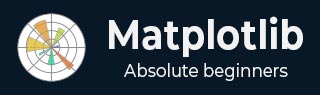
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色图
- Matplotlib - 颜色图归一化
- Matplotlib - 选择颜色图
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性刻度和对数刻度
- Matplotlib - 对称对数刻度和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 启用注释中的 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 艺术家
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化程序
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定艺术家
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建徽标
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - 带 EEG 的 MRI
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 计时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 区间选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 艺术家
理解 Matplotlib 中的艺术家
在 Matplotlib 中,你几乎在绘图上看到的任何东西都是艺术家的一个实例,它们是表示绘图各个组件的对象。无论是表示数据的线,文本标签,甚至是轴上的刻度标记,Matplotlib 绘图中的所有内容都是一个艺术家对象。
在探索艺术家层次结构之前,让我们观察下面的图像以了解 matplotlib 中图形的基本组件:
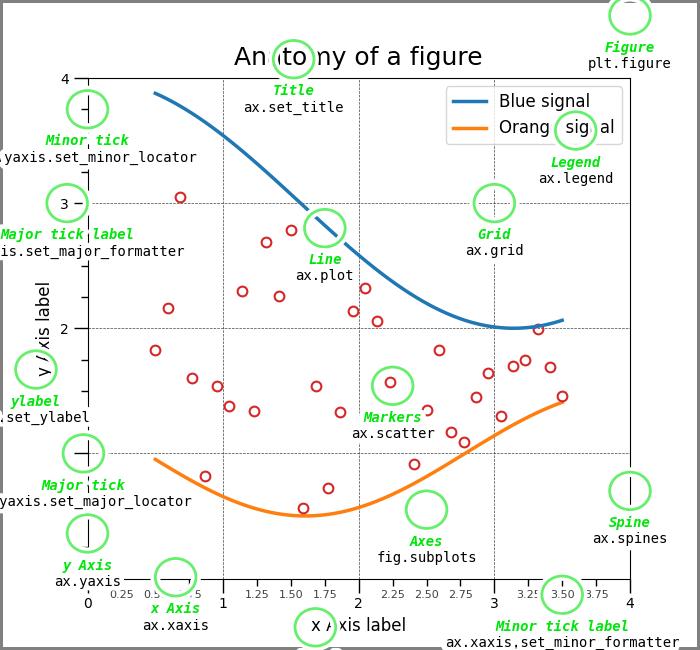
在图中,每个元素,例如表示数据的线和点,以及与文本标签相关的次要刻度和点,都可以识别为单独的艺术家对象。
艺术家层次结构
在 matplotlib 的层次结构中,艺术家可以大致分为两种类型:
- 基本艺术家 - 这些是绘图的基本构建块。示例包括Line2D、Rectangle、Text、AxesImage等等。这些是我们想要绘制到画布上的标准图形对象。
- 复合艺术家 - 这些是包含其他艺术家的更高级别结构。示例包括Figure、Axis和Axes。Figure 就像一个包含所有内容的画布,而 Axes 表示图形内的特定绘图。
创建和访问艺术家
艺术家通常是通过 Axes 对象上的绘图方法创建的。这些方法不仅促进了可视元素的创建,还返回与已绘制元素对应的特定艺术家实例。
以下是一些常见的绘图方法以及它们生成的相应艺术家:
- annotate - 为文本注释生成Annotation艺术家。
- bar - 为条形图创建Rectangle艺术家。
- errorbar - 为误差条形图生成Line2D和Rectangle艺术家。
- fill - 为共享区域生成Polygon艺术家。
- hist - 为直方图创建Rectangle艺术家。
- imshow - 为图像数据生成AxesImage艺术家。
- legend - 为 Axes 图例生成Legend艺术家。
- plot - 为 XY 图创建Line2D艺术家。绘制多个数据集时返回列表。
- scatter - 为散点图生成PolyCollection艺术家。
- text - 生成Text艺术家以在绘图上显示文本。
示例
让我们来看一个使用plot()方法创建和访问艺术家的示例。
import matplotlib.pyplot as plt import matplotlib.artist as martist import numpy as np # Create a subplot fig, ax = plt.subplots() # Generate random data x, y = np.random.rand(2, 100) # Use the plot method to create a Line2D artist lines = ax.plot(x, y, '-', label='example') # Accessing the Line2D artists created by the plot method print('Line2D artists created by the plot method:', lines) # Retrieve all Line2D artists associated with the Axes lines_on_axes = ax.get_lines() # Display the retrieved Line2D artists print('Line2D artists obtained using get_lines():', lines_on_axes) # Accessing the first (and in this case, the only) Line2D artist print('Accessing the first Line2D artist', ax.get_lines()[0])
输出
执行上述代码后,我们将得到以下输出:
Line2D artists created by the plot method: [<matplotlib.lines.Line2D object at 0x000001DB3666A620>] Line2D artists obtained using get_lines(): Accessing the first Line2D artist Line2D(example)
修改艺术家属性
艺术家具有各种定义其外观和行为的属性。这些属性包括颜色、线型、线宽和标记。修改这些属性使我们能够控制绘图的视觉方面。
示例
以下示例演示如何创建简单的绘图,然后修改关联的 Line2D 艺术家的属性。
import matplotlib.pyplot as plt import numpy as np # Creating a simple plot fig, ax = plt.subplots(figsize=(7, 4)) x = np.linspace(0, 2 * np.pi, 100) y = np.sin(x) # Use the plot method to create a Line2D artist lines = ax.plot(x, y) # Displaying initial properties of the Line2D artist print('Initial properties of the Line2D artist:') print('Color:', ax.get_lines()[0].get_color()) print('Line Style:', ax.get_lines()[0].get_linestyle()) print('Marker Size:', ax.get_lines()[0].get_markersize()) # Modifying properties lines[0].set(color='green', linewidth=2) lines[0].set_linestyle(':') lines[0].set_marker('s') lines[0].set_markersize(10) # Accessing the modified properties of the Line2D artist print('\nModified properties of the Line2D artist:') print('Color:', ax.get_lines()[0].get_color()) print('Line Style:', ax.get_lines()[0].get_linestyle()) print('Marker Size:', ax.get_lines()[0].get_markersize())
输出
执行上述代码后,我们将得到以下输出:
Initial properties of the Line2D artist: Color: #1f77b4 Line Style: - Marker Size: 6.0 Modified properties of the Line2D artist: Color: green Line Style: : Marker Size: 10.0
操作艺术家数据
除了样式属性之外,艺术家还可以包含数据。例如,Line2D 对象具有可以使用set_data()方法修改的数据属性。
示例
这是一个操作艺术家数据的示例。
import matplotlib.pyplot as plt import numpy as np # Creating a simple plot fig, ax = plt.subplots(figsize=(7, 4)) x = np.linspace(0, 2 * np.pi, 100) # Initial sinusoidal curve y = np.sin(x) # Use the plot method to create a Line2D artist a sinusoidal curve lines = ax.plot(x, y) # Modifying the artist data with the cosine lines[0].set_data([x, np.cos(x)]) # Displaying the plot plt.show()
输出
执行上述代码后,我们将得到以下输出:
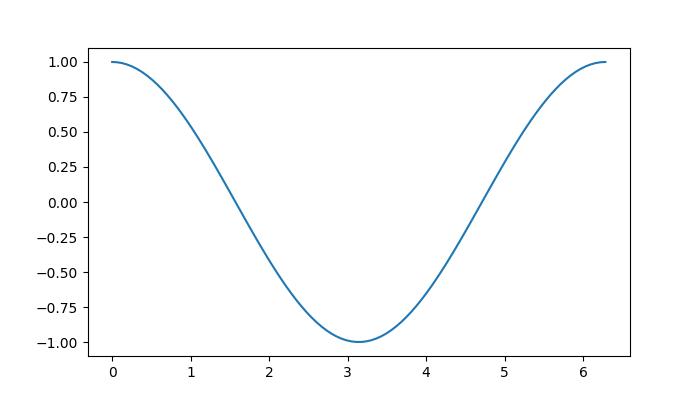
手动添加艺术家
虽然许多艺术家都有辅助方法,但在某些情况下需要手动添加。例如,可以通过使用add_artist()方法将圆形或矩形等补丁直接添加到 Axes 对象。
示例
在这个例子中,我们手动将圆形补丁添加到轴。
import matplotlib.pyplot as plt import numpy as np import matplotlib.patches as mpatches # Creating a simple plot fig, ax = plt.subplots(figsize=(7, 4)) x = np.linspace(0, 2 * np.pi, 100) # Initial sinusoidal curve y = np.sin(x) # Use the plot method to create a Line2D artist for a sinusoidal curve lines = ax.plot(x, y) # Adding a circle circle = mpatches.Circle((3, 0), 0.25, ec="none") ax.add_artist(circle) # Adding another clipped circle clipped_circle = mpatches.Circle((3, 1), 0.125, ec="none", facecolor='C1') ax.add_artist(clipped_circle) ax.set_aspect(1) # Adding a title to the plot plt.title('Manual Addition of Artists') # Displaying the plot plt.show()
输出
执行上述代码后,我们将得到以下输出:
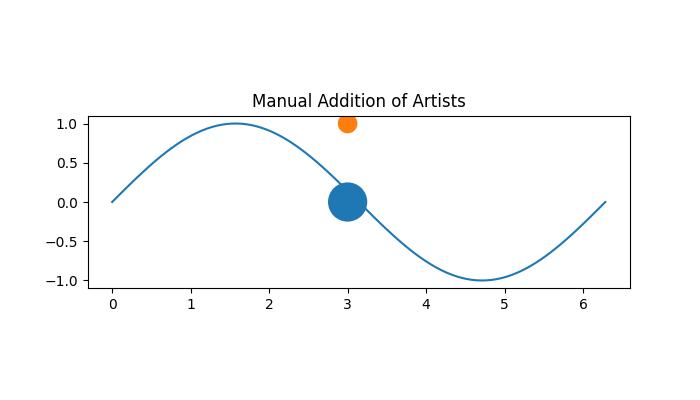
移除艺术家
Matplotlib 提供了一种灵活的方法来从绘图中移除特定的艺术家。艺术家的remove()方法允许从其 Axes 列表中移除艺术家。
示例
这是一个演示如何从绘图中移除特定艺术家(Line2D 和文本)的示例。
import numpy as np import matplotlib.pyplot as plt # Create the figure fig, ax = plt.subplots(figsize=(7, 4)) x = np.linspace(-10, 10, 100) y = np.sin(x) # plot the scatter plot scat = ax.scatter(x, y) # Assign the another Line2D artist to line_2 line_2 = plt.plot(x, np.cos(x), linestyle='dashed') plt.margins(0.2) plt.title("After removing the text and line artist") text = fig.text(0.5, 0.96, "$y=sin(x)$") # Remove the line_2 artist l = line_2.pop(0) l.remove() # Remove the text artist text.remove() # Displaying the plot plt.show()
输出
执行上述代码后,你将得到以下输出:
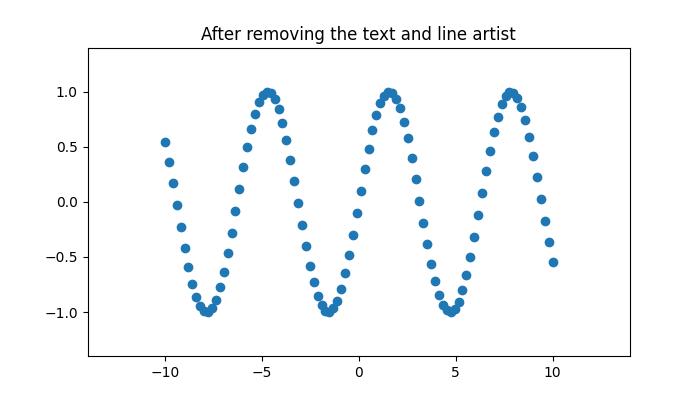