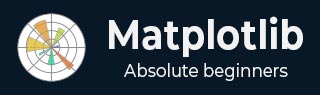
- Matplotlib 基础
- Matplotlib - 首页
- Matplotlib - 简介
- Matplotlib - 与 Seaborn 的比较
- Matplotlib - 环境设置
- Matplotlib - Anaconda 发行版
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - 保存图表
- Matplotlib - 标记
- Matplotlib - 图表
- Matplotlib - 风格
- Matplotlib - 图例
- Matplotlib - 颜色
- Matplotlib - 颜色映射
- Matplotlib - 颜色映射规范化
- Matplotlib - 选择颜色映射
- Matplotlib - 颜色条
- Matplotlib - 文本
- Matplotlib - 文本属性
- Matplotlib - 子图标题
- Matplotlib - 图片
- Matplotlib - 图片蒙版
- Matplotlib - 注释
- Matplotlib - 箭头
- Matplotlib - 字体
- Matplotlib - 什么是字体?
- 全局设置字体属性
- Matplotlib - 字体索引
- Matplotlib - 字体属性
- Matplotlib - 刻度
- Matplotlib - 线性与对数刻度
- Matplotlib - 对称对数和 Logit 刻度
- Matplotlib - LaTeX
- Matplotlib - 什么是 LaTeX?
- Matplotlib - 用于数学表达式的 LaTeX
- Matplotlib - 注释中的 LaTeX 文本格式
- Matplotlib - PostScript
- 启用注释中的 LaTeX 渲染
- Matplotlib - 数学表达式
- Matplotlib - 动画
- Matplotlib - 绘图元素
- Matplotlib - 使用 Cycler 进行样式设置
- Matplotlib - 路径
- Matplotlib - 路径效果
- Matplotlib - 变换
- Matplotlib - 刻度和刻度标签
- Matplotlib - 弧度刻度
- Matplotlib - 日期刻度
- Matplotlib - 刻度格式化器
- Matplotlib - 刻度定位器
- Matplotlib - 基本单位
- Matplotlib - 自动缩放
- Matplotlib - 反转坐标轴
- Matplotlib - 对数坐标轴
- Matplotlib - Symlog
- Matplotlib - 单位处理
- Matplotlib - 带单位的椭圆
- Matplotlib - 脊柱
- Matplotlib - 坐标轴范围
- Matplotlib - 坐标轴比例
- Matplotlib - 坐标轴刻度
- Matplotlib - 格式化坐标轴
- Matplotlib - Axes 类
- Matplotlib - 双坐标轴
- Matplotlib - Figure 类
- Matplotlib - 多图
- Matplotlib - 网格
- Matplotlib - 面向对象接口
- Matplotlib - PyLab 模块
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 固定位置的绘图元素
- Matplotlib - 手动等高线
- Matplotlib - 坐标报告
- Matplotlib - AGG 滤镜
- Matplotlib - 带状框
- Matplotlib - 填充螺旋线
- Matplotlib - Findobj 演示
- Matplotlib - 超链接
- Matplotlib - 图片缩略图
- Matplotlib - 使用关键字绘图
- Matplotlib - 创建 Logo
- Matplotlib - 多页 PDF
- Matplotlib - 多进程
- Matplotlib - 打印标准输出
- Matplotlib - 复合路径
- Matplotlib - Sankey 类
- Matplotlib - MRI 与 EEG
- Matplotlib - 样式表
- Matplotlib - 背景颜色
- Matplotlib - Basemap
- Matplotlib 事件处理
- Matplotlib - 事件处理
- Matplotlib - 关闭事件
- Matplotlib - 鼠标移动
- Matplotlib - 点击事件
- Matplotlib - 滚动事件
- Matplotlib - 按键事件
- Matplotlib - 选择事件
- Matplotlib - 透视镜
- Matplotlib - 路径编辑器
- Matplotlib - 多边形编辑器
- Matplotlib - 定时器
- Matplotlib - Viewlims
- Matplotlib - 缩放窗口
- Matplotlib 小部件
- Matplotlib - 光标小部件
- Matplotlib - 带注释的光标
- Matplotlib - 按钮小部件
- Matplotlib - 复选框
- Matplotlib - 套索选择器
- Matplotlib - 菜单小部件
- Matplotlib - 鼠标光标
- Matplotlib - 多光标
- Matplotlib - 多边形选择器
- Matplotlib - 单选按钮
- Matplotlib - RangeSlider
- Matplotlib - 矩形选择器
- Matplotlib - 椭圆选择器
- Matplotlib - 滑块小部件
- Matplotlib - Span Selector
- Matplotlib - 文本框
- Matplotlib 绘图
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- Matplotlib - 等高线图
- Matplotlib - 3D 绘图
- Matplotlib - 3D 等高线
- Matplotlib - 3D 线框图
- Matplotlib - 3D 表面图
- Matplotlib - Quiver 图
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用资源
- Matplotlib - 讨论
Matplotlib - 颜色映射
颜色映射(通常称为颜色表或调色板)是一组按特定顺序排列的颜色,用于直观地表示数据。请参见下图以供参考:
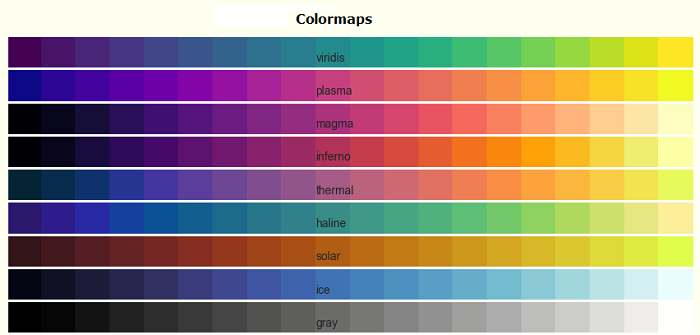
在Matplotlib的上下文中,颜色映射在将数值映射到各种绘图中的颜色方面起着至关重要的作用。Matplotlib 提供内置的颜色映射和外部库(如 Palettable),甚至允许我们创建和操作自己的颜色映射。
Matplotlib 中的颜色映射
Matplotlib 提供许多内置的颜色映射,例如“viridis”或“copper”,这些可以通过matplotlib.colormaps容器访问。它是一个通用的注册实例,返回一个颜色映射对象。
示例
以下示例获取 matplotlib 中所有已注册颜色映射的列表。
from matplotlib import colormaps print(list(colormaps))
输出
['magma', 'inferno', 'plasma', 'viridis', 'cividis', 'twilight', 'twilight_shifted', 'turbo', 'Blues', 'BrBG', 'BuGn', 'BuPu', 'CMRmap', 'GnBu', 'Greens', 'Greys', 'OrRd', 'Oranges', 'PRGn', 'PiYG', 'PuBu', 'PuBuGn', 'PuOr', 'PuRd', 'Purples', 'RdBu', 'RdGy', 'RdPu', 'RdYlBu', 'RdYlGn', 'Reds', 'Spectral', 'Wistia', 'YlGn', 'YlGnBu', 'YlOrBr', 'YlOrRd', 'afmhot', 'autumn', 'binary', 'bone', 'brg', 'bwr', 'cool', 'coolwarm', 'copper', 'cubehelix', 'flag', 'gist_earth', 'gist_gray', 'gist_heat', 'gist_ncar', 'gist_rainbow', 'gist_stern', 'gist_yarg', 'gnuplot', 'gnuplot2', 'gray', 'hot', 'hsv', 'jet', 'nipy_spectral', 'ocean', …]
访问颜色映射及其值
您可以使用matplotlib.colormaps['viridis']获取命名颜色映射,它返回一个颜色映射对象。获得颜色映射对象后,您可以通过重新采样颜色映射来访问其值。在这种情况下,viridis是一个颜色映射对象,当传递 0 到 1 之间的浮点数时,它会从颜色映射返回一个 RGBA 值。
示例
这是一个访问颜色映射值的示例。
import matplotlib viridis = matplotlib.colormaps['viridis'].resampled(8) print(viridis(0.37))
输出
(0.212395, 0.359683, 0.55171, 1.0)
创建和操作颜色映射
Matplotlib 提供了创建或操作您自己的颜色映射的灵活性。此过程涉及使用ListedColormap或LinearSegmentedColormap类。
使用 ListedColormap 创建颜色映射
ListedColormaps类可用于通过提供颜色规范列表或数组来创建自定义颜色映射。您可以使用它来使用颜色名称或 RGB 值构建新的颜色映射。
示例
以下示例演示如何使用具有特定颜色名称的ListedColormap类创建自定义颜色映射。
import matplotlib as mpl from matplotlib.colors import ListedColormap import matplotlib.pyplot as plt import numpy as np # Creating a ListedColormap from color names colormaps = [ListedColormap(['rosybrown', 'gold', "crimson", "linen"])] # Plotting examples np.random.seed(19680801) data = np.random.randn(30, 30) n = len(colormaps) fig, axs = plt.subplots(1, n, figsize=(7, 3), layout='constrained', squeeze=False) for [ax, cmap] in zip(axs.flat, colormaps): psm = ax.pcolormesh(data, cmap=cmap, rasterized=True, vmin=-4, vmax=4) fig.colorbar(psm, ax=ax) plt.show()
输出
执行上述程序后,您将获得以下输出:
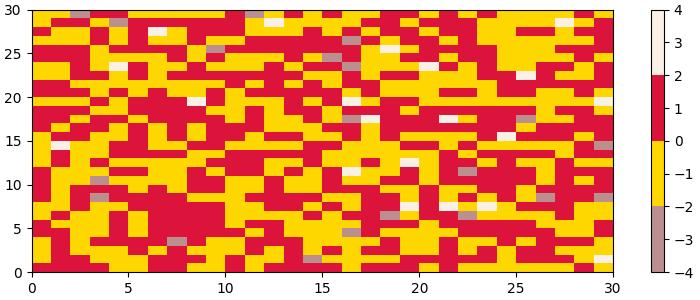
使用 LinearSegmentedColormap 创建颜色映射
LinearSegmentedColormap类允许通过指定锚点及其对应的颜色来进行更多控制。这使得能够创建具有插值值的颜色映射。
示例
以下示例演示如何使用具有特定颜色名称列表的LinearSegmentedColormap类创建自定义颜色映射。
import matplotlib as mpl from matplotlib.colors import LinearSegmentedColormap import matplotlib.pyplot as plt import numpy as np # Creating a LinearSegmentedColormap from a list colors = ["rosybrown", "gold", "lawngreen", "linen"] cmap_from_list = [LinearSegmentedColormap.from_list("SmoothCmap", colors)] # Plotting examples np.random.seed(19680801) data = np.random.randn(30, 30) n = len(cmap_from_list) fig, axs = plt.subplots(1, n, figsize=(7, 3), layout='constrained', squeeze=False) for [ax, cmap] in zip(axs.flat, cmap_from_list): psm = ax.pcolormesh(data, cmap=cmap, rasterized=True, vmin=-4, vmax=4) fig.colorbar(psm, ax=ax) plt.show()
输出
执行上述程序后,您将获得以下输出:
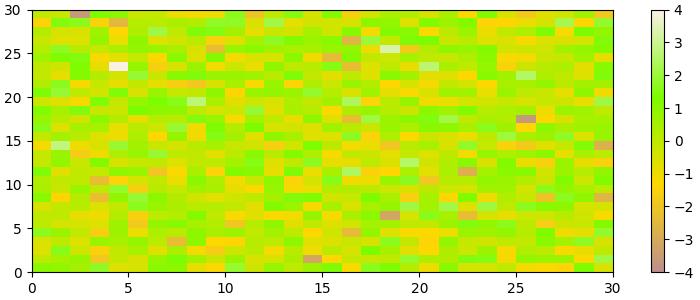
反转颜色映射
可以使用colormap.reversed()方法反转颜色映射。这将创建一个新的颜色映射,它是原始颜色映射的反转版本。
示例
此示例生成原始颜色映射及其反转版本的并排可视化。
from matplotlib.colors import ListedColormap import matplotlib.pyplot as plt import numpy as np # Define a list of color values in hexadecimal format colors = ["#bbffcc", "#a1fab4", "#41b6c4", "#2c7fb8", "#25abf4"] # Create a ListedColormap with the specified colors my_cmap = ListedColormap(colors, name="my_cmap") # Create a reversed version of the colormap my_cmap_r = my_cmap.reversed() # Define a helper function to plot data with associated colormap def plot_examples(colormaps): np.random.seed(19680801) data = np.random.randn(30, 30) n = len(colormaps) fig, axs = plt.subplots(1, n, figsize=(n * 2 + 2, 3), layout='constrained', squeeze=False) for [ax, cmap] in zip(axs.flat, colormaps): psm = ax.pcolormesh(data, cmap=cmap, rasterized=True, vmin=-4, vmax=4) fig.colorbar(psm, ax=ax) plt.show() # Plot the original and reversed colormaps plot_examples([my_cmap, my_cmap_r])
输出
执行上述程序后,您将获得以下输出:
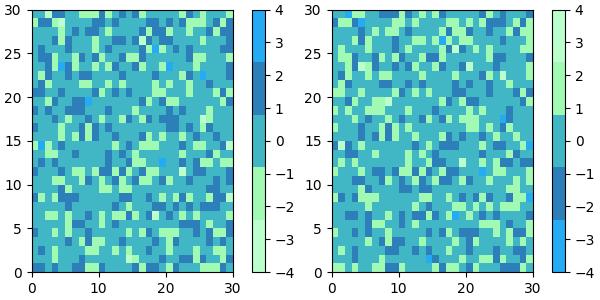
更改默认颜色映射
要更改所有后续绘图的默认颜色映射,您可以使用mpl.rc()修改默认颜色映射设置。
示例
这是一个通过修改全局 Matplotlib 设置(如 mpl.rc('image', cmap='RdYlBu_r'))将默认颜色映射更改为RdYlBu_r的示例。
import numpy as np from matplotlib import pyplot as plt import matplotlib as mpl # Generate random data data = np.random.rand(4, 4) # Create a figure with two subplots fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(7, 4)) # Plot the first subplot with the default colormap ax1.imshow(data) ax1.set_title("Default colormap") # Set the default colormap globally to 'RdYlBu_r' mpl.rc('image', cmap='RdYlBu_r') # Plot the modified default colormap ax2.imshow(data) ax2.set_title("Modified default colormap") # Display the figure with both subplots plt.show()
输出
执行上述代码后,我们将获得以下输出:
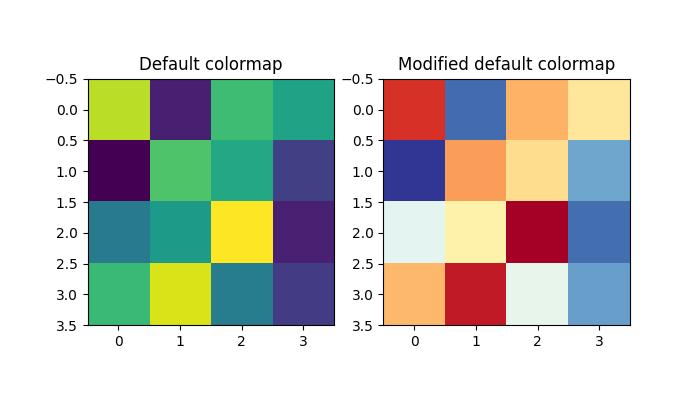
通过颜色映射绘制带颜色的线条
要通过颜色映射绘制具有不同颜色的多条线,您可以利用 Matplotlib 的 plot() 函数以及颜色映射来为每条线分配不同的颜色。
示例
以下示例通过迭代范围并使用 plot() 函数中的 color 参数从颜色映射中为每条线分配不同的颜色来绘制多条线。
import numpy as np import matplotlib.pyplot as plt plt.rcParams["figure.figsize"] = [7.00, 3.50] plt.rcParams["figure.autolayout"] = True # Generate x and y data x = np.linspace(0, 2 * np.pi, 64) y = np.exp(x) # Plot the initial line plt.plot(x, y) # Define the number of lines and create a colormap n = 20 colors = plt.cm.rainbow(np.linspace(0, 1, n)) # Plot multiple lines with different colors using a loop for i in range(n): plt.plot(x, i * y, color=colors[i]) plt.xlim(4, 6) plt.show()
输出
执行上述代码后,我们将获得以下输出:
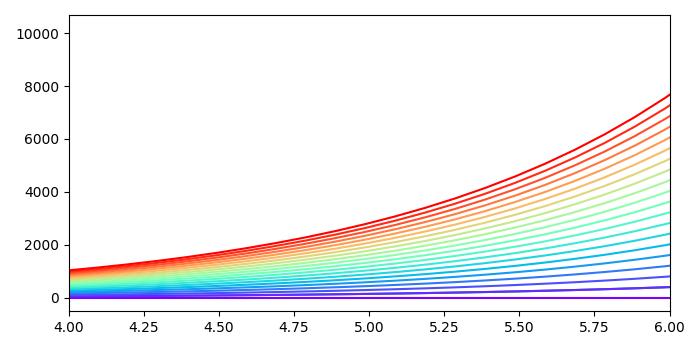