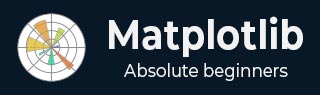
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色映射
- Matplotlib - 颜色映射归一化
- Matplotlib - 选择颜色映射
- Matplotlib - 色标
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性和对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - LaTeX 在注释中的文本格式化
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图形对象
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 转换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带有单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定图形对象
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 游标小部件
- Matplotlib - 带注释的游标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多游标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 图像缩略图
缩略图是原始图像的较小且压缩版本,是各种应用程序中必不可少的组件,包括图像预览、Web 开发以及优化包含大量图像的网页和应用程序的加载速度。
在本教程中,我们将探讨如何使用 Matplotlib 有效地生成图像缩略图。Matplotlib 利用Python Pillow 库进行图像处理,并允许我们轻松地从现有图像生成缩略图。
Matplotlib 中的图像缩略图
Matplotlib 在其图像模块中提供了thumbnail()函数来生成具有可自定义参数的缩略图,允许用户有效地为各种目的创建缩小版的图像。
以下是函数的语法:
语法
matplotlib.image.thumbnail(infile, thumbfile, scale=0.1, interpolation='bilinear', preview=False)
以下是其参数的详细信息:
infile - 输入图像文件。如您所知,Matplotlib 依赖 Pillow 读取图像,因此它支持各种文件格式,包括 PNG、JPG、TIFF 等。
thumbfile - 将保存缩略图的文件名或类文件对象。
scale - 缩略图的缩放因子。它确定缩略图相对于原始图像的大小缩减。较小的缩放因子会生成较小的缩略图。
interpolation - 重采样过程中使用的插值方案。此参数指定用于估计缩略图中像素值的方法。
preview - 如果设置为 True,则将使用默认后端(可能是用户界面后端),如果调用 show(),则可能会弹出一个图形。如果设置为 False,则使用 FigureCanvasBase 创建图形,并且选择 Figure.savefig 通常会选择的绘图后端。
该函数返回包含缩略图的 Figure 实例。此 Figure 对象可以根据需要进一步操作或保存。
为单个图像生成缩略图
Matplotlib 支持 png、pdf、ps、eps svg 等各种图像格式,使其适用于不同的用例。
示例
这是一个为单个 .jpg 图像创建缩略图的示例。
import matplotlib.pyplot as plt import matplotlib.image as mpimg input_image_path = "Images/Tajmahal.jpg" output_thumbnail_path = "Images/Tajmahal_thumbnail.jpg" # Load the original image img = mpimg.imread(input_image_path) # Create a thumbnail using Matplotlib thumb = mpimg.thumbnail(input_image_path, output_thumbnail_path, scale=0.15) print(f"Thumbnail generated for {input_image_path}. Saved to {output_thumbnail_path}")
输出
如果您访问保存图像的文件夹,您可以观察到原始图像和输出缩略图图像,如下所示:
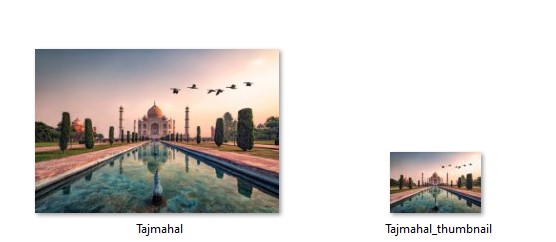
为多个图像生成缩略图
考虑这样一个场景:您在一个目录中有多个图像,并且您希望为每个图像生成缩略图。
示例
这是一个在目录中创建多个 PNG 图像的缩略图的示例。将以下脚本另存为 generate_thumbnails.py 文件。
from argparse import ArgumentParser from pathlib import Path import sys import matplotlib.image as image parser = ArgumentParser(description="Generate thumbnails of PNG images in a directory.") parser.add_argument("imagedir", type=Path) args = parser.parse_args() if not args.imagedir.is_dir(): sys.exit(f"Could not find the input directory {args.imagedir}") outdir = Path("thumbs") outdir.mkdir(parents=True, exist_ok=True) for path in args.imagedir.glob("*.png"): outpath = outdir / path.name try: fig = image.thumbnail(path, outpath, scale=0.15) print(f"Saved thumbnail of {path} to {outpath}") except Exception as e: print(f"Error generating thumbnail for {path}: {e}")
然后在命令提示符中按如下方式运行脚本
python generate_thumbnails.py /path/to/all_images
输出
执行上述程序后,将创建一个名为“thumbs”的目录,并为工作目录中的 PNG 图像生成缩略图。如果遇到格式不同的图像,它将打印错误消息并继续处理其他图像。
Saved thumbnail of Images\3d_Star.png to thumbs\3d_Star.png Saved thumbnail of Images\balloons_noisy.png to thumbs\balloons_noisy.png Saved thumbnail of Images\binary image.png to thumbs\binary image.png Saved thumbnail of Images\black and white.png to thumbs\black and white.png Saved thumbnail of Images\Black1.png to thumbs\Black1.png Saved thumbnail of Images\Blank.png to thumbs\Blank.png Saved thumbnail of Images\Blank_img.png to thumbs\Blank_img.png Saved thumbnail of Images\Circle1.png to thumbs\Circle1.png Saved thumbnail of Images\ColorDots.png to thumbs\ColorDots.png Saved thumbnail of Images\colorful-shapes.png to thumbs\colorful-shapes.png Saved thumbnail of Images\dark_img1.png to thumbs\dark_img1.png Saved thumbnail of Images\dark_img2.png to thumbs\dark_img2.png Saved thumbnail of Images\decore.png to thumbs\decore.png Saved thumbnail of Images\deform.png to thumbs\deform.png Saved thumbnail of Images\Different shapes.png to thumbs\Different shapes.png Error generating thumbnail for Images\Different shapes_1.png: not a PNG file Saved thumbnail of Images\Ellipses.png to thumbs\Ellipses.png Error generating thumbnail for Images\Ellipses_and_circles.png: not a PNG file Saved thumbnail of Images\images (1).png to thumbs\images (1).png Saved thumbnail of Images\images (3).png to thumbs\images (3).png Saved thumbnail of Images\images.png to thumbs\images.png Saved thumbnail of Images\image___1.png to thumbs\image___1.png Saved thumbnail of Images\Lenna.png to thumbs\Lenna.png Saved thumbnail of Images\logo-footer-b.png to thumbs\logo-footer-b.png Saved thumbnail of Images\logo-w.png to thumbs\logo-w.png Saved thumbnail of Images\logo.png to thumbs\logo.png Saved thumbnail of Images\logo_Black.png to thumbs\logo_Black.png