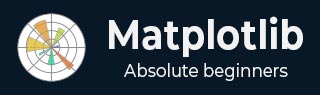
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性刻度和对数刻度
- Matplotlib - 对称对数刻度和logit刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是LaTeX?
- Matplotlib - 用于数学表达式的LaTeX
- Matplotlib - 注释中的LaTeX文本格式
- Matplotlib - PostScript
- 启用注释中的LaTeX渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用Cycler进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - subplots() 函数
- Matplotlib - subplot2grid() 函数
- Matplotlib - 固定位置的绘图元素
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 缎带框
- Matplotlib - 填充螺旋线
- Matplotlib - findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建Logo
- Matplotlib - 多页PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 游标小部件
- Matplotlib - 带注释的游标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 矢羽图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 图例
一般来说,图表中的图例提供对沿 Y 轴(通常称为图表序列)描绘的数据的视觉表示。它是一个包含符号和图表中每个序列标签的框。序列可以是折线图中的线、条形图中的条等等。当您在同一图表上有多个数据序列并且想要区分它们时,图例非常有用。在下图中,我们可以观察到图表中的图例(用红色矩形突出显示)−
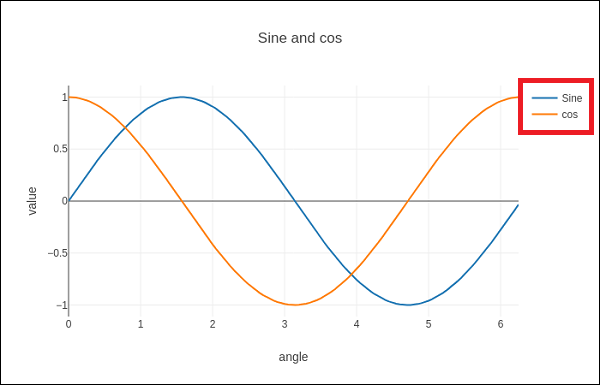
向 matplotlib 图表添加图例
要向 Matplotlib 图表添加图例,通常使用 matplotlib.pyplot.legend() 函数。此函数用于向 Axes 添加图例,为图表中的元素提供视觉指南。
语法
以下是函数的语法
matplotlib.pyplot.legend(*args, **kwargs)
可以以不同的方式调用该函数,具体取决于您希望如何自定义图例。
在调用legend()时不传递任何额外参数,Matplotlib 会自动确定要添加到图例的元素。这些元素的标签取自图表中的绘图元素。您可以在创建绘图元素时指定这些标签,也可以使用set_label()方法指定。
示例 1
在此示例中,图例取自创建绘图时使用的 label 参数中的数据。
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y = [2, 4, 6] # Plotting the data with labels line, = plt.plot(x, y, label='Legend describing a single line') # Adding a legend plt.legend() # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
输出
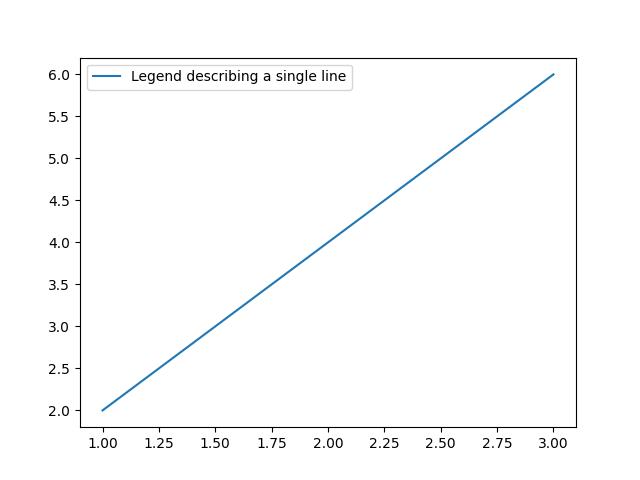
Successfully Placed a legend on the Axes...
示例 2
这是使用绘图元素上的 set_label() 方法的另一种方法。
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y = [2, 4, 6] # Plotting the data with labels line, = plt.plot(x, y) line.set_label('Place a legend via method') # Adding a legend plt.legend() # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
输出
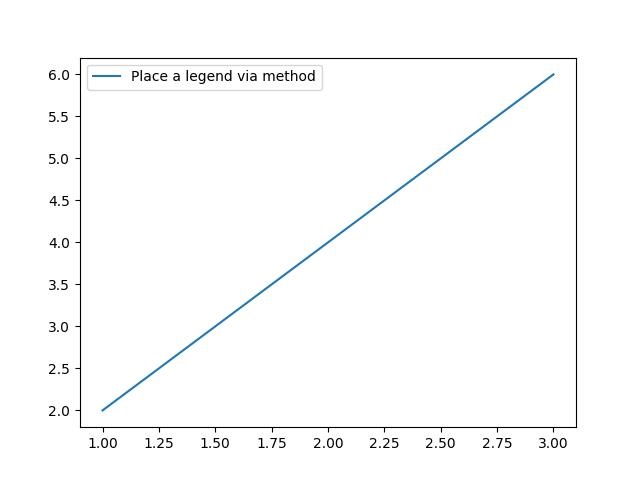
Successfully Placed a legend on the Axes...
手动添加图例
您可以传递图例绘图元素的迭代器,然后传递图例标签的迭代器,以明确控制哪些绘图元素具有图例条目。
示例 1
这是一个通过列出绘图元素和标签来调用 legend() 函数的示例。
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y1 = [2, 4, 6] y2 = [1, 3, 5] y3 = [3, 6, 9] # Plotting the data line1, = plt.plot(x, y1) line2, = plt.plot(x, y2) line3, = plt.plot(x, y3) # calling legend with explicitly listed artists and labels plt.legend([line1, line2, line3], ['Label 1', 'Label 2', 'Label 3']) # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
输出
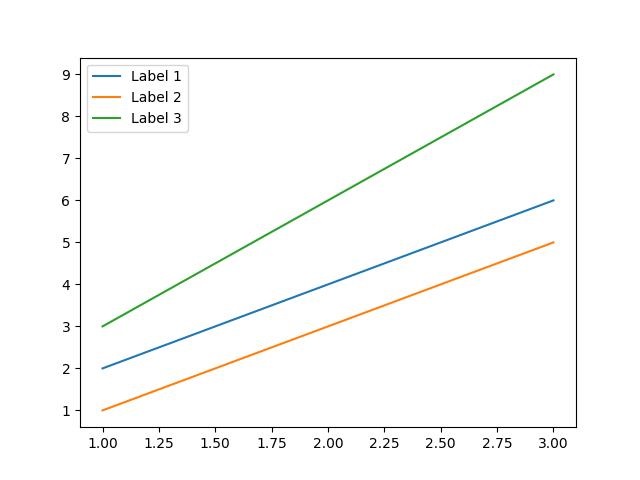
Successfully Placed a legend on the Axes...
示例 2
这是一个仅通过列出绘图元素来调用legend()函数的示例。这种方法与前面一种方法类似,但在这种情况下,标签取自绘图元素的标签属性。
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y1 = [2, 4, 6] y2 = [1, 3, 5] # Plotting the data with labels line1, = plt.plot(x, y1, label='Label 1') line2, = plt.plot(x, y2, label='Label 2') # Adding a legend with explicitly listed artists plt.legend(handles=[line1, line2]) # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
输出
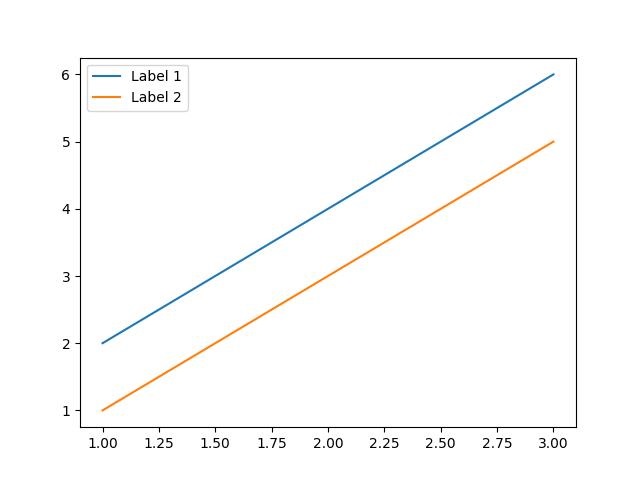
Successfully Placed a legend on the Axes...
示例 3
在此示例中,我们首先绘制两组数据,然后通过使用代表图例条目的字符串列表调用legend()函数来添加图例。
import matplotlib.pyplot as plt # Example data x = [1, 2, 3, 4, 5, 6] y1 = [1, 4, 2, 6, 8, 5] y2 = [1, 5, 3, 7, 9, 6] # Plotting the data plt.plot(x, y1) plt.plot(x, y2) # Adding a legend for existing plot elements plt.legend(['First line', 'Second line'], loc='center') # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
输出
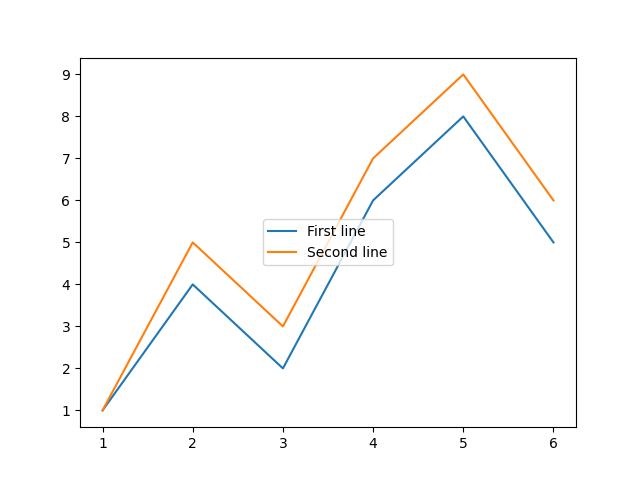
Successfully Placed a legend on the Axes...
在子图中添加图例
要在每个子图中添加图例,我们可以在图的每个 axes 对象上使用legend()函数。
示例 1
这是一个在 matplotlib 图的每个子图中添加图例的示例。此方法在 axes 对象上使用 legend() 函数。
import numpy as np from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7, 3.50] plt.rcParams["figure.autolayout"] = True # Sample data x = np.linspace(-2, 2, 100) y1 = np.sin(x) y2 = np.cos(x) y3 = np.tan(x) # Create the figure with subplots f, axes = plt.subplots(3) # plot the data on each subplot and add lagend axes[0].plot(x, y1, c='r', label="sine") axes[0].legend(loc='upper left') axes[1].plot(x, y2, c='g', label="cosine") axes[1].legend(loc='upper left') axes[2].plot(x, y3, c='b', label="tan") axes[2].legend(loc='upper left') # Display the figure plt.show()
输出
执行上述代码后,我们将得到以下输出 −
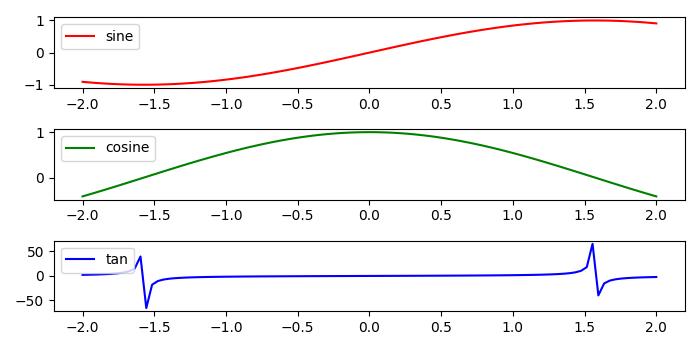
在一个 axes 中添加多个图例
要在 Matplotlib 中在同一个 axes 上绘制多个图例,我们可以使用axes.add_artist()方法以及 legend() 函数。
示例 2
以下示例演示如何在 matplotlib 图的一个 axes 中添加多个图例。
from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7, 4] plt.rcParams["figure.autolayout"] = True # plot some data line1, = plt.plot([1, 2, 3], label="Line 1", linestyle='--') line2, = plt.plot([3, 2, 1], label="Line 2", linewidth=4) # Add first legend at upper right of the axes first_legend = plt.legend(handles=[line1], loc='upper right') # Get the current axes to add legend plt.gca().add_artist(first_legend) # Add second legend at lower right of the axes plt.legend(handles=[line2], loc='lower right') # Display the output plt.show()
输出
执行上述代码后,我们将得到以下输出 −
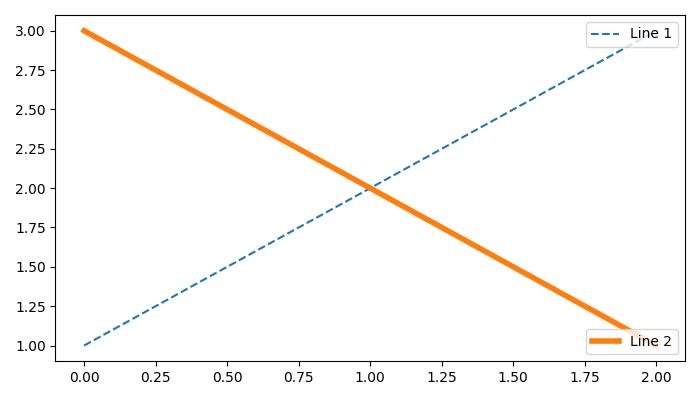