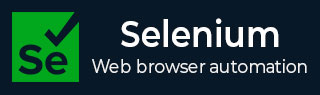
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 Iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊按键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 处理特殊按键
Selenium Webdriver 可用于在创建自动化测试时处理特殊按键。这可以通过使用Actions 类和 Selenium 中的 sendKeys() 方法来实现。使用 keyUp() 和 keyDown() 方法进行键上/下操作主要用于处理特殊按键。如果我们使用 sendKeys() 方法,则需要将 Key.chord 作为参数传递给此方法。
示例 1 - 使用特殊按键复制和粘贴
现在让我们讨论如何在网页上执行复制和粘贴操作的元素识别,如下面的图片所示。首先,右键单击下面的网页,然后单击 Chrome 浏览器中的“检查”按钮。要检查源元素和目标元素,请单击 HTML 代码顶部的向左上箭头。
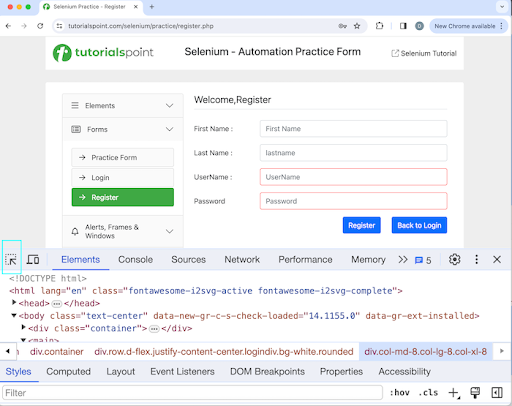
让我们以以下页面为例,我们首先在“全名:”标签旁边输入文本 - JavaSelenium。然后将相同的文本复制并粘贴到“姓氏:”标签旁边的另一个输入框中。
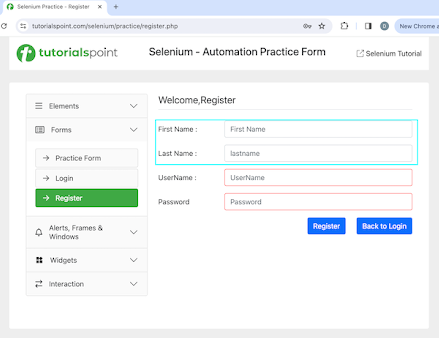
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class CopyPasteAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='firstname']")); // enter some text e.sendKeys("Selenium"); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // object of Actions class to copy then paste Actions a = new Actions(driver); a.keyDown(k); a.sendKeys("a"); a.keyUp(k); a.build().perform(); // Actions class methods to copy text a.keyDown(k); a.sendKeys("c"); a.keyUp(k); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(k); a.sendKeys("v"); a.keyUp(k); a.build().perform(); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='lastname']")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
输出
Value copied and pasted: JavaSelenium Process finished with exit code 0
在上面的示例中,我们首先在第一个输入框中输入了文本JavaSelenium,然后将相同的文本复制并粘贴到第二个输入框中,然后在控制台中获取了第二个输入框中的输入文本作为消息 - 已复制并粘贴的值:JavaSelenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2 - 使用特殊按键复制和粘贴
让我们使用与上面相同的示例,并在不使用 Actions 类的情况下实现相同的操作。
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CopyPaste { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='firstname']")); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='lastname']")); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // enter some text e.sendKeys("JavaSelenium"); // select the whole entered text e.sendKeys(Keys.chord(k, "a")); // copy the whole entered text e.sendKeys(Keys.chord(k, "c")); // tab and reach to next input box e.sendKeys(Keys.TAB); // paste the whole entered text s.sendKeys(Keys.chord(k, "v")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
输出
Value copied and pasted: JavaSelenium
在上面的示例中,我们首先在第一个输入框中输入了文本JavaSelenium,然后使用 sendKeys() 和 Key.chord() 方法将相同的文本复制并粘贴到第二个输入框中。最后,我们在控制台中获取了第二个输入框中的输入文本作为消息 - 已复制并粘贴的值:JavaSelenium。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 3 - 使用特殊按键输入大写文本
让我们再举一个例子,我们将使用特殊按键输入大写字母AUTOMATION。请注意,在将值发送到 sendKeys() 方法时,我们将传递automation并按下 SHIFT 键。
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class KeyAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // Actions class Actions a = new Actions(driver); // moving to an input box and clicking on it a.moveToElement(e).click(); // key UP and DOWN action for SHIFT a.keyDown(Keys.SHIFT); a.sendKeys("automation").keyUp(Keys.SHIFT).build().perform(); // get value entered System.out.println("Text entered: " + e.getAttribute("value")); // Closing browser driver.quit(); } }
输出
Text entered: AUTOMATION Process finished with exit code 0
在上面的示例中,我们输入了文本automation并按下了 SHIFT 键,然后在控制台中获取了大写输入文本以及消息 - 输入的文本:AUTOMATION。
结论
这总结了我们关于 Selenium Webdriver 处理特殊按键教程的全面介绍。我们首先描述了一个使用特殊按键(如 CONTROL、SHIFT、TAB、CONTROL + A、CONTROL + V、CONTROL + C 等)复制和粘贴文本的示例,并说明了如何使用 Selenium 输入大写文本。这使您深入了解了在 Selenium Webdriver 中处理特殊按键的方法。明智的做法是不断练习您所学到的知识,并探索其他与 Selenium 相关的知识,以加深您的理解并扩展您的视野。