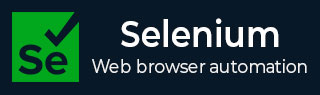
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 表单处理
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 表单处理
Selenium Webdriver 可用于处理网页上的表单。在 HTML 术语中,表单元素由名为Form 的标签名标识。此外,它还应该具有提交表单的功能,用于表单提交的元素应该具有名为input 的标签名,以及值为submit 的属性type。需要注意的是,网页上的表单可能包含文本框、链接、复选框、单选按钮和其他 Web 元素,这些元素可以帮助用户在网页上输入详细信息。
网页上表单的识别
右键单击 Chrome 浏览器中打开的页面。单击“检查”按钮。之后,整个页面的HTML 代码将可见。要检查页面上的表单元素,请单击 HTML 代码顶部左侧的向上箭头,如下所示。
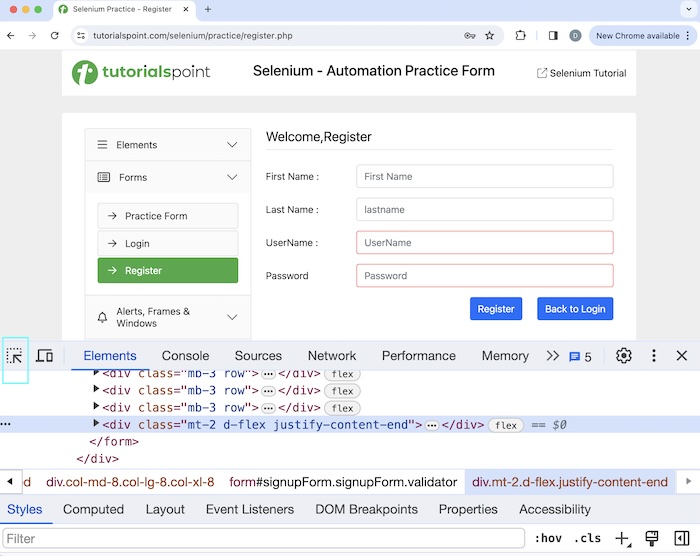
单击并将箭头指向表单内的任何元素(例如,“注册”按钮)后,其 HTML 代码将可用,反映了form 标签名。
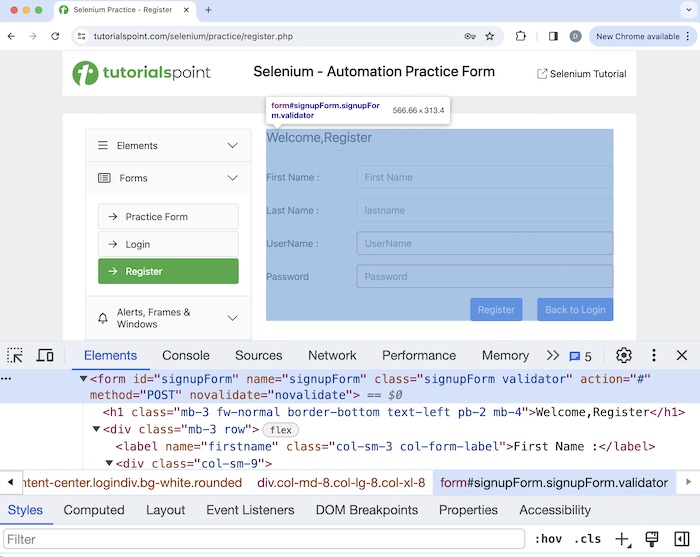
在上图表单中,我们可以使用注册按钮提交包含详细信息的表单。单击并将箭头指向注册按钮后,其 HTML 代码将出现,反映了input 标签名及其值为submit 的type 属性。
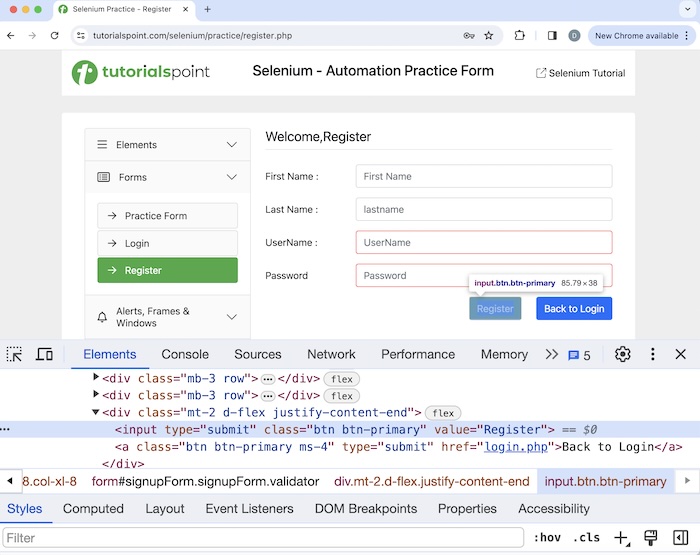
<input type="submit" class="btn btn-primary" value="Register">
我们可以使用 submit() 和 click() 方法提交表单。普通按钮和提交按钮之间的基本区别在于,普通按钮只能与 click() 方法交互,而提交按钮可以使用 click() 和 submit() 方法交互。
语法
使用 submit 方法的语法:
WebDriver driver = new ChromeDriver(); // identify input box 1 WebElement inputBx = driver.findElement (By.xpath("<value of xpath>")); inputBx.sendKeys("Selenium"); // submit form WebElement btn = driver.findElement (By.xpath("<value of xpath>")); btn.submit();
此外,普通按钮具有input 标签名,其type 属性的值应为button。在下面的页面中,让我们看看网页上Click Me按钮的HTML代码。
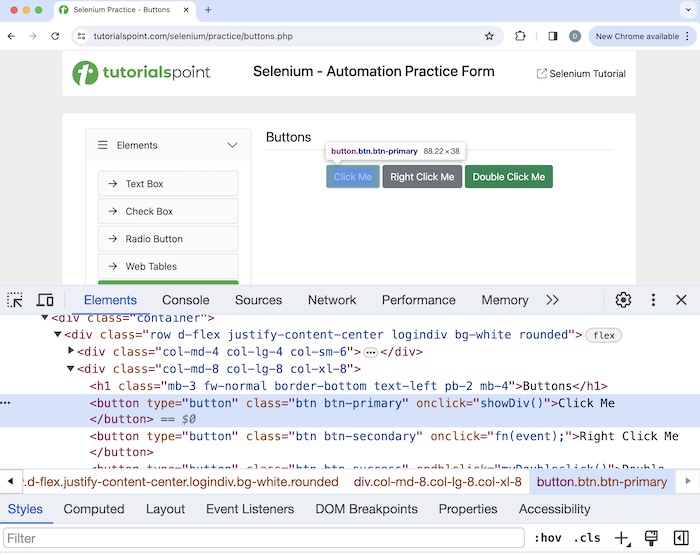
<button type="button" class="btn btn-primary" onclick="showDiv()">Click Me</button>
示例 1 - 使用 submit() 方法
让我们以下面页面中的表单为例,该表单包含 Web 元素 - 标签、输入框、按钮、密码等等。
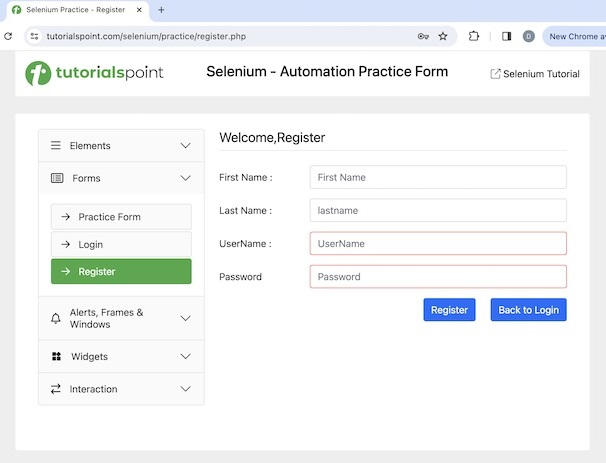
语法
WebDriver driver = new ChromeDriver(); // identify input box 1 WebElement inputBx = driver.findElement (By.xpath("<value of xpath>")); inputBx.sendKeys("Selenium"); // get value entered System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value")); // identify input box 2 WebElement inputBx2 = driver.findElement (By.xpath("<value of xpath>")); inputBx2.sendKeys("Tutorials"); // get value entered System.out.println("Value entered in LastName: " + inputBx2.getAttribute("value")); // identify input box 3 WebElement inputBx3 = driver.findElement (By.xpath("<value of xpath>")); inputBx3.sendKeys("Tutorialspoint"); // identify input box 4 WebElement inputBx4 = driver.findElement (By.xpath("<value of xpath>")); inputBx3.sendKeys("Tutorialspoint"); // submit form WebElement btn = driver.findElement (By.xpath("<value of xpath>")); btn.submit();
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class FormElements { public static void main(String[] args) { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify form driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // identify input box 1 WebElement inputBx = driver.findElement(By.xpath("//*[@id='firstname']")); inputBx.sendKeys("Selenium"); // get value entered System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value")); // identify input box 2 WebElement inputBx2 = driver.findElement(By.xpath("//*[@id='lastname']")); inputBx2.sendKeys("Tutorials"); // get value entered System.out.println("Value entered in LastName: " + inputBx2.getAttribute("value")); // identify input box 3 WebElement inputBx3 = driver.findElement(By.xpath("//*[@id='username']")); inputBx3.sendKeys("Tutorialspoint"); // get value entered System.out.println("Value entered in UserName: " + inputBx3.getAttribute("value")); // identify input box 4 WebElement inputBx4 = driver.findElement(By.xpath("//*[@id='password']")); inputBx3.sendKeys("Tutorialspoint"); // submit form with submit method WebElement btn = driver.findElement(By.xpath("//*[@id='signupForm']/div[5]/input")); btn.submit(); // Close browser driver.quit(); } }
输出
Value entered in FirstName: Selenium Value entered in LastName: Tutorials Value entered in UserName: Tutorialspoint Process finished with exit code 0
在上面的示例中,我们填写了包含输入框的表单,然后在控制台中获取输入的值(密码字段除外),显示消息 - FirstName 中输入的值:Selenium,LastName 中输入的值:Tutorials,以及UserName 中输入的值:Tutorialspoint。
最后,收到消息Process finished with exit code 0,表示代码成功执行。
示例 2 - 使用 click() 方法
让我们再以下面页面中的表单为例,该表单包含 Web 元素 - 标签、输入框、按钮、密码等等。
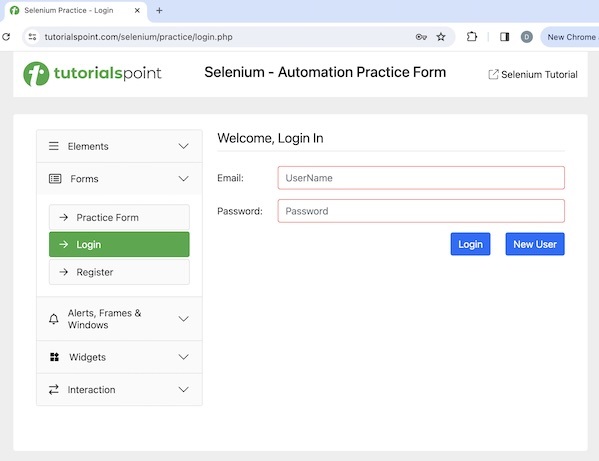
语法
使用 click() 方法的语法:
WebDriver driver = new ChromeDriver(); // identify input box 1 WebElement inputBx = driver.findElement(By.xpath("<value of xpath>")); inputBx.sendKeys("Selenium"); // get value entered System.out.println("Value entered in FirstName: " + inputBx.getAttribute("value")); // identify input box 2 WebElement inputBx2 = driver.findElement(By.xpath("<value of xpath>")); inputBx2.sendKeys("Selenium"); // submit form WebElement btn = driver.findElement(By.xpath("<value of xpath>")); btn.click();
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class FormElement { public static void main(String[] args) { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify form driver.get("https://tutorialspoint.com/selenium/practice/login.php"); // identify input box 1 WebElement inputBx = driver.findElement(By.xpath("//*[@id='email']")); inputBx.sendKeys("Selenium"); // get value entered System.out.println("Value entered in Email: " + inputBx.getAttribute("value")); // identify input box 2 WebElement inputBx2 = driver.findElement(By.xpath("//*[@id='password']")); inputBx2.sendKeys("Tutorials"); // submit form with click() method WebElement btn = driver.findElement(By.xpath("//*[@id='signInForm']/div[3]/input")); btn.click(); // Closing browser driver.quit(); } }
输出
Value entered in Email: Selenium
在上面的示例中,我们填写了包含输入框的表单,然后在控制台中获取输入的值(密码字段除外),显示消息 - Email 中输入的值:Selenium。
结论
本教程全面介绍了 Selenium WebDriver 表单处理。我们从描述如何在网页上识别表单开始,并逐步讲解了如何使用 Selenium Webdriver 处理表单的示例。这使您能够深入了解 Selenium WebDriver 表单处理。最好继续练习您学到的知识,并探索其他与 Selenium 相关的知识,以加深您的理解并拓宽您的视野。