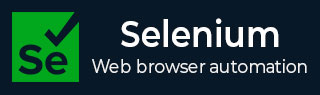
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 发射代码
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 Iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 复选框
Selenium Webdriver 可用于处理网页上的复选框。所有复选框都使用 input 标签名称进行识别。此外,网页上的每个复选框都具有一个名为 type 的属性,其值为 checkbox。
在网页上识别复选框
打开 Chrome 浏览器,并启动一个应用程序。右键单击网页,然后单击“检查”按钮。要识别页面上的复选框,请单击可用 HTML 代码顶部左侧的向上箭头,如下所示。
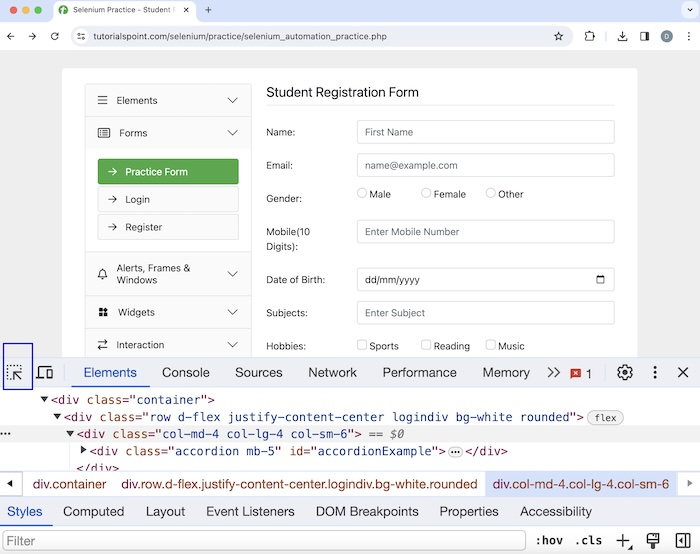
一旦我们单击并将箭头指向复选框(在下图中突出显示),其 HTML 代码就会出现,反映了 input 标签名称和 type 属性的值为复选框。
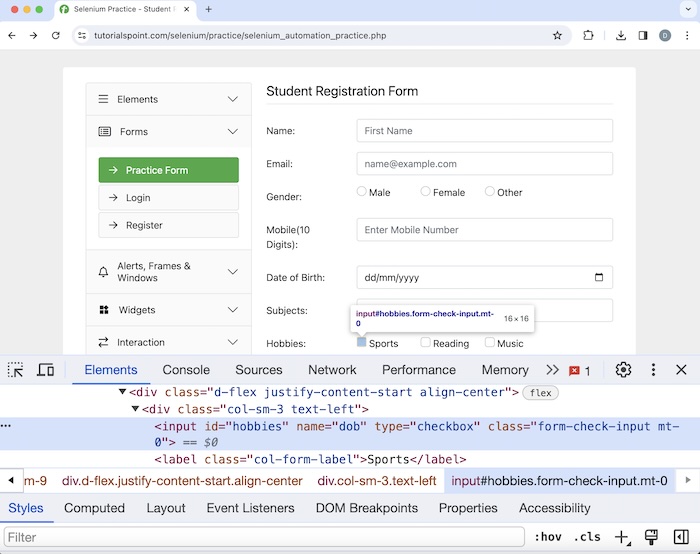
选择复选框并验证
让我们以上面页面的示例为例,我们将使用 click() 方法单击第一个复选框。然后,我们将使用 isSelected() 方法验证复选框是否已选中。
要获取有关 isSelected() 方法的更多信息,请参阅链接 Selenium WebDriver WebElement 命令。
语法
Webdriver driver = new ChromeDriver(); WebElement checkbox= driver.findElement(By.xpath("value of xpath")); checkbox.click(); boolean result = checkBox.isSelected();
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class HandlingCheckbox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify the first checkbox WebElement checkBox = driver.findElement(By.xpath("//*[@id='hobbies']")); // click the checkbox checkBox.click(); // check if a checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // Closing browser driver.quit(); } }
输出
Checking if a checkbox is selected: true Process finished with exit code 0
在上面的示例中,我们首先单击了第一个复选框,然后在控制台中验证了复选框是否已选中,消息为 - 检查复选框是否已选中:true。
最后,收到消息 进程已完成,退出代码为 0,表示代码已成功执行。
计算总复选框数
让我们以下面页面的另一个示例为例,我们将计算复选框的总数。在此示例中,复选框的总数应为 3。
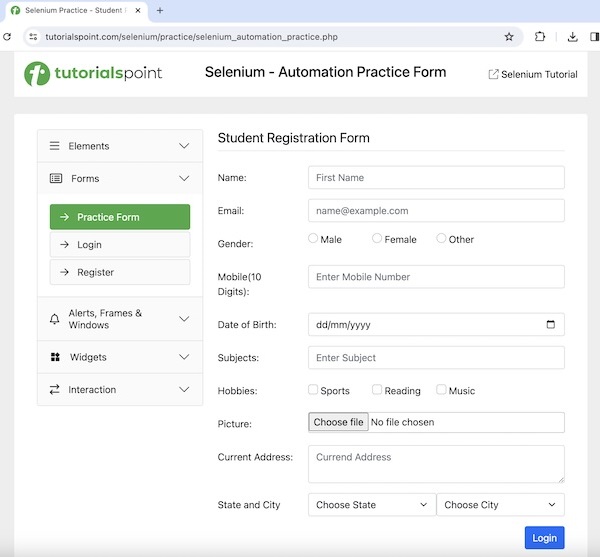
语法
Webdriver driver = new ChromeDriver(); List<WebElement> totalChks = driver.findElements (By.xpath("<xpath value of all checkboxes>")); int count = totalChks.size();
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import java.util.List; public class CountingCheckbox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Retrieve all checkboxes using locator and storing in List List<WebElement> totalChks = driver.findElements(By.xpath("//input[@type='checkbox']")); // count number of checkboxes int count = totalChks.size(); System.out.println("Count the checkboxes: " + count); //Closing browser driver.quit(); } }
输出
Count the checkboxes: 3
在上面的示例中,我们计算了网页上复选框的总数,并在控制台中收到了消息 - 计算复选框数:3。
验证复选框
让我们再举一个关于复选框的例子,我们将对复选框执行一些验证。首先,我们将使用 isEnabled() 方法检查复选框是否已启用/禁用。此外,我们还将分别使用 isDisplayed() 和 isSelected() 方法验证它是否显示以及是否已选中/未选中。
语法
Webdriver driver = new ChromeDriver(); // identify checkbox but not selected WebElement checkBox = driver.findElement(By.xpath("<xpath value of checkbox>")); // verify if checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // verify if checkbox is displayed boolean result1 = checkBox.isDisplayed(); System.out.println("Checking if a checkbox is displayed: " + result1); // verify if checkbox is enabled boolean result2 = checkBox.isEnabled(); System.out.println("Checking if a checkbox is enabled: " + result2);
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CheckboxValidates { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify checkbox driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify checkbox but not select WebElement checkBox = driver.findElement (By.xpath("//*[@id='practiceForm']/div[7]/div/div/div[2]/input")); // verify if checkbox is selected boolean result = checkBox.isSelected(); System.out.println("Checking if a checkbox is selected: " + result); // verify if checkbox is displayed boolean result1 = checkBox.isDisplayed(); System.out.println("Checking if a checkbox is displayed: " + result1); // verify if checkbox is enabled boolean result2 = checkBox.isEnabled(); System.out.println("Checking if a checkbox is enabled: " + result2); // Closing browser driver.quit(); } }
输出
Checking if a checkbox is selected: false Checking if a checkbox is displayed: true Checking if a checkbox is enabled: true
在上面的示例中,我们验证了复选框是否显示、启用和选中,并在控制台中收到了以下消息 - 检查复选框是否已选中:false,检查复选框是否已显示:true 和检查复选框是否已启用:true。
结论
这总结了我们关于 Selenium Webdriver 复选框教程的全面介绍。我们从描述 HTML 中复选框的识别开始,并提供了一些示例来说明如何在 Selenium Webdriver 中处理复选框。这使您能够深入了解 Selenium Webdriver 复选框。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。