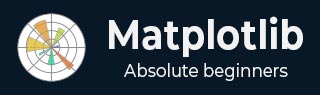
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图形
- Matplotlib - 标记
- Matplotlib - 图形
- Matplotlib - 样式
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 色图
- Matplotlib - 色图归一化
- Matplotlib - 选择色图
- Matplotlib - 色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图像
- Matplotlib - 图像蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 比例尺
- Matplotlib - 线性和对数比例尺
- Matplotlib - 对称对数和 Logit 比例尺
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - LaTeX 用于数学表达式
- Matplotlib - LaTeX 在注释中的文本格式
- Matplotlib - PostScript
- 在注释中启用 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 图形对象
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 坐标转换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例尺
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 固定图形对象
- Matplotlib - 手动等值线
- Matplotlib - 坐标报告
- Matplotlib - AGG 过滤器
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图像缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 放大镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 游标小部件
- Matplotlib - 带注释的游标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多游标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - 范围滑块
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - 跨度选择器
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等值线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等值线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 刻度定位器
在一般的图形和绘图中,刻度在通过小线段表示 x 和 y 轴的比例尺方面起着至关重要的作用,提供了对相关值的清晰指示。另一方面,刻度定位器定义了这些刻度沿轴线的位置,提供了比例尺的视觉表示。
下图显示了图形上的主刻度和次刻度 -
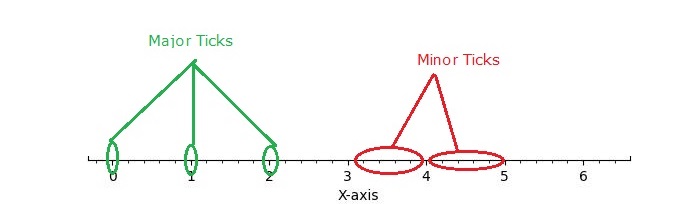
Matplotlib 中的刻度定位器
Matplotlib 提供了一种通过其刻度定位器来控制轴上刻度位置的机制。matplotlib.ticker 模块包含用于配置刻度定位和格式化的类。这些类包括通用刻度定位器、格式化器和特定于领域的自定义刻度定位器。虽然定位器不知道主刻度或次刻度,但它们由 Axis 类使用以支持主刻度和次刻度的定位和格式化。
不同的刻度定位器
matplotlib 在其 ticker 模块中提供了不同的刻度定位器,允许用户自定义轴上的刻度位置。一些刻度定位器包括 -
- AutoLocator
- MaxNLocator
- LinearLocator
- LogLocator
- MultipleLocator
- FixedLocator
- IndexLocator
- NullLocator
- SymmetricalLogLocator
- AsinhLocator
- LogitLocator
- AutoMinorLocator
- 定义自定义定位器
基本设置
在深入研究特定的刻度定位器之前,让我们建立一个通用的设置函数来绘制带有刻度的图形。
import matplotlib.pyplot as plt import numpy as np import matplotlib.ticker as ticker def draw_ticks(ax, title): # it shows the bottom spine only ax.yaxis.set_major_locator(ticker.NullLocator()) ax.spines[['left', 'right', 'top']].set_visible(False) ax.xaxis.set_ticks_position('bottom') ax.tick_params(which='major', width=1.00, length=5) ax.tick_params(which='minor', width=0.75, length=2.5) ax.set_xlim(0, 5) ax.set_ylim(0, 1) ax.text(0.0, 0.2, title, transform=ax.transAxes, fontsize=14, fontname='Monospace', color='tab:blue')
现在,让我们探索每个刻度定位器的运作方式。
自动定位器
AutoLocator 和 AutoMinorLocator 分别用于自动确定轴上主刻度和次刻度的位置。
示例
此示例演示了如何使用 AutoLocator 和 AutoMinorLocator 自动处理轴上主刻度和次刻度的位置。
# Auto Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="AutoLocator() and AutoMinorLocator()") ax.xaxis.set_major_locator(ticker.AutoLocator()) ax.xaxis.set_minor_locator(ticker.AutoMinorLocator()) ax.set_title('Auto Locator and Auto Minor Locator') plt.show()
输出
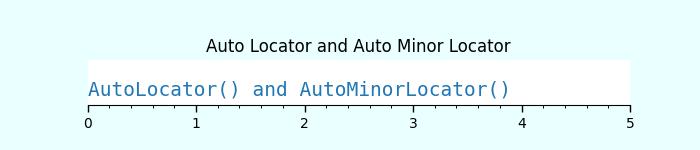
空定位器
NullLocator 不会在轴上放置任何刻度。
示例
让我们看看以下 NullLocator 的工作示例。
# Null Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="NullLocator()") ax.xaxis.set_major_locator(ticker.NullLocator()) ax.xaxis.set_minor_locator(ticker.NullLocator()) ax.set_title('Null Locator (No ticks)') plt.show()
输出
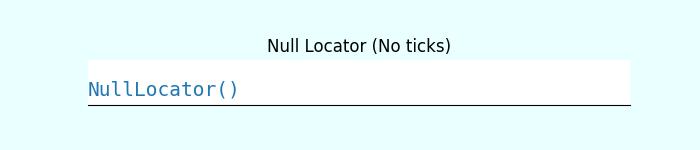
多重定位器
MultipleLocator() 类允许刻度位于指定基数的倍数处,支持整数和浮点值。
示例
以下示例演示了如何使用 MultipleLocator() 类。
# Multiple Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="MultipleLocator(0.5)") ax.xaxis.set_major_locator(ticker.MultipleLocator(0.5)) ax.xaxis.set_minor_locator(ticker.MultipleLocator(0.1)) ax.set_title('Multiple Locator') plt.show()
输出
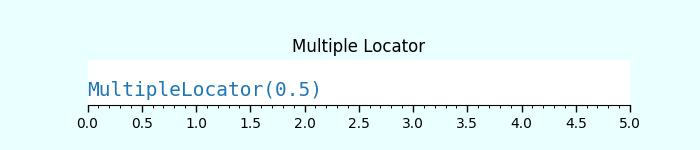
固定定位器
FixedLocator() 在指定固定的位置放置刻度。
示例
这是一个使用 FixedLocator() 类的示例。
# Fixed Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="FixedLocator([0, 1, 3, 5])") ax.xaxis.set_major_locator(ticker.FixedLocator([0, 1, 3, 5])) ax.xaxis.set_minor_locator(ticker.FixedLocator(np.linspace(0.2, 0.8, 4))) ax.set_title('Fixed Locator') plt.show()
输出
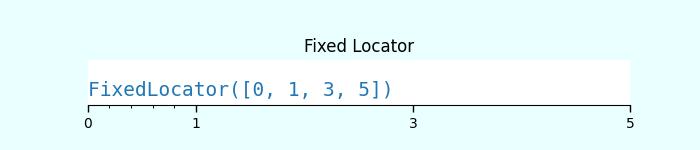
线性定位器
LinearLocator 在指定的最小值和最大值之间均匀地间隔刻度。
示例
这是一个将线性定位器应用于轴的主刻度和次刻度的示例。
# Linear Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="LinearLocator(numticks=3)") ax.xaxis.set_major_locator(ticker.LinearLocator(3)) ax.xaxis.set_minor_locator(ticker.LinearLocator(10)) ax.set_title('Linear Locator') plt.show()
输出
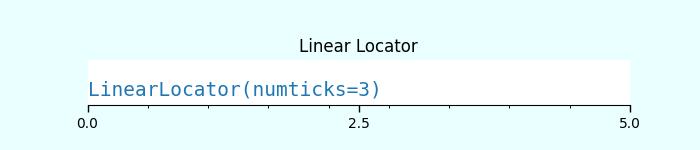
索引定位器
此定位器适用于索引图,其中 x = range(len(y))。
示例
这是一个使用索引定位器 (ticker.IndexLocator() 类) 的示例。
# Index Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="IndexLocator(base=0.5, offset=0.25)") ax.plot([0]*5, color='white') ax.xaxis.set_major_locator(ticker.IndexLocator(base=0.5, offset=0.25)) ax.set_title('Index Locator') plt.show()
输出
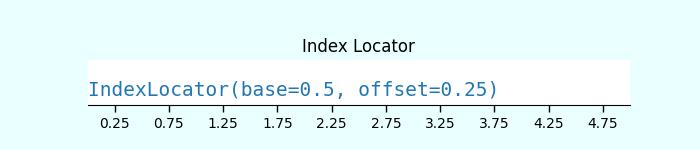
MaxN 定位器
MaxNLocator 找到最多一定数量的具有良好位置刻度的区间。示例
这是一个使用 MaxNLocator() 类对主刻度和次刻度进行操作的示例。
# MaxN Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="MaxNLocator(n=4)") ax.xaxis.set_major_locator(ticker.MaxNLocator(4)) ax.xaxis.set_minor_locator(ticker.MaxNLocator(40)) ax.set_title('MaxN Locator') plt.show()
输出
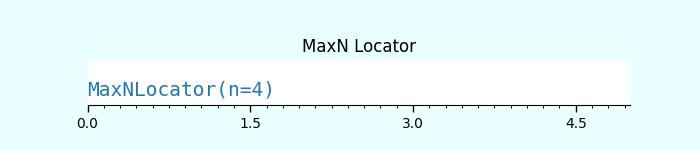
对数定位器
LogLocator 用于从 min 到 max 对数地间隔刻度。
示例
让我们看看使用对数定位器的示例。它显示了对数刻度上的次刻度标签。
# Log Locator fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff') plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4) draw_ticks(ax, title="LogLocator(base=10, numticks=15)") ax.set_xlim(10**3, 10**10) ax.set_xscale('log') ax.xaxis.set_major_locator(ticker.LogLocator(base=10, numticks=15)) ax.set_title('Log Locator') plt.show()
输出
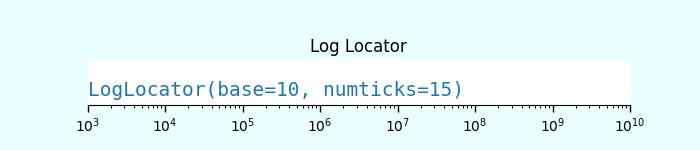