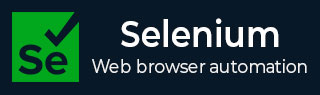
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 定位器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理Cookie
- Selenium - 日期时间选择器
- Selenium - 动态Web表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理Ajax调用
- Selenium - JSON 数据文件
- Selenium - CSV数据文件
- Selenium - Excel数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 相对定位器
Selenium 4 提供了使用相对定位器(也称为友好定位器)的选项,除了用于识别网页上元素的普通定位器之外。
Selenium 中的相对定位器是什么?
相对定位器用于识别在 Web 应用程序中没有足够信息唯一定位的元素。为了检测它,我们可以根据另一个元素(可以使用其属性唯一定位)的空间位置来识别它。
请注意,使用相对定位器时,需要添加导入语句:
import org.openqa.selenium.support.locators.RelativeLocator
此外,如果使用 Maven 项目,则应在 pom.xml 中添加 4.0 以上版本的 Selenium 依赖项。这是因为相对定位器仅从 Selenium 4.0 开始受支持。
Selenium WebDriver 中使用的相对定位器如下所示:
- above()
- below()
- near()
- toLeftOf()
- toRightOf()
示例 - above() 相对定位器
让我们以下面页面中突出显示的元素为例,我们将使用 **above** 定位器来识别出现在 **login** 链接上方的文本 **Practice Form**。
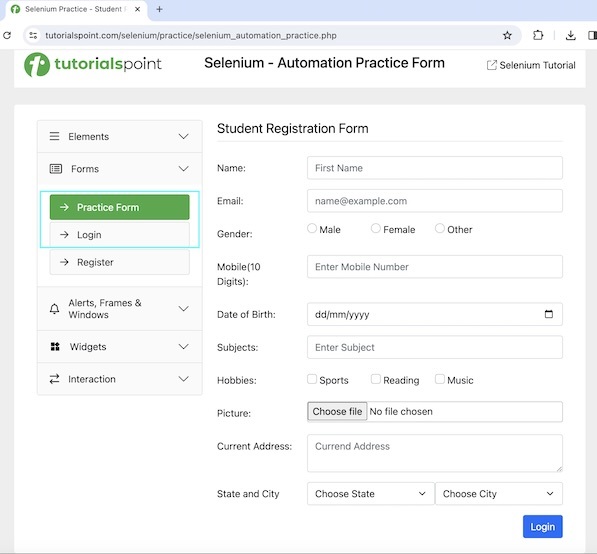
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element above the first link element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("a")).above(l));
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsAbove { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); // identify element above the first element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("a")).above(l)); // Getting element text value the above identified element System.out.println("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
添加到 pom.xml 文件中的依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> </dependencies> </project>
输出
Getting element text: Practice Form Process finished with exit code 0
在上面的示例中,我们借助 above 相对定位器识别了元素,并通过控制台中的消息获得了其文本 - **获取元素文本:Practice Form**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码成功执行。
示例 - below() 相对定位器
让我们再以下面页面中突出显示的元素为例,我们将使用 **below** 定位器来识别出现在 **Register** 链接下方的链接 **Login**。
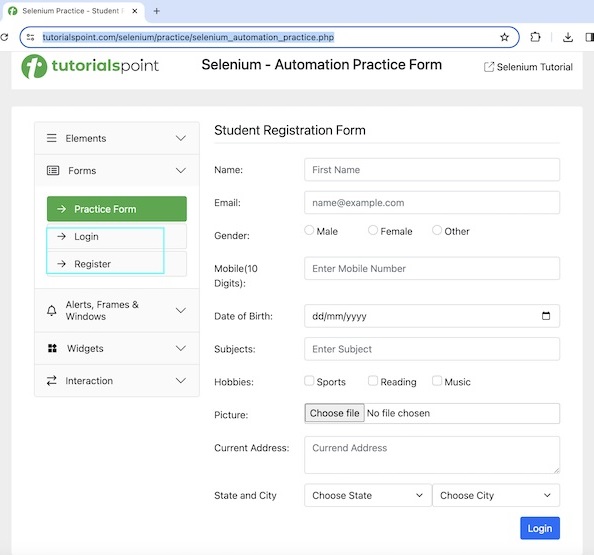
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element below the first link element WebElement e = driver. findElement(RelativeLocator.with(By.tagName("a")).below(l));
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsBelow { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); // identify element below the first element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("a")).below(l)); // Getting element text value the below identified element System.out.println("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
输出
Getting element text: Register
在上面的示例中,我们借助 below 相对定位器识别了元素,并通过控制台中的消息获得了其文本 - **获取元素文本:Register**。
示例 - toLeftOf() 相对定位器
让我们再以下面页面中突出显示的元素为例,我们将使用 **toLeftOf** 定位器来识别出现在输入框左侧的标签文本 **Name**。
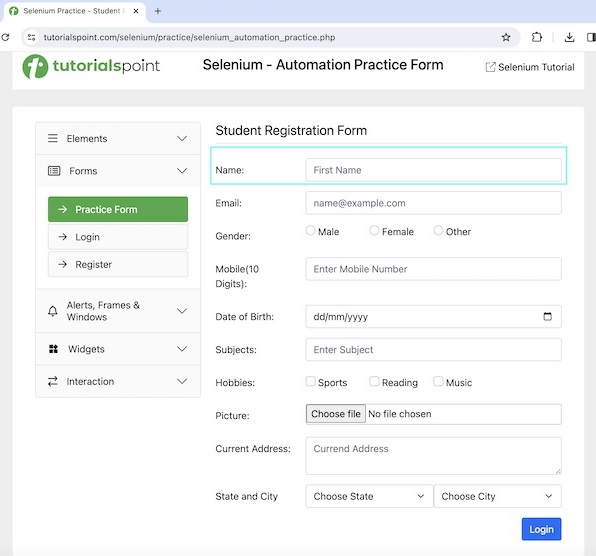
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element to left of the first element WebElement e = driver. findElement(RelativeLocator.with(By.tagName("a")).toLeftOf(l));
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsLeft { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("//*[@id='name']")); // identify element left of the first element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("label")).toLeftOf(l)); // Getting element text to left of identified element System.out.println("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
输出
Getting element text: Name:
在上面的示例中,我们借助 toLeftOf 相对定位器识别了元素,并通过控制台中的消息获得了其文本 - **获取元素文本:Name:**。
示例 - toRightOf() 相对定位器
让我们再以下面页面中突出显示的元素为例,我们将使用 **toRightOf** 定位器来识别出现在页面徽标右侧的文本 **Selenium - Automation Practice Form**。
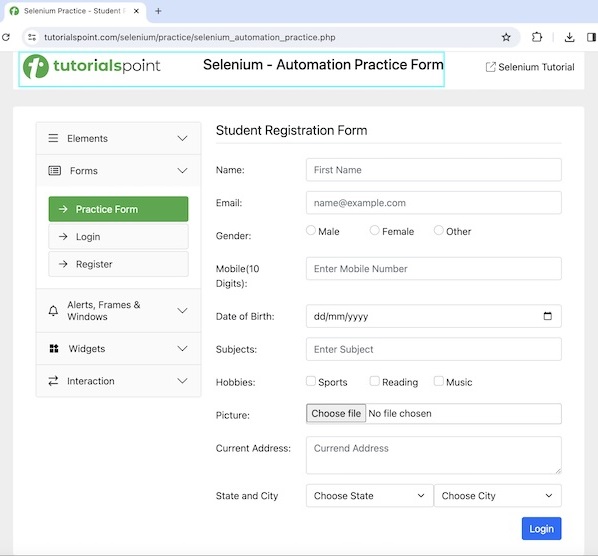
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element to right of the first element WebElement e = driver. findElement(RelativeLocator.with(By.tagName("h1")).toRightOf(l));
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsRight { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("/html/body/div/header/div[1]/a")); // identify element right of the first element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("h1")).toRightOf(l)); // Getting element text to right of identified element System.out.println("Getting element text: " + e.getText()); // Closing browser driver.quit(); } }
输出
Getting element text: Selenium - Automation Practice Form
在上面的示例中,我们借助 toRightOf 相对定位器识别了元素,并通过控制台中的消息获得了其文本 - **获取元素文本:Selenium - Automation Practice Form**。
示例 - near() 相对定位器
让我们再以下面页面中突出显示的元素为例,我们将使用 **near** 定位器来识别并选中出现在 **Sports** 标签附近的复选框。
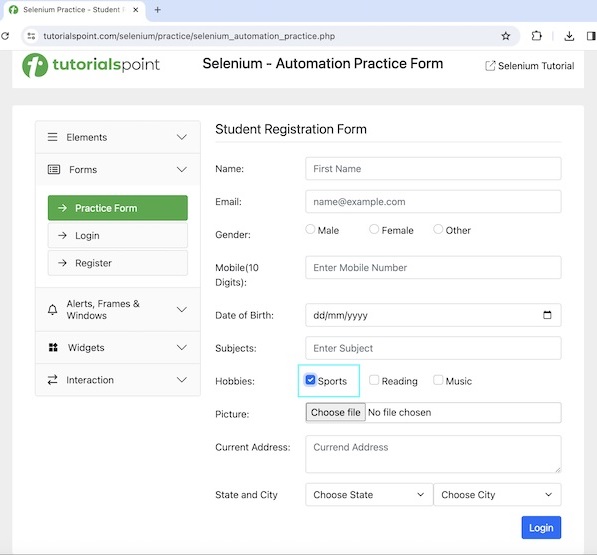
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element to near of the first element WebElement e = driver. findElement(RelativeLocator.with(By.tagName("input")).near(l));
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsNear { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("//*[@id='practiceForm']/div[7]/div/div/div[1]/label")); // identify element near the first element WebElement e = driver.findElement(RelativeLocator.with(By.tagName("input")).near(l)); // check checkbox e.click(); // verify is selected System.out.println("Verify if selected: " + e.isSelected()); // Closing browser driver.quit(); } }
输出
Verify if selected: true
在上面的示例中,我们借助 near 相对定位器识别了复选框,并通过控制台中的消息验证了该复选框是否已选中 - **验证是否选中:true**。
示例 - 相对定位器的链式调用
让我们再以下面页面中突出显示的元素为例,我们将使用 **above** 和 **toRightOf** 链式定位器,在出现在 **Email:** 标签上方且 **Name:** 标签右侧的输入框中输入文本 **Selenium**。
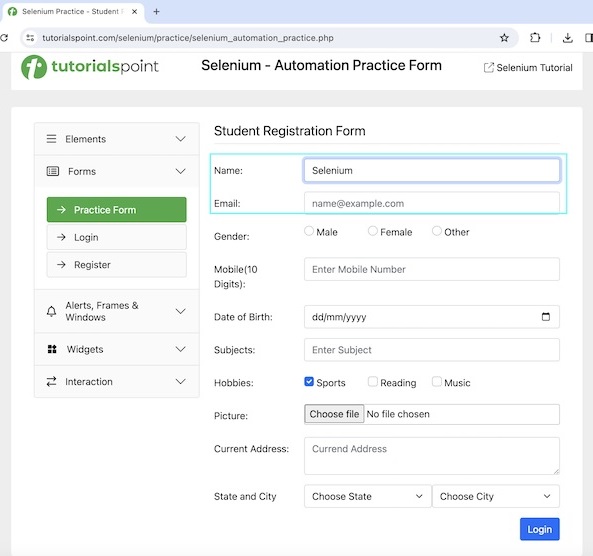
语法
WebDriver driver = new ChromeDriver(); // identify element the first element WebElement l = driver.findElement(By.xpath("value of xpath locator")); // identify element the second element WebElement m = driver.findElement(By.xpath("value of xpath locator")); // identify element by chaining relative locators // identify element by chaining elements WebElement e = driver.findElement(RelativeLocator.with (By.tagName("input")).above(l).toRightOf(m)); // enter some text e.sendKeys("Selenium");
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.locators.RelativeLocator; import java.util.concurrent.TimeUnit; public class RelativeLocatorsChain { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and navigate to a URL driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // identify first element WebElement l = driver.findElement(By.xpath("//*[@id='practiceForm']/div[1]/label")); // identify second element WebElement s = driver.findElement(By.xpath("//*[@id='practiceForm']/div[2]/label")); // identify element by chaining elements WebElement e = driver.findElement(RelativeLocator.with (By.tagName("input")).above(s).toRightOf(l)); // input text e.sendKeys("Selenium"); // verify is selected System.out.println("Value entered is: " + e.getAttribute("value")); // Closing browser driver.quit(); } }
输出
Value entered is: Selenium
在上面的示例中,我们借助相对定位器的链式调用识别了元素,并通过控制台中的消息获得了输入的文本 - **输入的值是:Selenium**。
结论
本教程全面介绍了 Selenium WebDriver 相对定位器。我们从描述 Selenium 中的相对定位器开始,并通过示例说明如何在 Selenium WebDriver 中使用相对定位器。这使您能够深入了解 Selenium WebDriver 相对定位器。明智的做法是继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。