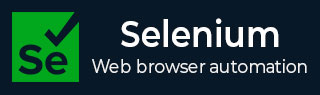
- Selenium 教程
- Selenium - 首页
- Selenium - 概述
- Selenium - 组件
- Selenium - 自动化测试
- Selenium - 环境搭建
- Selenium - 远程控制
- Selenium IDE 教程
- Selenium - IDE 简介
- Selenium - 功能特性
- Selenium - 限制
- Selenium - 安装
- Selenium - 创建测试
- Selenium - 创建脚本
- Selenium - 控制流
- Selenium - 存储变量
- Selenium - 警报和弹出窗口
- Selenium - Selenese 命令
- Selenium - Actions 命令
- Selenium - Accessors 命令
- Selenium - Assertions 命令
- Selenium - Assert/Verify 方法
- Selenium - 定位策略
- Selenium - 脚本调试
- Selenium - 验证点
- Selenium - 模式匹配
- Selenium - JSON 数据文件
- Selenium - 浏览器执行
- Selenium - 用户扩展
- Selenium - 代码导出
- Selenium - 代码输出
- Selenium - JavaScript 函数
- Selenium - 插件
- Selenium WebDriver 教程
- Selenium - 简介
- Selenium WebDriver vs RC
- Selenium - 安装
- Selenium - 第一个测试脚本
- Selenium - 驱动程序会话
- Selenium - 浏览器选项
- Selenium - Chrome 选项
- Selenium - Edge 选项
- Selenium - Firefox 选项
- Selenium - Safari 选项
- Selenium - 双击
- Selenium - 右击
- Python 中的 HTML 报告
- 处理编辑框
- Selenium - 单个元素
- Selenium - 多个元素
- Selenium Web 元素
- Selenium - 文件上传
- Selenium - 定位器策略
- Selenium - 相对定位器
- Selenium - 查找器
- Selenium - 查找所有链接
- Selenium - 用户交互
- Selenium - WebElement 命令
- Selenium - 浏览器交互
- Selenium - 浏览器命令
- Selenium - 浏览器导航
- Selenium - 警报和弹出窗口
- Selenium - 处理表单
- Selenium - 窗口和标签页
- Selenium - 处理链接
- Selenium - 输入框
- Selenium - 单选按钮
- Selenium - 复选框
- Selenium - 下拉框
- Selenium - 处理 iframe
- Selenium - 处理 Cookie
- Selenium - 日期时间选择器
- Selenium - 动态 Web 表格
- Selenium - Actions 类
- Selenium - Action 类
- Selenium - 键盘事件
- Selenium - 键向上/向下
- Selenium - 复制和粘贴
- Selenium - 处理特殊键
- Selenium - 鼠标事件
- Selenium - 拖放
- Selenium - 笔事件
- Selenium - 滚动操作
- Selenium - 等待策略
- Selenium - 显式/隐式等待
- Selenium - 支持功能
- Selenium - 多选
- Selenium - 等待支持
- Selenium - 选择支持
- Selenium - 颜色支持
- Selenium - ThreadGuard
- Selenium - 错误和日志记录
- Selenium - 异常处理
- Selenium - 其他
- Selenium - 处理 Ajax 调用
- Selenium - JSON 数据文件
- Selenium - CSV 数据文件
- Selenium - Excel 数据文件
- Selenium - 跨浏览器测试
- Selenium - 多浏览器测试
- Selenium - 多窗口测试
- Selenium - JavaScript 执行器
- Selenium - 无头执行
- Selenium - 截图捕获
- Selenium - 视频捕获
- Selenium - 页面对象模型
- Selenium - 页面工厂
- Selenium - 录制和回放
- Selenium - 框架
- Selenium - 浏览上下文
- Selenium - DevTools
- Selenium Grid 教程
- Selenium - 概述
- Selenium - 架构
- Selenium - 组件
- Selenium - 配置
- Selenium - 创建测试脚本
- Selenium - 测试执行
- Selenium - 端点
- Selenium - 自定义节点
- Selenium 报告工具
- Selenium - 报告工具
- Selenium - TestNG
- Selenium - JUnit
- Selenium - Allure
- Selenium & 其他技术
- Selenium - Java 教程
- Selenium - Python 教程
- Selenium - C# 教程
- Selenium - Javascript 教程
- Selenium - Kotlin 教程
- Selenium - Ruby 教程
- Selenium - Maven 和 Jenkins
- Selenium - 数据库测试
- Selenium - LogExpert 日志记录
- Selenium - Log4j 日志记录
- Selenium - Robot Framework
- Selenium - AutoIT
- Selenium - Flash 测试
- Selenium - Apache Ant
- Selenium - Github 教程
- Selenium - SoapUI
- Selenium - Cucumber
- Selenium - IntelliJ
- Selenium - XPath
- Selenium 其他概念
- Selenium - IE 驱动程序
- Selenium - 自动化框架
- Selenium - 关键字驱动框架
- Selenium - 数据驱动框架
- Selenium - 混合驱动框架
- Selenium - SSL 证书错误
- Selenium - 替代方案
- Selenium 有用资源
- Selenium - 问答
- Selenium - 快速指南
- Selenium - 有用资源
- Selenium - 自动化实践
- Selenium - 讨论
Selenium WebDriver - 截图捕获
Selenium WebDriver 可用于在运行自动化步骤时捕获网页的屏幕截图。屏幕截图是借助TakeScreenshot方法捕获的。
此方法指示 Selenium WebDriver 捕获屏幕截图。要在所需位置捕获屏幕截图,我们需要使用getScreenshotAs()方法。
网页上的元素识别
右键单击网页,然后单击 Chrome 浏览器中的“检查”按钮。然后,将提供整个页面的相应 HTML 代码。要检查页面上的编辑框,请单击可见 HTML 代码顶部的左上方箭头。
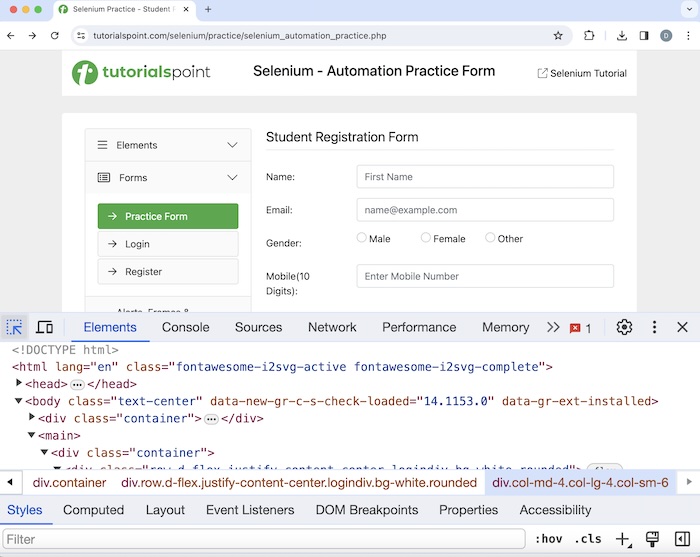
单击并将箭头指向编辑框(如下面的图像中突出显示的部分)后,其 HTML 代码将显示,反映输入标记名称。
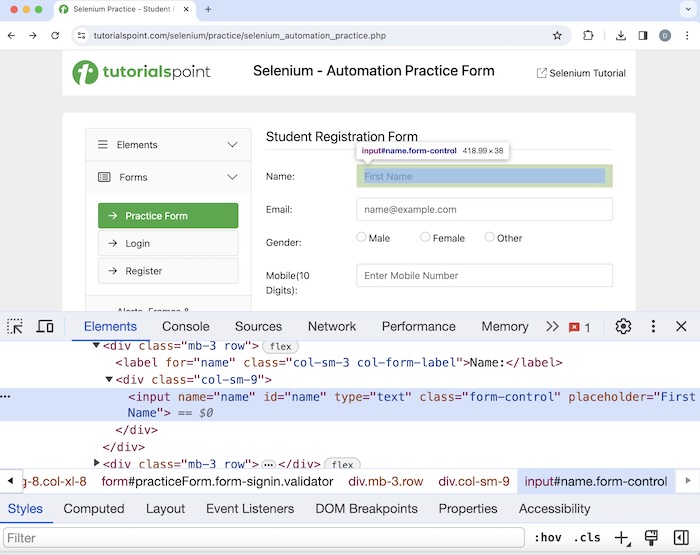
示例 1 - 捕获整个页面的屏幕截图
让我们以上面页面为例,我们首先使用sendKeys()方法在输入框中输入一些文本,然后捕获整个网页的屏幕截图。
package org.example; import org.apache.commons.io.FileUtils; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ScreenshotCapture { public static void main(String[] args) throws InterruptedException, IOException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // Get the value entered String text = e.getAttribute("value"); System.out.println("Entered text is: " + text); // take full screenshot and store in project location File scrFile = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE); FileUtils.copyFile(scrFile, new File("./ImageFullPage.png")); // Closing browser driver.quit(); } }
在 pom.xml 文件中添加的依赖项:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>SeleniumJava</artifactId> <version>1.0-SNAPSHOT</version> <properties> <maven.compiler.source>16</maven.compiler.source> <maven.compiler.target>16</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependencies> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.11.0</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.12.0</version> </dependency> </dependencies> </project>
在项目目录中捕获了一个名为ImageFullPage.png的屏幕截图。单击它,我们将获得整个网页的捕获屏幕截图。
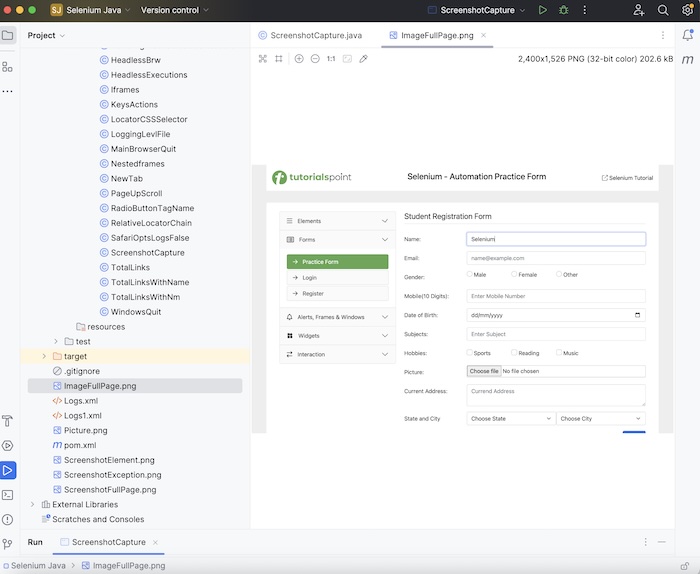
示例 2 - 捕获单个元素的屏幕截图
让我们使用与上面讨论的相同的示例,我们首先在编辑框中输入一些文本,然后仅捕获该元素的屏幕截图。这可以使用getScreenShotAs()方法完成。
package org.example; import org.apache.commons.io.FileUtils; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ScreenshotCaptureElement { public static void main(String[] args) throws InterruptedException, IOException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); // Identify the input box with xpath locator WebElement e = driver.findElement (By.xpath("//*[@id='name']")); // enter text in input box e.sendKeys("Selenium"); // Get the value entered String text = e.getAttribute("value"); System.out.println("Entered text is: " + text); // take screenshot of input box and store in project location File scrFile = e.getScreenshotAs(OutputType.FILE); FileUtils.copyFile(scrFile, new File("./ImageElement.png")); // Closing browser driver.quit(); } }
在项目目录中捕获了一个名为ImageElement.png的屏幕截图。单击它,我们将仅获得输入框(我们在其中输入文本Selenium)的捕获屏幕截图。
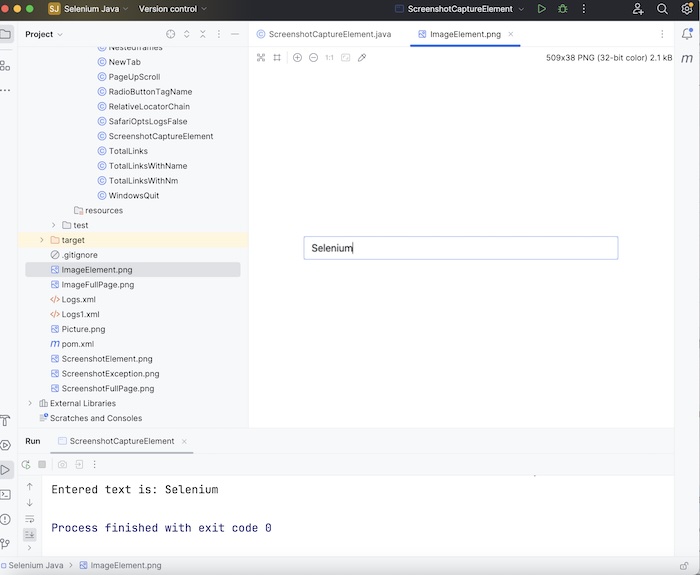
示例 3 - 在异常时捕获屏幕截图
让我们使用与上面讨论的相同的示例,我们尝试在输入框中输入一些文本,然后仅在该步骤失败时捕获该步骤的屏幕截图。这可以使用添加到 catch 块(通常用于异常处理)中的getScreenShotAs()方法完成。
package org.example; import org.apache.commons.io.FileUtils; import org.openqa.selenium.*; import org.openqa.selenium.chrome.ChromeDriver; import java.io.File; import java.io.IOException; import java.util.concurrent.TimeUnit; public class ScreenshotCaptureException { public static void main(String[] args) throws InterruptedException, IOException { // Initiate the Webdriver WebDriver driver = new ChromeDriver(); // adding implicit wait of 15 secs driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS); // Opening the webpage where we will identify an element driver.get("https://tutorialspoint.com/selenium/practice/selenium_automation_practice.php"); try { // Identify the input box with xpath locator WebElement e = driver.findElement(By.xpath("//*[@id='names']")); // enter text in input box e.sendKeys("Selenium"); System.out.println("Step successfully executed"); } catch(Exception e) { // take screenshot on failure and store in project location File scrFile = ((TakesScreenshot) driver). getScreenshotAs(OutputType.FILE); FileUtils.copyFile(scrFile, new File("./ImageException.png")); System.out.println("Element could not be found - Step failed"); } // Closing browser driver.quit(); } }
输出
Element could not be found - Step failed Process finished with exit code 0
在上面的示例中,我们的测试启动了一个网页并尝试与一个元素进行交互。在无法识别元素后,执行流程转移到 catch 块,我们在控制台中收到消息 - 找不到元素 - 步骤失败。
最后,收到消息进程已完成,退出代码为 0,表示代码已成功执行。
此外,在项目目录中捕获了一个名为ImageException.png的屏幕截图。单击它,我们将获得失败测试步骤的捕获屏幕截图(这意味着 Selenium 没有在输入框中输入)。
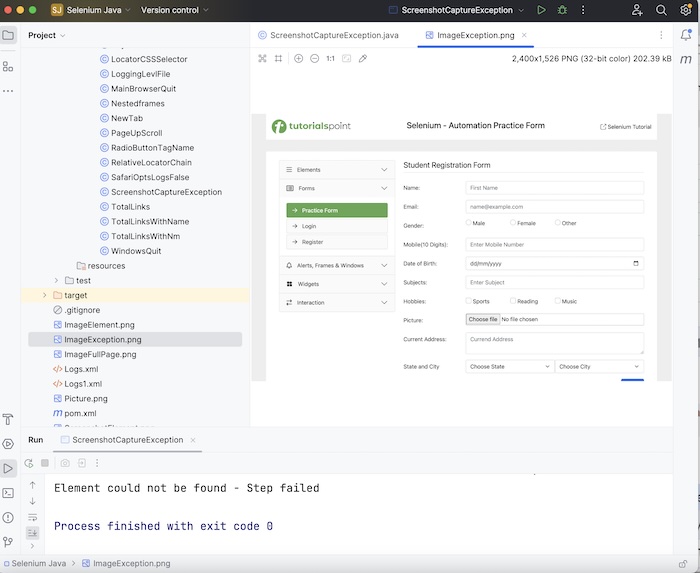
结论
本教程全面介绍了 Selenium WebDriver 截图捕获。我们从描述网页上的元素标识开始,并逐步讲解了如何使用 Selenium WebDriver 捕获屏幕截图的示例。这使您能够深入了解 Selenium WebDriver 截图捕获。最好继续练习您所学的内容,并探索与 Selenium 相关的其他内容,以加深您的理解并拓宽您的视野。