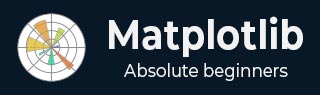
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 色图
- Matplotlib - 色图归一化
- Matplotlib - 选择色图
- Matplotlib - 色标
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像遮罩
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性与对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - LaTeX 在注释中的文本格式化
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图形对象
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 转换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - subplots() 函数
- Matplotlib - subplot2grid() 函数
- Matplotlib - 固定图形对象
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建徽标
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 图像遮罩
在 Matplotlib 库中,图像遮罩涉及根据指定的遮罩选择性地显示图像的某些部分,遮罩本质上是一个二值图像,用于定义要显示或隐藏的区域。它允许我们应用过滤器或条件来显示或隐藏图像的特定部分。
图像遮罩的过程
以下是执行图像遮罩的过程步骤。
创建遮罩
定义创建遮罩的标准或模式。它可以基于颜色、形状、渐变或特定的像素值。
应用遮罩
使用遮罩修改原始图像中相应像素的透明度或可见性。与遮罩中定义为“遮罩”区域相对应的像素通常会被隐藏或变得透明,而其他像素则保持可见。
叠加或混合
将遮罩图像与另一幅图像或背景叠加或混合,仅显示未遮罩的部分。可以将遮罩图像叠加在另一幅图像上,以组合和显示可见区域。
图像遮罩的类型
以下是图像遮罩的类型。
二值遮罩
由黑色和白色像素组成,其中白色表示可见区域,黑色表示遮罩区域。
灰度遮罩
使用各种灰度来定义透明度或部分可见性的级别。
图像遮罩的工具和技术
我们可以使用不同的工具和技术进行图像遮罩。
手动遮罩
Photoshop 或 GIMP 等工具允许用户使用选择工具、画笔或图层遮罩手动创建和编辑遮罩。
编程遮罩
在 Python 等编程语言中,使用 OpenCV 或 PIL (Pillow) 等库,我们可以使用基于颜色阈值、轮廓或特定图像特征的算法创建遮罩。
关键点
Matplotlib 中的图像遮罩涉及创建与图像具有相同维度的遮罩数组,其中标记特定区域以隐藏(遮罩)或显示(未遮罩)图像的部分。
遮罩数组由布尔值或数值组成,其中**True** 或非零值表示要显示的区域,而**False** 或零值表示要隐藏的区域。
遮罩允许根据指定的条件或标准选择性地可视化或操作图像的特定部分。
遮罩图像的特定区域
假设我们有一幅图像,并希望遮罩某个特定区域,仅根据某些标准显示图像的一部分。
示例
import matplotlib.pyplot as plt import numpy as np # Create a sample image (random pixels) image = np.random.rand(100, 100) # Create a mask to hide certain parts of the image mask = np.zeros_like(image) mask[30:70, 30:70] = 1 # Masking a square region # Apply the mask to the image masked_image = np.ma.masked_array(image, mask=mask) # Display the original and masked images plt.figure(figsize=(8, 4)) plt.subplot(1, 2, 1) plt.imshow(image, cmap='gray') plt.title('Original Image') plt.axis('off') plt.subplot(1, 2, 2) plt.imshow(masked_image, cmap='gray') plt.title('Masked Image') plt.axis('off') plt.show()
输出
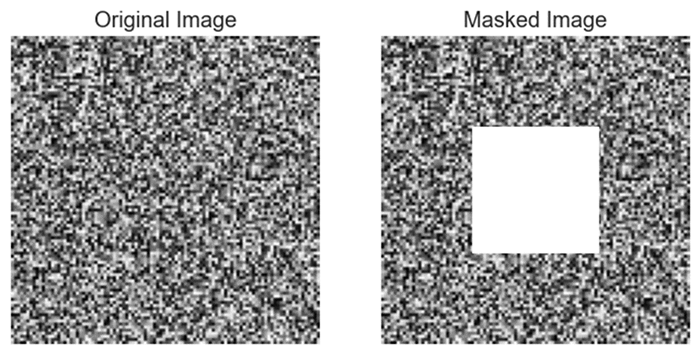
将遮罩应用于图像
这里这是另一个使用 matplotlib 库遮罩图像的示例。
示例
import matplotlib.pyplot as plt import numpy as np # Create a sample image image_size = 100 img = np.zeros((image_size, image_size, 3), dtype=np.uint8) img[:, :image_size // 2] = [255, 0, 0] # Set the left half to blue # Create a binary mask mask = np.zeros((image_size, image_size), dtype=np.uint8) mask[:, image_size // 4:] = 1 # Set the right quarter to 1 # Apply the mask to the image masked_img = img * mask[:, :, np.newaxis] # Display the original image, mask, and the masked image plt.figure(figsize=(12, 4)) plt.subplot(131) plt.imshow(img) plt.title('Original Image') plt.axis('off') plt.subplot(132) plt.imshow(mask, cmap='gray') plt.title('Mask') plt.axis('off') plt.subplot(133) plt.imshow(masked_img) plt.title('Masked Image') plt.axis('off') plt.show()
输出
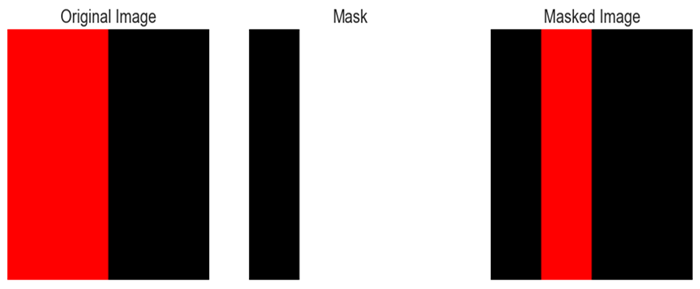
广告