - 椭圆选择器的交互性允许用户通过帮助理解复杂数据集来动态地探索和细化他们的选择。
最后,我们可以说,由于 Matplotlib 没有专门的椭圆选择器控件,因此可以使用现有的 Matplotlib 组件创建自定义椭圆选择器。提供的示例演示了实现椭圆选择器的两种潜在方法。通过捕获鼠标事件并相应地更新椭圆的属性,我们可以创建一个交互式工具,用于在 Matplotlib 绘图中选择和探索数据。