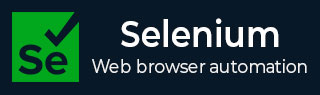
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理IFrames
- Selenium - 处理Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键上/键下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 捕获屏幕截图
- Selenium - 捕获视频
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 键盘事件
Selenium WebDriver 可用于执行键盘事件操作,例如键上、键下、在中间输入多个字符以及使用 Actions 类执行复制和粘贴操作。`keyUp()`、`keyDown()` 和 `sendKeys()` 方法用于执行这些操作。
Actions 类中键盘事件的基本方法
Actions 类中有多种方法可以执行键盘事件。有关这些方法的更多信息,请参考以下链接:
- keyDown(CharSequence key) − 此方法用于执行修饰键按下,作为参数传递。
- keyDown(WebElement e, CharSequence key) − 此方法用于在聚焦元素后执行修饰键按下。WebElement e 和要按下的键作为参数传递。
- keyUp(CharSequence key) − 此方法用于执行修饰键释放,作为参数传递。
- keyUp(WebElement e, CharSequence key) − 此方法用于在聚焦元素后执行修饰键释放。WebElement e 和要释放的键作为参数传递。
- sendKeys(CharSequence key) − 此方法用于将键发送到焦点元素。要发送的键作为参数传递。
- sendKeys(WebElement e, CharSequence key) − 此方法用于将键发送到作为参数传递的 WebElement。
- build() − 此方法用于创建一个包含所有要执行的操作的动作组合。
- perform() − 此方法用于在不先调用 build() 的情况下执行操作。
请注意,在使用Actions 类的方法时,我们需要添加导入语句:
import org.openqa.selenium.interactions.Actions in our tests.
示例 1 - 复制粘贴任务
让我们来看一下下面的页面示例,我们首先在第一个输入框(已突出显示)中,在全名:旁边输入文本 - Selenium,然后将相同的文本复制并粘贴到姓氏:旁边的另一个输入框(已突出显示)中。

语法
WebDriver driver = new ChromeDriver(); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // enter some text e.sendKeys("Selenium"); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // object of Actions class to copy then paste Actions a = new Actions(driver); a.keyDown(k); a.sendKeys("a"); a.keyUp(k); a.build().perform(); // Actions class methods to copy text a.keyDown(k); a.sendKeys("c"); a.keyUp(k); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(k); a.sendKeys("v"); a.keyUp(k); a.build().perform(); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("<value of xpath>")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text);
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class CopyPasteAction { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/register.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='firstname']")); // enter some text e.sendKeys("Selenium"); // chose the key as per platform Keys k = Platform.getCurrent().is(Platform.MAC) ? Keys.COMMAND : Keys.CONTROL; // object of Actions class to copy then paste Actions a = new Actions(driver); a.keyDown(k); a.sendKeys("a"); a.keyUp(k); a.build().perform(); // Actions class methods to copy text a.keyDown(k); a.sendKeys("c"); a.keyUp(k); a.build().perform(); // Action class methods to tab and reach to next input box a.sendKeys(Keys.TAB); a.build().perform(); // Actions class methods to paste text a.keyDown(k); a.sendKeys("v"); a.keyUp(k); a.build().perform(); // Identify the second input box with xpath locator WebElement s = driver.findElement(By.xpath("//*[@id='lastname']")); // Getting text in the second input box String text = s.getAttribute("value"); System.out.println("Value copied and pasted: " + text); // Closing browser driver.quit(); } }
输出
Value copied and pasted: Selenium Process finished with exit code 0
在上面的示例中,我们首先在第一个输入框中输入文本Selenium,然后将相同的文本复制并粘贴到第二个输入框中。
最后,我们在控制台中收到输入到第二个输入框中的文本消息 - 已复制并粘贴的值:Selenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
示例 2 - 在焦点输入框中输入文本
让我们来看另一个示例,我们将在全名:旁边的焦点输入框中输入文本Selenium。

语法
WebDriver driver = new ChromeDriver(); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // object of Actions class to input text in focus Actions a = new Actions(driver); a.click(e).sendKeys("Selenium").build().perform();
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class EnterTextInFocus { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/text-box.php"); // Identify the first input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='fullname']")); // object of Actions class to input text in focus Actions a = new Actions(driver); a.click(e).sendKeys("Selenium").build().perform(); // Getting text in the second input box String text = e.getAttribute("value"); System.out.println("Value entered to input box in focus: " + text); // Closing browser driver.quit(); } }
输出
Value entered to input box in focus: Selenium Process finished with exit code 0
在上面的示例中,我们首先在焦点输入框中输入文本Selenium,然后在控制台中收到文本消息 - 输入到焦点输入框中的值:Selenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
示例 3 - 在输入框中输入文本
让我们来看另一个示例,我们将在姓名:旁边的指定输入框中输入文本Selenium。

语法
WebDriver driver = new ChromeDriver(); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("<value of xpath>")); // object of Actions class to input text in focus Actions a = new Actions(driver); a.sendKeys(e, "Selenium").build().perform();
代码实现
package org.example; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.interactions.Actions; import java.util.concurrent.TimeUnit; public class EnterText { public static void main(String[] args) throws InterruptedException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // object of Actions class to input text Actions a = new Actions(driver); a.sendKeys(e,"Selenium").build().perform(); // Getting text in the second input box String text = e.getAttribute("value"); System.out.println("Value entered to input box: " + text); // Closing browser driver.quit(); } }
输出
Value entered to input box: Selenium Process finished with exit code 0
在上面的示例中,我们在输入框中输入了文本Selenium,然后在控制台中收到文本消息 - 输入到输入框中的值:Selenium。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
结论
本教程全面介绍了 Selenium WebDriver 键盘事件。我们首先介绍了 Actions 类中键盘事件的基本方法,并通过示例说明了如何在 Selenium WebDriver 中处理键盘事件。这使您能够深入了解 Selenium WebDriver 键盘事件。明智的做法是不断练习所学内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。