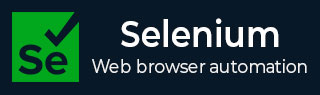
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver 与 RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键点击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 与其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 选择支持
Selenium WebDriver 可以使用 Select 类来选择网页上下拉框中的选项。网页上可能有两种类型的下拉框 - 单选(允许选择一个选项)和多选(允许选择多个选项)。
下拉框由称为 select 的标签名称识别。此外,其每个选项都具有称为 option 的标签名称。此外,多选下拉框包含一个属性 multiple。
HTML 中的下拉框识别
右键点击网页,然后点击 Chrome 浏览器中的“检查”按钮。然后,整个页面的相应 HTML 代码将可见。为了检查网页上的下拉框,我们点击左侧向上的箭头,该箭头位于可见的 HTML 代码顶部,如下所示。
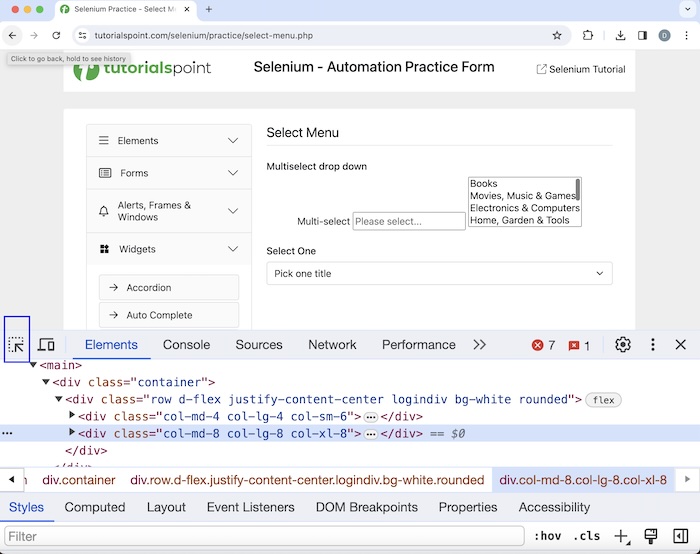
点击后,将箭头指向文本选择一个旁边的下拉框,其 HTML 代码可见,反映了 select 标签名称(括在 <> 中),以及 option 标签名称内的选项。
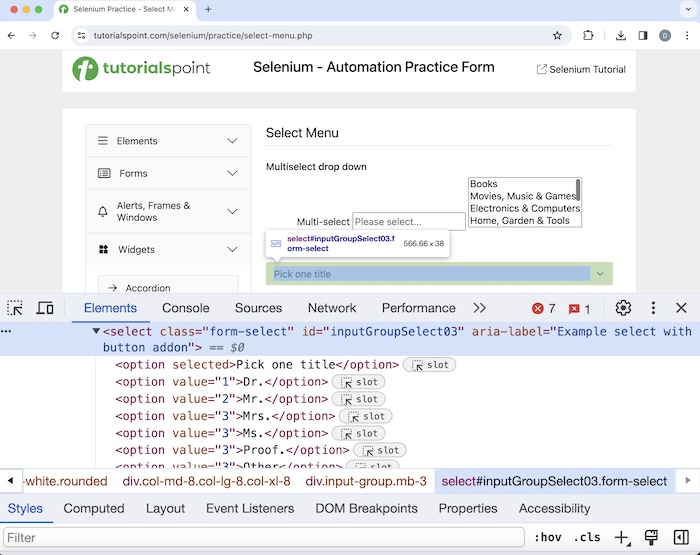
请注意,其中一个选项选择一个标题具有属性 selected,这意味着默认情况下会选择此选项,即使在选择任何选项之前也是如此。此外,如果下拉列表包含一个属性值 disabled,则该选项将无法选择。
基本选择方法
Selenium WebDriver 的 Select 类中提供了多种方法,可帮助我们处理多选和单选下拉框。
示例 1 - 单选下拉框
让我们以以下页面为例,我们将访问文本选择一个下方的下拉框,选择值其他,并使用上面讨论的方法执行一些验证。
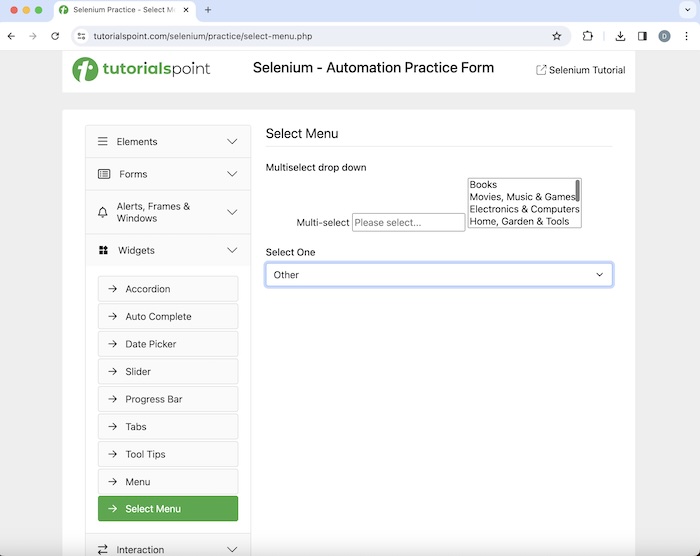
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify dropdown then select its options by index WebElement dropdown = driver.findElement (By.xpath("//*[@id='inputGroupSelect03']")); Select select = new Select(dropdown); // get option selected by default WebElement o = select.getFirstSelectedOption(); System.out.println("Option selected by default: " + o.getText()); // select an option by index select.selectByIndex(6); // get selected option List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Option is: " + opt.getText()); } // get all options of dropdown List<WebElement> options =select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // check if multiselect dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for checking is: "+ b); // quitting browser driver.quit(); } }
输出
Option selected by default: Pick one title Selected Option is: Other Options are: Pick one title Options are: Dr. Options are: Mr. Options are: Mrs. Options are: Ms. Options are: Proof. Options are: Other Boolean value for checking is: false Process finished with exit code 0
在上面的示例中,我们通过控制台中的消息获取了下拉框中选定的选项 - 选定的选项是:其他。然后获取下拉框的所有选项,控制台中的消息为 -选项是:选择一个标题,选项是:博士,选项是:非洲,选项是:先生,选项是:女士,选项是:小姐,选项是:证明,和选项是:其他。
我们还验证了下拉框没有多个选择选项,控制台中的消息为 - 检查的布尔值为:false。我们还检索了下拉框中默认选定的选项,控制台中的消息为 - 默认选定的选项:选择一个标题。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 2 - 多选下拉框
让我们再以以下页面为例,我们将访问文本多选下拉框旁边的多选下拉框,选择值电子产品和电脑和运动和户外,并使用上面讨论的方法执行一些验证。
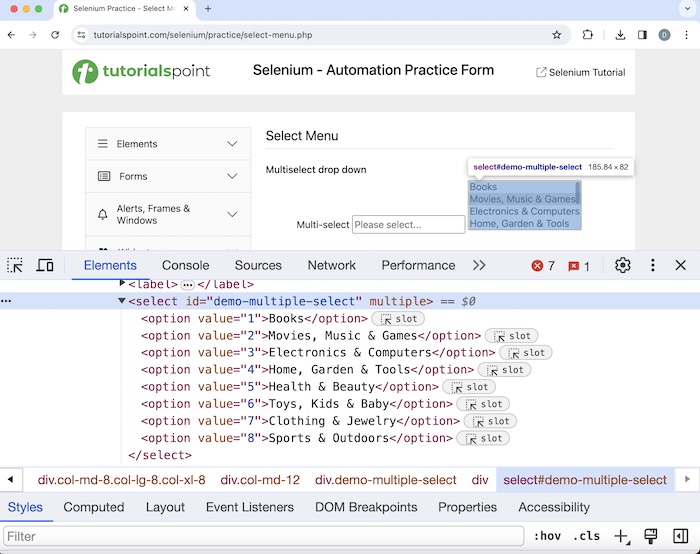
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.List; import java.util.concurrent.TimeUnit; public class SelectMultipleDropdowns { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will get dropdown driver.get("https://tutorialspoint.com/selenium/practice/select-menu.php"); // identify multiple dropdown WebElement dropdown = driver.findElement (By.xpath("//*[@id='demo-multiple-select']")); // object of Select class Select select = new Select(dropdown); // gets options of dropdown in list List<WebElement> options = select.getOptions(); for (WebElement opt : options){ System.out.println("Options are: " + opt.getText()); } // return true if multi-select dropdown Boolean b = select.isMultiple(); System.out.println("Boolean value for multiple dropdown: "+ b); // select item by value select.selectByValue("3"); // select item by index select.selectByIndex(7); // get all selected options of dropdown in list List<WebElement> selectedOptions = select.getAllSelectedOptions(); for (WebElement opt : selectedOptions){ System.out.println("Selected Options are: " + opt.getText()); } // get first selected option in dropdown WebElement f = select.getFirstSelectedOption(); System.out.println("First selected option is: "+ f.getText()); // deselect option by index select.deselectByIndex(7); // deselect all selected items select.deselectAll(); // get all selected options of dropdown after deselected List<WebElement> delectedOptions = select.getAllSelectedOptions(); System.out.println("No. options selected: " + delectedOptions.size()); // Closing browser driver.quit(); } }
输出
Options are: Books Options are: Movies, Music & Games Options are: Electronics & Computers Options are: Home, Garden & Tools Options are: Health & Beauty Options are: Toys, Kids & Baby Options are: Clothing & Jewelry Options are: Sports & Outdoors Boolean value for multiple dropdown: true Selected Options are: Electronics & Computers Selected Options are: Sports & Outdoors First selected option is: Electronics & Computers No. options selected: 0 Process finished with exit code 0
在上面的示例中,我们通过控制台中的消息获取了下拉框的所有选项 - 选项是:书籍,选项是:电影,音乐和游戏,选项是:家居,花园和工具,选项是:健康和美容,选项是:玩具,儿童和婴儿,选项是:服装和珠宝,选项是:运动和户外。
我们还验证了下拉框有多个选择选项,控制台中的消息为 - 检查的布尔值为:true。我们通过控制台中的消息检索了下拉框中选定的选项 - 选定的选项是:电子产品和电脑,选定的选项是:运动和户外。
我们还通过控制台中的消息获取了第一个选定的选项 - 第一个选定的选项是:电子产品和电脑。最后,我们取消选择下拉框中所有选定的选项,因此在控制台中获取了消息 - 已选选项数:0。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
这总结了我们关于 Selenium WebDriver - 选择支持教程的全面内容。我们从描述 HTML 中下拉框的识别、基本选择方法以及示例开始,说明如何在 Selenium WebDriver 中处理单选和多选下拉框。
这使您对 Selenium WebDriver - 选择支持有了深入的了解。明智的做法是不断练习您所学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。