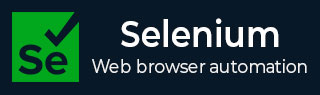
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理Cookie
- Selenium - 日期时间选择器
- Selenium - 动态Web表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理Ajax调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 与其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - JavaScript 执行器
Selenium WebDriver 用于运行 JavaScript 命令来与页面上浏览器中显示的元素进行通信。这是借助 JavaScriptExecutor 接口实现的。有时,Selenium WebDriver 中可用的定位器无法与页面上的元素交互,在这种情况下,我们可以借助 JavaScriptExecutor 方法。
运行 JavaScript 命令的基本方法
JavaScriptExecutor 接口中有多种可用方法。它们列在下面:
executeScript 方法
此方法用于在当前活动的窗口或框架中执行 JavaScript。它是一个匿名函数,支持各种数据类型,例如 WebElement、Long、String、List 和 Boolean。
executeAsyncScript 方法
这是一种多线程方法,用于在当前活动的窗口或框架中执行单个 JavaScript 任务。它允许页面解析继续进行,从而优化性能并提供更大的灵活性。此方法能够以最佳方式执行异步 JavaScript。
执行 JavaScript 命令的步骤
使用 JavaScriptExecutor 接口结合 Selenium WebDriver 执行 JavaScript 命令的步骤列在下面:
步骤 1 - 导入 JavaScriptExecutor 的包。
步骤 2 - 创建 JavaScriptExecutor 接口的引用变量。
步骤 3 - 调用 JavaScriptExecutor 方法。
语法
import org.openqa.selenium.JavascriptExecutor; JavascriptExecutor j = (JavascriptExecutor) driver; j.executeScript(script, args);
示例
让我们以以下页面为例,我们将在其中打开一个带有 URL 的应用程序:Selenium 自动化实践表单。
然后,获取文本:Selenium - 自动化实践表单,以及域为www.tutorialspoint.com。接下来,我们将在名称标签旁边的输入框中输入文本Selenium。
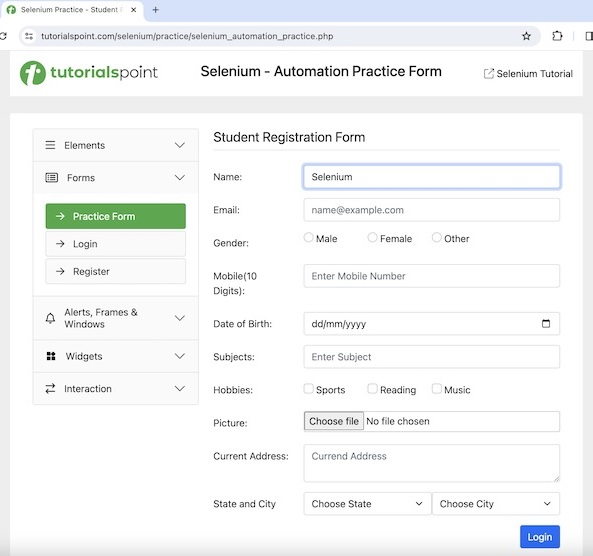
然后,我们将单击登录,因此我们将导航到一个新页面,该页面包含文本欢迎,登录中。我们将刷新浏览器,然后获取文本、URL 和域,分别为欢迎,登录中、Selenium 自动化实践表单和www.tutorialspoint.com。
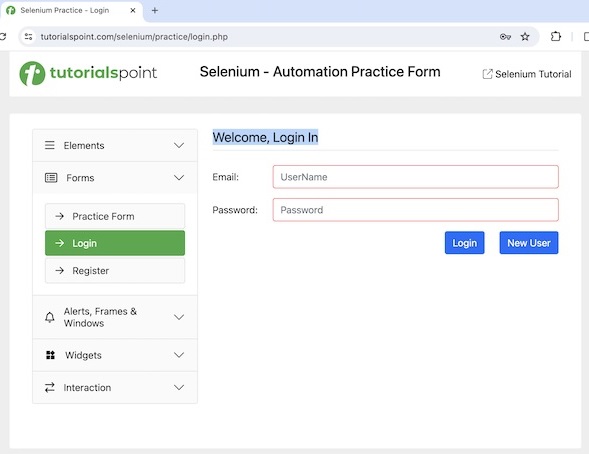
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class JavaScriptsExe { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 25 secs driver.manage().timeouts().implicitlyWait(25, TimeUnit.SECONDS); // Creating a reference variable JavaScriptExecutor interface. JavascriptExecutor j = (JavascriptExecutor) driver; // launching a URL j.executeScript("window.location = 'https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php'"); // getting current URL String url = j.executeScript("return document.URL;").toString(); System.out.println("Get the current URL: " + url); //identify text WebElement t = driver.findElement(By.xpath("/html/body/div/header/div[2]/h1")); // get text String text = (String) j.executeScript("return arguments[0].innerText", t); System.out.println("Text is: " + text); // getting current domain String domain = j.executeScript("return document.domain;").toString(); System.out.println("Get the current domain: " + domain); // enter text in input box WebElement e = driver.findElement(By.xpath("//*[@id='name']")); j.executeScript("arguments[0].value='Selenium';", e); // get text entered String text1 = (String) j.executeScript("return arguments[0].value", e); System.out.println("Entered text is: " + text1); // perform click WebElement b = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); j.executeScript("arguments[0].click();", b); //identify text WebElement w = driver.findElement(By.xpath("//*[@id='signInForm']/h1")); // get text after click String text2 = (String) j.executeScript("return arguments[0].innerText", w); System.out.println("Text found after clicking is: " + text2); // refresh browser j.executeScript("history.go(0)"); // getting current URL after browser refresh String url1 = j.executeScript("return document.URL;").toString(); System.out.println("Get the current URL after browser refresh: " + url1); //identify text again after refresh WebElement y = driver.findElement(By.xpath("//*[@id='signInForm']/h1")); // get text after refresh String text3 = (String) j.executeScript("return arguments[0].innerText", y); System.out.println("Text found after refresh is: " + text3); // getting current domain after browser refresh String domain1 = j.executeScript("return document.domain;").toString(); System.out.println("Get the current domain after browser refresh: " + domain1); // Quit browser driver.quit(); } }
输出
Get the current URL: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Text is: Selenium - Automation Practice Form Get the current domain: www.tutorialspoint.com Entered text is: Selenium Text found after clicking is: Welcome, Login In Get the current URL after browser refresh: https://tutorialspoint.com/selenium/practice/login.php Text found after refresh is: Welcome, Login In Get the current domain after browser refresh: www.tutorialspoint.com Process finished with exit code 0
在上面的示例中,我们启动了一个 URL 并获取了当前 URL,控制台中的消息为:获取当前 URL:Selenium 自动化实践表单
然后检索文本和域,控制台中的消息分别为:文本为:Selenium - 自动化实践表单和获取当前域:www.tutorialspoint.com。
接下来,我们在输入框中输入文本 Selenium 并检索其值,控制台中的消息为:输入的文本为:Selenium。然后,我们单击“登录”链接,并在导航后获取文本,控制台中的消息为:单击后找到的文本:欢迎,登录中。最后,我们刷新了页面并获取了页面的当前 URL、文本和域,控制台中的消息为:浏览器刷新后获取当前 URL:Selenium 有用资源
刷新后找到的文本:欢迎,登录中,以及浏览器刷新后获取当前域:www.tutorialspoint.com。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
Learn JavaScript in-depth with real-world projects through our JavaScript certification course. Enroll and become a certified expert to boost your career.
示例 - 使用 JavaScriptExecutor 向下滚动
现在让我们讨论如何使用 scrollTo 方法向下滚动到网页底部,并获取该页面底部显示的按钮登录,如下图所示。scrollTo 方法采用两个参数 - 水平和垂直顶点。
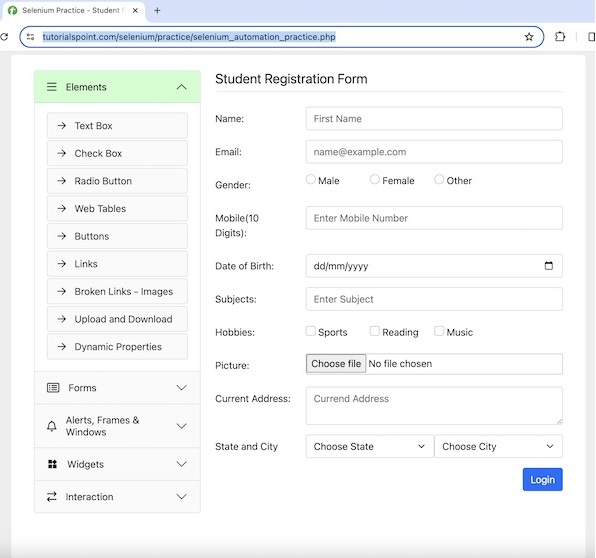
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class PageDownsScroll { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS); //Opening the webpage where we will perform the scroll driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // JavascriptExecutor to scrolling to page bottom JavascriptExecutor javascriptExecutor = (JavascriptExecutor) driver; javascriptExecutor.executeScript("window.scrollBy(0,document.body.scrollHeight)"); // access element at page bottom after scrolling WebElement w = driver.findElement(By.xpath("//*[@id='practiceForm']/div[11]/input")); System.out.println("Verify element presence after scroll down: " + w.isDisplayed()); // quit the browser driver.quit(); } }
输出
Verify element presence after scroll down: true
要获取有关使用 Selenium WebDriver 执行滚动操作的更多信息,请参考链接Selenium WebDriver 滚动操作。
示例 - 使用 JavaScriptExecutor 创建警报
让我们以以下页面为例,我们将在其中使用 JavaScriptExecutor 在网页上创建警报并获取文本Tutorialspoint。
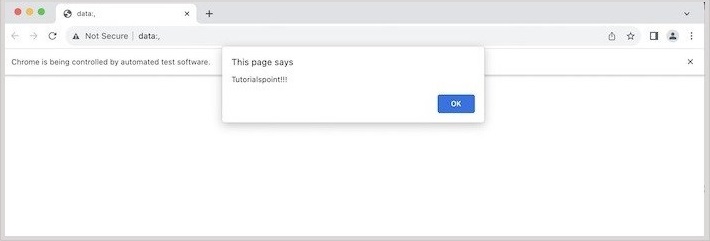
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; public class JavaScriptAlert { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Creating a reference variable JavaScriptExecutor interface JavascriptExecutor j = (JavascriptExecutor) driver; // create an Alert j.executeScript("alert('Tutorialspoint!!!');"); // switch driver context to alert Alert alrt = driver.switchTo().alert(); // Get alert text String s = alrt.getText(); System.out.println("Alert text is: " + s); // Quit browser driver.quit(); } }
输出
Alert text is: Tutorialspoint!!!
在上面的示例中,我们创建了一个警报并获取了它的文本,控制台中的消息为:警报文本为:Tutorialspoint!!!
示例 - 使用 JavaScriptExecutor 选择复选框
让我们再举一个以下页面的例子,我们将使用 JavaScript Executor 选中复选框以选择主级别 2选项:
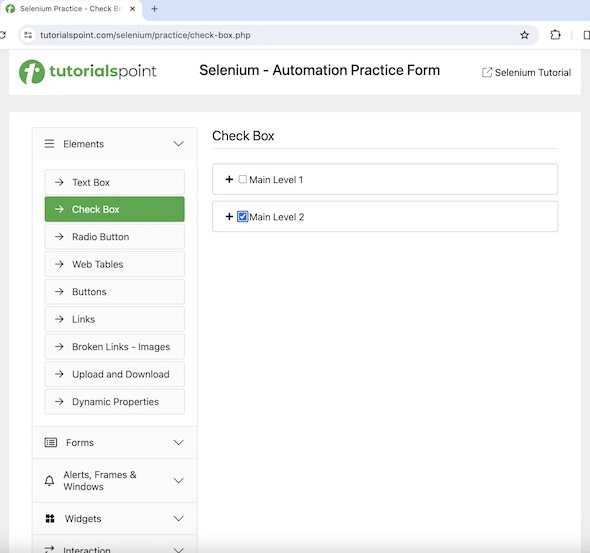
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.JavascriptExecutor; public class JavaScriptHandlesCheckbox { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // Creating a reference variable JavaScriptExecutor interface JavascriptExecutor j = (JavascriptExecutor) driver; // Opening the webpage where we will check checkbox driver.get("https://tutorialspoint.com/selenium/practice/check-box.php"); // identify checkbox with xpath WebElement chkbox = driver.findElement(By.xpath("//*[@id='c_bs_2']")); // check the checkbox j.executeScript("document.getElementById('c_bs_2').checked=true;"); // check if checkbox is selected System.out.println("Checkbox is selected: " + chkbox.isSelected()); // Quitting browser driver.quit(); } }
输出
Checkbox is selected: true
在上面的示例中,我们选中了一个复选框并验证了它是否被选中,控制台中的消息为:复选框已选中:true
结论
这总结了我们关于 Selenium WebDriver JavaScript 执行器教程的全面内容。我们从描述运行 JavaScript 命令的基本方法、执行 JavaScript 命令的步骤以及示例来说明如何在 Selenium WebDriver 中处理 JavaScript 执行器开始。这使您能够深入了解 Selenium WebDriver JavaScript 执行器。明智的做法是继续练习您所学的内容并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。