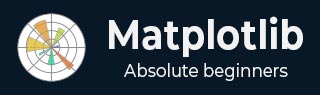
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 色标
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性和对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - LaTeX 在注释中的文本格式化
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 艺术家
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定艺术家
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多处理
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - 底图
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 羽毛图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 多光标
简介
Matplotlib 没有专门的多光标小部件。但是,Matplotlib 提供了光标小部件,可用于向绘图添加光标,以显示特定位置的信息。此外,matplotlib.widgets 模块提供了一个名为MultiCursor 的工具,允许我们在单个绘图中使用多个光标线。这对于比较 x 轴上不同点的值很有用。
让我们探讨如何在 Matplotlib 中使用 MultiCursor 工具以及光标小部件,并讨论其功能、实现和潜在用例。
MultiCursor 的功能
以下是 Multicursor 小部件的功能。
多个光标 - Matplotlib 中的MultiCursor 工具允许我们在单个绘图中添加多个光标线。每个光标线对应一组特定的轴。
光标之间的协调 - 光标是链接的,即当与其中一个交互时,它们会一起移动。这有助于轻松比较不同轴上的数据点。
可自定义的外观 - 可以根据我们的偏好自定义光标的外观,例如线条颜色和线型。
动态数据显示 - 当我们沿着 x 轴移动光标时,通过提供实时信息,动态显示光标位置处的相应 y 值。
基本光标示例
这是一个演示光标类用法的基本示例。
示例
import matplotlib.pyplot as plt from matplotlib.widgets import Cursor import numpy as np # Generating sample data x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a plot fig, ax = plt.subplots() line, = ax.plot(x, y, label='Sine Wave') # Adding a cursor to the plot cursor = Cursor(ax, horizOn=True, vertOn=True, useblit=True, color='red', linewidth=1) # Displaying the plot plt.show()
输出
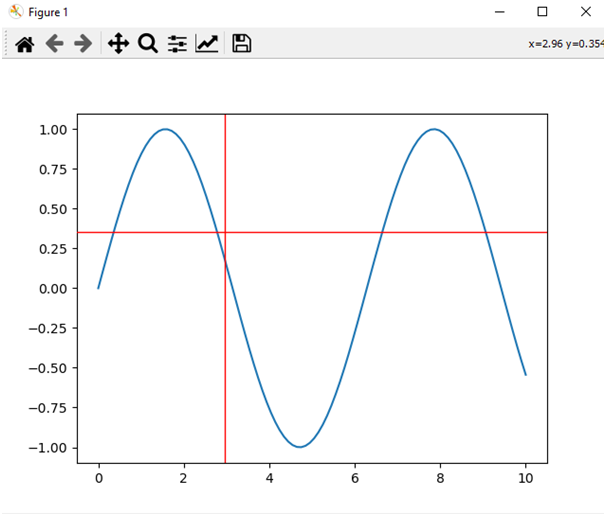
使用 mplcursors 实现多光标
要在 Matplotlib 中实现多光标,我们可以使用mplcursors 包,它提供了额外的光标功能。我们可以使用以下代码行安装mplcursors 包。
示例
pip install mplcursors
输出
Collecting mplcursors Downloading mplcursors-0.5.3.tar.gz (88 kB) -------------------------------------- 88.8/88.8 kB 557.3 kB/s eta 0:00:00 Installing build dependencies: started Installing build dependencies: finished with status 'done' Getting requirements to build wheel: started Getting requirements to build wheel: finished with status 'done' Installing backend dependencies: started Installing backend dependencies: finished with status 'done' Preparing metadata (pyproject.toml): started Preparing metadata (pyproject.toml): finished with status 'done'
mplcursors 的用法
现在让我们探索一个使用mplcursors 在 Matplotlib 中创建多光标的示例。
示例
import matplotlib.pyplot as plt import mplcursors import numpy as np # Generate sample data x = np.linspace(0, 10, 100) y1 = np.sin(x) y2 = np.cos(x) # Create subplots fig, (ax1, ax2) = plt.subplots(2, 1, sharex=True, figsize=(8, 6)) # Plot data on the first subplot ax1.plot(x, y1, label='Sin(x)') ax1.set_ylabel('Amplitude') ax1.legend() # Plot data on the second subplot ax2.plot(x, y2, label='Cos(x)') ax2.set_xlabel('x') ax2.set_ylabel('Amplitude') ax2.legend() # Enable multicursor on both subplots mplcursors.cursor(hover=True, multiple=True) # Display the plot plt.show()
输出
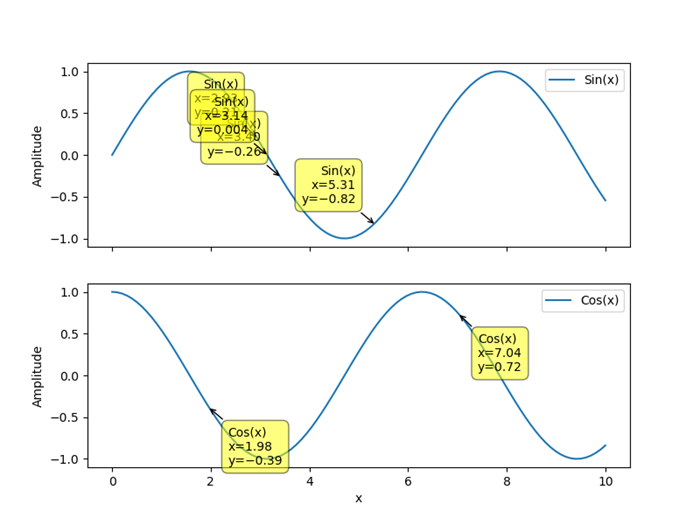
扩展到多光标
要将此概念扩展到真正多光标的场景(其中多个光标独立移动),我们需要为每条线或数据源创建光标类的单独实例,并相应地更新信息。
多光标的用例
以下是多光标小部件使用用例。
比较分析 - 多光标在比较不同绘图之间的对应数据点时非常有用,这有助于可视化关系。
时间序列探索 - 对于时间序列数据,多光标允许同时检查多个时间序列中特定时间点处的数值。
交互式数据探索 - 多光标通过允许用户探索不同维度的数据点来增强数据可视化的交互性。
相关性分析 - 在研究两个或多个变量之间的相关性时,多光标有助于识别模式和相关性。