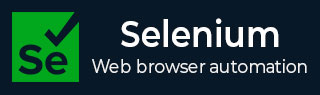
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理Cookie
- Selenium - 日期时间选择器
- Selenium - 动态Web表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理Ajax调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览器上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 与其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 基于关键字的框架
- Selenium - 数据驱动的框架
- Selenium - 混合驱动的框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 浏览器上下文
Selenium Webdriver 可用于浏览器上下文。有多个 API 用于浏览器上下文命令。可以使用浏览器上下文执行的一些操作包括打开新窗口、标签页,利用已打开的窗口,打开 URL 等等。
创建浏览器上下文
为了创建一个浏览器上下文,我们将使用 ChromeOptions 类,然后将 capability webSocketUrl 设置为 true 值,并将它的引用传递给 Webdriver。请注意,我们还需要导入以下语句才能使用浏览器上下文的方法。
import org.openqa.selenium.bidi.browsingcontext.BrowsingContext.
在使用浏览器上下文命令的 API 运行测试时,将打开一个标题为 **BiDi-CDP Mapper** 的标签页,其中包含消息 - **BiDi-CDP Mapper 正在控制此标签页以及调试信息**。这将证明自动化测试正在使用浏览器上下文。
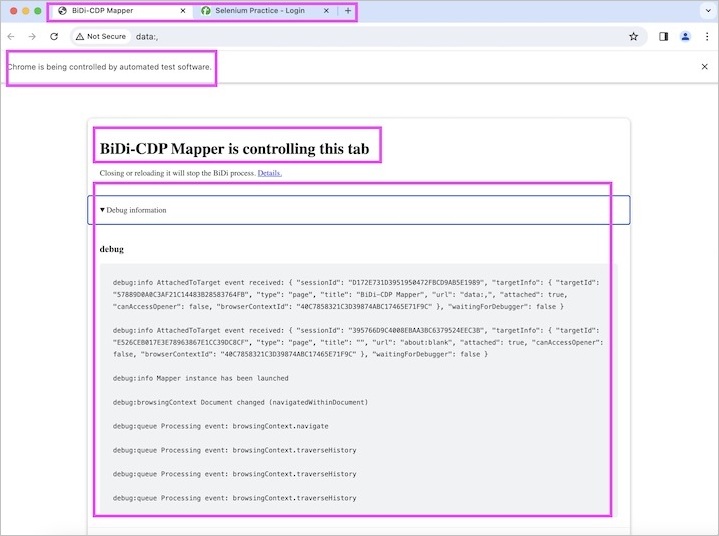
下一个标签页将打开自动化测试触发的应用程序。
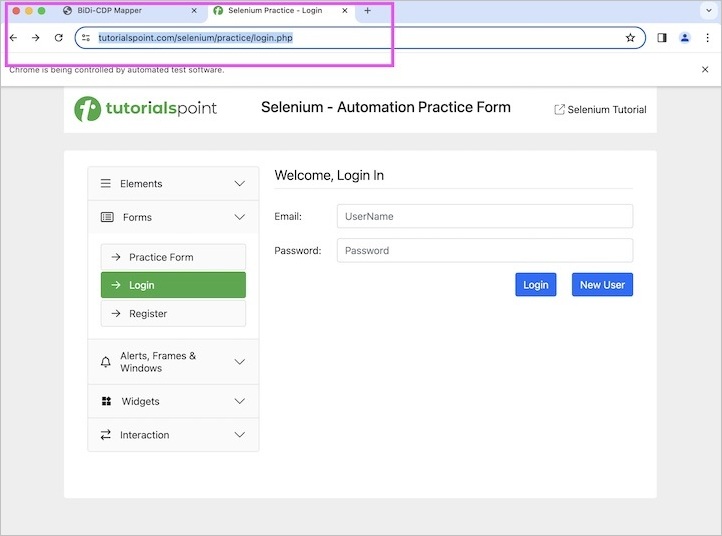
Selenium 中的浏览器上下文是 Selenium 4.x 版本中提供的一项功能。为了利用浏览器上下文命令上的所有 API,建议使用 Selenium 4.16.x 版本的 Selenium Maven 依赖项。
添加到 **pom.xml** 的浏览器上下文依赖项
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.16.1</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
在新窗口中创建浏览器上下文并导航到 URL
让我们来看一个例子,我们将打开一个浏览器,然后打开另一个新窗口并启动一个具有以下 URL 的应用程序以创建浏览器上下文:
https://tutorialspoint.com/selenium/.
**BrowsingContextNewWindows.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewWindows { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new window BrowsingContext bc = new BrowsingContext(driver, WindowType.WINDOW); // obtain id of browsing context in new window String text = bc.getId(); System.out.println("Id of browsing context in new window: " + text); // navigate to new url in the new window NavigationResult i = bc.navigate("https://tutorialspoint.com/selenium/practice/buttons.php"); // get new URL opened in the new window System.out.println("Get URL: " + i.getUrl()); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Id of browsing context in new window: 14CC57B70B48D044482821778B560618 Get URL: https://tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
在上面的示例中,我们打开了一个浏览器,然后打开另一个新窗口并启动了一个具有以下 URL 的应用程序以创建浏览器上下文。我们在控制台中获得了新窗口的浏览器上下文 ID 和启动的 URL,消息为 - **新窗口中浏览器上下文的 ID:14CC57B70B48D044482821778B560618** 和 **获取 URL:** https://tutorialspoint.com/selenium/。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
在新标签页中创建浏览器上下文并导航到 URL
让我们来看一个例子,我们将打开一个浏览器,然后打开另一个新标签页并在那里启动一个具有以下 URL 的应用程序以创建浏览器上下文:https://tutorialspoint.com/selenium/。
**BrowsingContextNewTabs.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTabs { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB); // obtain id of browsing context in new tab String text = bc.getId(); System.out.println("Id of browsing context in new tab: " + text); // navigate to new url in the new tab in readiness state NavigationResult i = bc.navigate("https://tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // get new URL opened in the new tab System.out.println("Get URL: " + i.getUrl()); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Id of browsing context in new tab: E7D9C6C1EA830EE2E968EFFDAE800EE2 Get URL: https://tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
在上面的示例中,我们打开了一个浏览器,然后打开另一个新标签页并启动了一个具有以下 URL 的应用程序以创建浏览器上下文。我们在控制台中获得了新标签页的浏览器上下文 ID 和启动的 URL,消息为 - **新标签页中浏览器上下文的 ID:E7D9C6C1EA830EE2E968EFFDAE800EE2** 和 **获取 URL:** https://tutorialspoint.com/selenium/。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
使用现有窗口/标签页句柄创建浏览器上下文
让我们来看一个例子,我们将使用现有窗口/标签页创建一个浏览器上下文。
**BrowsingContextNewTab.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTab { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // obtain id of browsing context in new tab/window String text = bc.getId(); System.out.println("Id of browsing context in new tab/window: " + text); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Id of browsing context in new tab/window: E5EB8BA6EBAFBA14561CC8EB23360034 Process finished with exit code 0
在上面的示例中,我们使用现有窗口/标签页句柄创建了浏览器上下文。我们在控制台中获得了浏览器上下文 ID,消息为 - **新窗口/标签页中的浏览器上下文 ID:E5EB8BA6EBAFBA14561CC8EB23360034**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
打开具有参考浏览器上下文的新窗口后创建浏览器上下文
让我们来看一个例子,我们将通过打开一个具有参考浏览器上下文的新窗口来创建一个浏览器上下文。
**BrowsingContextNewWindow.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewWindow { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new window using browsing context BrowsingContext bc = new BrowsingContext(driver, WindowType.WINDOW, windowID); // obtain id of browsing context String text = bc.getId(); System.out.println("Id of browsing context in new window: " + text); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Id of browsing context in new window: F82CF86144C26AEA4168F672C11B8C92 Process finished with exit code 0
在上面的示例中,我们在打开具有参考浏览器上下文的新窗口后创建了浏览器上下文。我们在控制台中获得了浏览器上下文 ID,消息为 - **窗口中浏览器上下文的 ID:F82CF86144C26AEA4168F672C11B8C92**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
打开具有参考浏览器上下文的新标签页后创建浏览器上下文
让我们来看一个例子,我们将通过打开一个具有参考浏览器上下文的新标签页来创建一个浏览器上下文。
**BrowsingContextNewTab.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextNewTab { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab using browsing context BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB, windowID); // obtain id of browsing context String text = bc.getId(); System.out.println("Id of browsing context in new tab: " + text); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Id of browsing context in new tab: C88F54A24AF3C6DE71C5B5E37913BA77 Process finished with exit code 0
在上面的示例中,我们在打开具有参考浏览器上下文的新标签页后创建了浏览器上下文。我们在控制台中获得了浏览器上下文 ID,消息为 - **标签页中浏览器上下文的 ID:C88F54A24AF3C6DE71C5B5E37913BA77**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
获取浏览器上下文树
让我们来看一个例子,我们将从父浏览器上下文开始获取每个浏览器上下文。
**BrowsingContextTree.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTree { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // open another URL bc.navigate("https://tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // list of browsing context tree List<BrowsingContextInfo> tree = bc.getTree(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total browsing contexts: " + size); // obtain total children BrowsingContextInfo c = tree.get(0); int size1 = c.getChildren().size(); System.out.println("Total children: " + size1); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Total browsing contexts: 1 Total children: 0 Process finished with exit code 0
获取具有深度值的浏览器上下文树
让我们来看一个例子,我们将从父浏览器上下文开始获取每个浏览器上下文以及深度值。
**BrowsingContextTreeDepth.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTreeDepth { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); System.out.println("Window Handle Id: " + windowID); // open browsing context in new tab/window BrowsingContext bc = new BrowsingContext(driver, windowID); // open another URL bc.navigate("https://tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // list of browsing context tree with depth value List<BrowsingContextInfo> tree = bc.getTree(0); // obtain total browsing context tree int size = tree.size(); System.out.println("Total browsing contexts: " + size); // obtain total children BrowsingContextInfo c = tree.get(0); List children = c.getChildren(); System.out.println("Total children: " + children); System.out.println("Browsing Context id: " + c.getId()); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Window Handle Id: DE5E963CFFCC9A42A5F7A4903BF0C12C Total browsing contexts: 1 Total children: null Browsing Context id: DE5E963CFFCC9A42A5F7A4903BF0C12C Process finished with exit code 0
获取所有顶级浏览器上下文
让我们来看一个例子,我们将获取所有顶级浏览器上下文。
**BrowsingContextTopLevel.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTopLevel { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context in new tab/window BrowsingContext bc1 = new BrowsingContext(driver, windowID); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context: " + size); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Total top level browsing context: 2 Process finished with exit code 0
在上面的示例中,我们在控制台中获得了所有顶级浏览器上下文,消息为 - **顶级浏览器上下文总数:2**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
关闭窗口浏览器上下文
让我们来看一个例子,我们将使用 close() 方法关闭窗口浏览器上下文。
**BrowsingContextWindowClose.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextWindowClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new window BrowsingContext bc1 = new BrowsingContext(driver, WindowType.WINDOW); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context before closing: " + size); // close one browsing context bc2.close(); // obtain total browsing context tree after closing one List<BrowsingContextInfo> tree1 = bc1.getTopLevelContexts(); int size1 = tree1.size(); System.out.println("Total top level browsing context after closing: " + size1); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Total top level browsing context before closing: 3 Total top level browsing context after closing: 2 Process finished with exit code 0
关闭标签页浏览器上下文
让我们来看一个例子,我们将使用 close() 方法关闭标签页浏览器上下文。
**BrowsingContextTabClose.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.BrowsingContextInfo; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.List; import java.util.concurrent.TimeUnit; public class BrowsingContextTabClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc1 = new BrowsingContext(driver, WindowType.TAB); // open another browsing context in new tab BrowsingContext bc2 = new BrowsingContext(driver, WindowType.TAB); // list of every top level browsing contexts List<BrowsingContextInfo> tree = bc1.getTopLevelContexts(); // obtain total browsing context tree int size = tree.size(); System.out.println("Total top level browsing context before closing: " + size); // close one browsing context bc2.close(); // obtain total browsing context tree after closing one List<BrowsingContextInfo> tree1 = bc1.getTopLevelContexts(); int size1 = tree1.size(); System.out.println("Total top level browsing context after closing: " + size1); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Total top level browsing context before closing: 3 Total top level browsing context after closing: 2 Process finished with exit code 0
激活浏览器上下文
让我们来看一个例子,我们将使用 activate() 方法激活浏览器上下文。
**BrowsingContextTabClose.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextTabClose { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // get window handle id String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc1 = new BrowsingContext(driver, windowID); // open another browsing context in new window BrowsingContext bc2 = new BrowsingContext(driver, WindowType.WINDOW); // activate browsing context bc1.activate(); // Quitting browser driver.quit(); } }
重新加载浏览器上下文
让我们来看一个例子,我们将重新加载浏览器上下文并获取导航 ID 和 URL。
**BrowsingContextReload.java** 中的代码实现
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WindowType; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.NavigationResult; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextReload { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // open browsing context in new tab BrowsingContext bc = new BrowsingContext(driver, WindowType.TAB); // navigate to URL in a browsing context bc.navigate("https://tutorialspoint.com/selenium/practice/buttons.php", ReadinessState.COMPLETE); // reload browsing context NavigationResult load = bc.reload(ReadinessState.INTERACTIVE); // get navigation id String text = load.getNavigationId(); System.out.println("Navigation ID: " + text); // get URL opened in browsing context String url = load.getUrl(); System.out.println("Navigation URL: " + url); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Navigation ID: 0C12BD5C26E08392DB18F6C7928F8020 Navigation URL: https://tutorialspoint.com/selenium/practice/buttons.php Process finished with exit code 0
在上面的示例中,我们重新加载了浏览器上下文,并在控制台中获得了导航 ID 和 URL,消息为 - **导航 ID:0C12BD5C26E08392DB18F6C7928F8020** 和 **导航 URL:** https://tutorialspoint.com/selenium/。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
使用浏览上下文处理用户提示
让我们来看一个使用handleUserPrompt()方法通过浏览上下文处理用户提示的例子。
代码实现位于BrowsingContextHandlePrompt.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextHandlePrompt { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, driver.getWindowHandle()); // identify button WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); btn.click(); // accept and input text in prompt bc.handleUserPrompt(true, "Tutorialspoint"); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Process finished with exit code 0
在上面的例子中,我们已经使用浏览上下文处理了用户提示。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码已成功执行。
使用浏览上下文捕获屏幕截图
让我们来看一个使用captureScreenshot()方法通过浏览上下文捕获屏幕截图的例子。
代码实现位于BrowsingContextCaptureScreenshot.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextCaptureScreenshot { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, driver.getWindowHandle()); // identify button then click WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); btn.click(); // capture screenshot String sc = bc.captureScreenshot(); // get number of screenshot int size = sc.length(); System.out.println("Size of Screenshot: " + size); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Size of Screenshots: 209152 Process finished with exit code 0
使用浏览上下文捕获元素的屏幕截图
让我们来看一个使用captureElementScreenshot()方法通过浏览上下文捕获元素屏幕截图的例子。
代码实现位于BrowsingContextElemntCaptureScreenshot.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.remote.RemoteWebElement; import java.util.concurrent.TimeUnit; public class BrowsingContextElemntCaptureScreenshot { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // identify button WebElement btn = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/div[1]/button")); String sc = bc.captureElementScreenshot(((RemoteWebElement) btn).getId()); // get number of screenshot int size = sc.length(); System.out.println("Size of Screenshot of an element: " + size); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Size of Screenshot of an element: 3080 Process finished with exit code 0
使用浏览上下文设置视口像素
让我们来看一个使用setViewport()方法通过浏览上下文设置视口像素的例子。
代码实现位于SetViewPort.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class SetViewPort { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // set view port bc.setViewport(200, 600, 6); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Process finished with exit code 0
使用浏览上下文打印页面
让我们来看一个使用print()方法通过浏览上下文打印页面的例子。
代码实现位于PrintPage.java
package org.example; import org.openqa.selenium.WebDriver; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.print.PrintOptions; import java.util.concurrent.TimeUnit; public class PrintPage { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // launching a browser and open a URL driver.get("https://tutorialspoint.com/selenium/practice/alerts.php"); // object of PrintOptions PrintOptions p = new PrintOptions(); // get print String print = bc.print(p); // Quitting browser driver.quit(); } }
使用浏览上下文在浏览器历史记录中导航、前进、后退和遍历
让我们来看一个使用浏览上下文在浏览器历史记录中前进、后退和遍历的例子。这些操作是使用BrowsingContext类的forward()、back()和traverse(-1)方法执行的。
代码实现位于BrowsingContextHistory.java
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.bidi.browsingcontext.BrowsingContext; import org.openqa.selenium.bidi.browsingcontext.ReadinessState; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import java.util.concurrent.TimeUnit; public class BrowsingContextHistory { public static void main(String[] args) throws InterruptedException { // object of ChromeOptions ChromeOptions opt = new ChromeOptions(); opt.setCapability("webSocketUrl", true); // Initiate the Webdriver WebDriver driver = new ChromeDriver(opt); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // get window handle String windowID = driver.getWindowHandle(); // open browsing context BrowsingContext bc = new BrowsingContext(driver, windowID); // navigate to URL bc.navigate("https://tutorialspoint.com/selenium/practice/login.php", ReadinessState.COMPLETE); // identify element then click WebElement btn = driver.findElement(By.xpath("//*[@id='signInForm']/div[3]/a")); btn.click(); // get page title after click String title = driver.getTitle(); System.out.println("Page title after click: " + title); // navigate back in browser history bc.back(); // get page title after navigating back in browser history String title1 = driver.getTitle(); System.out.println("Page title after navigating back in browser history: " + title1); // navigate forward in browser history bc.forward(); // get page title after navigating forward in browser history String title2 = driver.getTitle(); System.out.println("Page title after navigating forward in browser history: " + title2); // traverse history bc.traverseHistory(-1); // Quitting browser driver.quit(); } }
它将显示以下 **输出**:
Page title after click: Selenium Practice - Register Page title after navigating back in browser history: Selenium Practice - Login Page title after navigating forward in browser history: Selenium Practice - Register Process finished with exit code 0
在上面的例子中,我们启动了一个应用程序,并在浏览器历史记录中进行了后退和前进导航,并在控制台中获取了导航后的浏览器标题,消息为:点击后页面标题:Selenium Practice - Register,浏览器历史记录后退后页面标题:Selenium Practice - Login,以及浏览器历史记录前进后页面标题:Selenium Practice - Register。
本教程到此结束,我们全面介绍了Selenium WebDriver - 浏览器上下文。我们首先描述了什么是浏览器上下文以及浏览器上下文所需的Selenium依赖项,然后逐步讲解了在新的窗口和标签页中创建浏览器上下文、使用现有窗口句柄在新的窗口和标签页中创建浏览器上下文、在正常状态和就绪状态下导航到URL、获取浏览器上下文树、其深度和所有顶级浏览器上下文、关闭标签页或窗口、激活和重新加载浏览器上下文、处理用户提示、捕获网页和特定元素的屏幕截图、设置视口像素、打印页面以及使用Selenium在浏览器历史记录中前进、后退和遍历的示例。
这使您具备了Selenium WebDriver - 浏览器上下文的深入知识。最好继续练习您学到的知识,并探索与Selenium相关的其他知识,以加深您的理解并拓宽您的视野。