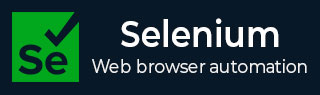
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - 操作命令
- Selenium - 获取器命令
- Selenium - 断言命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右键点击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和选项卡
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 IFrame
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 向上/向下键
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持特性
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 记录和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven & Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
Selenium - TestNG
当我们使用 Selenium 或任何其他工具运行任何自动化测试时,都需要查看和分析执行结果,以得出已执行、通过、失败的测试数量、失败数据等信息,并以报告的形式呈现。
有时,测试失败时捕获的屏幕截图也会包含在报告中。测试报告也需要定期与项目利益相关者共享。为此,我们可以借助 TestNG 报告。
TestNG 报告是在使用 TestNG 构建并运行测试用例后自动生成的 HTML 报告。它是一个单元测试框架,可以与 Selenium 测试集成并用于报告目的。
此外,TestNG 还有一个名为 Reporter 的默认报告类,用于帮助记录日志。这有助于检测故障的根本原因,以调试失败的测试。
创建 TestNG 报告的先决条件
在系统中安装 Java(版本高于 8),并使用命令检查它是否存在:java -version。如果安装已成功完成,则将显示已安装的 Java 版本。
在系统中安装 Maven,并使用命令检查它是否存在:mvn -version。如果安装已成功完成,则将显示已安装的 Maven 版本。
安装任何 IDE,如 Eclipse、IntelliJ 等。
从以下链接添加 TestNG 依赖项:https://mvnrepository.com/artifact/。
从以下链接添加 Selenium Java 依赖项:selenium-java
保存包含所有依赖项的 pom.xml 并更新 Maven 项目
生成 TestNG 报告的不同方法有哪些?
下面列出了生成 TestNG 报告的不同方法:
emailable-report.html
index.html 报告
Reporter 类报告
emailable-report.html
创建 emailable-report.html 的步骤如下:
步骤 1 - 创建一个 TestNG 测试类,其中包含以下示例的实现,我们首先将点击欢迎页面上的新用户按钮。
请注意,我们将通过 testng.xml 文件运行测试。
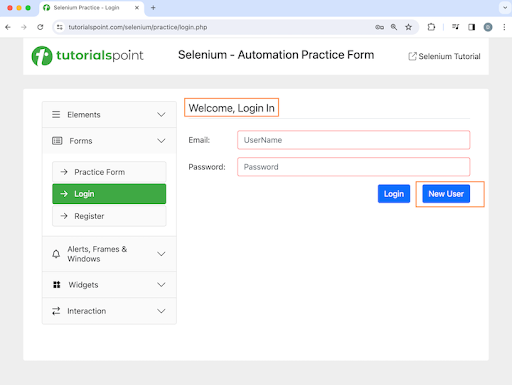
点击新用户按钮后,我们将导航到注册页面,其中包含欢迎,注册文本,如下图所示。
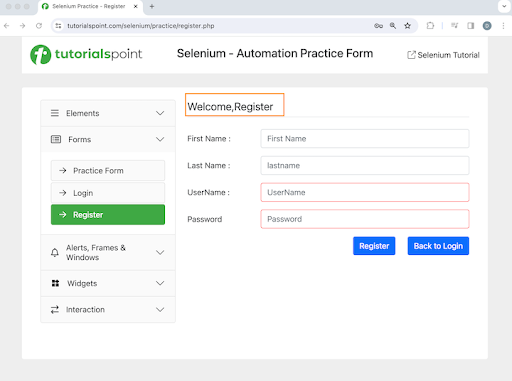
示例
package Report; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.*; import java.util.concurrent.TimeUnit; import static org.testng.Assert.assertEquals; public class TestNGTest { WebDriver driver; @BeforeTest public void setup() throws Exception{ // Initiate browser driver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/login.php"); } @Test(priority = 1) public void verifyWelcomePageHeading() { // identify header then get text WebElement header = driver.findElement (By.xpath("//*[@id='signInForm']/h1")); String text = header.getText(); // assertion to verify login page header assertEquals("Welcome, Login In", text); } @Test(priority = 2) public void moveToRegisterPage() { // identify button then click WebElement btn = driver.findElement (By.xpath("//*[@id='signInForm']/div[3]/a")); btn.click(); } @Test(priority = 3) public void verifyRegisterPageHeading() { // identify header then get text WebElement heder = driver.findElement (By.xpath("//*[@id='signupForm']/h1")); String text = heder.getText(); // assertion to verify register page header assertEquals("Welcome,Register", text); } @AfterTest public void teardown() { // quitting browser driver.quit(); } }
testng.xml 文件中的配置。
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name="All Test Suite"> <test verbose="2" preserve-order="true" name="TestNGTest.java"> <classes> <class name="Report.TestNGTest"> <methods> <include name="verifyWelcomePageHeading"/> <include name="moveToRegisterPage"/> <include name="verifyRegisterPageHeading"/> </methods> </class> </classes> </test> </suite>
pom.xml 文件中的依赖项。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <!-- https://mvnrepository.com/artifact/org.testng/testng --> <dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.9.0</version> <scope>test</scope> </dependency> </dependencies> </project>
上述实现的项目结构如下图所示:
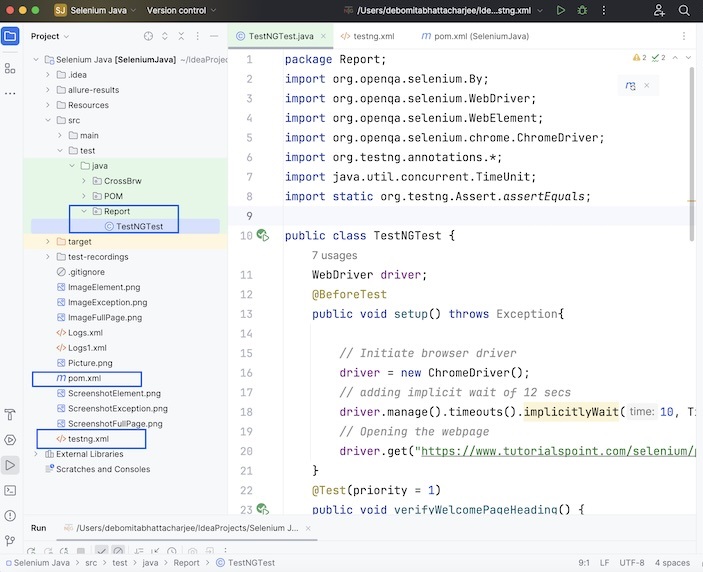
我们将通过 testng.xml 运行测试。
输出
=============================================== All Test Suite Total tests run: 3, Passes: 3, Failures: 0, Skips: 0 =============================================== Process finished with exit code 0
我们借助 TestNG 测试框架创建了测试,并检索了页面标题并使用断言对其进行了验证。
控制台中的结果显示总测试运行次数:3,因为有三个带有 @Test 注释的方法 - verifyWelcomePageHeading()、moveToRegisterPage() 和 verifyRegisterPageHeading()。
最后,收到消息通过:3和进程已完成,退出代码为 0,表示代码已成功执行。
步骤 2 - 刷新项目,项目结构中应该会生成一个名为test-output的新文件夹。
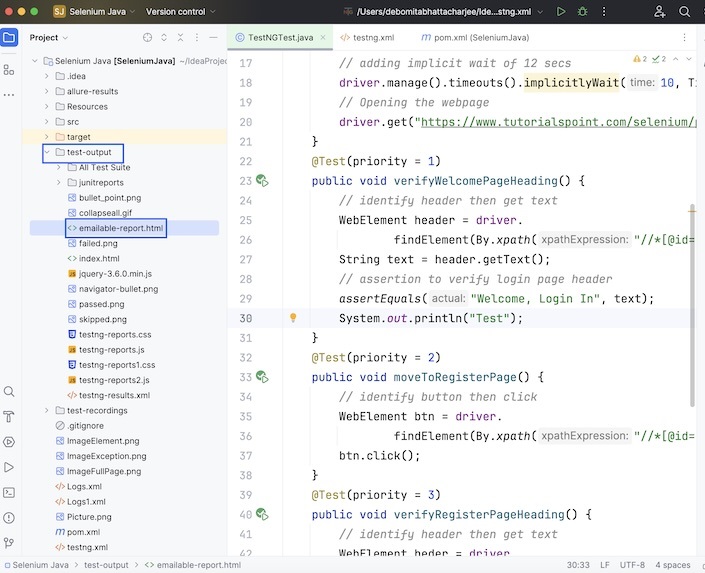
步骤 3 - 右键点击emailable-report.html,然后选择在浏览器中打开的选项。
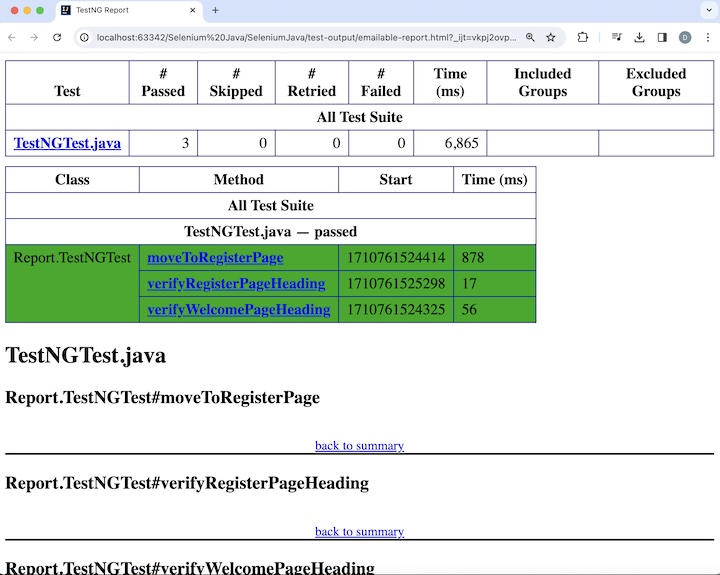
该报告将在浏览器中打开,显示测试类名称 - TestNGTest.java,以及通过、跳过、失败的总数、测试持续时间等。此外,报告中还包含测试方法名称 moveToRegisterPage、verifyRegisterPageHeading 和 verifyWelcomePageHeading。
index.html 报告
创建 index.html 报告的步骤如下:
步骤 1 - 按照创建 emailable-report.html 所述步骤的步骤 1 进行操作。
步骤 2 - 刷新项目,项目结构中应该会生成一个名为test-output的新文件夹。
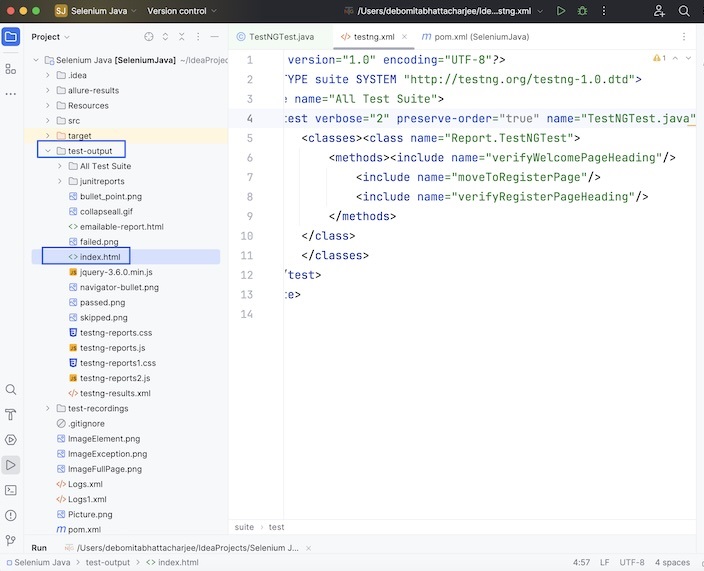
步骤 3 - 右键点击index.html,然后选择在浏览器中打开的选项。
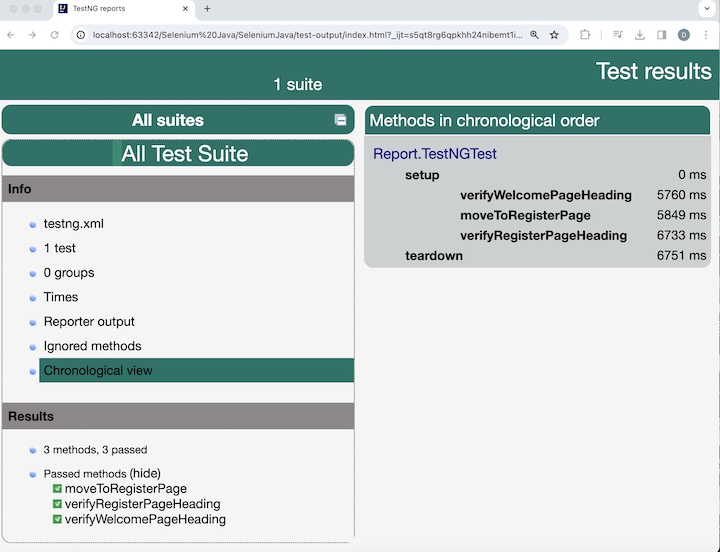
该报告将在浏览器中打开,显示 testng.xml、测试数量、组、时间、报告程序输出、忽略的方法和按时间顺序查看。此外,“结果”部分包含测试方法名称 moveToRegisterPage、verifyRegisterPageHeading 和 verifyWelcomePageHeading,以及方法数量、通过和失败的数量。
从 Reporter 类生成的报告
TestNG 提供了 Reporter 类用于记录日志。它有四种不同的方法来处理要记录的信息:
Reporter.log( String str);
Reporter.log( String str, Boolean logToOut);
Reporter.log( String str, int l);
Reporter.log( String str, int l, Boolean logToOut);
在报告中使用 Reporter 类生成日志消息的步骤如下:
步骤 1 - 创建一个 TestNG 测试类,其中包含以下示例的实现,我们首先将点击欢迎页面上的新用户按钮。
请注意,我们将通过 testng.xml 文件运行测试。此外,测试执行完成后,报告中将包含以下日志消息:
正在转到注册页面
已验证登录页面标题
已验证注册页面标题
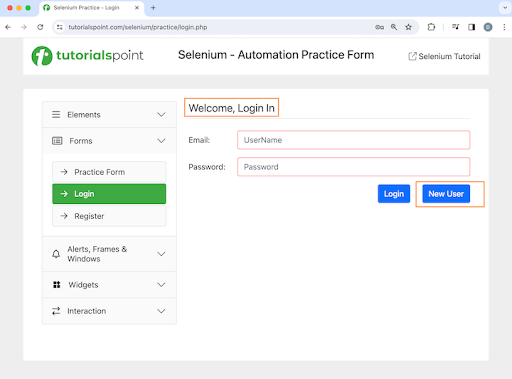
点击新用户按钮后,我们将导航到注册页面,其中包含欢迎,注册文本,如下图所示。
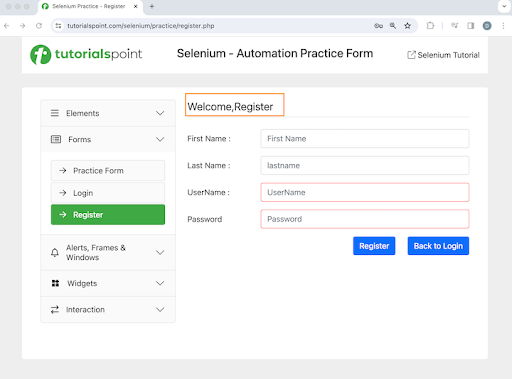
请注意,我们将通过 testng.xml 文件运行测试。此外,测试执行完成后,报告中将包含以下日志消息:
正在转到注册页面
已验证登录页面标题
已验证注册页面标题
示例
package Report; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.Reporter; import org.testng.annotations.*; import java.util.concurrent.TimeUnit; import static org.testng.Assert.assertEquals; public class TestNGTest { WebDriver driver; @BeforeTest public void setup() throws Exception{ // Initiate browser driver driver = new ChromeDriver(); // adding implicit wait of 12 secs driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Opening the webpage driver.get("https://tutorialspoint.com/selenium/practice/login.php"); } @Test(priority = 1) public void verifyWelcomePageHeading() { // identify header then get text WebElement header = driver.findElement(By.xpath("//*[@id='signInForm']/h1")); String text = header.getText(); // assertion to verify login page header assertEquals("Welcome, Login In", text); Reporter.log("Verified Login Page header"); } @Test(priority = 2) public void moveToRegisterPage() { // identify button then click WebElement btn = driver.findElement(By.xpath("//*[@id='signInForm']/div[3]/a")); btn.click(); Reporter.log("Moving to Registration Page"); } @Test(priority = 3) public void verifyRegisterPageHeading() { // identify header then get text WebElement heder = driver.findElement(By.xpath("//*[@id='signupForm']/h1")); String text = heder.getText(); // assertion to verify register page header assertEquals("Welcome,Register", text); Reporter.log("Verified Register Page header"); } @AfterTest public void teardown() { // quitting browser driver.quit(); } }
步骤 2 - 刷新项目,项目结构中应该会生成一个名为test-output的新文件夹。
步骤 3 − 右键点击emailable-report.html 和 index.html,选择在浏览器中打开的选项。
以下 emailable-report.html 报告应该在浏览器中打开,显示测试类名称 - TestNGTest.java,以及通过、跳过、失败的总数、测试持续时间等信息。此外,报告中还包含测试方法名称 moveToRegisterPage、verifyRegisterPageHeading 和 verifyWelcomePageHeading。
在报告的下方部分,可以查看日志消息以及测试方法名称。
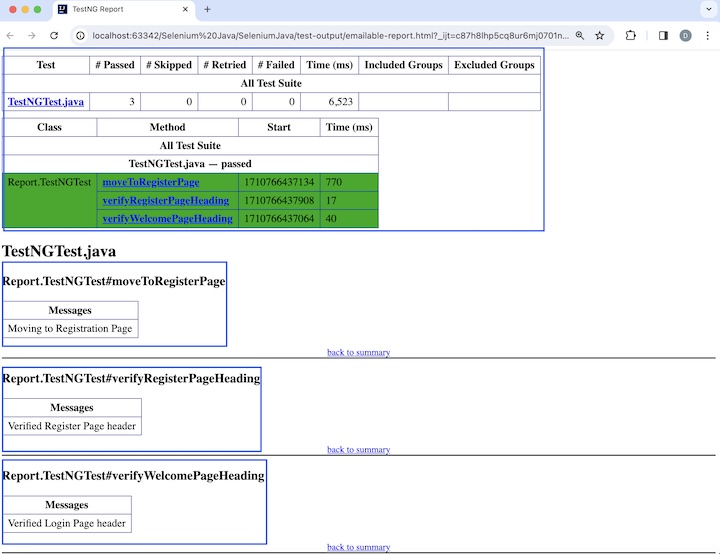
以下 index.html 报告应该在浏览器中打开,显示 testng.xml、测试数量、组、时间、报告输出、忽略的方法和按时间顺序查看。此外,结果部分包含测试方法名称 moveToRegisterPage、verifyRegisterPageHeading 和 verifyWelcomePageHeading,以及方法数量、通过和失败的数量。
点击左侧的“报告输出”选项卡,可以查看日志消息以及测试方法名称。
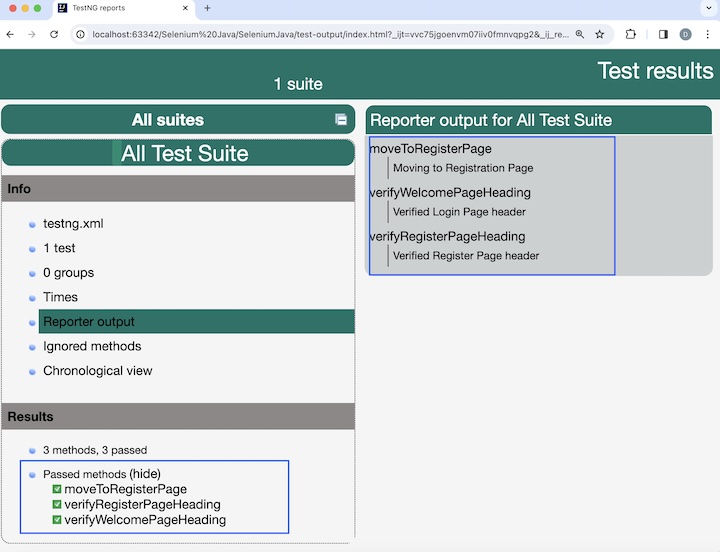
以下链接提供了 TestNG 的详细描述:TestNG
本教程到此结束,我们全面介绍了 Selenium - TestNG 报告。我们从描述 TestNG 报告、设置 TestNG 报告的先决条件开始,并逐步演示了如何创建不同类型的 TestNG 报告,例如 emailable-report.html、index.html 以及使用 Reporter 类的报告,并通过示例说明了如何将它们与 Selenium 结合使用。这使您深入了解了 TestNG 报告。建议您不断练习所学知识,并探索与 Selenium 相关的其他内容,以加深理解并扩展视野。