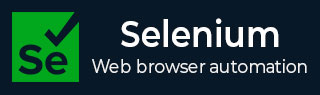
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 发射代码
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver 与 RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键单击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 向上/向下键
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium 和其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 杂项概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium 和 JavaScript 教程
Selenium Webdriver 可用于执行 JavaScript 命令来与网页浏览器中出现的元素的 html 交互。这是使用 JavaScriptExecutor 接口实现的。JavaScript 命令与 Selenium Webdriver 一起使用的最常见示例之一是在网页上执行滚动操作时。
运行 JavaScript 命令的方法
在Selenium JavaScriptExecutor 接口中,有多种方法可用于运行 JavaScript 命令。
语法
import org.openqa.selenium.JavascriptExecutor; JavascriptExecutor js = (JavascriptExecutor) driver; js.executeScript(script, args);
Selenium 中执行 JavaScript 命令的示例
让我们以以下页面为例,我们将使用 URL 启动应用程序:Selenium 自动化实践表单。然后我们将获取文本:学生注册表单,以及域名 www.tutorialspoint.com。接下来,我们将在“姓名:”标签旁边的输入框中输入文本JavaScript。
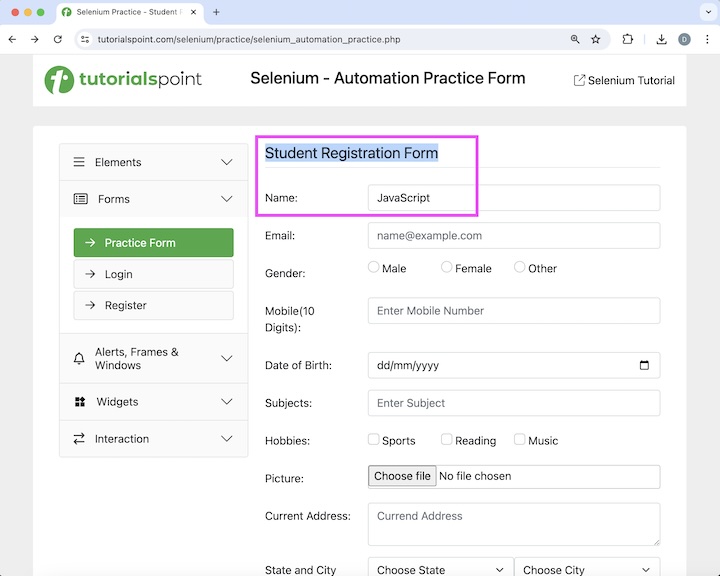
然后,我们将单击“登录”按钮,之后我们将导航到另一个页面,该页面显示文本欢迎,登录。最后,我们将刷新浏览器并再次获取文本、URL 和域名,分别为欢迎,登录、Selenium 自动化实践表单和www.tutorialspoint.com。
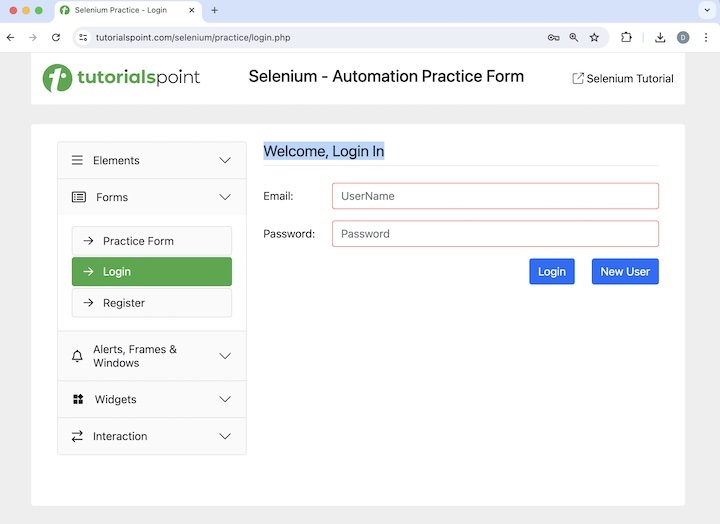
代码实现
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.edge.EdgeDriver; import java.util.concurrent.TimeUnit; public class JavaScriptSelenium { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new EdgeDriver(); // adding implicit wait of 25 secs driver.manage().timeouts().implicitlyWait(25, TimeUnit.SECONDS); // Creating a reference variable JavaScriptExecutor interface. JavascriptExecutor j = (JavascriptExecutor) driver; // launching a URL j.executeScript("window.location ='https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php'"); // getting current URL String url = j.executeScript("return document.URL;").toString(); System.out.println("Getting the current URL: " + url); //identify text WebElement t = driver.findElement(By.xpath("//*[@id='practiceForm']/h1")); String text = (String) j.executeScript("return arguments[0].innerText", t); System.out.println("Text is: " + text); // getting current domain String domain = j.executeScript("return document.domain;").toString(); System.out.println("Getting the current domain: " + domain); // enter text in input box WebElement e = driver.findElement(By.xpath("//*[@id='name']")); j.executeScript("arguments[0].value='JavaScript';", e); // get text entered String text1 = (String) j.executeScript("return arguments[0].value", e); System.out.println("Entered text is: " + text1); // perform click WebElement b = driver.findElement(By.xpath("//*[@id='collapseTwo']/div/ul/li[2]/a")); j.executeScript("arguments[0].click();", b); //identify text WebElement w = driver.findElement(By.xpath("//*[@id='signInForm']/h1")); // get text after click String text2 = (String) j.executeScript("return arguments[0].innerText", w); System.out.println("Text found after clicking: " + text2); // refresh browser j.executeScript("history.go(0)"); // getting current URL after browser refresh String url1 = j.executeScript("return document.URL;").toString(); System.out.println("Getting the current URL after browser refresh: " + url1); //identify text again after refresh WebElement y = driver.findElement(By.xpath("//*[@id='signInForm']/h1")); // get text after refresh String text3 = (String) j.executeScript("return arguments[0].innerText", y); System.out.println("Text found after refresh: " + text3); // getting current domain after browser refresh String domain1 = j.executeScript("return document.domain;").toString(); System.out.println("Getting the current domain after browser refresh: " + domain1); // Quitting browser driver.quit(); } }
输出
Getting the current URL: https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php Text is: Student Registration Form Getting the current domain: www.tutorialspoint.com Entered text is: JavaScript Text found after clicking: Welcome, Login In Getting the current URL after browser refresh: https://tutorialspoint.com/selenium/practice/login.php Text found after refresh: Welcome, Login In Getting the current domain after browser refresh: www.tutorialspoint.com Process finished with exit code 0
在上面的示例中,我们启动了一个 URL 并获取了当前 URL,并在控制台中显示消息 - 获取当前 URL:Selenium 自动化实践表单。然后分别检索了文本和域名,并在控制台中显示消息 - 文本为:学生注册表单和获取当前域名:www.tutorialspoint.com。
接下来,我们在输入框中输入了文本 Selenium 并检索了其值,并在控制台中显示消息 - 输入的文本为:Selenium。然后,我们单击登录链接并在导航后获取文本,并在控制台中显示消息:单击后找到的文本:复选框。
最后,我们刷新了页面并获取了页面的当前 URL、文本和域名,并在控制台中显示消息 - 浏览器刷新后获取当前 URL:Selenium 自动化实践表单、刷新后找到的文本:复选框和浏览器刷新后获取当前域名:www.tutorialspoint.com。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
在本教程中,我们讨论了如何使用 Selenium Webdriver 使用 JavaScript。