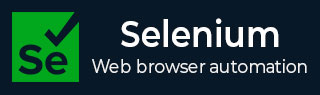
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境设置
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 访问器命令
- Selenium - 断言命令
- Selenium - 断言/验证方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 杂项
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium WebDriver - 日期时间选择器
Selenium Webdriver 可用于从日历中选择日期和时间。Web UI 上可以以多种方式设计日历中的日期时间选择器。根据 UI,需要设计测试用例。
什么是日期时间选择器?
日期时间选择器基本上是在被测应用程序中创建日历的用户界面。日期时间选择器的功能是从一个范围内选择特定的日期和/或时间。
如何检查日期时间选择器?
要识别下图中显示的日历,首先我们会点击“选择日期”字段下的第一个输入框,然后右键点击网页,最后点击 Chrome 浏览器中的“检查”按钮。要调查页面上的日历,请点击 HTML 代码顶部可用的左上箭头,如下所示。
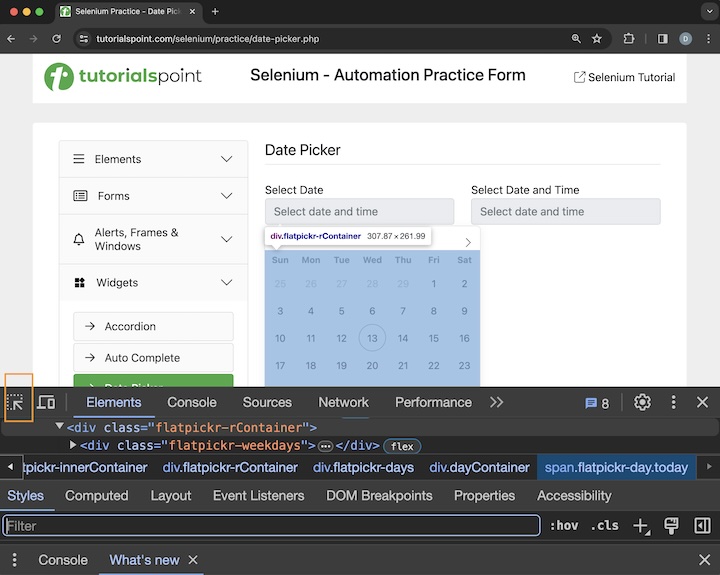
过去日期选择
让我们以下面页面中的日期时间选择器为例,它具有选择年份、月份、日期、小时、分钟等选项。它还包含选择过去或未来日期和时间的选项。让我们选择一个过去的日期和时间 - 上午 2023 年 6 月 4 日 09:05,然后验证它们。
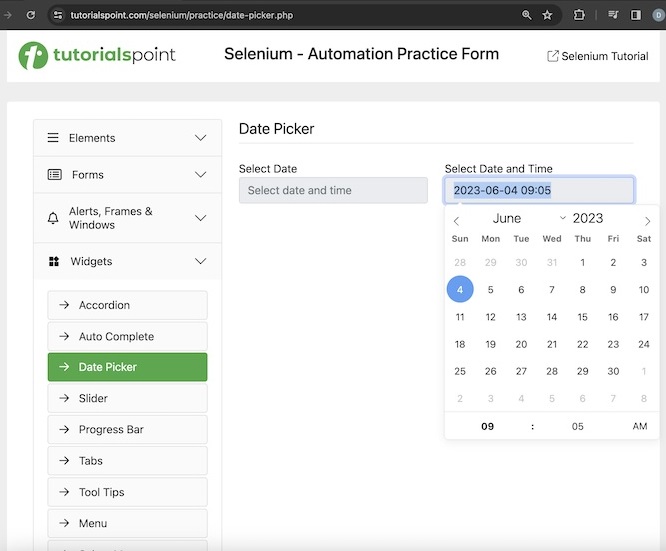
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.concurrent.TimeUnit; public class DatTimePickers { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://tutorialspoint.com/selenium/practice/date-picker.php"); // identify element to get calendar WebElement f = driver .findElement(By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting month June WebElement month = driver .findElement(By.xpath("/html/body/div[3]/div[1]/div/div/select")); Select select = new Select(month); select.selectByVisibleText("June"); // getting selected month String selectedMonth = select.getFirstSelectedOption().getText(); // selecting year 2023 WebElement year = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/div/input")); // removing existing year then entering year.clear(); year.sendKeys("2023"); // selecting day 4 WebElement day = driver.findElement(By.xpath("//span[contains(@aria-label,'"+selectedMonth+" 4')]")); day.click(); // selecting AM time WebElement time = driver.findElement(By.xpath("/html/body/div[3]/div[3]/span[2]")); if (time.getText().equalsIgnoreCase("PM")){ time.click(); } // selecting hour WebElement hour = driver .findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hour.clear(); hour.sendKeys("9"); // selecting minutes WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys("5"); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase("2023-06-04 09:05")){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
输出
Date and time selected by Date Time Picker: 2023-06-04 09:05 Date and Time selected successfully Process finished with exit code 0
在上面的示例中,我们使用日期时间选择器从日历中选择了过去的日期和时间,然后通过消息验证是否选择了正确的日期 - **日期时间选择器选择的日期和时间:2023-06-04 09:05** 和 **日期和时间选择成功**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码成功执行。
未来日期选择
让我们再举一个下面页面中日期时间选择器的例子,我们将选择一个未来的日期和时间 - 晚上 2025 年 6 月 4 日 21:05,然后验证它们。
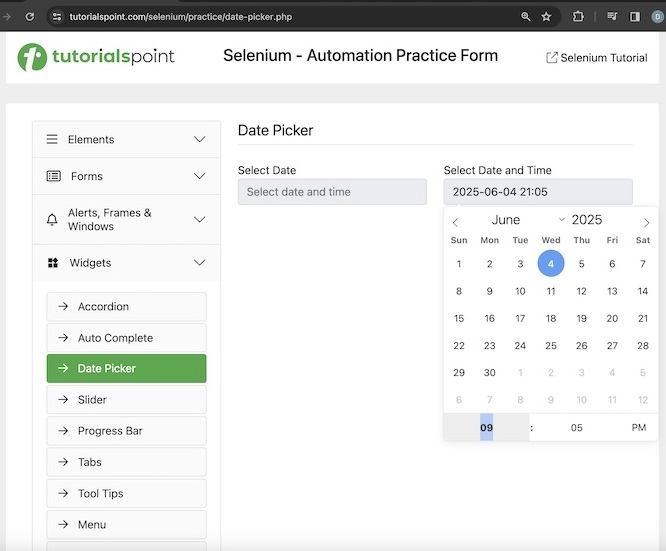
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.Select; import java.util.concurrent.TimeUnit; public class DatTimePickersForward { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://tutorialspoint.com/selenium/practice/date-picker.php"); // identify element to get calendar WebElement f = driver.findElement(By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting month June WebElement month = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/select")); Select select = new Select(month); select.selectByVisibleText("June"); // getting selected month String selectedMonth = select.getFirstSelectedOption().getText(); // selecting year 2023 WebElement year = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/div/input")); // removing existing year then entering year.clear(); year.sendKeys("2025"); // selecting day 4 WebElement day = driver.findElement(By.xpath ("//span[contains(@aria-label,'"+selectedMonth+" 4')]")); day.click(); // selecting PM time WebElement time = driver.findElement(By.xpath("/html/body/div[3]/div[3]/span[2]")); if (time.getText().equalsIgnoreCase("AM")){ time.click(); } // selecting hour WebElement hour = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hour.clear(); hour.sendKeys("9"); // selecting minutes WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys("5"); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase("2025-06-04 21:05")){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
输出
Date and time selected by Date Time Picker: 2025-06-04 21:05 Date and Time selected successfully Process finished with exit code 0
在上面的示例中,我们使用日期时间选择器从日历中选择了未来的日期和时间,然后通过消息验证是否选择了正确的日期 - **日期时间选择器选择的日期和时间:2025-06-04 21:05** 和 **日期和时间选择成功**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码成功执行。
当前日期选择
让我们再举一个下面页面中日期时间选择器的例子,我们将选择一个当前日期的日期和时间,然后验证它们。请注意,在此日期时间选择器中,默认情况下会选择当前日期,我们只需要选择当前时间即可。
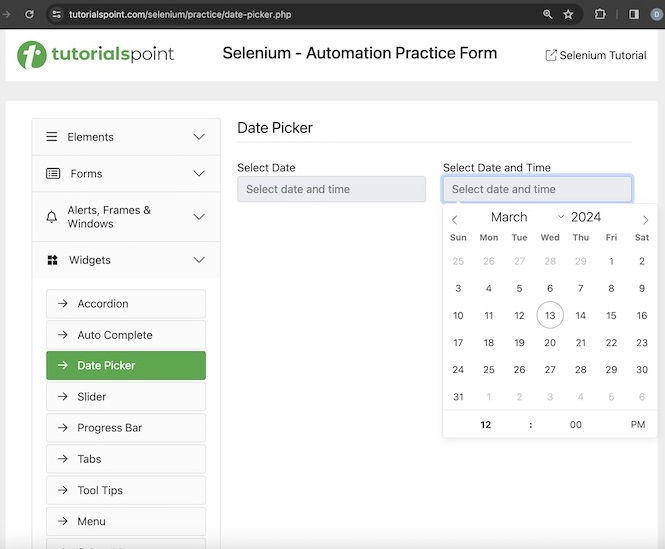
示例
package org.example; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.concurrent.TimeUnit; public class DateTimePickersCurrent { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 20 secs driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS); // URL launch for accessing calendar driver.get("https://tutorialspoint.com/selenium/practice/date-picker.php"); // get current time LocalDateTime l = LocalDateTime.now(); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm"); String dateTime = l.format(formatter); String[] dtTime = dateTime.split(" "); String time = dtTime[1]; String[] t = time.split(":"); String hour = t[0]; String minute = t[1]; // identify element to get calendar WebElement f = driver.findElement(By.xpath("//*[@id='datetimepicker2']")); f.click(); // selecting hour based on current WebElement hours = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input")); // removing existing hour then entering hours.clear(); hours.sendKeys(hour); // selecting minutes based on current minutes WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input")); // removing existing minutes then entering minutes.clear(); minutes.sendKeys(minute); // reflecting both date and time f.click(); // get date and time selected String v = f.getAttribute("value"); System.out.println("Date and time selected by Date Time Picker: " + v); // check date and time selected if (v.equalsIgnoreCase(dateTime)){ System.out.print("Date and Time selected successfully"); } else { System.out.print("Date and Time selected unsuccessfully"); } // close browser driver.quit(); } }
输出
Date and time selected by Date Time Picker: 2024-03-13 13:29 Date and Time selected successfully Process finished with exit code 0
在上面的示例中,我们使用日期时间选择器从日历中选择了当前日期和时间,然后通过消息验证是否选择了正确的日期 - **日期时间选择器选择的日期和时间:2024-03-13 13:29** 和 **日期和时间选择成功**。
最后,收到消息 **进程已完成,退出代码为 0**,表示代码成功执行。
结论
这总结了我们关于 Selenium Webdriver 日期时间选择器教程的全面内容。我们首先描述了什么是日期时间选择器,如何检查它以及一些示例来逐步演示如何使用 Selenium 选择过去、当前和将来的日期和时间。这使您深入了解 Selenium 中的日期时间选择器。明智的做法是不断练习您学到的知识,并探索与 Selenium 相关的其他知识,以加深您的理解并扩展您的视野。