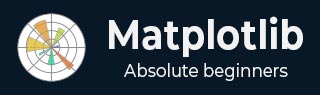
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 色图
- Matplotlib - 色图归一化
- Matplotlib - 选择色图
- Matplotlib - 色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性和对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - LaTeX 在注释中的文本格式化
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图形对象
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转轴
- Matplotlib - 对数轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 轴范围
- Matplotlib - 轴刻度
- Matplotlib - 轴刻度
- Matplotlib - 格式化轴
- Matplotlib - Axes 类
- Matplotlib - 双轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 锚定图形对象
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建徽标
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 放大镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - 矢羽图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 路径效果
路径效果指的是在计算机图形学中操纵路径(路线)的方式。 “路径”是指使用绘图工具创建的线条或形状,而“路径效果”允许您对该线条或形状应用各种修改。
想象一下,您绘制了一条简单的线条,使用路径效果,您可以使该线条看起来波浪形、点状或应用其他视觉变化,而无需手动重新绘制。这就像为创建的路径添加特殊效果,使您的绘图更有趣和动态。
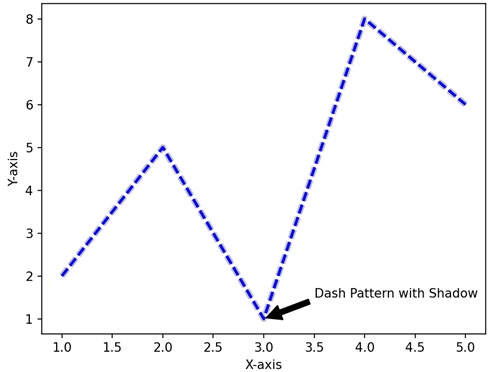
Matplotlib 中的路径效果
您可以使用 matplotlib 中的“path_effects”模块通过创建路径效果来增强绘图的视觉表示。 首先,请考虑“path_effects”模块中的“withStroke()”函数。此函数允许您为线条和标记添加笔触或轮廓。
Matplotlib 中的路径效果允许您通过对绘图中的线条和形状应用特殊的视觉效果来增强其外观。
带有阴影路径效果的简单线条
在 Matplotlib 中,创建带有阴影的简单线条涉及在绘图上绘制基本线条,然后使用阴影效果对其进行增强以使其在视觉上更有趣。此效果使线条下方出现阴影,就像在绘图表面上 tạo ra một bóng mờ tinh tế一样。
示例
在下面的示例中,我们绘制了一条波浪线,然后使用 path_effects 模块向线条添加阴影效果。阴影效果由“灰色”笔触组成,在线条后面 tạo ra vẻ ngoài của một cái bóng。
import matplotlib.pyplot as plt import matplotlib.patheffects as path_effects import numpy as np # Generating data x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a simple line plot fig, ax = plt.subplots() line, = ax.plot(x, y, label='Simple Line') # Adding a shadow effect to the line shadow_effect = path_effects.withStroke(linewidth=5, foreground='gray') line.set_path_effects([shadow_effect]) ax.set_title('Simple Line with Shadow Effect') plt.legend() plt.show()
输出
以下是上述代码的输出 -
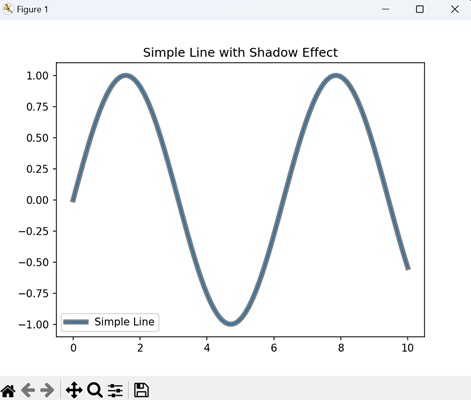
带有轮廓路径效果的虚线
在 Matplotlib 中创建带有轮廓路径效果的虚线涉及在绘图上绘制点状线条,然后通过添加粗轮廓对其进行增强。要创建轮廓,您可以使用较粗的线宽和实线样式在虚线上方绘制另一条线。
示例
在这里,我们正在创建一条虚线并使用“黑色”笔触轮廓对其进行增强,以使线条具有粗体边框并使其脱颖而出。
import matplotlib.pyplot as plt import matplotlib.patheffects as path_effects import numpy as np # Generating data x = np.linspace(0, 10, 100) y = np.cos(x) # Creating a dashed line plot with outline fig, ax = plt.subplots() line, = ax.plot(x, y, linestyle='dashed', label='Dashed Line') # Adding an outline effect to the line outline_effect = path_effects.withStroke(linewidth=3, foreground='black') line.set_path_effects([outline_effect]) ax.set_title('Dashed Line with Outline Effect') plt.legend() plt.show()
输出
执行上述代码后,我们将获得以下输出 -
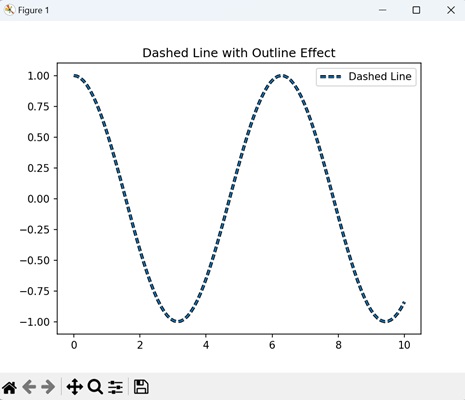
粗体轮廓散点图路径效果
在 Matplotlib 中使用路径效果创建粗体轮廓散点图涉及在图形上绘制一组点,并通过添加粗轮廓来增强每个点。
示例
在下面的示例中,我们使用随机数据点生成散点图。为了增强这些点的可见性,我们通过在每个散点周围添加“黑色”笔触和增加的“线宽”来为每个点应用粗体轮廓效果。
import matplotlib.pyplot as plt import matplotlib.patheffects as path_effects import numpy as np # Generating data x = np.random.rand(50) y = np.random.rand(50) # Creating a scatter plot with bold outline effect fig, ax = plt.subplots() scatter = ax.scatter(x, y, label='Scatter Plot') # Adding a bold outline effect to the scatter points outline_effect = path_effects.withStroke(linewidth=3, foreground='black') scatter.set_path_effects([outline_effect]) ax.set_title('Scatter Plot with Bold Outline') plt.legend() plt.show()
输出
执行上述代码后,我们将获得以下输出 -
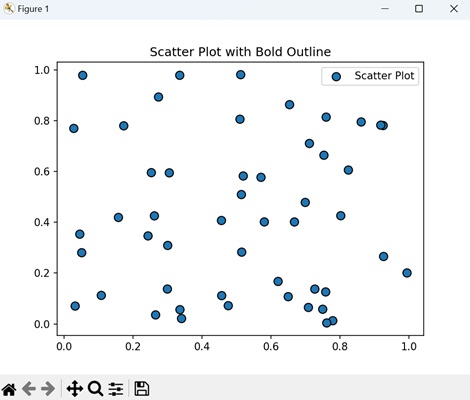
组合路径效果
在 Matplotlib 中创建组合路径效果图允许我们对线条应用多种艺术增强,例如线型、标记、颜色渐变和透明度。
- 线型 - 您可以选择各种线型选项,例如实线(' - ')、虚线(' -- ')、点线(':')等。
- 标记 - 可以添加标记以突出显示特定数据点。常见的标记包括圆圈('o')、正方形('s')、三角形('^')等。
- 颜色渐变 - 您可以使用颜色渐变创建视觉上吸引人的线条。您可以通过指定“色图”并使用它根据变量为线条着色来实现此目的。
- 透明度 - 为线条添加透明度可以使重叠线条更易于区分。您可以使用“alpha”参数调整透明度。
示例
现在,我们正在将三种路径效果应用于线条:细微的阴影效果、粗体的黑色轮廓和轻微的模糊效果。结果显示了一个组合了这些效果的线图。
import matplotlib.pyplot as plt import matplotlib.patheffects as path_effects import numpy as np # Generating data x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a line plot fig, ax = plt.subplots() line, = ax.plot(x, y, label='Combined Effects Line') # Combine multiple path effects: Shadow, Bold Outline, and Blur shadow_effect = path_effects.withStroke(linewidth=5, foreground='cyan') outline_effect = path_effects.withStroke(linewidth=3, foreground='red') blur_effect = path_effects.withStroke(linewidth=5, foreground='magenta', alpha=0.4) # Applying the combined effects to the line line.set_path_effects([shadow_effect, outline_effect, blur_effect]) ax.set_title('Combined Path Effects Line') plt.legend() plt.show()
输出
执行上述代码后,我们将获得以下输出 -
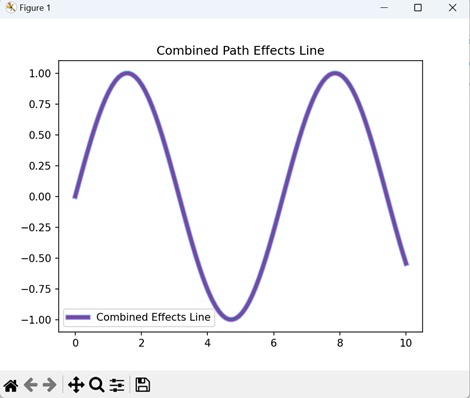